What is Spring MVC?
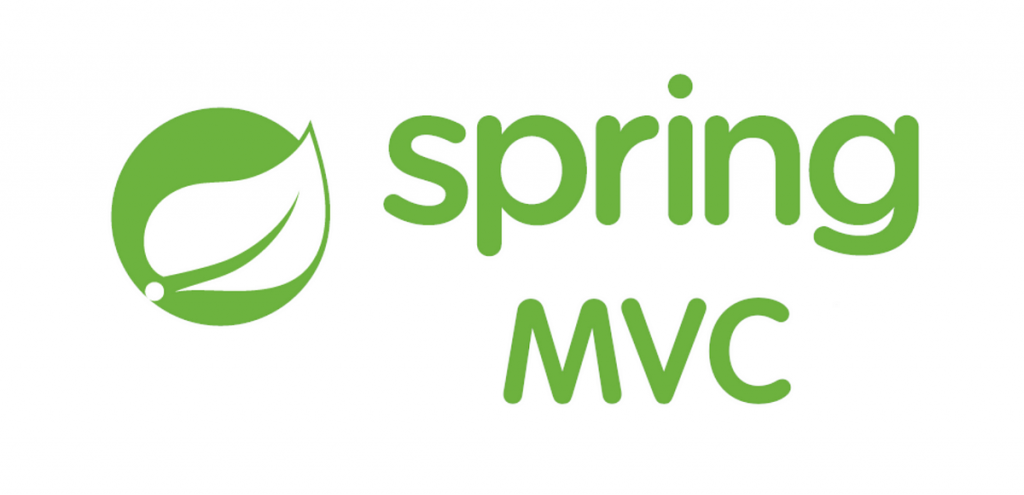
Spring MVC (Model-View-Controller) is a web framework within the broader Spring Framework for building Java-based enterprise web applications. It follows the MVC design pattern, where the application is divided into three main components: Model (data and business logic), View (user interface), and Controller (handles user input and manages the flow of data between Model and View). Spring MVC is widely used for developing robust and scalable web applications.
What is top use cases of Spring MVC?
Top Use Cases of Spring MVC:
- Web Application Development:
- Spring MVC is primarily designed for building web applications. It provides a structured and modular approach to develop scalable and maintainable web-based systems.
- Enterprise-Level Applications:
- Spring MVC is well-suited for developing enterprise-level applications where the separation of concerns and scalability are crucial. It integrates seamlessly with other components of the Spring Framework, such as Spring Security and Spring Data.
- RESTful Web Services:
- Spring MVC is used to build RESTful web services by leveraging the features of the framework, including the ability to produce and consume JSON or XML payloads. It is commonly chosen for implementing the backend of RESTful APIs.
- Interactive Websites:
- Spring MVC is employed for creating interactive and dynamic websites that require server-side processing and interaction. It is often used in conjunction with frontend frameworks like Angular, React, or Vue.js to build modern, single-page applications.
- Content Management Systems (CMS):
- Spring MVC is suitable for building content management systems where content creation, modification, and presentation need to be managed through a web interface. The modular structure of Spring MVC facilitates the development of extensible CMS applications.
- E-commerce Platforms:
- E-commerce platforms often use Spring MVC for developing the backend of online shopping websites. It supports features such as user authentication, product catalog management, and order processing.
- Financial Applications:
- Spring MVC is chosen for developing financial applications that require a high level of security, transaction management, and data integrity. It is well-suited for applications dealing with financial transactions and reporting.
- Customer Relationship Management (CRM) Systems:
- CRM systems that manage customer interactions, sales, and marketing activities often leverage Spring MVC for developing the web-based interface. It provides a scalable architecture for handling large amounts of customer data.
- Healthcare Information Systems:
- Spring MVC is used in the healthcare sector to build information systems for managing patient records, scheduling appointments, and facilitating communication among healthcare providers. Its modular architecture supports the development of complex healthcare applications.
- Educational Portals:
- Educational institutions use Spring MVC for developing web portals that manage student information, course registration, grading, and communication between students and faculty.
- Government Applications:
- Government agencies and organizations use Spring MVC to build web applications for citizen services, document management, and administrative processes. It provides a reliable and secure framework for government systems.
- Travel and Booking Systems:
- Spring MVC is employed in travel and booking systems for managing reservations, ticketing, and other transactions related to travel. It supports the development of scalable and transactional applications in the travel industry.
- Human Resources Management Systems (HRMS):
- HRMS applications, which handle employee information, payroll, and HR processes, often utilize Spring MVC for the development of user-friendly web interfaces.
- Social Networking Platforms:
- Spring MVC can be used to build the backend of social networking platforms, supporting features like user profiles, friend requests, and activity feeds. It integrates well with frontend technologies for creating modern social networking applications.
- Real-time Applications:
- While Spring MVC is traditionally request-driven, it can be combined with technologies like WebSocket to develop real-time web applications, providing features such as live updates and messaging.
Spring MVC’s versatility, modular architecture, and integration capabilities make it a popular choice for a wide range of web application development scenarios, especially in enterprise-level projects where maintainability and scalability are critical.
What are feature of Spring MVC?
Features of Spring MVC:
- MVC Architecture:
- Spring MVC follows the Model-View-Controller (MVC) architectural pattern, promoting a clear separation of concerns. This modular structure enhances maintainability and facilitates unit testing.
- Flexibility and Customization:
- Spring MVC provides a high degree of flexibility and customization. Developers can choose various components such as view resolvers, handler mappings, and interceptors, tailoring the framework to specific application needs.
- Integration with Other Spring Modules:
- Spring MVC seamlessly integrates with other modules of the Spring Framework, such as Spring Core, Spring Security, and Spring Data. This integration allows developers to leverage the full capabilities of the Spring ecosystem.
- Annotation-Based Configuration:
- Spring MVC supports annotation-based configuration, reducing the need for XML configuration files. Annotations such as
@Controller
,@RequestMapping
, and others simplify the configuration of controllers and request mappings.
- Spring MVC supports annotation-based configuration, reducing the need for XML configuration files. Annotations such as
- ModelAndView for Data and View:
- The use of
ModelAndView
allows controllers to pass data to views and specify the view to render. This separation enables controllers to focus on handling requests, while views are responsible for rendering the response.
- The use of
- View Resolvers:
- Spring MVC supports view resolvers, allowing developers to define logical view names that are resolved to actual view implementations. This abstraction makes it easy to switch between different view technologies (JSP, Thymeleaf, FreeMarker, etc.).
- Handler Mapping:
- Handler mappings in Spring MVC determine which controller should handle a particular request. Various handler mapping strategies are available, including annotations, URLs, and more, providing flexibility in mapping requests to controllers.
- Interceptor Support:
- Interceptors allow developers to pre-process requests and post-process responses globally across controllers. Interceptors can be used for tasks such as authentication, logging, or modifying the model before rendering the view.
- Data Binding and Validation:
- Spring MVC provides robust data binding and validation mechanisms. It can automatically bind form data to Java objects and perform validation based on annotations or custom validators.
- Internationalization and Localization:
- Spring MVC supports internationalization (i18n) and localization (l10n) through message bundles. This allows developers to create applications that can be easily adapted to different languages and regions.
- RESTful Capabilities:
- While originally designed for traditional web applications, Spring MVC has evolved to support RESTful web services. It includes features such as content negotiation, HTTP method handling, and support for producing and consuming JSON or XML payloads.
- Exception Handling:
- Spring MVC provides a comprehensive mechanism for handling exceptions in a consistent manner. Developers can define global exception handlers and customize error views to present meaningful error messages to users.
- File Upload and Download:
- Spring MVC supports file upload and download capabilities, making it straightforward to handle multipart requests for file uploads and serve files for download.
- Testability:
- Spring MVC applications are highly testable. The framework provides a suite of testing tools for unit testing controllers, integration testing, and performing end-to-end testing of web applications.
What is the workflow of Spring MVC?
Workflow of Spring MVC:
- Request Processing Lifecycle:
- When a client sends a request, it is first intercepted by the
DispatcherServlet
. TheDispatcherServlet
is configured in theweb.xml
file and acts as the entry point for all requests.
- When a client sends a request, it is first intercepted by the
- Handler Mapping:
- The
DispatcherServlet
consults the configured handler mappings to determine which controller should handle the incoming request. Handler mappings can use various strategies, such as URL patterns or annotations.
- The
- Controller Handling:
- The selected controller processes the request and returns a
ModelAndView
object. The controller is responsible for handling the business logic, accessing services, and preparing data to be displayed in the view.
- The selected controller processes the request and returns a
- Data Binding and Validation:
- If the request involves form submission, Spring MVC performs data binding, automatically populating Java objects with form data. Validation can be applied using annotations or custom validators.
- ModelAndView and View Resolvers:
- The controller returns a
ModelAndView
object containing the data and the logical view name. View resolvers are then used to translate the logical view name into an actual view implementation (JSP, Thymeleaf, etc.).
- The controller returns a
- View Rendering:
- The selected view renders the response, incorporating the data provided by the
ModelAndView
. The rendered output is sent back to the client as the response.
- The selected view renders the response, incorporating the data provided by the
- Interceptors:
- Interceptors, if configured, can intercept the request before it reaches the controller or after the controller has processed the request. Interceptors are commonly used for tasks such as authentication, logging, or modifying the model.
- Exception Handling:
- If an exception occurs during request processing, Spring MVC invokes the configured exception handlers to handle and respond to the exception appropriately. This may involve displaying a custom error page or redirecting to a specific error view.
- Response Rendering:
- The final rendered response is sent back to the client, completing the request processing lifecycle. The client receives the HTML, JSON, or other content generated by the view.
- Internationalization and Localization:
- If internationalization and localization are enabled, Spring MVC uses message bundles to translate and present content in the desired language or locale.
- File Upload and Download:
- Spring MVC handles file uploads and downloads seamlessly. Controllers can process multipart requests for file uploads, and the framework facilitates serving files for download.
- Testing:
- Spring MVC applications are designed to be highly testable. Developers can write unit tests for controllers, integration tests for the entire application, and end-to-end tests to ensure that the web application functions correctly.
Understanding the workflow of Spring MVC helps developers build scalable and maintainable web applications by leveraging the features and abstractions provided by the framework.
How Spring MVC Works & Architecture?
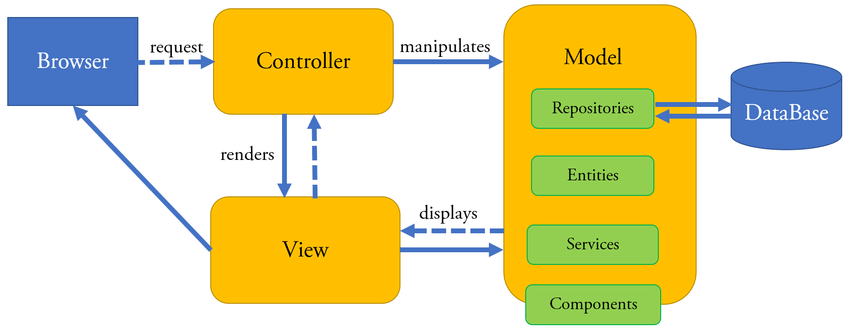
Spring MVC is a Java framework built on the Model-View-Controller (MVC) design pattern for developing web applications. It streamlines the development process by separating business logic, presentation logic, and data into distinct layers. Here’s a breakdown of its working and architecture:
Components:
- DispatcherServlet: Acts as the central controller, receiving all incoming HTTP requests. It identifies the appropriate handler (usually a controller) based on the request URL and parameters.
- Controllers: Process user requests and map them to business logic. They interact with various Spring beans including services, repositories, and models.
- Models: Represent the data of the application, holding information and business logic related to a specific concept.
- Views: Responsible for presenting the processed data to the user. They can be JSP files, Thymeleaf templates, or any other templating technology.
- Beans: Reusable components managed by Spring. They include controllers, services, repositories, and other application building blocks.
Workflow:
- Client sends an HTTP request.
- DispatcherServlet receives the request and analyzes it.
- Based on the request, it finds the appropriate controller using HandlerMapping.
- The controller processes the request, interacting with models and services as needed.
- The model prepares the data for presentation.
- The controller chooses the appropriate view to render the data.
- The view generates the final response to be sent back to the client.
Architecture:
Spring MVC has a layered architecture consisting of four main layers:
- Presentation Layer: This layer deals with the user interface, primarily composed of views and controllers.
- Business Logic Layer: This layer encapsulates the application’s core logic, consisting of services and models.
- DataAccess Layer: This layer interacts with the data source, using repositories and data access objects.
- Spring Framework Layer: This underlying layer provides essential services like dependency injection, transaction management, and security.
Benefits:
- Separation of concerns: MVC architecture promotes cleaner code and easier maintenance.
- Loose coupling: Components are loosely coupled, making them more reusable and testable.
- Flexibility: Developers can choose different technologies for each layer.
- Scalability: The framework can be easily scaled to handle large applications.
How to Install and Configure Spring MVC?
Following is a detailed guide on how to install and configure Spring MVC:
Prerequisites:
- Java Development Kit (JDK): Download and install the latest JDK version from Oracle’s website.
- Integrated Development Environment (IDE): Choose a suitable IDE like Eclipse, Spring Tool Suite (STS), or IntelliJ IDEA.
- Build Tool (optional): Maven or Gradle can be used for managing dependencies and building the project.
Installation Methods:
- Using Spring Initializr:
- Access the Spring Initializr website.
- Choose “Maven Project” or “Gradle Project” as desired.
- Select “Spring Web” dependency.
- Create the project and download it as a ZIP file.
- Import the project into your IDE.
- Manual Setup:
- Create a new Maven or Gradle project in your IDE.
- Add Spring MVC dependencies to your project’s pom.xml (Maven) or build.gradle (Gradle) file.
Maven Dependency:
XML
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>5.3.25</version> </dependency>
Gradle Dependency:
Gradle
implementation 'org.springframework:spring-webmvc:5.3.25' ```
**Configuration:**
1. **Create `DispatcherServlet` in `web.xml`:**
```xml
<servlet>
<servlet-name>dispatcher</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>dispatcher</servlet-name>
<url-pattern>/</url-pattern>
</servlet-mapping>
- Create Spring MVC configuration file (
dispatcher-servlet.xml
by default):
XML
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context.xsd">
<context:component-scan base-package="com.example.myapp" />
<bean class="org.springframework.web.servlet.view.InternalResourceViewResolver">
<property name="prefix" value="/WEB-INF/views/" />
<property name="suffix" value=".jsp" />
</bean>
</beans>
Important Point (if not using Spring Boot):
- Add Java Servlet API dependency to your project.
- Configure a web application server (Tomcat, Jetty, etc.) in your IDE.
Building and Running:
- Build the project using your build tool or IDE’s build functionality.
- Deploy the generated WAR file to your web server.
- Access the application using the configured URL (e.g., http://localhost:8080/)
Troubleshooting:
- Ensure correct dependency versions and configuration.
- Check for typos and configuration errors.
- Refer to Spring MVC documentation and community forums for assistance.
Fundamental Tutorials of Spring MVC: Getting started Step by Step
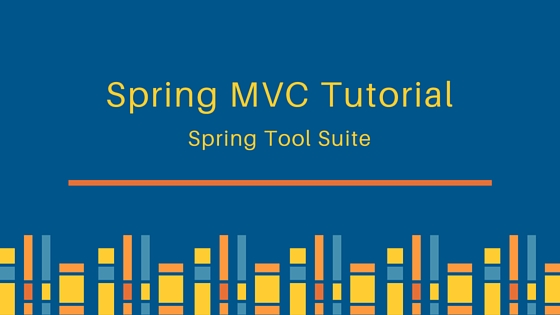
Building a simple “Hello World” application with Spring MVC is a great way to get acquainted with the framework’s core concepts. Let’s walk through the steps together:
1. Prerequisites:
- Java Development Kit (JDK): Download and install a suitable JDK version (e.g., JDK 17).
- Integrated Development Environment (IDE): Choose an IDE with Spring support, like Eclipse, Spring Tool Suite (STS), or IntelliJ IDEA.
2. Project Setup:
- Generate a new Maven project in your IDE.
- Add the Spring WebMVC dependency:
- Maven: In
pom.xml
file, add this dependency inside<dependencies>
tag:
<dependency> <groupId>org.springframework</groupId> <artifactId>spring-webmvc</artifactId> <version>5.3.25</version> </dependency>
- Gradle: In
build.gradle
file, add this line insidedependencies
block:
implementation 'org.springframework:spring-webmvc:5.3.25'
- Maven: In
3. Configure DispatcherServlet:
- Create a file named
web.xml
in the/src/main/webapp/WEB-INF
directory. - Add the following configuration inside the
<web-app>
element:
XML
<servlet>
<servlet-name>dispatcher</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>dispatcher</servlet-name>
<url-pattern>/</url-pattern>
</servlet-mapping>
4. Create a Controller:
- Create a Java class named
HelloWorldController
in the package of your choice (e.g.,com.example.myapp
). - Add the
@Controller
annotation to the class and define a method annotated with@RequestMapping("/")
:
Java
@Controller
public class HelloWorldController {
@RequestMapping("/")
public String helloWorld() {
return "hello"; // This returns the view name
}
}
5. Create a View:
- Create a JSP file named
hello.jsp
in the/src/main/webapp/WEB-INF/views
directory. - Add the following content to the file:
HTML
<h1>Hello, World!</h1>
6. Build and Run:
- Build the project using your IDE’s build functionality.
- Deploy the generated WAR file to your web server (e.g., Tomcat, Jetty).
- Access the application URL (e.g., http://localhost:8080/).
Congratulations! You should now see “Hello, World!” displayed on your browser. This is a basic Spring MVC application demonstrating the MVC pattern and the interaction between controller, view, and DispatcherServlet.
Next Steps:
- Explore Spring beans and dependency injection to manage application objects.
- Add form handling and data validation to your application.
- Use databases and repositories to persist and retrieve data.
- Build more complex functionalities and user interfaces.
This is just a starting point. As you continue exploring Spring MVC, you’ll discover its powerful capabilities and flexibility for building various web applications.
- Mutual of Omaha: Selection and Interview process, Questions/Answers - April 15, 2024
- AES: Selection and Interview process, Questions/Answers - April 15, 2024
- Amphenol: Selection and Interview process, Questions/Answers - April 15, 2024