What is Scala?
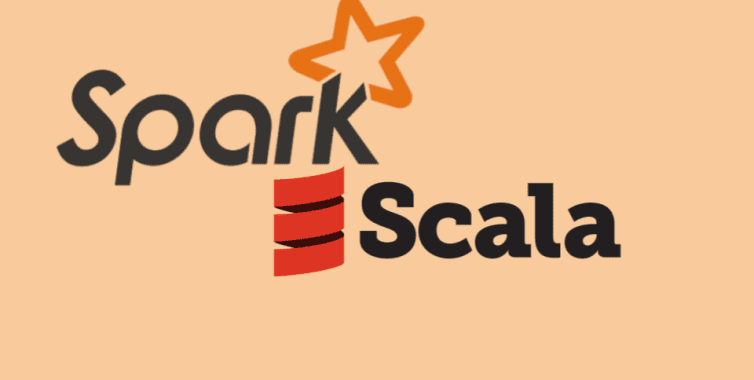
Scala is a general-purpose programming language that mixes object-oriented and functional programming paradigms. It was designed to be concise, elegant, and to integrate seamlessly with existing Java code, allowing developers to leverage the extensive Java ecosystem while enjoying the expressiveness of a modern programming language. Scala runs on the Java Virtual Machine (JVM) and is statically typed.
Key Features of Scala:
- Object-Oriented and Functional:
- Scala is a hybrid language that favors both object-oriented and functional programming. It allows developers to define classes and objects while also providing powerful functional programming constructs.
- Static Typing:
- Scala is statically typed, meaning that types are checked at compile-time, reducing the likelihood of runtime errors.
- Concurrency and Parallelism:
- Scala has built-in favor for concurrent and parallel programming. The language includes features like actors and the Akka toolkit, making it well-suited for building concurrent and distributed systems.
- Immutable Collections:
- Scala encourages the use of immutable data structures, which contributes to better code quality, concurrency support, and functional programming practices.
- Pattern Matching:
- Scala includes powerful pattern matching capabilities, allowing developers to write expressive and concise code for tasks such as data extraction and case analysis.
- Type Inference:
- Scala features strong type inference, allowing developers to write code with fewer explicit type annotations while still benefiting from static typing.
- Integration with Java:
- Scala is fully interoperable with Java. Scala code can call Java libraries and vice versa, making it easy to leverage existing Java code and libraries.
- Expressive Syntax:
- Scala’s syntax is designed to be concise and expressive, promoting readability and reducing boilerplate code.
- Functional Composition:
- Scala provides powerful constructs for functional composition, allowing developers to create expressive and modular functional code.
- Tooling:
- Scala has good tooling support, including build tools like sbt and integrated development environments (IDEs) such as IntelliJ IDEA and Eclipse.
What is top use cases of Scala?
Top Use Cases of Scala:
- Web Development:
- Scala is used for building web applications and RESTful APIs. Frameworks like Play Framework and Akka HTTP are popular choices for Scala web development.
- Big Data Processing:
- Scala, along with the Apache Spark framework, is widely used for big data processing and analytics. The expressive syntax and functional programming features make it well-suited for writing distributed data processing applications.
- Concurrency and Distributed Systems:
- Scala’s support for concurrency, along with frameworks like Akka, makes it suitable for building concurrent and distributed systems. Akka actors provide a powerful model for building scalable and fault-tolerant distributed applications.
- Functional Programming:
- Scala is often chosen for projects that leverage functional programming paradigms. Its support for immutability, pattern matching, and higher-order functions makes it well-suited for functional programming practices.
- Middleware Development:
- Scala is used for building middleware components and services. Its integration with Java allows developers to work seamlessly with Java-based middleware and enterprise systems.
- Data Science:
- Scala is increasingly used in data science and machine learning applications. Libraries like Breeze and ScalaNLP provide numerical and machine learning capabilities in Scala.
- Financial Applications:
- Scala is employed in the finance industry for building applications related to algorithmic trading, risk management, and financial analytics.
- Command-Line Tools and Utilities:
- Scala’s concise syntax and expressive features make it suitable for building command-line tools and utilities.
- Education:
- Scala is used in academic settings for teaching programming concepts, thanks to its blend of object-oriented and functional programming features.
- Microservices Architecture:
- Scala, often combined with Akka, is used for building microservices architectures. Its support for concurrency and distributed systems aligns well with the requirements of microservices.
Scala’s versatility, expressiveness, and compatibility with Java have contributed to its adoption in a variety of application domains. Whether in web development, big data processing, or distributed systems, Scala provides a powerful and modern programming language option for developers.
What are feature of Scala?
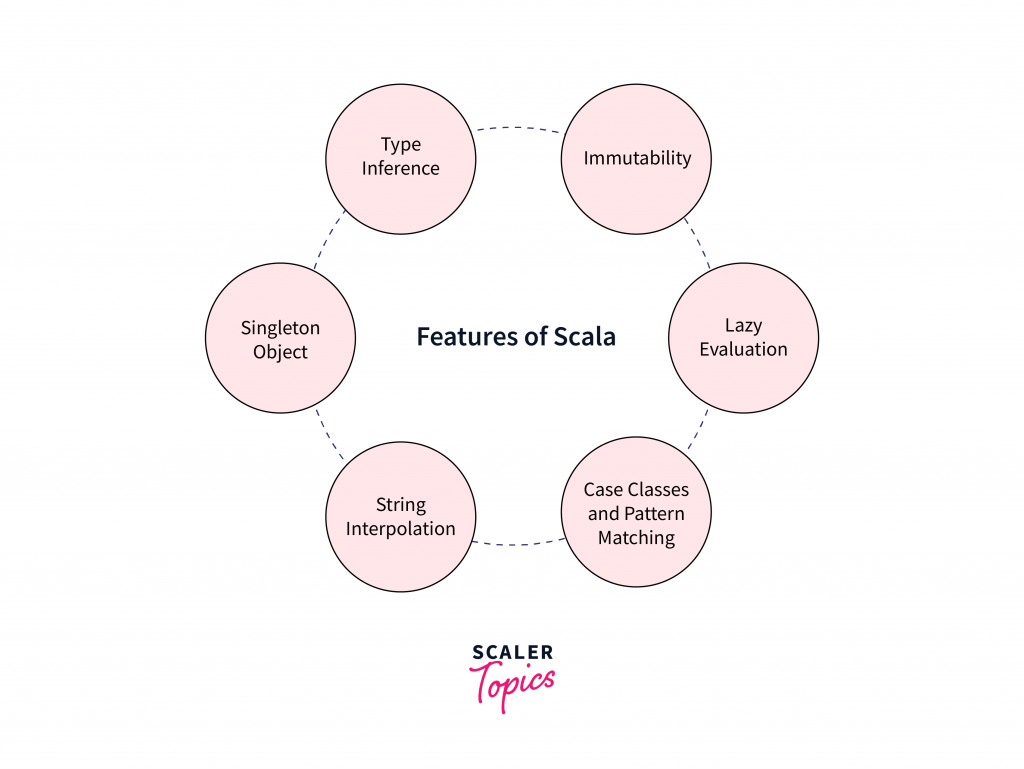
Scala is a versatile programming language that incorporates features from both object-oriented and functional programming paradigms. Let’s have a look at some key features of Scala:
- Object-Oriented:
- Scala is a pure object-oriented language, and everything in Scala is an object. It supports classes and objects, inheritance, and other principles of object-oriented programming.
- Functional Programming:
- Scala embraces functional programming concepts, including immutability, first-class functions, pattern matching, and higher-order functions.
- Static Typing:
- Scala is statically typed, which means that types are checked at compile-time, providing early error detection and improved code quality.
- Concurrency and Parallelism:
- Scala favors built-in support for concurrent and parallel programming. It includes libraries and constructs for managing concurrent tasks, and the Akka toolkit is widely used for building scalable, concurrent, and distributed systems.
- Type Inference:
- Scala features a powerful type inference system, allowing developers to write expressive code with fewer explicit type annotations. The compiler can often deduce types based on context.
- Pattern Matching:
- Scala includes a sophisticated pattern matching mechanism that allows developers to match complex data structures and extract values in a concise and expressive manner.
- Immutability:
- Scala encourages the use of immutable data structures, promoting safer and more predictable code. Immutable collections are a fundamental part of Scala.
- Expressive Syntax:
- Scala’s syntax is designed to be expressive and concise, reducing boilerplate code and enhancing code readability.
- Integration with Java:
- Scala is interoperable with Java, permitting seamless integration with existing Java code and libraries. Java libraries can be used directly in Scala, and vice versa.
- Actor Model:
- Scala supports the Actor model for concurrent and distributed computing. The Akka framework, built in Scala, provides a powerful implementation of the Actor model for building scalable and fault-tolerant systems.
- Tooling:
- Scala has a range of tools and IDE support, including build tools like sbt, and compatibility with popular IDEs such as IntelliJ IDEA and Eclipse.
- Case Classes:
- Scala introduces case classes, which are special classes for immutable data modeling. Case classes automatically generate methods for pattern matching, equality, and more.
- Lazy Evaluation:
- Scala allows lazy evaluation of expressions, which means that computations are deferred until their results are actually needed. This can contribute to better performance in certain scenarios.
What is the workflow of Scala?
The workflow of working with Scala typically involves the following steps:
- Setting Up the Environment:
- Install the Scala compiler and optionally choose an Integrated Development Environment (IDE) such as IntelliJ IDEA or use text editors like Visual Studio Code.
2. Creating a Project:
- Start a new Scala project using a build tool like sbt (Scala Build Tool) or Maven. Define project dependencies, including any necessary Scala or Java libraries.
3. Writing Scala Code:
- Write Scala code using your chosen editor or IDE. Scala code can be organized into classes, objects, traits, and functions, blending object-oriented and functional programming concepts.
4. Compiling Code:
- Use the Scala compiler to compile your code. This step checks for syntax errors and performs type checking. The compiler produces bytecode that runs on the Java Virtual Machine (JVM).
scalac MyProgram.scala
- Running the Program:
- Execute the compiled program using the
scala
command. This step involves running the generated JVM bytecode.
scala MyProgram
- Testing:
- Write and run tests using a testing framework such as ScalaTest or JUnit. Testing is an integral part of the development process to ensure code correctness.
7. Building with sbt:
- If you’re using sbt as your build tool, you can use it to compile, run, and test your Scala project. sbt simplifies the build process and manages project dependencies.
sbt compile
sbt run
sbt test
- Integration with Java:
- Leverage Java libraries and frameworks in your Scala code seamlessly. Scala classes and objects can be used in Java, and vice versa.
9. Concurrency and Parallelism:
- Utilize Scala’s concurrency features, such as Akka actors, for building concurrent and distributed systems. Design and implement concurrent components as needed.
10. Continuous Integration:
- Set up continuous integration using tools like Jenkins, Travis CI, or GitHub Actions to automate the build, test, and deployment processes.
11. Documentation:
- Document your Scala code using comments, and consider generating documentation using tools like Scaladoc.
12. Scaling and Optimizing:
- As your project grows, optimize and refactor code as needed. Leverage profiling tools to identify bottlenecks and improve performance.
13. Collaboration and Code Reviews:
- Collaborate with team members using version control systems like Git. Conduct code reviews to ensure code quality and adherence to best practices.
14. Deployment:
- Deploy your Scala application to the desired environment, whether it’s on-premises servers, cloud platforms, or containerized environments.
15. Monitoring and Maintenance:
- Monitor your deployed application for performance and issues. Perform regular maintenance tasks and updates as needed.
This workflow is adaptable based on the specific requirements of your Scala project. Whether you’re building web applications, distributed systems, or leveraging Scala in data science, the workflow provides a general guide for developing and maintaining Scala projects.
How Scala Works & Architecture?
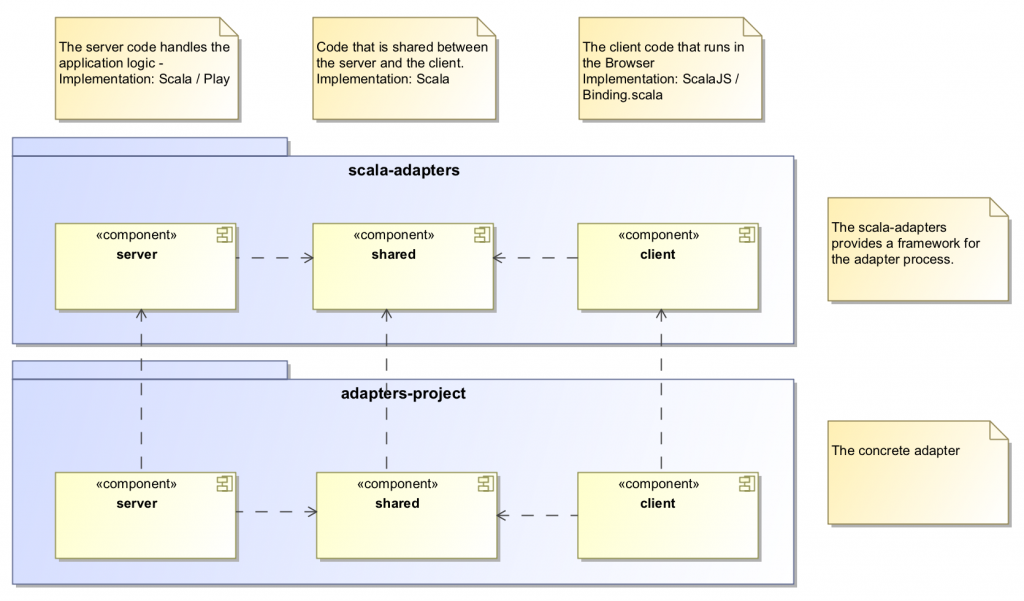
Scala, a powerful and versatile general-purpose programming language, combines the best of object-oriented and functional programming paradigms. Let’s delve into its workings and architecture:
Core features:
- Object-oriented: Encapsulation, inheritance, polymorphism are built-in.
- Functional: Immutable data structures, higher-order functions, lazy evaluation.
- Static typing: Improves code correctness and catches errors early.
- Type inference: Reduces boilerplate code and increases readability.
- Concurrency: Built-in support for parallel and asynchronous programming.
Key components:
- JVM integration: Scala compiles to bytecode that runs on the Java Virtual Machine (JVM), enabling access to vast Java libraries and frameworks.
- Actors: Lightweight, concurrent entities that communicate through asynchronous messages, promoting scalability and responsiveness.
- Scala collections: Rich and powerful library of immutable data structures, offering efficient operations and parallel processing potential.
- Implicit conversions: Automatic conversions between types, simplifying code and enhancing expressiveness.
- Traits: Reusable components that define interfaces and implementations, promoting modularity and code reuse.
Architecture:
- Layered design: Scala is divided into layers, with the core language at the bottom and libraries and frameworks built on top. This allows for independent development and updates.
- Object model: Objects are the fundamental building blocks, with attributes (fields) and methods (functions). Inheritance and polymorphism enable code reuse and specialization.
- Type system: Static typing ensures type safety and prevents runtime errors. Scala’s type system is powerful and flexible, allowing for advanced type hierarchies and generics.
- Concurrency model: Actors provide a message-passing model for concurrent programming, facilitating scalable and responsive applications.
Benefits:
- Expressive and concise: Scala’s features like pattern matching and closures allow for concise and readable code.
- Scalable and performant: Actor-based concurrency and efficient collections make Scala suitable for large-scale systems.
- Versatile: Applicable to various domains, including web development, data processing, machine learning, and more.
How to Install and Configure Scala?
Installing and configuring Scala depends on your operating system and preferences. Here’s a general guide for three popular options:
1. Using cs setup (recommended):
This is the official Scala installer powered by Coursier and works for Windows, macOS, and Linux.
- Download
- Follow on-screen instructions: Choose your Scala version, install directory, and optional configurations like IDE integration.
- Verify installation: Open a terminal and run
scala -version
. You should see the installed Scala version.
2. Manual installation:
- Download the Scala ZIP file
- Extract the ZIP file to your preferred directory (e.g., C:\Program Files\scala`).
- Set environment variables:
PATH
: Add thebin
directory of your Scala installation to your system path.JAVA_HOME
: Set this to the path of your Java JRE or JDK (required for Scala to run).
- Verify installation: Open a terminal and run
scala -version
.
3. Using an IDE:
Several IDEs like IntelliJ IDEA offer built-in Scala support. You can install Scala directly within the IDE:
- IntelliJ IDEA: Open “Preferences” > “Plugins” > Search for “Scala” > Install.
- Others: Check the specific installation instructions for your chosen IDE.
Additional configuration:
- SBT (Simple Build Tool): Recommended for building and managing Scala projects. Download from Scala official site and follow its installation guide.
- IDE configuration: If using an IDE, configure it to use your installed Scala version and SBT (if applicable).
Remember to choose the method that best suits your needs and operating system. Feel free to ask if you have any further questions or encounter any difficulties during the installation process!
Fundamental Tutorials of Scala: Getting started Step by Step
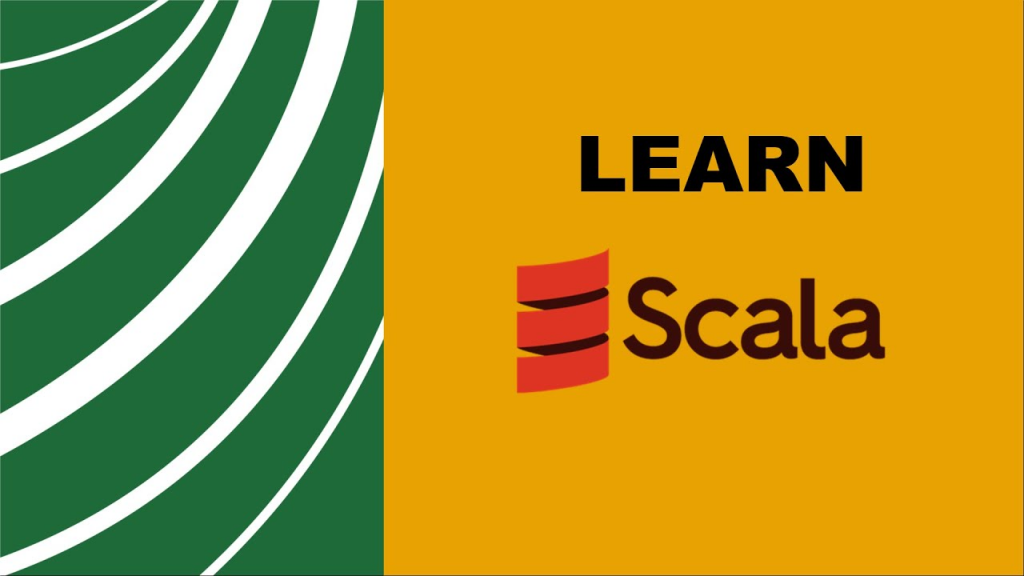
Let’s have a look at some step-by-step fundamental tutorials to get you started with Scala:
1. Setting Up:
- Install Scala: Choose your preferred method from the previous explanation (cs setup, manual, or IDE).
- Open a terminal or IDE: This is where you’ll write your Scala code.
2. Hello World!:
- Code:
Scala
println("Hello, World!")
- Explanation:
println
: Prints a message to the console."Hello, World!"
: The message to be printed.
- Run the code: Execute the code in your terminal or IDE. You should see “Hello, World!” printed.
3. Variables and Data Types:
- Code:
Scala
var name: String = "John" // Declare and assign a String variable
var age: Int = 30 // Declare and assign an Int variable
val greet = "Hello, " + name + "!" // Concatenate strings
println(greet) // Print the greeting with the name
- Explanation:
var
: Keyword for a variable that can be reassigned.val
: Keyword for a constant that cannot be changed.String
: Data type for text.Int
: Data type for whole numbers.+
: Operator for string concatenation.
4. Operators and Expressions:
- Code:
Scala
val sum = 10 + 5
val difference = 20 - 3
val product = 2 * 5
val quotient = 10 / 2
println(sum, difference, product, quotient)
- Explanation:
- Basic arithmetic operators:
+
,-
,*
,/
. println
can take multiple arguments to print on the same line.
- Basic arithmetic operators:
5. Conditional Statements:
- Code:
Scala
val age = 25
if (age >= 18) {
println("You are an adult!")
} else {
println("You are not an adult yet.")
}
- Explanation:
if
statement checks a condition and executes a block of code if true.else
block accomplishes if the condition is false.
6. Loops:
- Code:
Scala
for (i <- 1 to 5) {
println(i)
}
var i = 0
while (i < 5) {
println(i)
i += 1
}
- Explanation:
for
loop iterates a certain number of times.while
loop iterates as large as a condition is true.
7. Functions:
- Code:
Scala
def greet(name: String): String = {
"Hello, " + name + "!"
}
val message = greet("Scala")
println(message)
- Explanation:
def
keyword defines a function.greet
function takes a String parameter and returns a String greeting.message
stores the returned value from thegreet
function.
These are just a few basic tutorials to get you started with Scala. There are many more things you can learn, like object-oriented programming, functional programming concepts, collections, and libraries.
Remember, practice is key to learning Scala. Don’t hesitate to experiment and try out different things! Feel free to ask me any questions you encounter along the way.
- PPG Industries: Selection and Interview process, Questions/Answers - April 3, 2024
- Fiserv: Selection and Interview process, Questions/Answers - April 3, 2024
- Estee Lauder: Selection and Interview process, Questions/Answers - April 3, 2024