What is Vue.js?
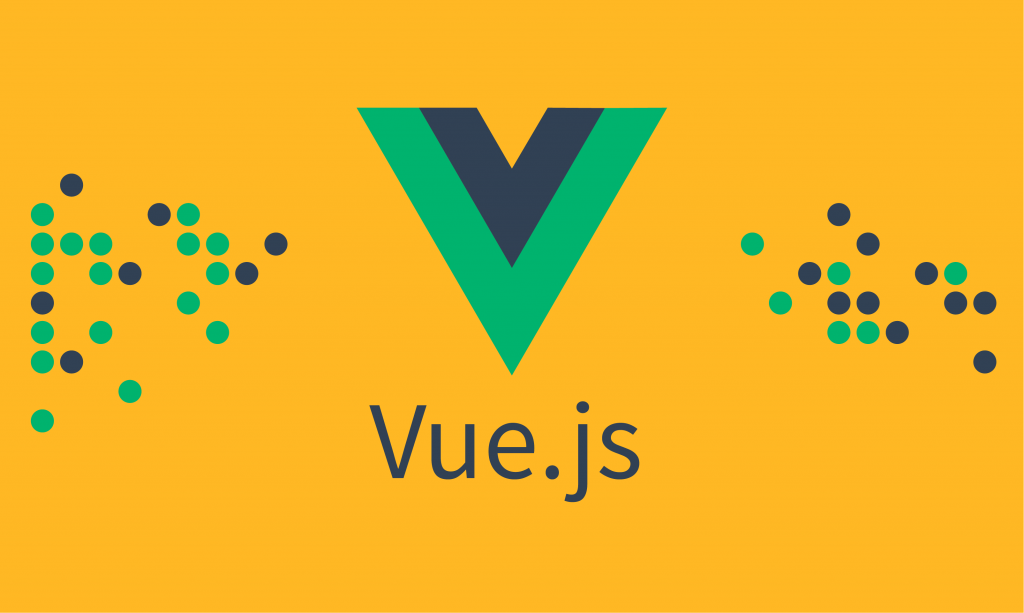
Vue.js is a moderate JavaScript framework for building user interfaces. It is often referred to as a “progressive framework” because it can be incrementally adopted. Vue.js is designed to be flexible, scalable, and efficient, making it a popular choice for developing modern web applications. Vue.js is known for its simplicity, ease of integration, and a reactive data-binding system.
Key Features of Vue.js:
- Reactive Data Binding:
- Vue.js provides a reactive data-binding system, allowing developers to declaratively describe the UI based on the underlying data model. When the data changes, the UI automatically upgrades.
- Component-Based Architecture:
- Vue.js follows a component-based architecture, allowing developers to build reusable and modular components. Components encapsulate both the structure and behavior of elements.
- Directives:
- Vue.js comes with a set of built-in directives, such as
v-if
,v-for
, andv-bind
, which allow developers to manipulate the DOM and bind data to the UI declaratively.
- Vue.js comes with a set of built-in directives, such as
- Template Syntax:
- Vue.js uses an HTML-based template syntax that allows developers to bind variables, iterate over lists, and apply conditional logic directly within the HTML markup.
- Vue CLI (Command Line Interface):
- Vue CLI is a command-line tool that simplifies the process of creating, configuring, and managing Vue.js projects. It includes features like project scaffolding, build configurations, and more.
- Vuex (State Management):
- Vuex is the official state management library for Vue.js. It helps manage the state of the application in a predictable way, particularly in large and complex applications.
- Vue Router:
- Vue Router is the official router for Vue.js, providing navigation and routing capabilities for single-page applications (SPAs).
- Computed Properties:
- Vue.js allows the creation of computed properties, which are derived from the reactive data model. Computed properties are updated automatically when their dependencies change.
- Lifecycle Hooks:
- Vue.js provides lifecycle hooks that allow developers to execute code at different stages of a component’s lifecycle, such as before the component is created, mounted, updated, or destroyed.
- Custom Directives and Plugins:
- Vue.js allows the creation of custom directives and plugins, extending its functionality to meet specific project requirements.
What is top use cases of Vue.js?
Top Use Cases of Vue.js:
- Single-Page Applications (SPAs):
- Vue.js is well-suited for building SPAs where dynamic user interfaces and smooth transitions are crucial. Vue Router simplifies the implementation of client-side navigation.
- User Interfaces (UIs):
- Vue.js is commonly used for building interactive and dynamic user interfaces. Its component-based architecture makes it easy to manage complex UI structures.
- Prototyping and Rapid Development:
- Vue.js is suitable for prototyping and rapid development due to its simplicity and ease of integration. Developers can incrementally adopt Vue.js into existing projects.
- Small to Medium-Sized Projects:
- Vue.js is often preferred for small to medium-sized projects where a lightweight and flexible framework is desirable. It offers a balance between features and simplicity.
- E-commerce Websites:
- Vue.js is used in the development of e-commerce websites to create dynamic product listings, shopping carts, and seamless user experiences.
- Content Management Systems (CMS):
- Vue.js can be integrated into content management systems to enhance the user interface and provide a more interactive and responsive experience for content creators.
- Dashboards and Analytics Tools:
- Vue.js is suitable for building dashboards and analytics tools where real-time data visualization and manipulation are essential.
- Cross-Platform Applications:
- With tools like Vue Native and Quasar, Vue.js can be used to build cross-platform applications for mobile devices, leveraging a single codebase.
- Collaborative Tools and Applications:
- Vue.js is employed in collaborative tools and applications, such as project management tools and collaborative document editing platforms.
- Dynamic Forms:
- Vue.js is effective for creating dynamic and responsive forms that update based on user input. Its reactivity system simplifies form management.
- Real-Time Updates:
- Vue.js is used in applications that require real-time updates, such as chat applications and collaboration platforms, where changes in data should be reflected immediately.
- Community Websites and Forums:
- Vue.js is chosen for building community websites and forums that require an interactive user interface and dynamic content updates.
Vue.js’s flexibility and simplicity make it a versatile choice for a wide range of applications, from small projects to large-scale applications, and it is often selected for its ease of integration and learning curve.
What are feature of Vue.js?
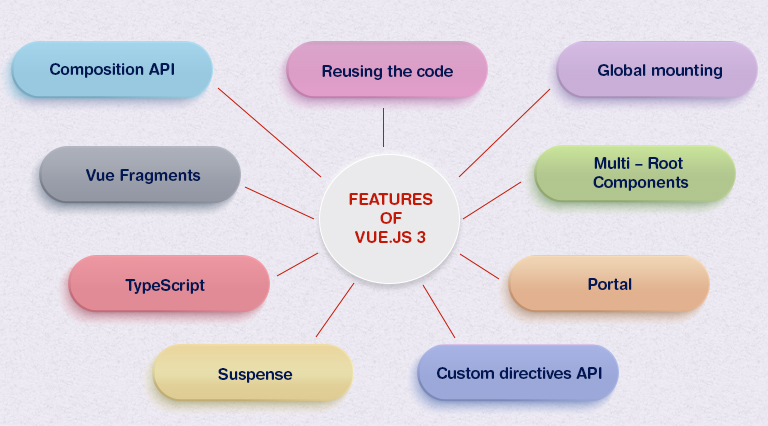
Vue.js comes with a set of features that make it a popular and versatile JavaScript framework for building user interfaces. Here are some key features of Vue.js:
- Reactive Data Binding:
- Vue.js employs a reactive data-binding system. When the underlying data changes, the UI is automatically updated to reflect those changes. This simplifies the process of managing and updating the user interface.
- Component-Based Architecture:
- Vue.js follows a component-based architecture, allowing developers to create modular and reusable components. Each component encapsulates its own logic, template, and styles, promoting maintainability and reusability.
- Vue Directives:
- Vue.js provides a set of built-in directives that allow developers to bind data to the DOM, conditionally render elements, iterate over lists, and more. Directives are prefixed with “v-” and provide declarative syntax for common tasks.
- Vue CLI (Command Line Interface):
- Vue CLI is a command-line tool that simplifies the process of creating, configuring, and managing Vue.js projects. It includes project scaffolding, build configurations, and development server options.
- Vue Router:
- Vue Router is the official routing solution for Vue.js applications. It enables the creation of single-page applications (SPAs) with client-side navigation and dynamic route handling.
- Vuex (State Management):
- Vuex is the formal state management library for Vue.js applications. It provides a centralized state management pattern, helping manage shared state in a predictable way, especially in large applications.
- Computed Properties:
- Vue.js allows the creation of computed properties. These properties are derived from the underlying data model and are automatically updated when their dependencies change. Computed properties are useful for performing calculations based on data.
- Lifecycle Hooks:
- Vue.js provides a set of lifecycle hooks that allow developers to execute code at different stages of a component’s lifecycle. This includes hooks like
beforeCreate
,created
,mounted
,updated
, anddestroyed
.
- Vue.js provides a set of lifecycle hooks that allow developers to execute code at different stages of a component’s lifecycle. This includes hooks like
- Event Handling:
- Vue.js supports handling DOM events and custom events in a declarative manner. It provides the
v-on
directive for binding event handlers.
- Vue.js supports handling DOM events and custom events in a declarative manner. It provides the
- Template Syntax:
- Vue.js uses an HTML-based template syntax that allows developers to bind data, display content conditionally, and iterate over lists directly within the HTML markup.
- Custom Directives:
- Vue.js allows the creation of custom directives, enabling developers to extend the behavior of HTML elements. Custom directives can be used for tasks like manipulating the DOM or integrating with third-party libraries.
- Filters:
- Vue.js provides filters that can be used to format and manipulate data in the template. Filters are used within expressions to transform the output.
- Transitions and Animations:
- Vue.js facilitates the implementation of transitions and animations. It provides the
v-transition
andv-animation
directives to add effects to elements when they are inserted, updated, or removed.
- Vue.js facilitates the implementation of transitions and animations. It provides the
- Mixins:
- Vue.js supports mixins, allowing developers to encapsulate and reuse component options. Mixins are a way to divide reusable functionalities across components.
- Custom Events:
- Vue.js enables components to communicate with each other through custom events. Child components can emit events, and parent components can listen for and respond to these events.
What is the workflow of Vue.js?
The workflow of working with Vue.js involves several steps:
- Setup:
- Start by including the Vue.js library in your project. You can do this by linking to a CDN or by using a package manager like npm. Additionally, set up a project using Vue CLI if you prefer a more structured project setup.
- Create Components:
- Break down your user interface into components. Create Vue component files that include the template, script, and styles for each component. Components can be nested to create a hierarchical structure.
- Data Binding:
- Define a data model for your application. Use Vue directives like
{{ }}
for text interpolation,v-bind
for attribute binding, andv-model
for two-way data binding.
- Define a data model for your application. Use Vue directives like
- Vue Router (Optional):
- If your application involves multiple views or pages, set up Vue Router to handle client-side navigation. Define routes and link them to components.
- State Management (Optional):
- For complex applications with shared state, set up Vuex for centralized state management. Define actions, mutations, and getters to manage and modify the state.
- Computed Properties:
- Use computed properties to perform calculations based on reactive data. Computed properties are automatically updated when their dependencies change.
- Event Handling:
- Handle user interactions and events using the
v-on
directive. Bind methods to events and update the data model accordingly.
- Handle user interactions and events using the
- Lifecycle Hooks:
- Utilize lifecycle hooks to perform actions at different stages of a component’s lifecycle. For example, fetching data when a component is mounted.
- Testing:
- Write unit tests for your components and application logic. Tools like Jest and Vue Test Utils can be applied for testing Vue.js applications.
- Styling:
- Apply styles to your components using CSS or pre-processors like SCSS. Vue components can have scoped styles to prevent global style conflicts.
- Transitions and Animations:
- Implement transitions and animations using Vue.js directives and classes. Add visual effects to enhance the user experience.
- Build and Deployment:
- Use the Vue CLI to build your application for production. This process typically involves minification, bundling, and optimization of assets. Deploy the built files to a web server or a hosting platform.
- Continuous Integration (Optional):
- Set up continuous integration for your project using tools like Jenkins, Travis CI, or GitHub Actions. Automate the build and testing processes.
- Documentation:
- Document your Vue.js components, directives, and application structure. Tools like VuePress can be used to create documentation.
- Maintenance and Updates:
- Regularly update dependencies and address any issues that arise. Monitor the application’s performance and user feedback for continuous improvement.
Vue.js’s flexible and incremental adoption model makes it suitable for a variety of projects, from small prototypes to large-scale applications. The workflow outlined above provides a general guide for developing Vue.js applications.
How Vue.js Works & Architecture?
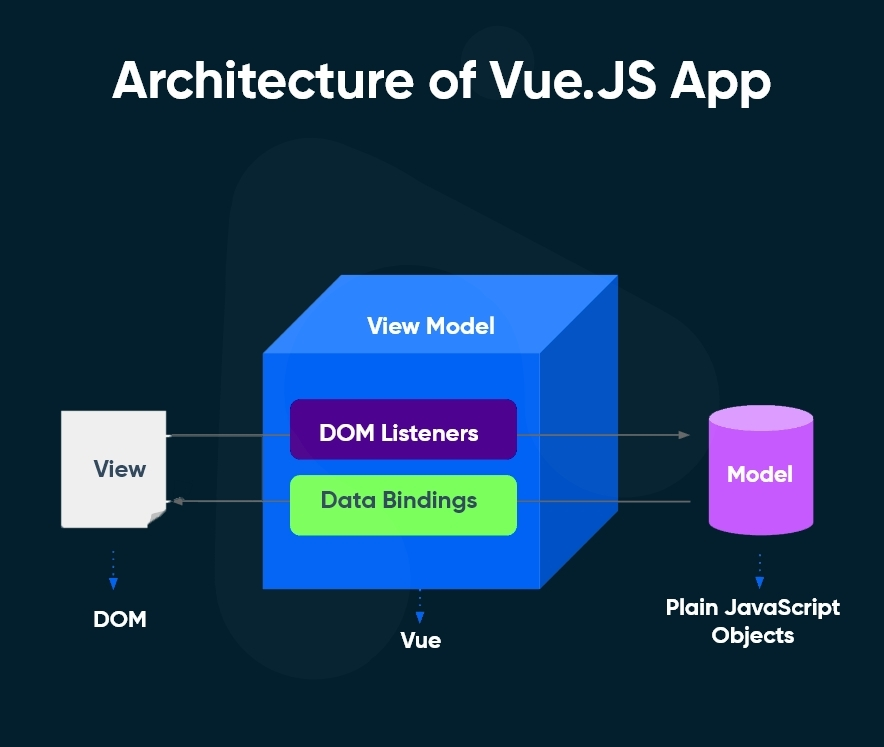
Vue.js is a famous JavaScript framework for building user interfaces. It’s known for its simplicity, flexibility, and powerful features. Here’s a breakdown of how it works and its architecture:
1. Core Principles:
- Declarative: Vue uses a template syntax to describe the UI, separating it from logic and making the code more readable.
- Component-based: The UI is built from reusable components, each with its own template, logic, and state. This promotes modularity and code reuse.
- Data-driven: The UI dynamically updates based on changes in the underlying data. This creates reactive and responsive interfaces.
- MVVM (Model-View-ViewModel): Vue follows the MVVM pattern, separating the data (Model), the UI (View), and the logic that binds them (ViewModel).
2. Key Components:
- Templates: Define the structure of the UI using HTML-like syntax with additional directives for binding data and behavior.
- Components: Reusable UI building blocks that encapsulate functionality and state. They can be nested and composed to create complex UIs.
- Data: Plain JavaScript objects that hold the state of the application. Changes in data automatically trigger updates to the UI.
- Directives: Special attributes added to HTML elements to bind data, control DOM manipulations, and implement functionality.
- Lifecycle Hooks: Functions that run at different stages of a component’s lifecycle, allowing for initialization, manipulation, and cleanup.
3. Architecture:
- Virtual DOM: Vue maintains an in-memory representation of the DOM called the virtual DOM. This enables efficient diffing and updates to the real DOM only when necessary, improving performance.
- Reactivity: Vue automatically tracks data dependencies and triggers UI updates whenever the data changes. This keeps the UI and data in sync seamlessly.
- Two-way data binding: Data can flow both ways between the UI and the data, making it easy to react to user interactions and update the state.
- Single File Components (SFCs): Vue components can be written in single files containing HTML, CSS, and JavaScript, promoting code organization and maintainability.
4. Benefits:
- Simple and easy to learn: Vue has a gentle learning curve, even for beginners.
- Flexible and customizable: Vue can be used to build various types of web applications, from simple single-page applications to complex web platforms.
- High performance: Vue’s virtual DOM and reactivity system enable efficient UI rendering and smooth performance.
- Large community and ecosystem: Vue has a vibrant community and a vast ecosystem of libraries and tools.
I hope this gives you a good understanding of how Vue.js works and its architecture. If you have any further questions about specific aspects of Vue.js, feel free to ask!
How to Install and Configure Vue.js?
There are several ways to achieve this, so let’s explore the most popular options:
1. Using a CDN (Easiest, but limited flexibility):
- Add the following script to your HTML file:
<script src="https://unpkg.com/vue@3/dist/vue.global.js"></script>
- Now, you can write your Vue.js code directly in your HTML files.
2. Using a package manager (Recommended for larger projects):
- Install the Vue.js package using your preferred package manager (npm or yarn):
Bash
npm install vue
# or
yarn add vue
- In your JavaScript files, import Vue:
import Vue from 'vue';
new Vue({
// Your Vue application setup
});
3. Using the Vue CLI (Best for project scaffolding and dependency management):
- Install the Vue CLI:
Bash
npm install -g @vue/cli
# or
yarn global add @vue/cli
- Create a new project:
Bash
vue create my-app
This sets up a basic project structure with dependencies and configuration files.
4. Configuration (Optional, but recommended):
- Extend Vue’s functionality with plugins like Vue Router or Vuex.
- Customize compilation options.
- Use tools like Webpack or Vite for production bundling.
Important Tips:
- Choose the method that best suits your needs and project size.
- Don’t hesitate to consult the resources for detailed instructions and configuration options.
- Feel free to ask specific questions if you encounter any difficulties!
I hope this clarifies the options and provides a better starting point for your Vue.js journey. Good luck!
Fundamental Tutorials of Vue.Js: Getting started Step by Step
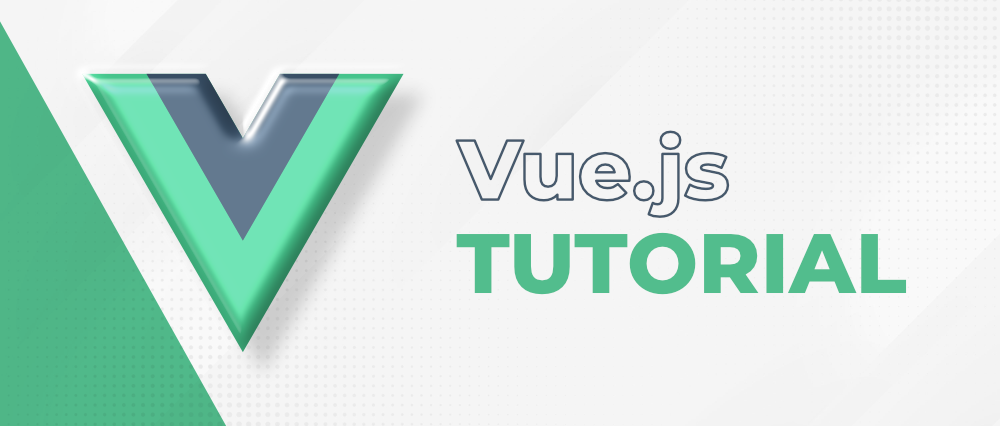
Let’s have a look at more detailed breakdown of stepwise fundamental tutorials for Vue.js, focusing on building a simple counter app:
1. Setting Up:
- Choose your preferred method:
- CDN: Add the Vue CDN script to your HTML file (
https://unpkg.com/vue@3/dist/vue.global.js
). - Package Manager: Install Vue (
npm install vue
oryarn add vue
). Import Vue in your JavaScript file (import Vue from 'vue'
).
- CDN: Add the Vue CDN script to your HTML file (
- Create an HTML file with a basic structure and a root element (e.g.,
div
) with an ID (#app
).
2. Data Definition:
- In your Vue instance, define an initial data object with a
count
property set to 0.
JavaScript
new Vue({
el: '#app',
data: {
count: 0,
},
});
3. Template and Rendering:
- In your HTML, create a section to display the counter value:
<div id="app">
<h1>Count: {{ count }}</h1>
</div>
- Use mustache syntax (
{{ count }}
) to bind the data property to the displayed text.
4. Incrementing the Counter:
- Create a button element with a click event bound to a method in your Vue instance:
<div id="app">
<h1>Count: {{ count }}</h1>
<button @click="incrementCount">Increment</button>
</div>
- Define the
incrementCount
method in your Vue instance:
JavaScript
methods: {
incrementCount() {
this.count++; // Increase the count by 1
},
},
- This method accesses the
count
data property usingthis
and increments its value.
5. Updating the UI:
- When the button is clicked, the
incrementCount
method runs, updating thecount
data. - Vue automatically detects the data change and re-renders the template, reflecting the updated counter value.
6. Enhancing the App (Optional):
- Add a button to decrease the counter value with a corresponding method.
- Integrate styles using CSS to customize the app’s appearance.
- Explore additional features like conditional rendering and event modifiers for richer interactions.
Bonus tips :
- Use the Vue Devtools browser extension to inspect the Vue component hierarchy and data in real-time for deeper understanding.
Points to Remember:
- These are just basic steps. As you learn more, explore advanced features and build more complex apps.
- Don’t hesitate to ask questions and consult resources like the official Vue.js documentation and tutorials for further guidance.
Have fun exploring the exciting world of Vue.js!
- PPG Industries: Selection and Interview process, Questions/Answers - April 3, 2024
- Fiserv: Selection and Interview process, Questions/Answers - April 3, 2024
- Estee Lauder: Selection and Interview process, Questions/Answers - April 3, 2024