What is Spring?
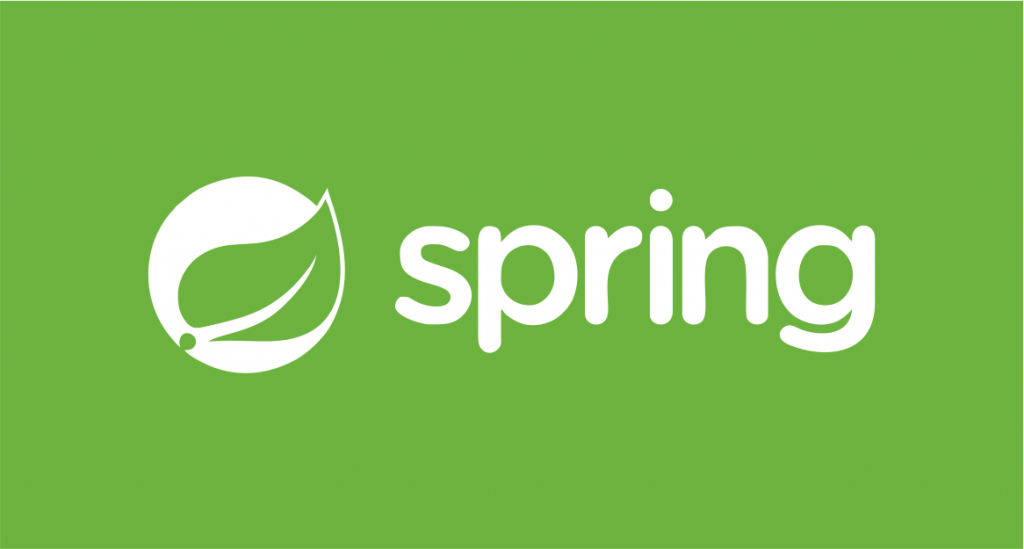
The Spring Framework is a comprehensive framework for Java development that provides infrastructure support, alleviates the complexities of Java development, and promotes good design practices. Developed by Pivotal Software, Spring is widely used for building enterprise-level applications in Java.
Key Features of Spring:
- Inversion of Control (IoC):
- Spring promotes the Inversion of Control principle, allowing the framework to manage and control the flow of the application. It achieves this through dependency injection, where objects receive their dependencies rather than creating them.
- Aspect-Oriented Programming (AOP):
- AOP in Spring allows developers to separate cross-cutting concerns, such as logging and security, from the main business logic. Aspects encapsulate these concerns, promoting modularization.
- Data Access:
- Spring provides a comprehensive abstraction layer for data access, supporting JDBC and various Object-Relational Mapping (ORM) frameworks like Hibernate. This simplifies database operations and promotes consistent data access.
- Transaction Management:
- Spring simplifies transaction management by supporting declarative transaction control using annotations or XML configurations. It integrates with Java Transaction API (JTA) and other transaction management strategies.
- Model-View-Controller (MVC):
- Spring MVC is a powerful web module for building web applications. It follows the Model-View-Controller architectural pattern and provides features for handling HTTP requests, managing session state, and rendering views.
- Security:
- Spring Security is a robust and customizable authentication and access control framework. It addresses common security concerns in web applications, including authentication, authorization, and protection against common vulnerabilities.
- Dependency Injection (DI):
- Spring’s DI feature facilitates loose coupling and promotes better testability and maintainability of code. Objects receive their dependencies through configuration rather than creating them directly.
- Bean Lifecycle Management:
- Spring manages the lifecycle of Java objects, known as beans. Developers can define initialization and destruction methods for beans, and the framework ensures proper execution.
- Internationalization (i18n) Support:
- Spring provides support for internationalization and localization through message bundles, making it easier to develop applications that support multiple languages.
- Testing:
- Spring supports unit testing and integration testing of applications. It provides tools and annotations for writing tests that can be easily executed in a Spring environment.
- Incorporation of Enterprise Design Patterns:
- Spring incorporates various enterprise design patterns, such as Singleton, Prototype, Factory, and Observer, making it easier to implement well-established architectural patterns.
- Simplified Java EE Development:
- Spring simplifies Java EE development by providing an alternative to many complex and verbose Java EE technologies, such as Enterprise JavaBeans (EJB).
What is top use cases of Spring?
Top Use Cases of Spring:
- Enterprise Application Development:
- Spring is widely used for building large-scale enterprise applications, providing a comprehensive set of tools and features for managing complexity.
- Web Application Development:
- Spring MVC is a popular choice for developing web applications, offering a flexible and modular approach to building web-based user interfaces.
- Microservices Architecture:
- Spring Boot, a part of the Spring ecosystem, is commonly used for developing microservices. It simplifies the setup and development of microservices-based applications.
- RESTful Web Services:
- Spring provides robust support for building RESTful web services. Spring MVC, along with Spring Boot, simplifies the development of RESTful APIs.
- Data Access:
- Spring’s data access features, including the use of JDBC and ORM frameworks like Hibernate, are extensively used for simplifying database operations in applications.
- Batch Processing:
- Spring Batch is a module for batch processing applications. It provides features for reading, processing, and writing large amounts of data, making it suitable for tasks like data migration and ETL (Extract, Transform, Load).
- Integration with Messaging Systems:
- Spring Integration facilitates the integration of enterprise systems by providing support for messaging patterns. It is commonly used for building message-driven applications.
- Authentication and Authorization:
- Spring Security is widely used for implementing authentication and authorization in web applications. It provides features for securing applications against common security threats.
- Caching:
- Spring’s caching support enables developers to easily integrate caching mechanisms into their applications, improving performance by reducing the need to repeatedly fetch data from the database.
- Asynchronous Programming:
- Spring supports asynchronous programming, allowing developers to build applications that can handle concurrent requests efficiently.
- Cloud-Native Development:
- Spring Cloud, a part of the Spring ecosystem, provides tools for building and deploying cloud-native applications. It facilitates the development of distributed and scalable systems.
- Testing and Test Automation:
- Spring’s features for testing, such as the use of annotations for dependency injection and the availability of the Spring TestContext Framework, make it easier to write and execute tests for Spring applications.
Spring’s flexibility, modular design, and extensive ecosystem make it a versatile choice for a wide range of Java development scenarios, from small projects to large-scale enterprise applications.
What are feature of Spring?
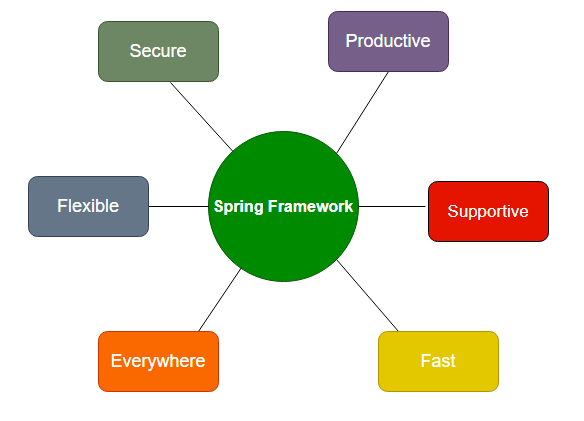
The Spring Framework is a comprehensive framework for Java development, providing a wide range of features that simplify application development and promote good design practices. Here are key features of the Spring Framework:
- Inversion of Control (IoC):
- Spring implements IoC, allowing the framework to manage object dependencies. This promotes loose coupling and makes applications more modular and easier to maintain.
- Dependency Injection (DI):
- DI is a core feature of Spring, enabling developers to inject dependencies into classes rather than having the classes create their dependencies. This enhances flexibility, testability, and maintainability.
- Aspect-Oriented Programming (AOP):
- AOP allows developers to separate cross-cutting concerns (such as logging and security) from the main business logic. Spring provides AOP support, enabling the modularization of concerns.
- Model-View-Controller (MVC):
- Spring MVC is a robust web module that follows the Model-View-Controller pattern. It provides features for handling HTTP requests, managing session state, and rendering views.
- Data Access:
- Spring provides a comprehensive abstraction layer for data access, supporting JDBC and ORM frameworks like Hibernate. This simplifies database operations and promotes consistent data access.
- Transaction Management:
- Spring clarifies transaction management, supporting both programmatic and declarative approaches. It integrates with various transaction management strategies, including Java Transaction API (JTA).
- Security:
- Spring Security is a powerful and customizable authentication and access control framework. It addresses common security concerns, including authentication, authorization, and protection against vulnerabilities.
- Spring Boot:
- Spring Boot simplifies the process of building production-ready applications by providing convention-over-configuration and a set of defaults. It includes an embedded web server and opinionated defaults for various configurations.
- Internationalization (i18n) Support:
- Spring supports internationalization and localization through message bundles, making it easier to develop applications that support multiple languages.
- Testing Support:
- Spring provides support for unit testing and integration testing. It includes features such as the Spring TestContext Framework, which simplifies the testing of Spring applications.
- Bean Lifecycle Management:
- Spring manages the lifecycle of Java objects, known as beans. Developers can define initialization and destruction methods for beans, and Spring ensures proper execution.
- Customization and Extension:
- Spring is highly customizable and extensible. Developers can extend the framework or customize its behavior by using various extension points and interfaces.
- Messaging Support:
- Spring provides support for messaging patterns, including point-to-point and publish-subscribe models. This is achieved through the integration of messaging technologies like Java Message Service (JMS).
- RESTful Web Services:
- Spring supports the development of RESTful web services through the Spring MVC framework. It simplifies the creation of REST APIs and the handling of HTTP requests.
- Scheduling and Asynchronous Processing:
- Spring includes features for scheduling tasks and asynchronous processing. This is useful for executing tasks at specified intervals or handling time-consuming operations in the background.
What is the workflow of Spring?
The workflow of building applications with the Spring Framework generally follows these steps:
- Project Setup:
- Set up a new Java project using a build tool such as Maven or Gradle. Include the necessary Spring dependencies in the project configuration.
- Define Beans:
- Define Java classes as Spring beans by annotating them with
@Component
,@Service
, or@Repository
. Beans represent the components of the application.
- Define Java classes as Spring beans by annotating them with
- Configure Dependencies:
- Use XML configuration, Java-based configuration, or annotation-based configuration to define and inject dependencies between beans. This is where the Inversion of Control (IoC) principle is applied.
- Enable Aspect-Oriented Programming (AOP):
- If needed, configure AOP aspects to address cross-cutting concerns like logging, security, or transactions.
- Implement Data Access:
- Use Spring’s data access features to interact with databases. Configure data sources, transaction management, and choose between JDBC or ORM frameworks like Hibernate.
- Implement Business Logic:
- Develop the core business logic of the application within the defined beans. Leverage dependency injection and other Spring features to enhance modularity.
- Create Spring MVC Controllers (Web Applications):
- For web applications, create Spring MVC controllers to handle HTTP requests. Map URLs to controller methods, and define views for rendering HTML or other responses.
- Configure Security (If Applicable):
- If the application requires security, configure Spring Security to handle authentication and authorization. Customize security settings based on application requirements.
- Enable Spring Boot (Optional):
- If using Spring Boot, leverage its features for convention-over-configuration. Create a
main
class annotated with@SpringBootApplication
to enable embedded web servers, auto-configuration, and defaults.
- If using Spring Boot, leverage its features for convention-over-configuration. Create a
- Internationalization and Localization:
- If the application needs to support multiple languages, use Spring’s internationalization (i18n) support to manage messages in different languages.
- Testing:
- Write unit tests and integration tests for the application using Spring’s testing features. Utilize the Spring TestContext Framework for effective testing.
- Run and Deploy:
- Run the Spring application either through the application server or using embedded servers provided by Spring Boot. Package the application for deployment.
- Monitoring and Debugging:
- Use Spring’s monitoring and debugging tools to identify issues, monitor performance, and ensure the proper functioning of the application.
- Asynchronous Processing (Optional):
- If the application requires asynchronous processing or scheduling, leverage Spring’s features for handling such tasks.
- Maintenance and Updates:
- Continuously maintain and update the application as needed. Take advantage of Spring’s extensibility and flexibility for adapting to changing requirements.
The Spring workflow emphasizes modularity, dependency injection, and the use of various Spring features to simplify the development process and enhance the quality of Java applications. The specific steps may vary based on the type of application being developed (e.g., web applications, microservices, batch processing).
How Spring Works & Architecture?
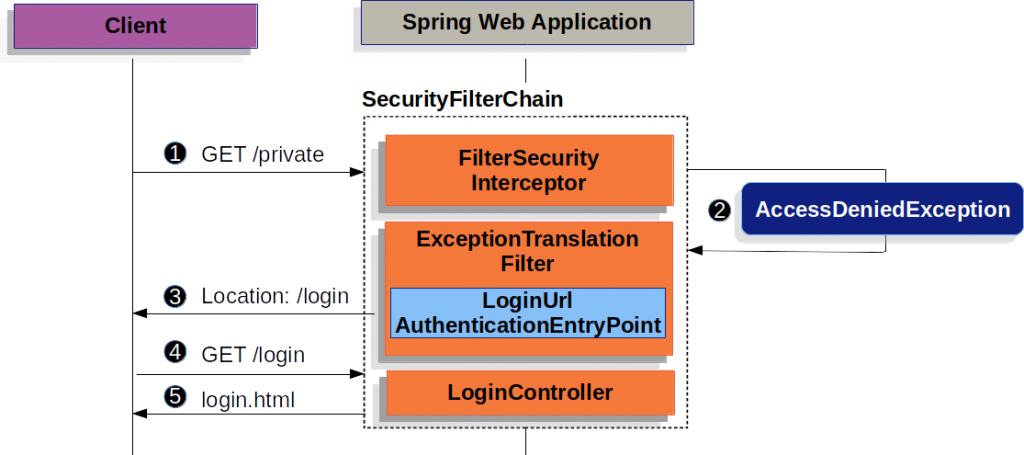
Spring is a popular open-source Java framework used for building enterprise-level applications. It provides a comprehensive set of features to simplify development and improve application reliability, scalability, and maintainability.
Following is a breakdown of how Spring works and its architecture:
1. Core Principles:
- Dependency Injection (DI): Spring manages and injects dependencies between objects, promoting loose coupling and modular design.
- Aspect-Oriented Programming (AOP): Allows for cross-cutting concerns like logging and security to be implemented independently and applied across the application.
- Lightweight and Non-Invasive: Spring integrates seamlessly with existing Java code without requiring major changes.
- Container and Modules: Spring provides a container for managing the application lifecycle and various modules for specific functionalities like web development, data access, and security.
2. Architecture:
- Core Container: Provides basic services like bean management, configuration, and event handling.
- Spring MVC: Framework for building web applications with MVC (Model-View-Controller) architecture.
- Spring Data: Simplifies data access and integration with various databases and repositories.
- Spring Security: Framework for authentication, authorization, and access control.
- Spring Cloud: Suite of tools for building and deploying cloud-native applications.
- Additional Modules: Various modules cater to specific needs like messaging, testing, and transaction management.
3. Components:
- Beans: Objects managed by Spring container with defined lifecycle and dependencies.
- Configurations: Specify configuration details like bean definitions, properties, and application context.
- Annotations: Provide metadata for Spring to interpret and configure beans and components.
- AOP Interceptors: Implement cross-cutting concerns and intercept method calls for specific functionalities.
4. Working Process:
- Spring container loads and parses configuration files.
- Beans are created and dependencies are injected based on configuration.
- Applications interact with beans and Spring modules to perform tasks.
- AOP interceptors apply cross-cutting concerns where applicable.
- Spring manages the lifecycle of beans and ensures proper resource cleanup.
5. Benefits:
- Reduced Complexity: Simplifies development by handling common tasks and boilerplate code.
- Increased Modularity: Promotes loose coupling and improves maintainability.
- Enhanced Scalability: Supports building applications that can scale efficiently.
- Improved Security: Provides robust security features for building secure applications.
- Rich Ecosystem: Vast community and numerous libraries and tools available.
Points to Remember:
- Spring offers a comprehensive set of features and modules to address various development needs.
- Understanding the core principles and architecture is crucial for leveraging Spring effectively.
- Utilize available resources and communities to learn and expand your Spring expertise.
By exploring the provided resources and practicing with Spring, you can unlock its powerful features and significantly enhance the development process and quality of your Java applications.
How to Install and Configure Spring?
Installing and Configuring Spring:
Spring offers various installation and configuration options depending on your needs and development environment. Here’s a breakdown of different approaches:
1. Setting Up Java Development Environment:
- Ensure you have a properly configured Java Development Kit (JDK) installed.
- Choose an Integrated Development Environment (IDE) like Eclipse or IntelliJ IDEA for developing your Spring applications.
2. Installing Spring Framework:
- Spring Boot: The recommended option for quickly getting started. Download the Spring Boot CLI (Command-Line Interface) or distribution based on your preferred operating system.
- Maven/Gradle: Use dependency management tools like Maven or Gradle to download and integrate Spring libraries into your project.
- Manual Download: Download individual Spring libraries and manually configure them in your project, although this approach is less convenient than using tools.
3. Project Configuration:
- Create a new project in your chosen IDE and configure it for Spring development.
- Define Spring configuration files like
application.properties
orapplication.yml
to specify beans, properties, and other settings. - You can leverage annotations or XML configurations to define bean definitions and configure components.
4. IDE Plugins:
- Install Spring-specific plugins for your chosen IDE to enhance development experience.
- These plugins offer features like code completion, Spring bean management tools, and Spring configuration validation.
5. Spring Tool Suite:
- Consider using Spring Tool Suite, a pre-configured Eclipse distribution specifically designed for Spring development.
- It includes various tools and plugins to streamline the Spring development process.
Points to Remember:
- Choose the installation approach that best suits your project requirements and development environment.
- Refer to the official Spring Boot documentation for detailed instructions and configuration options.
- Utilize IDE plugins and Spring Tool Suite for a more efficient development experience.
- Explore online resources and tutorials to learn more about specific aspects of Spring configuration.
By following these steps and utilizing the available resources, you can effectively install and configure Spring for your development needs and start building robust and scalable Java applications.
Fundamental Tutorials of Spring: Getting started Step by Step
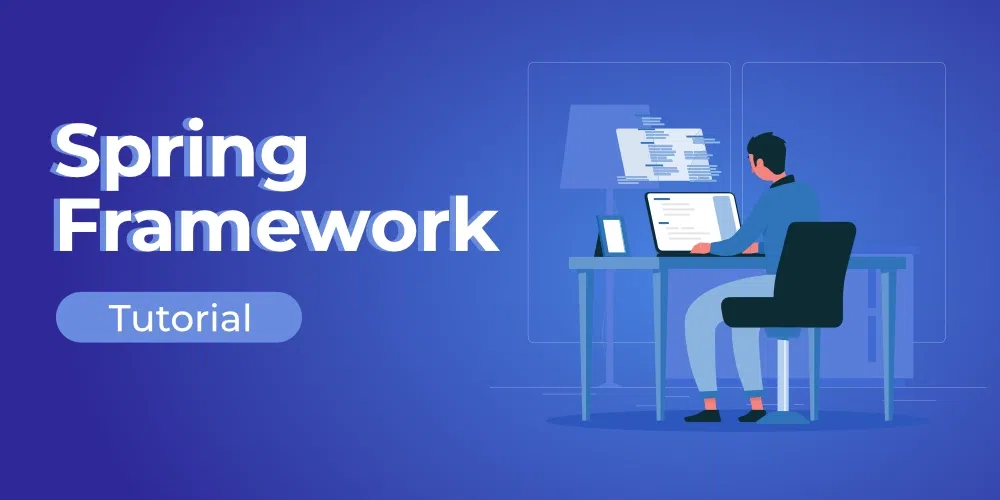
Following is a step-by-step guide for beginners to learn Spring Framework:
1. Setting Up the Environment:
- Install Java Development Kit (JDK): Ensure you have a compatible JDK installed. Java 11 or later is recommended.
- Choose an IDE: Popular options include IntelliJ IDEA, Eclipse, and Visual Studio Code. Install plugins like Spring Boot Tools for enhanced development experience.
- Download Spring Boot: Download the Spring Boot CLI or distribution based on your operating system.
2. Building Your First Spring Boot Application:
- Open your IDE and generate a new Spring Boot project.
- Choose a web application starter dependency for web development.
- Create a simple
@RestController
class with a basic@GetMapping("/")
method to return a message. - Run the application using the Spring Boot CLI or your IDE’s run configuration.
3. Exploring Spring Core Concepts:
- Dependency Injection (DI): Learn how Spring manages object dependencies and injects them into other objects. Explore
@Autowired
and constructor injection. - Beans and Configuration: Understand how Spring manages beans, objects managed by the Spring container. Explore configuration files like
application.properties
and annotations. - Aspect-Oriented Programming (AOP): Learn how AOP allows cross-cutting concerns like logging and security to be applied across the application.
4. Working with Data:
- Spring Data JPA: Learn how Spring Data JPA simplifies data access with JPA and repositories. Explore basic CRUD operations with entities.
- Database Connection: Configure your application to connect to a database like MySQL or PostgreSQL.
- Data Access Layer: Implement data access logic using repositories and Spring Data JPA methods.
5. Security and Authentication:
- Spring Security: Learn how Spring Security provides robust security features. Explore user authentication, authorization, and access control.
- Configure Login Page: Implement a basic login page for user authentication.
- Authorization Rules: Define authorization rules to control access to different resources based on user roles.
6. Building RESTful Web Services:
- Spring MVC: Learn how Spring MVC framework facilitates building RESTful web services.
- Mapping URL Endpoints: Map specific URL endpoints to handle client requests with different HTTP methods like GET, POST, PUT, and DELETE.
- Consuming APIs: Learn how to consume external APIs using Spring RestTemplate or WebClient.
7. Additional Spring Modules:
- Spring Cloud: Explore Spring Cloud tools for building cloud-native applications.
- Spring Batch: Learn about Spring Batch for processing large datasets and batch jobs.
- Spring Kafka: Explore Spring Kafka for building event-driven applications.
Points to Remember:
- Begin with the basics and gradually progress to more advanced topics.
- Practice building simple applications to solidify your understanding.
- Utilize online resources and tutorials for guidance and learning.
- Join online communities and forums to ask questions and learn from other developers.
By following these steps and actively engaging with the learning process, you can acquire the necessary skills and knowledge to effectively use Spring Framework and build robust and scalable Java applications.
- Mutual of Omaha: Selection and Interview process, Questions/Answers - April 15, 2024
- AES: Selection and Interview process, Questions/Answers - April 15, 2024
- Amphenol: Selection and Interview process, Questions/Answers - April 15, 2024