What is Ruby on Rails?
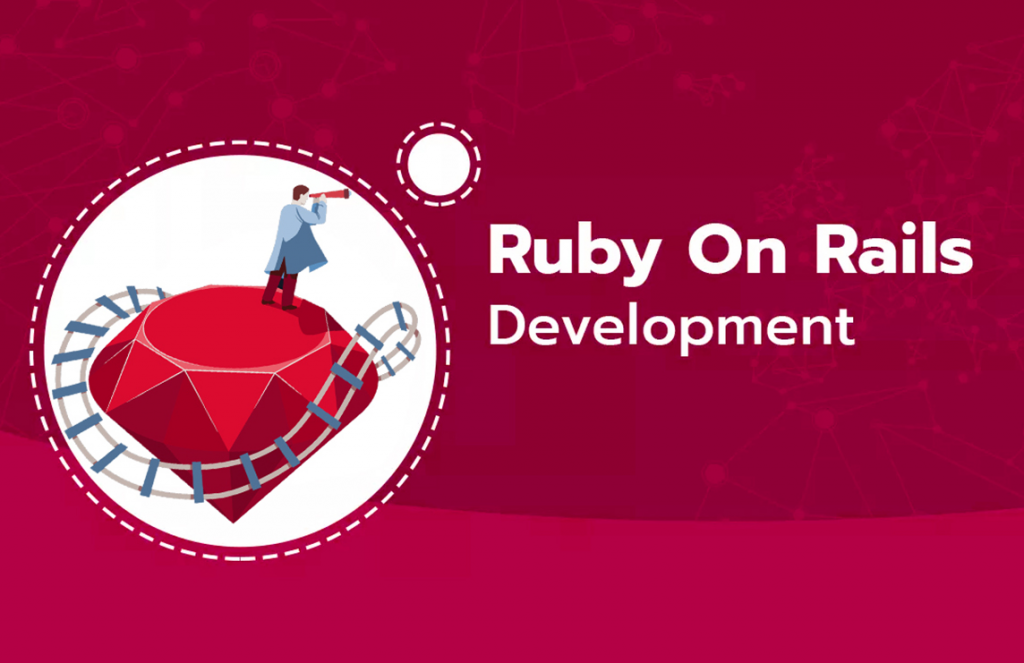
Ruby on Rails, often referred to simply as Rails, is an open-source web application framework written in the Ruby programming language. It follows the Model-View-Controller (MVC) architectural pattern and is designed to make web application development faster and more straightforward by providing a set of conventions and best practices. Rails was created by David Heinemeier Hansson and released in 2005.
What is top use cases of Ruby on Rails?
- Web Application Development: Ruby on Rails is commonly used for developing web applications, ranging from small startups to large-scale, enterprise-grade applications. Its convention-driven approach streamlines development and promotes rapid prototyping.
- Content Management Systems (CMS): Rails is used to build content management systems and publishing platforms, allowing users to create, edit, and manage website content easily.
- E-commerce Websites: Ruby on Rails is suitable for building e-commerce websites and online stores. Developers can leverage existing e-commerce libraries and frameworks built on Rails to speed up development.
- Social Networking Sites: Rails has been used to develop social networking platforms and community websites, providing the necessary features for user registration, authentication, and interaction.
- Marketplace Platforms: Rails is chosen for building online marketplaces that connect buyers and sellers, enabling transactions, product listings, and payment processing.
- Real-Time Chat Applications: Rails can be used as a backend for real-time chat and messaging applications when combined with technologies like WebSockets and Action Cable.
- API Development: Ruby on Rails is suitable for creating RESTful APIs, making it a solid choice for building backends that power mobile apps and single-page applications.
- SaaS Applications: Software as a Service (SaaS) providers often use Rails to develop their applications due to its flexibility and scalability. Rails can handle multi-tenancy and user management effectively.
- Educational Platforms: Rails is used to create online learning management systems, course platforms, and educational websites that offer content delivery, assessments, and user tracking.
- Healthcare Solutions: Rails is employed in healthcare applications for patient management, medical records, appointment scheduling, and telemedicine platforms.
- Project Management Tools: Ruby on Rails is used to develop project management and collaboration tools that help teams organize tasks, share documents, and track progress.
- Financial Services: Some financial technology (FinTech) companies use Rails to build applications for online banking, financial planning, and investment management.
Ruby on Rails has a strong and active community, and its philosophy of convention over configuration has made it a popular choice for startups and organizations looking to develop web applications rapidly and efficiently. Its flexibility and scalability make it suitable for a wide range of web development projects.
What are the features of Ruby on Rails?
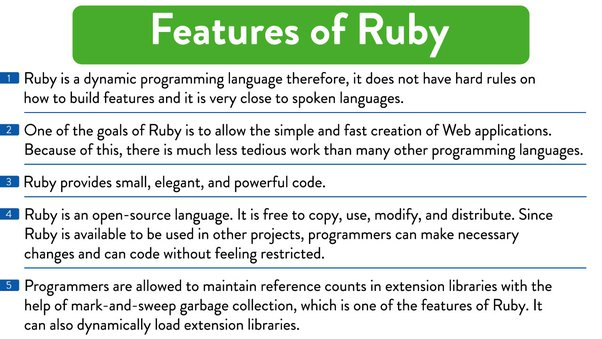
Ruby on Rails (often referred to as Rails) is a popular web application framework known for its developer-friendly features and conventions that emphasize simplicity and productivity. Here are some of the key features of Ruby on Rails:
- Convention over Configuration (CoC): Rails follows the principle of convention over configuration, which means it provides sensible defaults and conventions for various aspects of web development. This minimizes the need for extensive configuration, allowing developers to focus on writing application code.
- Don’t Repeat Yourself (DRY): Rails encourages the DRY principle, aiming to eliminate redundancy in code. Reusable components and the use of partials and helpers help achieve this goal.
- Model-View-Controller (MVC) Architecture: Rails adheres to the MVC architectural pattern, separating an application’s concerns into three distinct layers:
- Model: Represents the data and business logic, often connected to a database.
- View: Handles the presentation logic and rendering of data.
- Controller: Manages user interactions, processes requests, and coordinates between the model and view.
- Active Record: Rails includes the Active Record library, which simplifies database interactions by mapping database tables to Ruby objects. This allows developers to work with databases using Ruby code and follows the Object-Relational Mapping (ORM) pattern.
- RESTful Routing: Rails promotes RESTful routing, aligning URL structures with the HTTP methods (GET, POST, PUT, DELETE) to create clean and logical routes. This makes it easy to design RESTful APIs.
- Scaffolding: Rails provides scaffolding generators that create boilerplate code for common tasks like creating, reading, updating, and deleting records (CRUD operations). This accelerates the development of basic functionality.
- Asset Pipeline: Rails includes an asset pipeline for managing and optimizing assets like JavaScript and CSS. It facilitates asset organization, concatenation, minification, and versioning to improve performance.
- Testing Framework: Rails offers a built-in testing framework that supports unit tests, integration tests, and functional tests. This promotes test-driven development (TDD) and ensures code quality.
- Strong Community and Ecosystem: Ruby on Rails has a vibrant community and an extensive ecosystem of gems (libraries) that extend its functionality. Many open-source projects and resources are available for Rails developers.
- Security Features: Rails includes built-in security features to protect against common web application vulnerabilities, such as Cross-Site Scripting (XSS), Cross-Site Request Forgery (CSRF), and SQL injection.
- Internationalization (I18n) and Localization (L10n): Rails provides robust support for handling internationalization and localization, making it easy to create multilingual applications.
- Dependency Management: Rails uses the Bundler tool for dependency management, allowing developers to specify and manage gem dependencies easily.
What is the workflow of Ruby on Rails?
The workflow of developing a web application with Ruby on Rails typically follows these steps:
- Project Initialization: Create a new Rails project using the
rails new
command. This sets up the initial project structure and configuration. - Database Setup: Configure the database connection and create database tables using migrations. Rails supports multiple database systems, such as SQLite, PostgreSQL, MySQL, and more.
- Model Creation: Define models to represent the data structures of the application. Models often include validations, associations, and methods for working with data.
- Controller and Routes: Create controllers to handle user requests and define routes in the
config/routes.rb
file to map URLs to controller actions. Use RESTful routing conventions to maintain consistency. - Views: Create view templates (HTML with embedded Ruby code) to render the user interface. Views often use layout templates and partials for code reuse.
- Scaffolding or Custom Code: Depending on the project’s complexity, you can use scaffolding to generate basic CRUD functionality. For custom features, write controllers and views manually.
- Asset Management: Manage JavaScript, CSS, and other assets using the asset pipeline. Organize assets in the appropriate directories and use asset tags in views.
- Authentication and Authorization: Implement user authentication and authorization as needed. Gems like Devise are commonly used for authentication.
- Testing: Write tests for models, controllers, and views to ensure functionality and catch regressions. Run tests using tools like RSpec or MiniTest.
- Deployment: Deploy the application to a hosting platform or server. Popular deployment options include Heroku, AWS, DigitalOcean, and traditional web hosting.
- Monitoring and Maintenance: Monitor the application’s performance and security. Perform regular maintenance, including updates to Rails and gem dependencies.
- Scaling: As the application grows, scale it horizontally or vertically to handle increased traffic and data volume.
- Documentation and User Guides: Document the application’s features and usage for developers and end-users. This helps with onboarding and support.
- Continuous Integration and Deployment (CI/CD): Implement CI/CD pipelines to automate testing, building, and deployment processes for smoother development workflows.
- Community and Support: Engage with the Ruby on Rails community for support, advice, and collaboration. Attend Rails meetups and conferences to stay up-to-date with best practices and trends.
The Rails workflow emphasizes rapid development, code organization, and adherence to conventions, making it well-suited for building web applications efficiently. The framework’s comprehensive ecosystem and strong community support contribute to its popularity among developers.
How Ruby on Rails Works & Architecture?
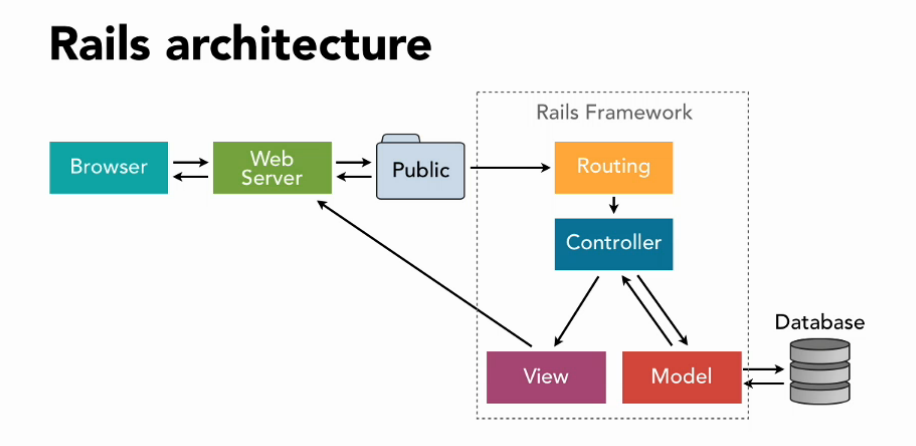
Ruby on Rails, also known as Rails, is an open-source web application framework written in the Ruby programming language. It is a popular framework for developing web applications because it is:
- Mature: Ruby on Rails has been around for over 15 years and is used by many large companies, such as Airbnb, GitHub, and Shopify.
- Fast: Ruby on Rails is known for its performance.
- Extensible: Ruby on Rails is highly extensible, so you can customize it to fit your needs.
- Secure: Ruby on Rails has a number of security features built-in.
Ruby on Rails applies the MVC (Model-View-Controller) architectural pattern. The MVC pattern separates the application into three layers:
- The model layer is responsible for storing and retrieving data.
- The view layer is responsible for presenting data to the user.
- The controller layer is responsible for handling user requests and communicating with the model and view layers.
How to Install and Configure Ruby on Rails?
To install and configure Ruby on Rails, you can follow these steps:
- Install Ruby. You can find instructions on how to install Ruby on the Ruby website: https://www.ruby-lang.org/en/documentation/installation/
- Install Rails. You can install Rails using the following command:
gem install rails
- Create a new Rails application. You can create a new Rails application using the following command:
rails new my_app
This will create a new directory called my_app
with all the files you need to start developing a Rails application.
- Run the Rails server. You can run the Rails server using the following command:
rails server
This will start the Rails server on port 3000. You can then access your Rails application at http://localhost:3000.
Fundamental Tutorials of Ruby on Rails: Getting Started Step by Step
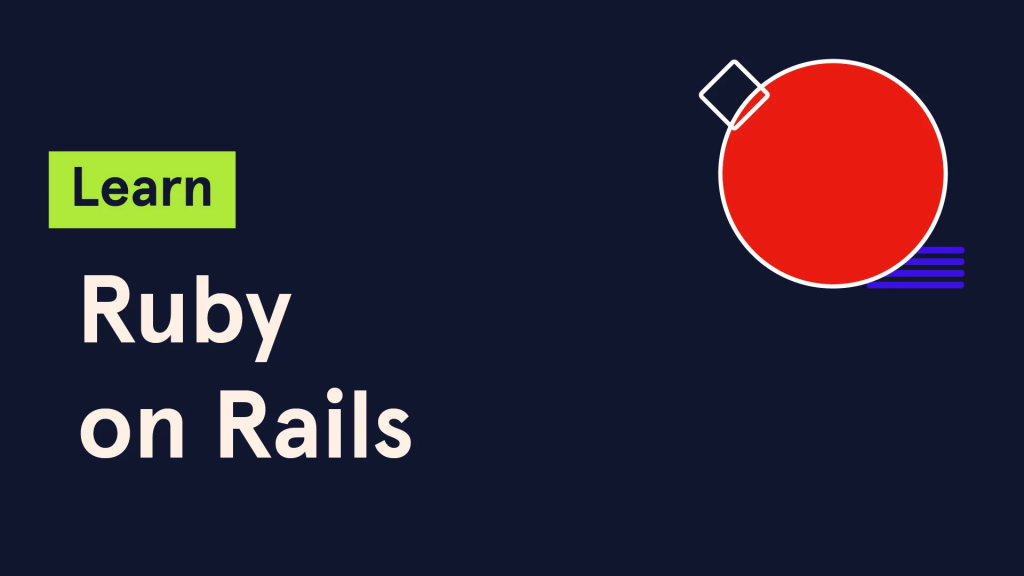
The following are the step-by-step fundamental tutorials of Ruby on Rails:
- Install Ruby and Rails
To install Ruby and Rails, you can follow these steps:
1. Install Ruby. You can find instructions on how to install Ruby on the Ruby
website: https://www.ruby-lang.org/en/documentation/installation/
2. Install Rails. You can install Rails using the following command:
gem install rails
- Create a new Rails application
To create a new Rails application, you can follow these steps:
1. Create a new directory for your Rails application.
2. In the directory, run the following command:
rails new my_app
This will create a new directory called my_app
with all the files you need to start developing a Rails application.
- Run the Rails server
To run the Rails server, you can follow these steps:
1. In the directory of your Rails application, run the following command:
rails server
This will start the Rails server on port 3000. You can then access your Rails application at http://localhost:3000.
- Create a controller
A controller is responsible for handling user requests and communicating with the model and view layers. To create a controller, you can follow these steps:
1. In the directory of your Rails application, create a new file called
app/controllers/articles_controller.rb`.
2. In the file, add the following code:
Ruby
class ArticlesController < ApplicationController
def index
@articles = Article.all
end
end
This code creates a controller called ArticlesController
with an index
action. The index
action will retrieve all the articles from the database and store them in an instance variable called @articles
.
- Create a view
A view is responsible for displaying data to the user. To create a view, you can follow these steps:
1. In the directory of your Rails application, create a new file called
app/views/articles/index.html.erb`.
2. In the file, add the following code:
<h1>Articles</h1>
<ul>
<% @articles.each do |article| %>
<li><%= article.title %></li>
<% end %>
</ul>
This code creates a view called articles/index.html.erb
which displays a list of all the articles in the database.
- Create a model
A model is responsible for storing and retrieving data. To create a model, you can follow these steps:
1. In the directory of your Rails application, create a new file called
app/models/article.rb`.
2. In the file, add the following code:
class Article < ApplicationRecord
validates :title, presence: true
validates :content, presence: true
end
This code creates a model called Article
with two attributes: title
and content
. The validates
method ensures that the title
and content
attributes are not empty.
- Tips on How to Become a DevOps Engineer - April 28, 2024
- Computer Programming Education Requirements – What You Need to Know - April 28, 2024
- The Role of Big Data in Higher Education - April 28, 2024