What is Swift?
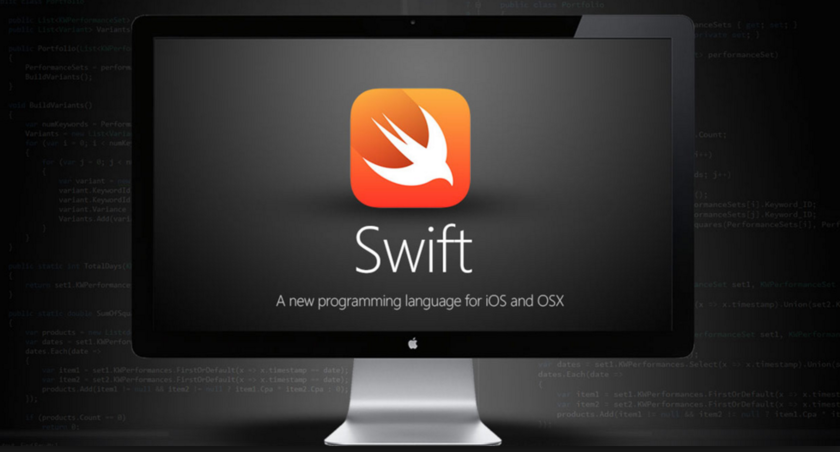
Swift is a powerful and intuitive programming language developed by Apple Inc. It was introduced in 2014 as a replacement for Objective-C and is designed to work seamlessly with Apple’s Cocoa and Cocoa Touch frameworks. Swift is known for its performance, safety, and ease of use, making it a popular choice for iOS, macOS, watchOS, and tvOS app development.
Key features of Swift include:
- Readability and Conciseness: Swift syntax is designed to be clear and expressive, making code easy to read and write. It reduces the amount of code needed to perform common tasks compared to Objective-C.
- Safety: Swift includes features like optionals, type inference, and automatic memory management (ARC) to enhance code safety and prevent common programming errors.
- Performance: Swift is designed to be fast and efficient. It achieves performance comparable to C and C++ by using modern optimization techniques.
- Interoperability: Swift is interoperable with Objective-C, allowing developers to use Swift and Objective-C code together in the same project. This is particularly useful for transitioning from existing Objective-C projects to Swift.
- Open Source: In December 2015, Apple open-sourced Swift, allowing the developer community to contribute to its development. This has led to increased adoption and support from developers outside of the Apple ecosystem.
- Playgrounds: Swift Playgrounds provide an interactive environment for experimenting with Swift code and exploring its features. It’s a great tool for learning and prototyping.
What is top use cases of Swift?
- iOS App Development: Swift is the primary language for developing applications for iOS devices such as iPhones and iPads. It is used for creating a wide range of apps, including productivity apps, games, social networking apps, and more.
- macOS App Development: Swift is also the preferred language for macOS app development. Developers use it to create applications for Mac desktops and laptops.
- watchOS App Development: Swift is used for building apps for Apple Watch, extending the functionality of iOS apps to the wrist-worn device.
- tvOS App Development: Swift is employed in the development of applications for the Apple TV platform, offering an immersive experience for television-based apps.
- Cross-Platform Development: While Swift is primarily associated with Apple platforms, there are efforts to make it more versatile for cross-platform development. Some projects, like SwiftUI and Swift for TensorFlow, aim to leverage Swift’s capabilities in different domains.
- Server-Side Development: Swift is used for server-side development, allowing developers to build backend services and APIs. Projects like Vapor and Kitura provide server-side frameworks for Swift.
- Game Development: Swift is gaining popularity in the game development community. Developers use it to build games for iOS and macOS, taking advantage of its performance and ease of use.
- Education: Swift is widely used in educational settings as an introductory programming language. Its readability and simplicity make it an excellent choice for teaching programming concepts to beginners.
- Prototyping and Experimentation: Swift Playgrounds and the interactive nature of Swift make it suitable for rapid prototyping and experimentation, allowing developers to quickly test ideas and concepts.
Overall, Swift is a versatile language with a growing ecosystem, and its use cases extend beyond app development to various domains within the software development landscape.
What are feature of Swift?
Features of Swift:
- Readability and Conciseness: Swift syntax is designed to be clear and concise, making code more readable and reducing boilerplate code.
- Safety: Swift incorporates modern programming concepts to reduce common programming errors. Features like optionals and automatic memory management (ARC) enhance code safety.
- Performance: Swift is designed for high performance and efficiency, comparable to languages like C and C++. It achieves this through optimization techniques and a focus on low-level control.
- Interoperability: Swift is interoperable with Objective-C, allowing developers to use both languages within the same project. This is particularly useful for transitioning from existing Objective-C codebases.
- Open Source: Swift is an open-source programming language, encouraging community collaboration and contributions. This has led to the development of various libraries, frameworks, and tools by the Swift community.
- Playgrounds: Swift Playgrounds provide an interactive and visual environment for writing Swift code, making it easy for developers to experiment, prototype, and learn.
- Functional Programming Features: Swift supports functional programming paradigms, including first-class functions, closures, and immutability, providing expressive ways to write code.
- Optionals: Swift uses optionals to represent the absence of a value. This helps in handling situations where a variable might not have a value, reducing unexpected crashes.
- Type Inference: Swift uses type inference to determine the data type of a variable based on its value. This reduces the need for explicit type declarations, making the code more concise.
- Pattern Matching: Swift includes powerful pattern matching capabilities, allowing developers to write expressive code for complex conditional statements.
- Generics: Swift supports generics, enabling developers to write flexible and reusable code by creating functions and data types that can work with any type.
- Memory Management (ARC): Automatic Reference Counting (ARC) is used in Swift to automatically manage memory, reducing the likelihood of memory leaks.
- Access Control: Swift provides access control mechanisms to restrict the access of certain parts of code, enhancing code modularity and security.
What is the workflow of Swift?
Workflow of Swift:
- Project Setup:
- Create a new Swift project using Xcode, Apple’s integrated development environment (IDE).
- Choose the project template based on the type of application you are building (iOS app, macOS app, etc.).
- Coding:
- Write Swift code to define the application’s logic and functionality.
- Use Swift syntax to create classes, functions, and data structures.
- Leverage Swift’s modern features like optionals, generics, and functional programming constructs.
- Interface Design:
- Design the user interface using Interface Builder in Xcode for visual layout (iOS/macOS development).
- Connect UI elements to Swift code using Interface Builder or programmatically.
- Testing:
- Write unit tests to protect the correctness of your code.
- Utilize Xcode’s testing framework to run tests and identify any issues.
- Debugging:
- Use Xcode’s debugging tools to identify and fix bugs in your code.
- Utilize breakpoints, the debugger console, and visual debugging tools.
- Building and Compilation:
- Build the project to compile the Swift code into machine-readable binary code.
- Check for any build errors and resolve them.
- Running on Simulator or Device:
- Run the application on a simulator for testing or deploy it to a physical device.
- Monitor the application’s performance and behavior.
- Optimization:
- Analyze the performance of the application using profiling tools in Xcode.
- Optimize code for better performance and responsiveness.
- Version Control:
- Use version control systems (e.g., Git) to track changes in the codebase.
- Collaborate with team members using version control.
- Deployment:
- Prepare the application for distribution by configuring settings in Xcode.
- Submit the application to the App Store (iOS/macOS) or distribute it through other channels.
- Documentation:
- Document the code using comments and generate documentation to make it understandable for other developers.
This workflow provides a general overview of the typical steps involved in developing a Swift-based application. The specifics may vary based on the type of project and the development platform (iOS, macOS, etc.).
How Swift Works & Architecture?
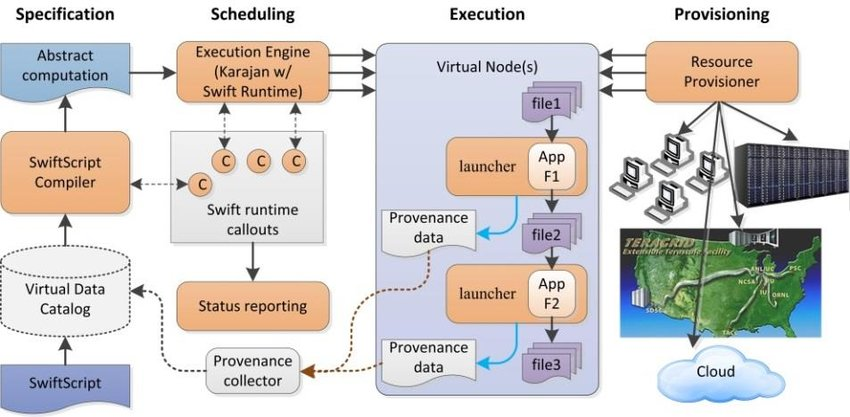
Following is an overview of how Swift works and its architecture, as well as how to install and configure Swift:
Swift Overview
Swift is a modern, multi-paradigm programming language developed by Apple for developing iOS, macOS, watchOS, tvOS, and Linux applications. It is a general-purpose language that supports object-oriented programming, functional programming, and imperative programming paradigms. Swift is designed to be safe, concise, and powerful, making it a popular choice for developing cross-platform applications.
Swift Architecture
Swift has a layered architecture that consists of the following components:
- Front-end: The front-end is responsible for parsing and compiling Swift code into LLVM bytecode. It includes the following components:
- Lexer: The lexer breaks down Swift code into tokens.
- Parser: The parser analyzes the tokens and constructs a parse tree.
- Semantic analyzer: The semantic analyzer checks the parse tree for errors and verifies that the code is semantically correct.
- Type checker: The type checker checks that types are used correctly throughout the code.
- Code generator: The code generator generates LLVM bytecode from the parse tree.
- LLVM: LLVM is a compiler infrastructure that provides a common backend for various programming languages. It includes the following components:
- IR: The IR (intermediate representation) is a low-level representation of the program that is independent of the target platform.
- Optimizer: The optimizer optimizes the IR to improve performance.
- Code generator: The code generator generates machine code from the IR.
- Back-end: The back-end is responsible for generating machine code for the target platform. It includes the following components:
- Assembler: The assembler assembles the machine code into object files.
- Linker: The linker links the object files into an executable file.
How Swift Works
When you compile a Swift program, the following steps occur:
- The front-end parses and compiles the Swift code into LLVM bytecode.
- The LLVM optimizer optimizes the bytecode to improve performance.
- The LLVM code generator generates machine code from the optimized bytecode.
- The machine code is executed by the CPU.
How to Install and Configure Swift?
Installing and configuring Swift is a straightforward process that involves downloading and installing Xcode, which includes the Swift compiler and other tools for developing iOS and macOS applications. Following is a step-by-step guide:
Prerequisites:
- MacOS 10.15 or later: Ensure your Mac is running macOS 10.15 or later. This is the minimum required operating system version for installing Xcode.
- Active Apple Developer Account (Optional): If you plan to develop apps for the App Store, you’ll need an active Apple Developer account. You can create one from the Apple Developer website.
Installation Steps:
- Download Xcode from the App Store: Open the App Store on your Mac and search for “Xcode.” Click the “Get” button to download and install Xcode.
- Accept License Agreement: After downloading, launch Xcode and review the license agreement. Press “Agree” to accept the terms and conditions.
- Choose Installation Location: Select the location where you want to install Xcode and its components. Press “Install” to begin the installation process.
- Wait for Installation: The installation process may take some time depending on your internet speed and system configuration. Once completed, Xcode will launch automatically.
- Verify Swift Installation: Open a Terminal window and type the following command to check the Swift version:
swift --version
If Swift is installed correctly, it should display the installed Swift version.
Optional Configuration:
- Environment Variables: You can set environment variables to control various aspects of Swift compilation, such as the optimization level and the output format. For example, to set the optimization level to “O3,” use the following command:
export SWIFT_OPTIMIZATION_LEVEL=O3
- Compiler Flags: You can pass compiler flags to the Swift compiler to control various aspects of compilation, such as the target platform and the debug level. For example, to compile for the iOS simulator, use the following flag:
swift -target x86_64-apple-ios-simulator
- Xcode Project Settings: You can set project-specific configuration options in Xcode, such as the Swift language version and the code signing settings. These settings can be found in the project’s build settings.
Xcode provides a comprehensive development environment for building Swift applications for iOS, macOS, watchOS, and tvOS. The Swift compiler is integrated into Xcode, making it easy to write, compile, and debug Swift code.
Fundamental Tutorials of Swift: Getting started Step by Step
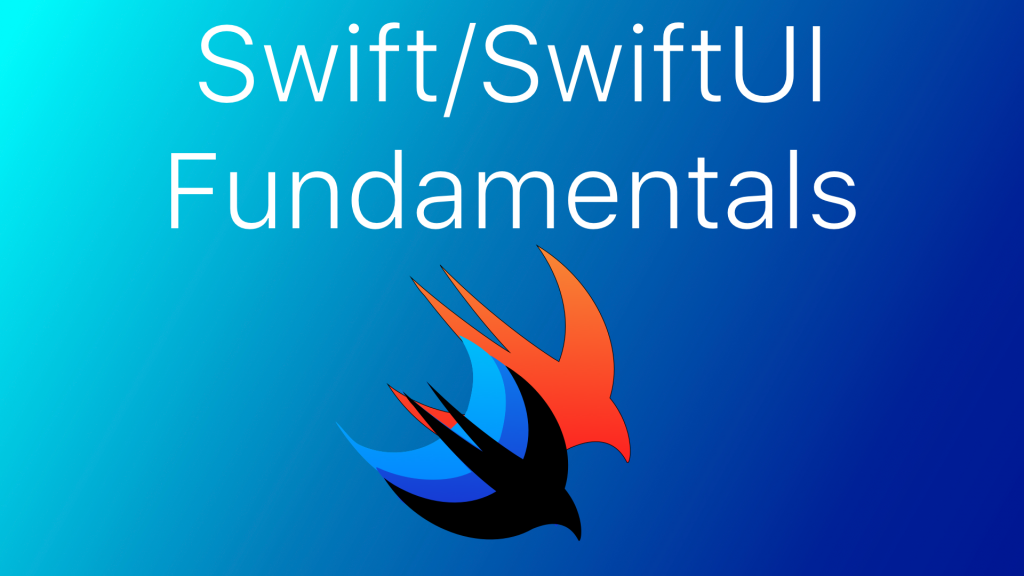
The following is a step-by-step tutorial of Swift that will teach you the basics of the language.
Introduction to Swift
Swift is a powerful and modern programming language developed by Apple for building applications for iOS, macOS, watchOS, tvOS, and Linux. It is known for its conciseness, safety, and expressiveness, making it a popular choice for developers of all levels.
Step 1: Setting Up the Development Environment
- Install Xcode: Xcode is the integrated development environment (IDE) for Swift and provides everything you need to write, compile, and debug Swift code. Download Xcode from the Mac App Store.
- Create a Playground: A playground is a self-contained environment for experimenting with Swift code. To create a playground, open Xcode and select “File” > “New” > “Playground”.
Step 2: Basic Syntax and Data Types
- Variables and Constants: Variables are used to store data that can change, while constants are used to store data that remains fixed. To declare a variable, use the
var
keyword followed by the variable name and data type. For a constant, use thelet
keyword.
var message: String = "Hello, World!"
let pi: Double = 3.14159
- Data Types: Swift supports various data types, including integers (Int), floating-point numbers (Double), strings (String), and booleans (Bool).
let age: Int = 25
let temperature: Double = 22.5
let name: String = "John Doe"
let isActive: Bool = true
Step 3: Operators and Expressions
- Arithmetic Operators: Arithmetic operators perform mathematical operations, such as addition (+), subtraction (-), multiplication (*), and division (/).
let sum = 5 + 3
let difference = 10 - 4
let product = 2 * 3
let quotient = 8 / 2
- Comparison Operators: Comparison operators compare values and return a boolean result (true or false).
let isEqual = 5 == 5
let isGreaterThan = 10 > 5
let isLessThanOrEqual = 4 <= 4
Step 4: Control Flow Statements
- Conditional Statements: Conditional statements allow you to execute code based on certain conditions. The most common conditional statement is the
if
statement.
if age >= 18 {
print("You are an adult.")
} else {
print("You are a minor.")
}
- Loops: Loops allow you to repeat a block of code multiple times. The most common loops are the
for
loop and thewhile
loop.
// `for` loop
for i in 1...5 {
print(i)
}
// `while` loop
var count = 1
while count <= 10 {
print(count)
count += 1
}
Step 5: Functions
- Defining Functions: Functions are reusable blocks of code that perform specific tasks. To define a function, use the
func
keyword followed by the function name, parameters (if any), return type (if any), and the function body.
func greet(name: String) -> String {
return "Hello, \(name)!"
}
- Calling Functions: To call a function, simply use its name followed by parentheses and any required arguments.
let greeting = greet(name: "Alice")
print(greeting) // Output: Hello, Alice!
This is a brief introduction to the basics of Swift. There are many more features and concepts to explore, and numerous resources available to help you learn more about this powerful programming language.
- Mutual of Omaha: Selection and Interview process, Questions/Answers - April 15, 2024
- AES: Selection and Interview process, Questions/Answers - April 15, 2024
- Amphenol: Selection and Interview process, Questions/Answers - April 15, 2024