What is Spring Boot ?
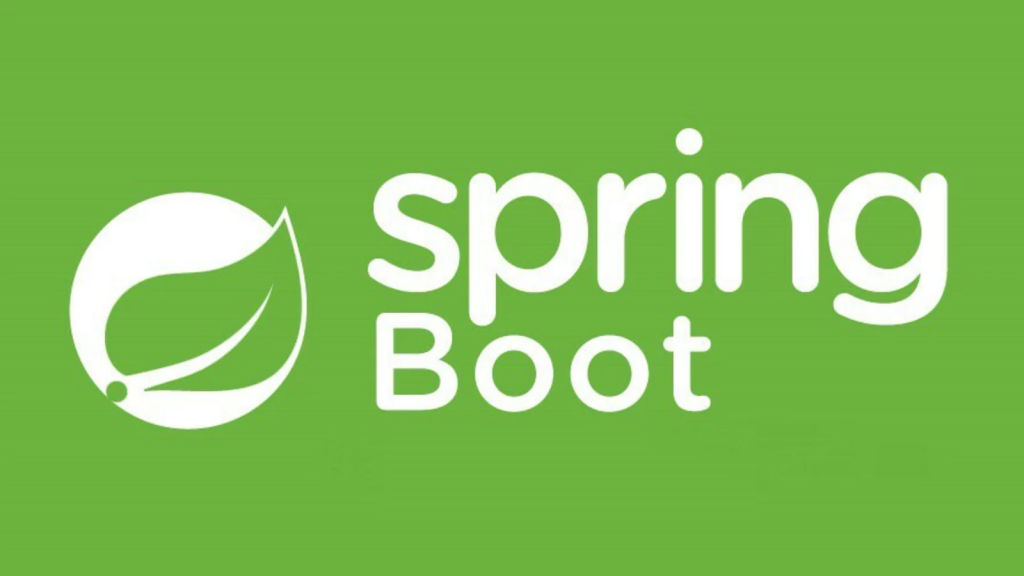
Spring Boot is an open-source Java-based framework developed by the Pivotal Team, part of the larger Spring Framework. It is designed to simplify the development of production-ready applications with minimal effort. Spring Boot provides a convention-over-configuration approach, which means it comes with sensible defaults, reducing the need for extensive configuration.
What is top use cases of Spring Boot ?
Top Use Cases of Spring Boot:
- Microservices Architecture:
- Description: Spring Boot is widely used for building microservices-based applications. Its ease of development, embedded container support, and built-in features for microservices make it a preferred choice for developers building distributed systems.
- Web Application Development:
- Description: Spring Boot simplifies web application development by providing a set of defaults and conventions. It is commonly used for building RESTful APIs, web services, and traditional web applications.
- Enterprise-level Applications:
- Description: Spring Boot is suitable for developing large-scale enterprise applications, offering features like dependency injection, aspect-oriented programming, and transaction management.
- Spring Cloud Integration:
- Description: Spring Boot integrates seamlessly with the Spring Cloud ecosystem, making it a go-to choice for building cloud-native applications. Developers can leverage Spring Cloud for services like service discovery, configuration management, and load balancing.
- Data Processing Applications:
- Description: Spring Boot is used for developing data processing applications, such as ETL (Extract, Transform, Load) processes, batch processing, and data analytics applications.
- RESTful Web Services:
- Description: Spring Boot simplifies the development of RESTful web services. It provides annotations for quickly building and configuring endpoints, handling HTTP methods, and managing request and response formats.
- Real-time Applications:
- Description: For real-time applications like chat applications, Spring Boot, when combined with technologies like WebSocket, provides a solid foundation for building scalable and responsive real-time features.
- Integration with Frontend Frameworks:
- Description: Spring Boot is often used in conjunction with frontend frameworks like Angular, React, or Vue.js to build full-stack applications. It facilitates the development of the backend while providing RESTful APIs for frontend interactions.
- Containerized Applications:
- Description: Spring Boot applications can be easily containerized using technologies like Docker. This makes it convenient for deploying applications in container orchestration platforms like Kubernetes.
- IoT (Internet of Things) Applications:
- Description: Spring Boot’s lightweight nature and modular design make it suitable for developing applications in the IoT space. It can be used to create backend services for managing IoT devices and processing data from sensors.
- Authentication and Authorization Services:
- Description: Spring Boot provides robust support for implementing authentication and authorization mechanisms. It is commonly used for building secure applications with features like OAuth2, JWT (JSON Web Tokens), and Spring Security.
- Event-Driven Applications:
- Description: Spring Boot is suitable for developing event-driven applications where components communicate through events. It can be used with message brokers like Apache Kafka or RabbitMQ for building scalable and responsive event-driven systems.
- Caching Services:
- Description: Spring Boot simplifies the integration of caching mechanisms, such as Ehcache or Redis, to improve the performance of applications by storing frequently accessed data in memory.
- Job Scheduling:
- Description: Spring Boot includes features for job scheduling and batch processing. It is commonly used for running scheduled tasks, data processing jobs, and batch operations.
- DevOps Automation:
- Description: Spring Boot, when combined with tools like Spring Cloud Config, can be part of a DevOps pipeline for automating application configuration management and deployment.
Spring Boot’s versatility and developer-friendly features make it a popular choice for a wide range of use cases, from small projects to large-scale enterprise applications and microservices architectures. Its convention-over-configuration approach and seamless integration with the Spring ecosystem contribute to its widespread adoption in the Java development community.
What are feature of Spring Boot?
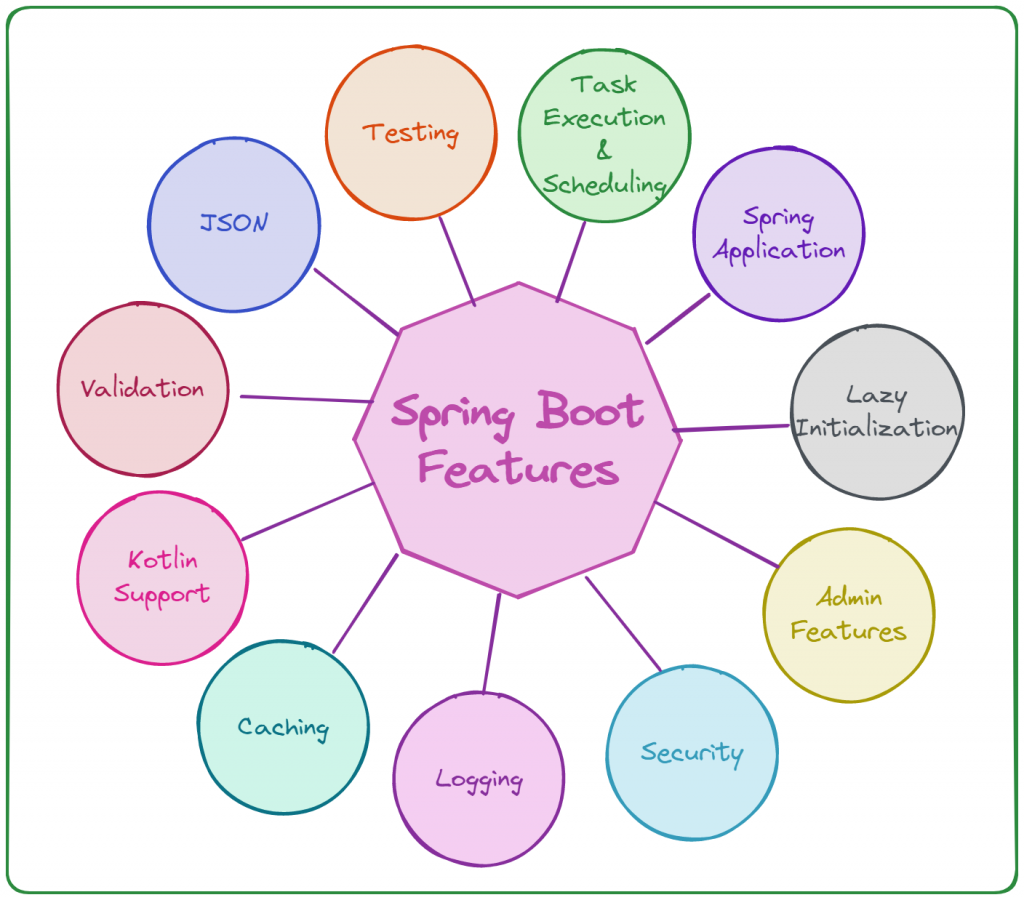
Features of Spring Boot:
- Opinionated Defaults:
- Description: Spring Boot provides sensible defaults and opinionated configurations, reducing the need for developers to make extensive decisions about configurations. This helps in quick setup and development.
- Embedded Containers:
- Description: Spring Boot comes with embedded containers like Tomcat, Jetty, and Undertow. This allows developers to package the application with its runtime, making it easy to deploy and run without external container dependencies.
- Auto-Configuration:
- Description: Spring Boot’s auto-configuration feature automatically configures the application based on its dependencies. It simplifies the configuration process by eliminating the need for manual setup in many cases.
- Standalone:
- Description: Spring Boot applications can be developed as standalone applications with minimal external dependencies. This makes it easy to create self-contained, executable JARs.
- Spring Boot Starters:
- Description: Starters are pre-configured templates that help in simplifying the dependency management. Spring Boot provides a variety of starters for common use cases like web applications, data access, messaging, and more.
- Spring Boot Actuator:
- Description: Actuator provides production-ready features to help monitor and manage applications. It includes endpoints for health checks, metrics, environment information, and more.
- Spring Boot DevTools:
- Description: DevTools offer features like automatic application restarts, which accelerate the development process by reducing the need for manual restarts after code changes.
- Spring Boot CLI (Command Line Interface):
- Description: The CLI allows developers to run Groovy scripts and create Spring Boot applications from the command line. It provides a quick and convenient way to prototype and develop applications.
- Spring Boot Annotations:
- Description: Spring Boot uses annotations to simplify the configuration process. Annotations like
@SpringBootApplication
and@RestController
help in creating Spring-powered applications with minimal boilerplate code.
- Description: Spring Boot uses annotations to simplify the configuration process. Annotations like
- Microservices Support:
- Description: Spring Boot is well-suited for building microservices-based architectures. It integrates seamlessly with Spring Cloud for implementing patterns such as service discovery, distributed configuration, and circuit breakers.
- Spring Boot Data:
- Description: Spring Boot provides simplified data access through Spring Data, making it easy to interact with databases using JPA, JDBC, or other data technologies.
- Testing Support:
- Description: Spring Boot simplifies testing by providing annotations and utilities for testing various components of the application. It favors unit testing, integration testing, and end-to-end testing.
- Externalized Configuration:
- Description: Configuration properties in Spring Boot can be externalized, allowing developers to configure the application without modifying the code. This is particularly useful for configuration in different environments.
- Spring Boot Initializr:
- Description: The Initializr is a web-based tool that simplifies the process of creating a new Spring Boot project. Developers can select dependencies, configure project settings, and download a pre-configured project structure.
- Thymeleaf and Spring MVC:
- Description: Spring Boot integrates seamlessly with Thymeleaf, a modern server-side Java template engine, and Spring MVC for building dynamic web applications.
What is the workflow of Spring Boot?
Workflow of Spring Boot:
- Initialize Project:
- Action: Use the Spring Initializr or a build tool (e.g., Maven, Gradle) to initialize a new Spring Boot project.
- Activity: Define project metadata, select dependencies, and generate the project structure.
- Develop Application:
- Action: Write application code using Spring Boot annotations and features.
- Activity: Create controllers, services, repositories, and other components. Leverage Spring Boot’s auto-configuration and conventions.
- Configure Application:
- Action: Customize application configuration if needed.
- Activity: Modify application.properties or application.yml files, set environment-specific properties, or use externalized configuration.
- Run and Test Locally:
- Action: Run the application locally for development and testing.
- Activity: Use the development tools provided by Spring Boot for features like automatic restarts during development.
- Package Application:
- Action: Package the application for deployment.
- Activity: Use build tools (e.g., Maven or Gradle) to create an executable JAR or WAR file.
- Deploy Application:
- Action: Deploy the packaged application to a container or cloud platform.
- Activity: Deploy the JAR or WAR file to a server, container, or cloud environment.
- Monitor and Manage:
- Action: Monitor and manage the application in production.
- Activity: Utilize Spring Boot Actuator endpoints for health checks, metrics, and other production-related features.
- Update and Maintain:
- Action: Update the application and maintain it over time.
- Activity: Manage dependencies, apply updates, and continuously improve the application based on requirements.
The workflow is iterative, allowing developers to quickly make changes, test them, and deploy the application with minimal friction. Spring Boot’s conventions and defaults streamline the development process, making it efficient and developer-friendly.
How Spring Boot Works & Architecture?
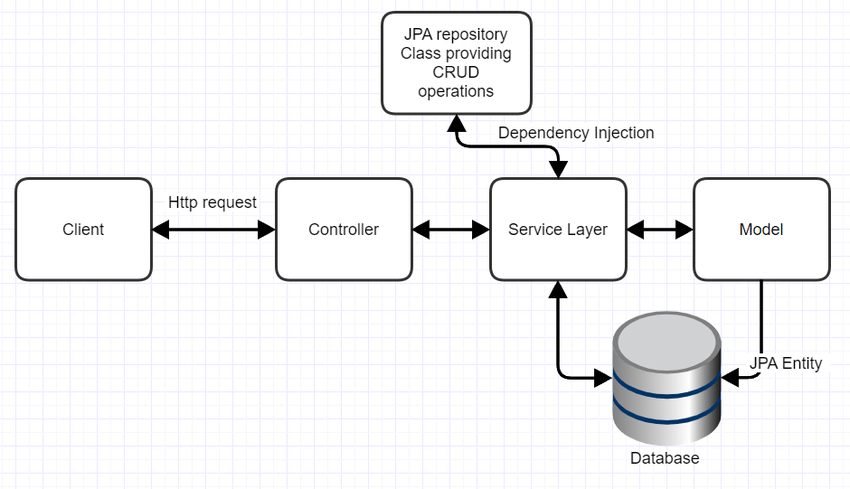
Spring Boot is an open-source framework for building modern Java applications. It simplifies the development process by providing:
- Autoconfiguration: Automatically configures beans and components based on available dependencies and annotations.
- Embedded Servers: Integrates embedded servers like Tomcat and Jetty, eliminating the need for external configuration.
- Starter POMs: Provides pre-configured dependencies for various functionalities like database access, web development, etc.
- Opinionated Defaults: Encourages best practices and conventions for building reliable and maintainable applications.
Architecture:
Spring Boot follows a layered architecture with various components working together:
1. Core: Provides the foundation for the framework, including: * BeanFactory: Creates and manages beans. * ApplicationContext: Holds configuration information and provides access to beans. * Environment: Stores configuration properties and environment variables.
2. Web: Enables building web applications with features like: * MVC (Model-View-Controller) architecture. * Embedded web servers. * Security and session management.
3. Data: Facilitates data access and interaction with databases: * JDBC and JPA integration. * Object-relational mapping (ORM) tools. * NoSQL database support.
4. Starter POMs: Pre-configured dependencies for specific functionalities, including: * Spring Security * Spring Data (JDBC, JPA, MongoDB, etc.) * Spring Web * Spring Cloud
5. Application: The topmost layer, where your application logic resides: * Controllers handle user requests. * Services implement business logic. * Models represent data objects.
Benefits:
- Increased Development Speed: Autoconfiguration and starter POMs reduce configuration time.
- Simplified Application Setup: Embedded servers eliminate the need for external configuration.
- Consistent Application Structure: Encourages best practices and makes applications easier to maintain.
- Wide Range of Functionality: Supports various functionalities through starter POMs and integrations.
By understanding how Spring Boot works and its architecture, developers can leverage its features to build robust, scalable, and maintainable Java applications.
How to Install and Configure Spring Boot?
Installing and Configuring Spring Boot
Following is a step-by-step guide on installing and configuring Spring Boot:
1. Prerequisites:
- JDK 17 or later: You need a compatible Java Development Kit (JDK) to run Spring Boot applications.
- Maven or Gradle: These build tools are used to manage dependencies and build your project.
2. Installation:
Option 1: Using Spring Initializr:
- Visit Spring Initializr official website.
- Select your desired project dependencies.
- Click “Generate” to download a pre-configured project with the chosen dependencies.
- Unzip the downloaded file and import it into your IDE (e.g., IntelliJ IDEA, Eclipse).
Option 2: Installing Spring Boot CLI:
- Download the Spring Boot CLI distribution from their official website.
- Unzip the downloaded file and add the
bin
directory to your system path. - Open a terminal and run
spring --version
to verify the installation.
3. Configuration:
- application.properties: This file stores your application’s configuration details like server port, database connection information, etc.
- pom.xml (Maven) or build.gradle (Gradle): These files manage project dependencies and build configurations.
- Java classes: These contain your application logic, including controllers, services, and models.
4. Running your application:
- Spring Boot CLI:
- Open a terminal and navigate to your project directory.
- Run
spring boot run
to start your application.
- IDE:
- Right-click on your main class (e.g.,
Application.java
). - Select “Run As” -> “Spring Boot App”.
- Right-click on your main class (e.g.,
5. Additional Configurations:
- Spring Boot Starter POMs: You can add pre-configured dependencies for specific functionalities like database access, web development, etc.
- Logging configuration: Configure logging using libraries like Logback or SLF4J.
- Security configuration: Implement security measures like user authentication and authorization.
Important Tips:
- Start with a basic project and gradually add features as needed.
- Utilize online resources and tutorials for detailed guidance.
- Practice regularly to gain experience with Spring Boot development.
- Refer to the official documentation for specific configuration choices and troubleshooting.
By following these steps and exploring the available resources, you can learn to install, configure, and build Spring Boot applications effectively. Remember, practice and continuous learning are key to mastering Spring Boot development.
Fundamental Tutorials of Spring Boot: Getting started Step by Step
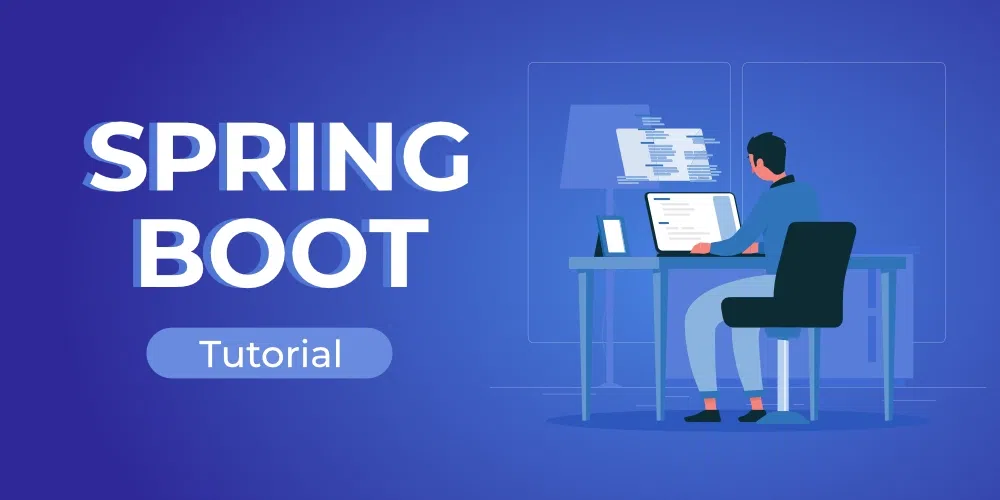
Following is a breakdown of basic tutorials to get you started with Spring Boot:
1. Setting Up:
- Install Prerequisites: Ensure you have Java Development Kit (JDK) 17 or later and a build tool like Maven or Gradle.
- Choose an option:
- Spring Initializr: Generate a pre-configured project with desired dependencies from their spring Initializr official site.
- Spring Boot CLI: Download and install the CLI from their offficial website for command-line application management.
2. Create a Basic Project:
- With Spring Initializr, download and import the project into your IDE.
- With Spring Boot CLI, create a new directory and run
spring init
to create a basic project structure.
3. Explore Project Structure:
- Familiarize yourself with key files like
pom.xml
(Maven) orbuild.gradle
(Gradle),application.properties
, and Java source files.
4. Build and Run the Application:
- Open a terminal and navigate to your project directory.
- For Spring Boot CLI, run
spring boot run
to start your application. - For IDEs, right-click on the main class (e.g.,
Application.java
) and select “Run As” -> “Spring Boot App”.
5. Explore Basic Features:
- Understand autoconfiguration: Spring Boot automatically configures beans based on available dependencies.
- Write simple controllers: Create controllers to handle user requests and serve responses.
- Use Spring MVC: Implement the Model-View-Controller pattern for building web applications.
6. Connect to Databases:
- Add database dependencies like Spring Data JPA and configure connection information in
application.properties
. - Create entities to represent database tables and use repositories for data access.
7. Implement Security:
- Add Spring Security dependencies and configure user authentication and authorization.
- Utilize libraries like Spring Security Web for secure web applications.
8. Explore Spring Boot Starter POMs:
- Leverage pre-configured dependencies for various functionalities like database access, web development, etc.
- Add desired starter POMs to your project’s
pom.xml
orbuild.gradle
file.
9. Practice and Experiment:
- Build small example applications to solidify your understanding.
- Explore online resources and tutorials for further learning.
- Utilize the Spring Boot community for support and guidance.
Points to Remember:
- Start simple and gradually progress to more complex features.
- Practice regularly and experiment with different functionalities.
- Utilize available resources and communities for learning and support.
- Refer to the official documentation for detailed guidance and troubleshooting.
By following these steps and exploring the resources, you can build a solid foundation in Spring Boot. Consistent practice and learning will help you master this powerful framework for Java application development.
- Mutual of Omaha: Selection and Interview process, Questions/Answers - April 15, 2024
- AES: Selection and Interview process, Questions/Answers - April 15, 2024
- Amphenol: Selection and Interview process, Questions/Answers - April 15, 2024