What is Typescript?
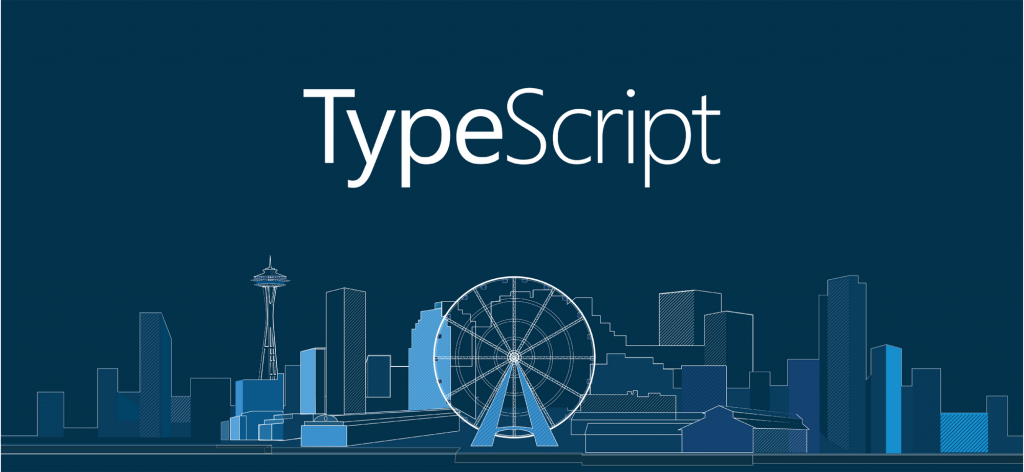
TypeScript is a superset of JavaScript elaborated by Microsoft. It adds static typing and other features to the JavaScript language, allowing developers to write more maintainable and scalable code. TypeScript code is transpiled into plain JavaScript, making it compatible with existing JavaScript environments.
Key Features of TypeScript:
- Static Typing: TypeScript introduces static typing, allowing developers to define and enforce types for variables, function parameters, and return values. This helps find errors during development and improves code quality.
- Interfaces: TypeScript supports interfaces, which enable developers to define contracts for object shapes. This promotes code consistency and helps catch potential issues early in the development process.
- Classes and Inheritance: TypeScript provides a class-based object-oriented programming model with support for inheritance, encapsulation, and other features commonly found in languages like Java or C#.
- Modules: TypeScript supports the use of modules, enabling developers to organize code into reusable and maintainable units. Modules help manage dependencies and promote a modular architecture.
- Enums: Enums allow developers to define named constant values, improving code readability and maintainability.
- Generics: TypeScript includes support for generics, allowing the creation of flexible and reusable functions, classes, and interfaces that work with various data types.
- Decorators: Decorators provide a way to annotate and modify classes and class members. They are often used in frameworks like Angular for features such as dependency injection and component metadata.
- Intersection and Union Types: TypeScript allows the creation of intersection types (combining multiple types) and union types (accepting one of several possible types), enhancing flexibility in type definitions.
What is top use cases of Typescript?
Top Use Cases of TypeScript:
- Web Development:
- TypeScript is commonly used for web development, particularly in conjunction with front-end frameworks like Angular, React, or Vue.js. It helps catch errors early in the development process and enhances the developer experience.
- Large-Scale Applications:
- TypeScript is well-suited for large-scale applications where maintainability, scalability, and code organization are critical. The static typing feature helps prevent common errors in large codebases.
- Library and Framework Development:
- Developers often use TypeScript when building libraries or frameworks to provide clear interfaces, improve documentation, and enhance the overall developer experience.
- Node.js Development:
- TypeScript is increasingly adopted in server-side development using Node.js. It brings the benefits of static typing to server-side JavaScript applications.
- Cross-Platform Development:
- TypeScript is used for cross-platform development, allowing developers to write code that can run on both the client and server sides.
- Enterprise Applications:
- Enterprises with large, complex applications benefit from TypeScript’s features, such as static typing, which helps reduce bugs and improve maintainability.
- Mobile App Development:
- TypeScript is used in mobile app development, particularly with frameworks like React Native or NativeScript, providing a typed language for building cross-platform mobile applications.
- Tooling and Build Processes:
- TypeScript is integrated into various build tools and development workflows. It is often used to improve tooling and provide better code analysis during development.
- Migration from JavaScript:
- Organizations transitioning from JavaScript to TypeScript can gradually introduce static typing, benefiting from TypeScript’s features while maintaining compatibility with existing JavaScript code.
- Education and Training:
- TypeScript is used in educational settings to teach programming concepts, especially when introducing students to modern web development practices.
TypeScript’s popularity has grown significantly, and it continues to be widely adopted in various domains, contributing to the overall improvement of JavaScript development practices.
What are feature of Typescript?
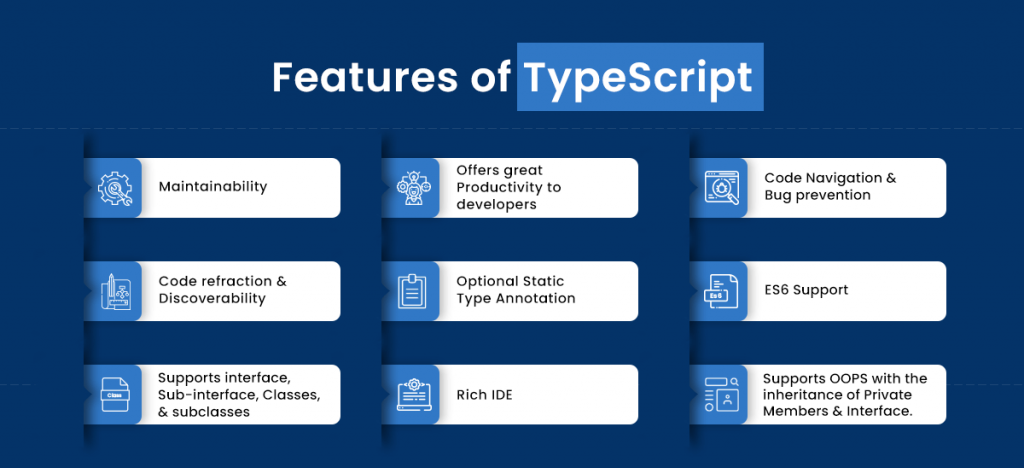
Features of TypeScript:
- Static Typing:
- TypeScript introduces static typing, allowing developers to define and enforce types for variables, function parameters, and return values. This helps find errors during development and improves code quality.
- Interfaces:
- TypeScript supports interfaces, allowing developers to define contracts for object shapes. This promotes code consistency and helps catch potential issues early in the development process.
- Classes and Inheritance:
- TypeScript provides a class-based object-oriented programming model with support for inheritance, encapsulation, and other features commonly found in languages like Java or C#.
- Modules:
- TypeScript supports the use of modules, enabling developers to organize code into reusable and maintainable units. Modules help manage dependencies and promote a modular architecture.
- Enums:
- Enums allow developers to define named constant values, improving code readability and maintainability.
- Generics:
- TypeScript includes support for generics, allowing the creation of flexible and reusable functions, classes, and interfaces that work with various data types.
- Decorators:
- Decorators provide a way to annotate and modify classes and class members. They are often used in frameworks like Angular for features such as dependency injection and component metadata.
- Intersection and Union Types:
- TypeScript allows the creation of intersection types (combining multiple types) and union types (accepting one of several possible types), enhancing flexibility in type definitions.
- Tuple Types:
- TypeScript introduces tuple types, allowing developers to specify an array where the type of each element is known.
- Literal Types:
- Literal types enable developers to specify exact values for variables, allowing for more precise type definitions.
- Optional and Default Parameters:
- TypeScript allows developers to define optional parameters in functions and provides default values for parameters.
- Type Inference:
- TypeScript has a powerful type inference system, reducing the need for explicit type annotations in many cases.
- Compatibility with JavaScript:
- TypeScript is a superset of JavaScript, meaning that existing JavaScript code is valid TypeScript. This makes it easy to gradually adopt TypeScript in existing projects.
- Tooling Support:
- TypeScript is well-supported by development tools and IDEs, providing features like code completion, navigation, and error checking.
- Declaration Files:
- TypeScript allows the use of declaration files (
.d.ts
) to describe the shape of existing JavaScript libraries and code, enabling type checking and autocompletion for external libraries.
- TypeScript allows the use of declaration files (
What is the workflow of Typescript?
- Installation:
- Start by installing TypeScript globally on your system using a package manager like npm:
npm install -g typescript
- Initialization:
- Initialize a new TypeScript project by creating a
tsconfig.json
file. This file specifies the TypeScript configuration for your project.
tsc --init
- Writing TypeScript Code:
- Write your code in TypeScript using a
.ts
file extension. Use TypeScript features such as static typing, interfaces, and classes to enhance code structure and maintainability.
// example.ts
interface Person {
name: string;
age: number;
}
function greet(person: Person): string {
return `Hello, ${person.name}! You are ${person.age} years old.`;
}
const user: Person = { name: "John", age: 25 };
console.log(greet(user));
- Compilation:
- Compile TypeScript code to JavaScript using the TypeScript compiler (
tsc
). Run the following command in the terminal:
tsc
This will generate corresponding JavaScript files.
- Execution:
- Run the generated JavaScript files using Node.js or in a browser environment, depending on your application type.
node example.js
- Development and Testing:
- Continue the development process, making use of TypeScript features to catch errors early. Use tools like IDEs, linters, and testing frameworks for a robust development workflow.
7. Module Resolution:
- If your project involves multiple TypeScript files, use module resolution to organize and manage dependencies.
8. Declaration Files for External Libraries:
- If using external JavaScript libraries, obtain or create declaration files (
.d.ts
) to provide type information for those libraries.
9. Debugging:
- Debug TypeScript code using developer tools in browsers or integrated debugging tools in your chosen development environment.
10. Deployment:
- Deploy the compiled JavaScript code to your production environment.
The TypeScript workflow involves writing code with enhanced features, compiling it to JavaScript, and executing it in the desired environment. TypeScript provides a smooth transition from JavaScript, adding static typing and other features without requiring a complete rewrite of existing code.
How TypeScript Works & Architecture?
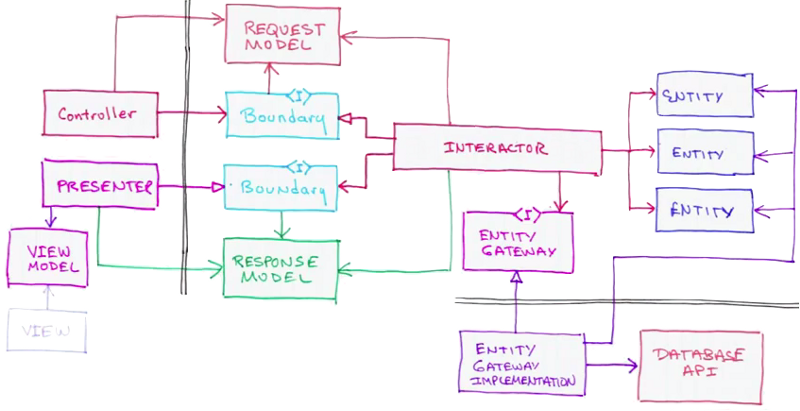
TypeScript is a superset of JavaScript that adds optional static typing. This means you can choose to annotate your code with types, which can help improve the accuracy and development experience. Following is a breakdown of how TypeScript works and its architecture:
1. Compiler:
- The core of TypeScript is the compiler, which takes your TypeScript code and transpiles it into plain JavaScript code.
- The compiler performs several tasks:
- Type checking: Ensures your code adheres to the specified types.
- Erasing type annotations: Removes type annotations from the compiled JavaScript code.
- Generating JavaScript code: Converts the TypeScript code into JavaScript that can be run by any JavaScript engine.
2. Type System:
- TypeScript adds a powerful type system to JavaScript.
- This type system allows you to define the types of variables, functions, objects, and other constructs in your code.
- Types provide several benefits:
- Improved code clarity: Makes your code easier to understand and maintain.
- Reduced errors: Catches type errors at compile time, preventing runtime errors.
- Improved IDE support: Enables features like code completion and type checking within IDEs.
3. Architectural Overview:
- TypeScript’s architecture is composed of several key components:
- Scanner: Reads and tokenizes the source code.
- Parser: Converts the tokenized source code into an abstract syntax tree (AST).
- Binder: Resolves references and binds names to their declarations.
- Checker: Performs type checking and reports any type errors.
- Emitter: Generates the final JavaScript code.
4. Benefits of using TypeScript:
- Improved code quality: Type checking helps to catch errors early, preventing bugs and improving code stability.
- Increased developer productivity: Code completion and type checking in IDEs can significantly improve development speed.
- Enhanced maintainability: Types make code easier to understand and modify, improving long-term maintainability.
- Better tooling support: Many popular tools and libraries are specifically designed or optimized for TypeScript.
5. Getting started with TypeScript:
- Install the TypeScript compiler:
npm install -g typescript
- Create a TypeScript file (e.g.,
my-file.ts
) - Write your code with type annotations
- Compile the TypeScript code:
tsc my-file.ts
- Run the generated JavaScript code (e.g.,
node my-file.js
)
By understanding how TypeScript works and its architecture, you can leverage its benefits to write better, more robust, and maintainable JavaScript code.
How to Install and Configure TypeScript?
Installing and Configuring TypeScript:
Here’s how to install and configure TypeScript:
1. Installation:
There are two primary ways to install TypeScript:
– Globally:
Bash
npm install -g typescript
This installs the TypeScript compiler globally, allowing you to compile TypeScript files from any directory.
– Locally:
Bash
npm install typescript --save-dev
This installs the TypeScript compiler as a development dependency for your project. This is recommended for most projects, as it allows you to manage the TypeScript version alongside your other project dependencies.
2. Configuration:
While TypeScript can be used without any configuration, creating a tsconfig.json
file provides several benefits:
- Specifying compiler options: You can control various aspects of the compilation process, such as the target JavaScript version, source maps, and type checking strictness.
- Defining compiler flags: You can use compiler flags to enable specific features or suppress warnings.
- Configuring project structure: You can define the root directory of your project and specify which files should be compiled.
Here’s a basic example of a tsconfig.json
file:
JSON
{
"compilerOptions": {
"target": "es5",
"sourceMap": true,
"strict": true
},
"include": ["src/**/*.ts"]
}
This configuration identifies that the target JavaScript version is ES5, creates source maps, enables strict type checking, and includes all TypeScript files in the src
directory and its subdirectories for compilation.
3. Compiling TypeScript:
Once you’ve installed and configured TypeScript, you can compile your TypeScript files using the following command:
Bash
tsc [filename.ts]
This command will generate the corresponding JavaScript file. You can also use the -w
flag to enable watch mode, which automatically recompiles the TypeScript files whenever they are saved.
4. Using TypeScript with tools:
Many popular tools and frameworks support TypeScript natively, providing features like code completion, type checking within IDEs, and integration with other build tools. Some of these tools include:
- Visual Studio Code
- WebStorm
- Webpack
- Parcel
By following these steps and exploring the provided resources, you’ll be well-equipped to start using TypeScript and leverage its powerful features to take your JavaScript development to the next level.
Fundamental Tutorials of TypeScript: Getting started Step by Step
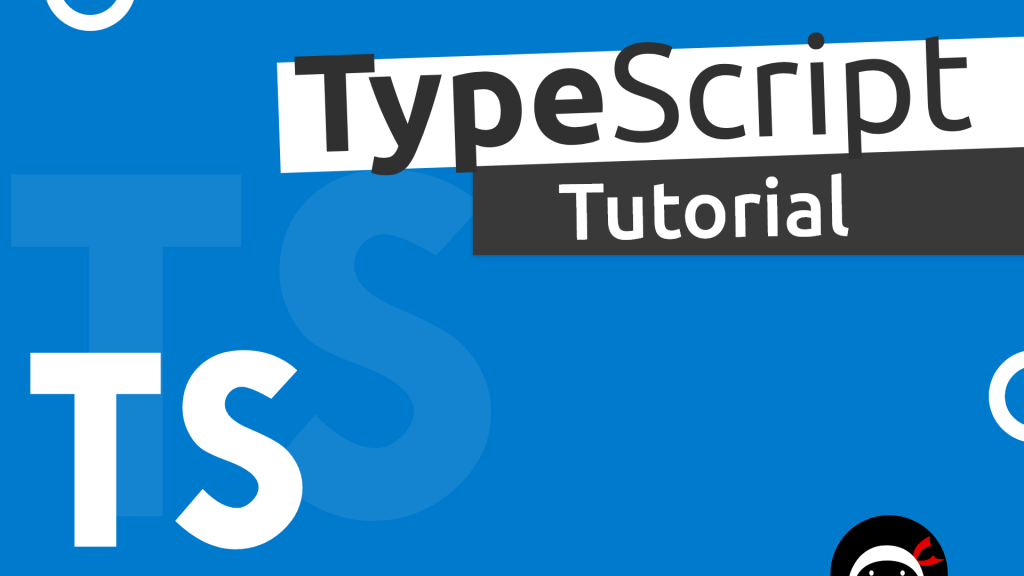
Following is a step-by-step Basic Tutorials of TypeScript:
1. Setting Up Your Development Environment:
- Install Node.js
- Install TypeScript:
npm install -g typescript
- Choose a code editor or IDE: Visual Studio Code, WebStorm, Atom, etc.
- Create a TypeScript file:
touch my-file.ts
2. Basic Types:
number
: Integers and decimals (e.g., 1, 3.14)string
: Text data (e.g., “Hello, World!”)boolean
: True or false values (e.g., true, false)null
: Represents the absence of a valueundefined
: Represents a value that is not defined
3. Variables and Type Annotations:
- Declare variables with type annotations (e.g.,
let name: string = "John";
) - Use built-in types or create custom types (e.g.,
interface Person { name: string; age: number; }
)
4. Functions:
- Define functions with type annotations for parameters and return values (e.g.,
function add(a: number, b: number): number { return a + b; }
) - Use optional parameters and destructuring for function arguments
5. Objects and Arrays:
- Define objects with properties and types (e.g.,
const user: { name: string; age: number; } = { name: "Jane", age: 30 };
) - Create arrays with specific element types (e.g.,
const numbers: number[] = [1, 2, 3];
) - Access and manipulate elements using indexing and methods
6. Operators and Control Flow:
- Use standard arithmetic, comparison, and logical operators
- Write conditional statements (e.g.,
if
,else
,switch
) - Iterate through loops (e.g.,
for
,while
)
7. Classes and Interfaces:
- Define classes with properties and methods (e.g.,
class Person { name: string; age: number; constructor(name: string, age: number) { this.name = name; this.age = age; } }
) - Use interfaces to define contracts for classes (e.g.,
interface User { name: string; age: number; }
) - Implement inheritance and abstract classes
8. Debugging and Testing:
- Use debugger statements and browser developer tools to identify and fix errors
- Write unit tests to ensure code functionality
9. Next Steps:
- Explore advanced TypeScript features like generics, decorators, and modules
- Use popular libraries and frameworks built with TypeScript
- Build real-world applications with TypeScript
Points to Remember:
- Start with the basics and gradually progress to more complex concepts.
- Practice regularly to solidify your understanding.
- Utilize online resources and communities for help and support.
By following these steps and utilizing the provided resources, you’ll be well on your way to mastering the fundamentals of TypeScript and unlocking its full potential for building robust and maintainable JavaScript applications.
- Mutual of Omaha: Selection and Interview process, Questions/Answers - April 15, 2024
- AES: Selection and Interview process, Questions/Answers - April 15, 2024
- Amphenol: Selection and Interview process, Questions/Answers - April 15, 2024