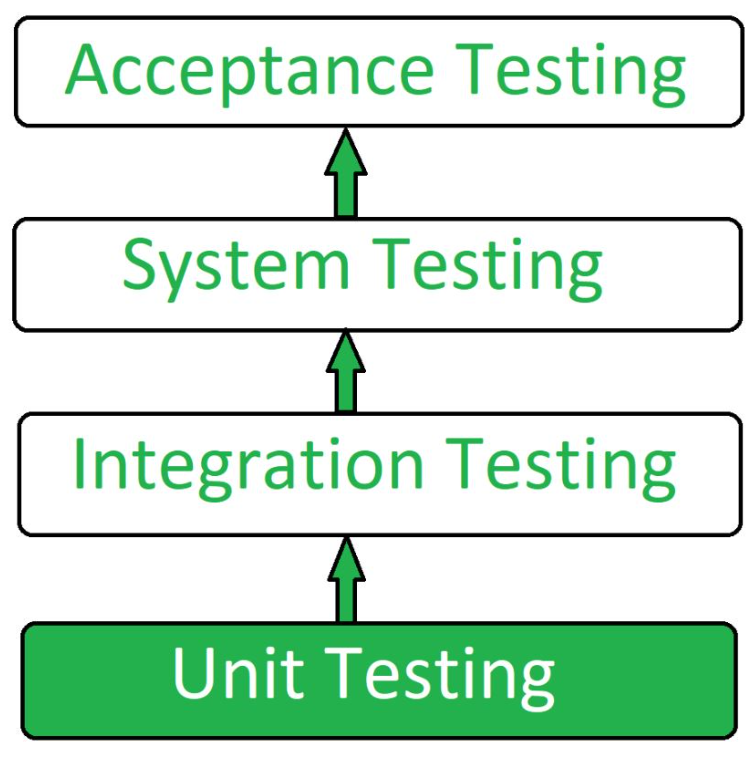
What is Unit Testing?
Unit testing is a software testing method by which individual units of source code—sets of one or more computer program modules together with associated control data, usage procedures, and operating procedures—are tested to determine whether they are fit for use. It is a standard step in development and implementation approaches such as Agile.
Unit tests are typically automated tests written and run by software developers to ensure that a section of an application (known as the “unit”) meets its design and behaves as intended. Unit tests are typically written before the code they are testing is written, and they are run frequently as the code is developed and modified.
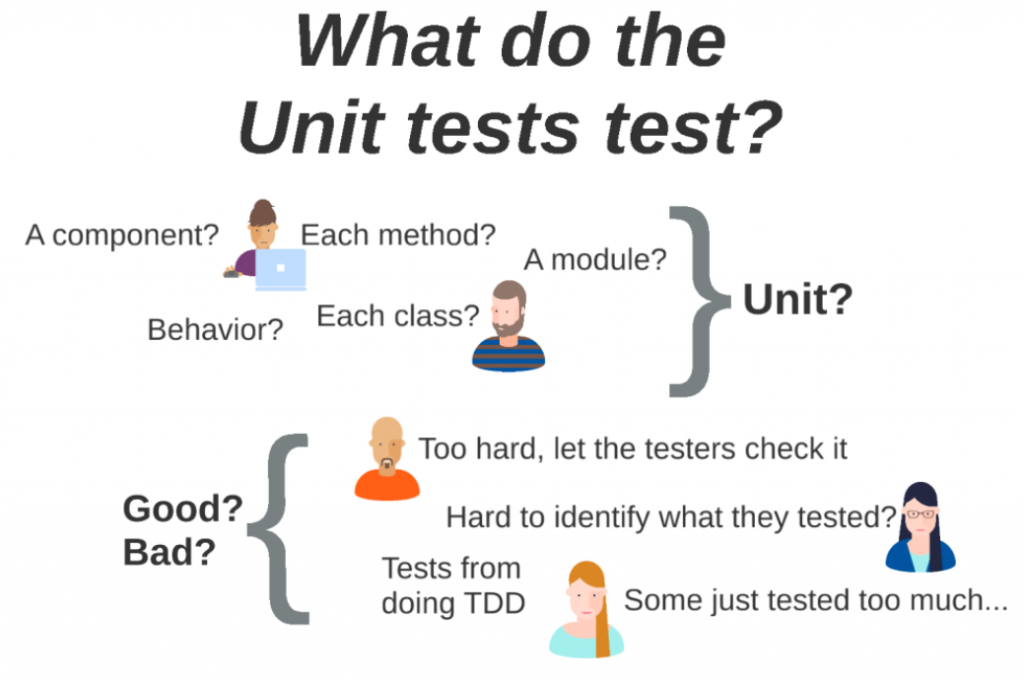
Unit tests can be used to test a wide variety of things, such as:
- The functionality of individual functions and methods
- The behavior of classes and objects
- The interaction between different parts of the code
- The correctness of algorithms and data structures
Unit testing is an important part of the software development process because it can help to catch bugs early and prevent them from causing problems in production. Unit testing can also help to improve the quality of the code by making it more reliable and maintainable.
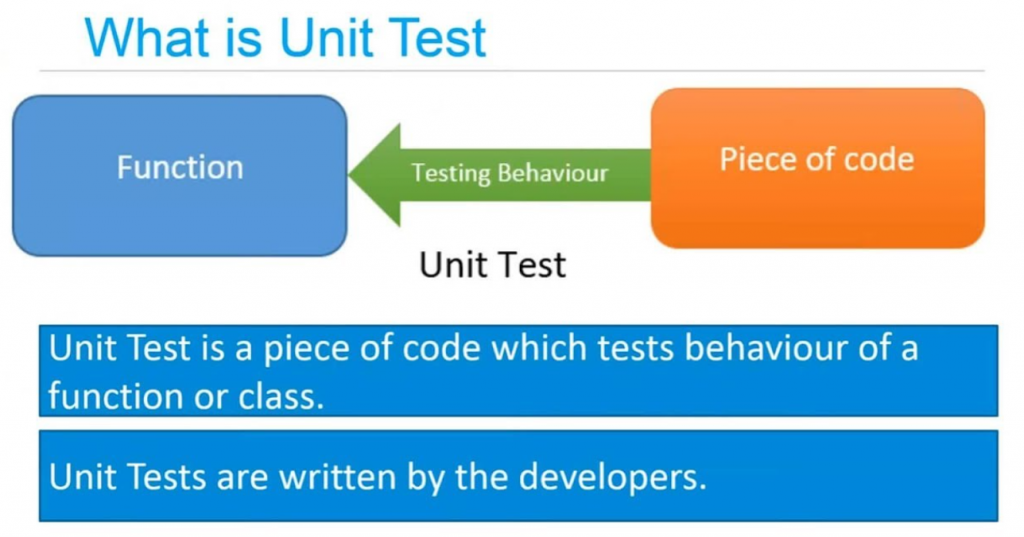
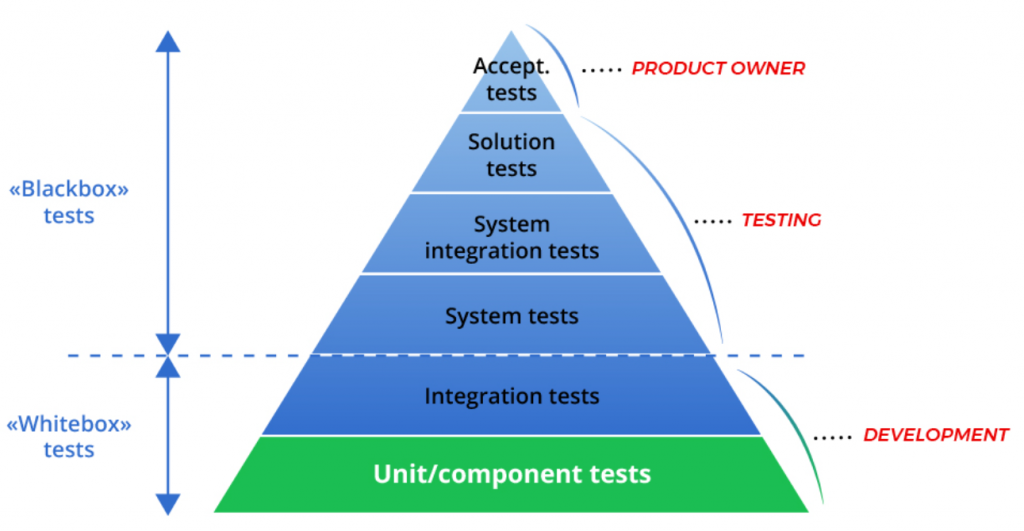
Where Unit Testing Stands in SDLC?
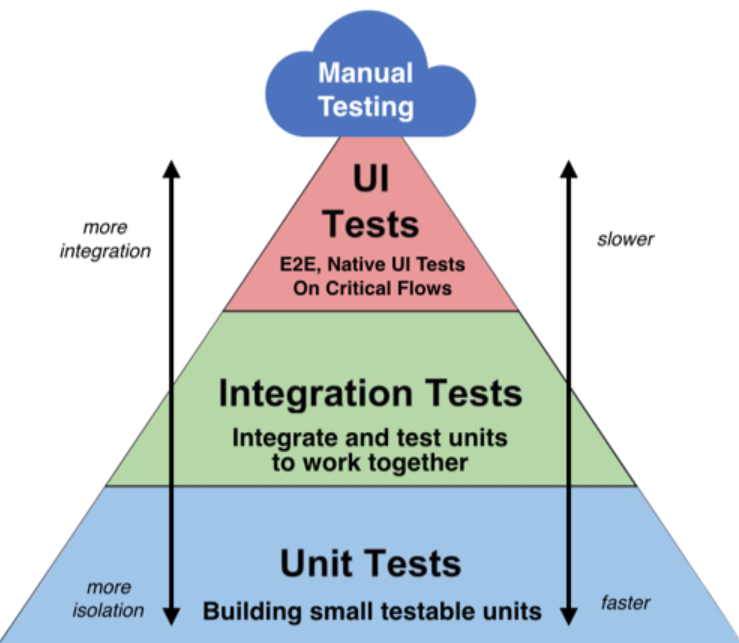
Benefits of unit testing
Here are some of the benefits of unit testing:
- Reduces the number of bugs in production: Unit tests can help to catch bugs early in the development process, before they have a chance to cause problems in production. This can save time and money in the long run.
- Improves the quality of code: Unit testing can help to improve the quality of code by making it more reliable and maintainable. Well-written unit tests can help to ensure that the code is working as expected and that it is easy to understand and modify.
- Increases developer confidence: Unit tests can help to increase developer confidence in the code. By having a suite of unit tests that pass, developers can be confident that their code is working as expected. This can lead to less stress and more productive development.
Overall, unit testing is an important tool that can help to improve the quality of software development.
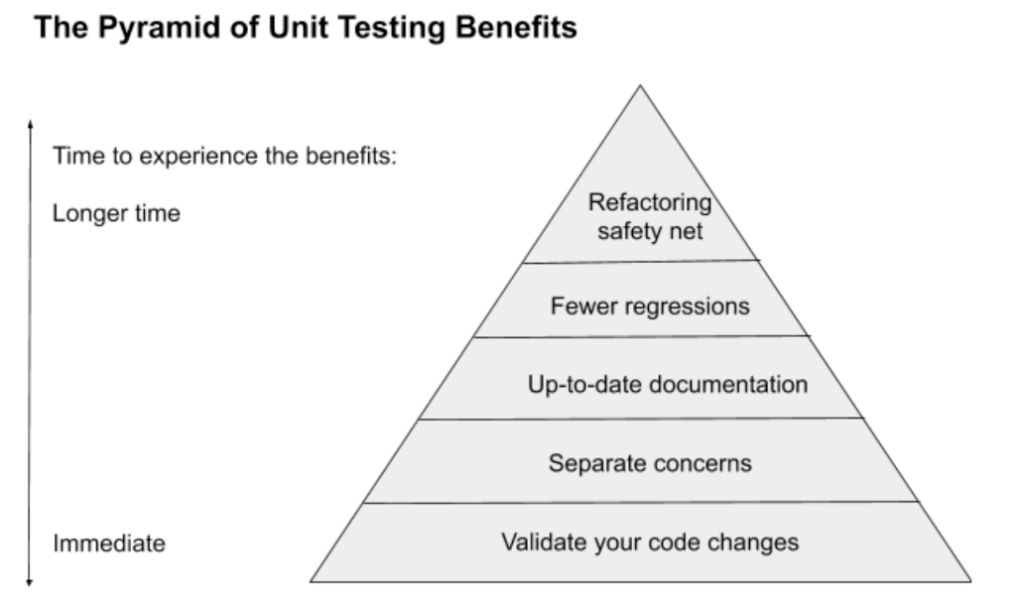
Lifecycle of Unit Testing
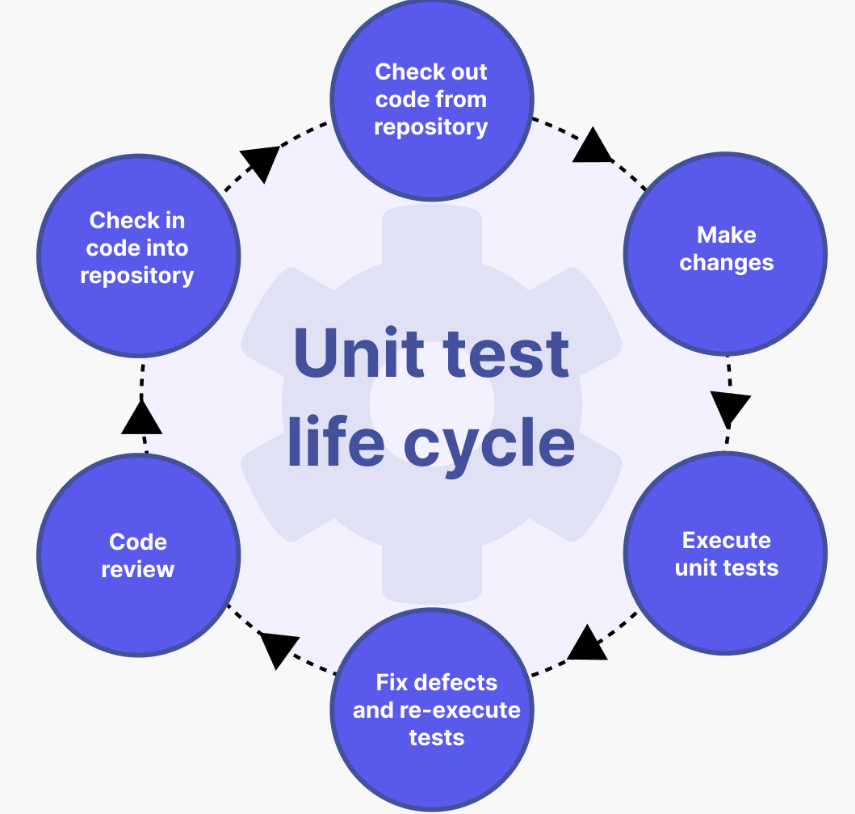
How Unit Test works?
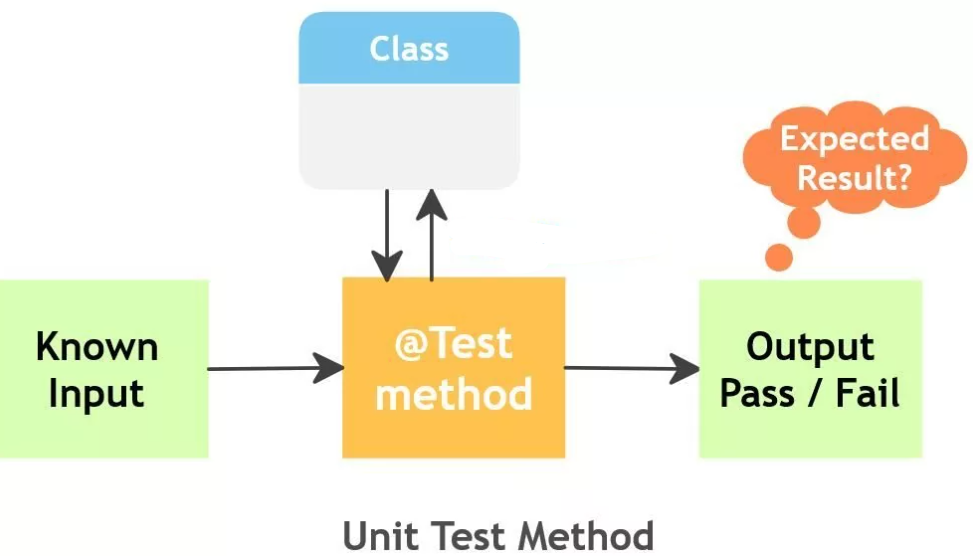
Unit testing is a software testing technique that focuses on verifying the correctness of individual units or components of a program in isolation. The goal of unit testing is to ensure that each unit of code (usually a function or method) behaves as expected. Here’s how unit testing works:
- Isolation:Unit testing emphasizes the isolation of individual units of code. Each unit is tested independently of the rest of the program. This isolation ensures that the behavior of one unit doesn’t affect the behavior of another, making it easier to pinpoint and fix issues.
- Test Cases:For each unit of code, you write one or more test cases. A test case is a set of inputs, the expected output, and any specific conditions that need to be met for the test to pass. These test cases represent various scenarios and edge cases that the unit should handle correctly.
- Test Execution:Unit tests are executed automatically by a testing framework or test runner. In Python, the
unittest
framework is commonly used, but there are other testing frameworks likepytest
,nose
, and more. The testing framework runs the test cases and checks whether the actual output matches the expected output. - Assertions:Within each test case, you use assertions to check whether the unit under test behaves as expected. Assertions are statements that verify specific conditions. If the condition is true, the test passes; if it’s false, the test fails.Common assertions include:
assertEqual(a, b)
: Checks ifa
is equal tob
.assertNotEqual(a, b)
: Checks ifa
is not equal tob
.assertTrue(expr)
: Checks if the expressionexpr
is true.assertFalse(expr)
: Checks if the expressionexpr
is false.assertRaises(exception, callable, *args, **kwargs)
: Checks if callingcallable
with the provided arguments raises the specifiedexception
.
- Test Results:After running all the test cases for a unit, the testing framework reports the results. A test can be one of the following:
- Pass: The actual output matches the expected output, and the test case succeeds.
- Fail: The actual output does not match the expected output or an assertion condition is not met, resulting in a test case failure.
- Error: An unexpected error occurred during test execution, typically due to an unhandled exception.
- Fixing Failures:When a test case fails, you need to examine the failure details, such as the expected and actual values, to diagnose the issue. You then make the necessary code changes to fix the problem and rerun the tests to ensure that the issue is resolved.
- Regression Testing:Unit tests serve as a form of regression testing. Once a unit is tested and working correctly, you can run the unit tests whenever you make changes to the code to ensure that new changes do not introduce regressions or break existing functionality.
- Test Coverage:Test coverage measures how much of your code is exercised by your unit tests. The goal is to achieve high test coverage to ensure that most parts of your code are tested. Various tools can help you measure and visualize test coverage.
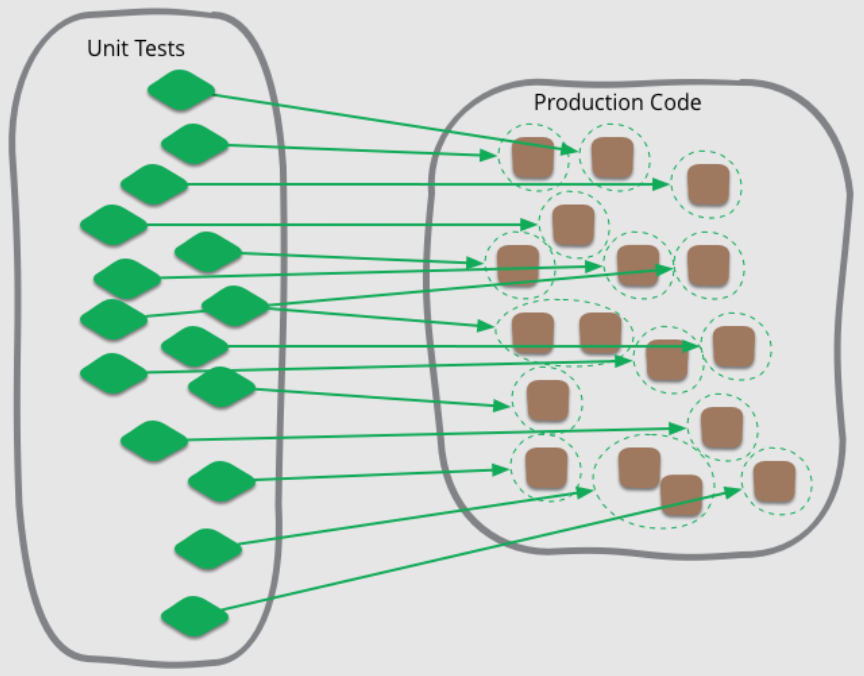
Difference Tools for Unit Testing
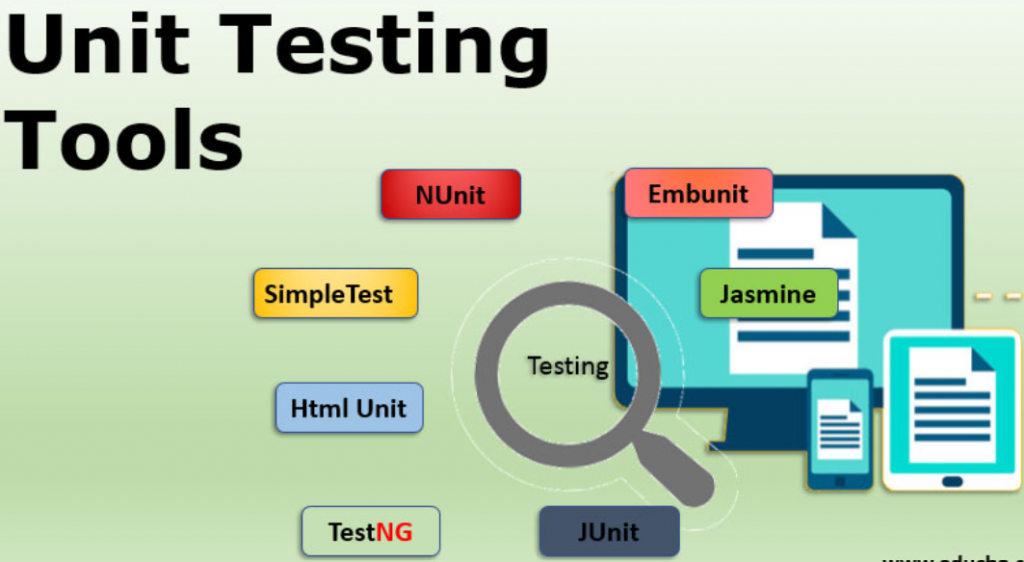
Language | Unit Testing Tools |
---|---|
Python | unittest, pytest |
Java | JUnit, TestNG |
C++ | Google Test, Catch2, CppUnit |
C# | NUnit, xUnit.net |
JavaScript | Jest, Mocha, Jasmine |
PHP | PHPUnit, Codeception |
Go | testify, go-test, gocov |
Ruby | RSpec, minitest |
Swift | XCTest |
Kotlin | JUnit, Kotlinx Test |
Scala | ScalaTest, JUnit |
TypeScript | Jest, Mocha, Jasmine |
One example of Unit test in Python?
math_functions.py
# math_functions.py
def add(a, b):
return a + b
# test_math_functions.py
import unittest
from math_functions import add # Import the function you want to test
class TestMathFunctions(unittest.TestCase):
def test_addition(self):
result = add(2, 3)
self.assertEqual(result, 5)
def test_negative_numbers(self):
result = add(-1, -2)
self.assertEqual(result, -3)
def test_mixed_numbers(self):
result = add(5, -3)
self.assertEqual(result, 2)
if __name__ == '__main__':
unittest.main()
$ python -m unittest test_math_functions.py
One example of Unit test in Java?
// MathFunctions.java
public class MathFunctions {
public int add(int a, int b) {
return a + b;
}
}
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class MathFunctionsTest {
@Test
public void testAddition() {
MathFunctions mathFunctions = new MathFunctions();
int result = mathFunctions.add(2, 3);
assertEquals(5, result);
}
@Test
public void testNegativeNumbers() {
MathFunctions mathFunctions = new MathFunctions();
int result = mathFunctions.add(-1, -2);
assertEquals(-3, result);
}
@Test
public void testMixedNumbers() {
MathFunctions mathFunctions = new MathFunctions();
int result = mathFunctions.add(5, -3);
assertEquals(2, result);
}
}
mvn test
FAQ on Unit Testing
Here are some frequently asked questions (FAQs) related to unit testing:
- What is unit testing?Unit testing is a software testing technique where individual units or components of a software application are tested in isolation. The goal is to ensure that each unit of code (such as functions or methods) behaves as expected.
- Why is unit testing important?Unit testing is important because it helps catch and fix bugs early in the development process, improves code quality, increases confidence in code changes, and provides documentation for how code should work.
- What is the difference between unit testing and integration testing?Unit testing focuses on testing individual units of code in isolation, while integration testing verifies that multiple units work correctly when combined. Integration testing checks interactions between components.
- What should you test in unit testing?In unit testing, you should test the smallest testable parts of your code, typically functions or methods. Test cases should cover various scenarios, including normal cases, edge cases, and error cases.
- What is a test case in unit testing?A test case in unit testing is a set of inputs, the expected output, and any specific conditions that need to be met for the test to pass. Test cases help verify the correctness of the code.
- What are test fixtures in unit testing?Test fixtures are the setup and teardown code that prepares the environment for running tests and cleans up after tests have finished. Fixtures ensure that tests start in a known state and do not affect each other.
- What are assertions in unit testing?Assertions are statements within test cases that verify specific conditions. If an assertion condition is met, the test passes; otherwise, it fails. Assertions are used to check whether the code behaves as expected.
- What is a testing framework, and why use one?A testing framework (e.g., JUnit for Java, pytest for Python) provides tools and conventions for writing, organizing, and running tests. Using a testing framework simplifies test setup, execution, and reporting.
- What is test coverage?Test coverage measures the extent to which your code is exercised by your unit tests. It helps identify areas of code that are not tested and ensures that most parts of your code are tested.
- Should unit tests be fast?Yes, unit tests should be fast because they are typically run frequently during development. Fast tests encourage developers to run them frequently, enabling early bug detection and quicker feedback.
- What are mock objects in unit testing?Mock objects are placeholders for real objects used to simulate their behavior. They are often used to isolate the unit under test by replacing complex or external dependencies.
- How often should you write unit tests?It’s recommended to write unit tests as you develop your code. Adopting a test-driven development (TDD) approach, where tests are written before implementing code, can help ensure comprehensive test coverage.
- What is regression testing, and how does it relate to unit testing?Regression testing is the practice of re-running tests to ensure that new code changes do not introduce regressions (new bugs) or break existing functionality. Unit tests play a crucial role in regression testing.
- Can you automate unit tests?Yes, unit tests can be automated, and automation is common practice. Automated tests can be run repeatedly to ensure code correctness and stability.
- What are some popular unit testing frameworks?Some popular unit testing frameworks include JUnit (Java), pytest (Python), NUnit (.NET), Jasmine (JavaScript), and PHPUnit (PHP), among others.
- How Email Marketing and Website Development Join Forces to Redefine Marketing Success - April 4, 2024
- Newrelic Tutorials: Critical Violation - April 2, 2024
- What is custom software development? - March 31, 2024
It is very helpful for every person, and I truly understand how to do unit testing and the different types of steps involved in it. Once again, thanks for uploading this helpful blog.
Thanks for sharing blog ,the testing is essential for developer to test the source code behavoiur and functionality before deploying the code on production to bug free test code so that we maintain software life cycle
Thanks for sharing the blog to know about importance of testing for developer to bug free source code is essential part before deploying code in production