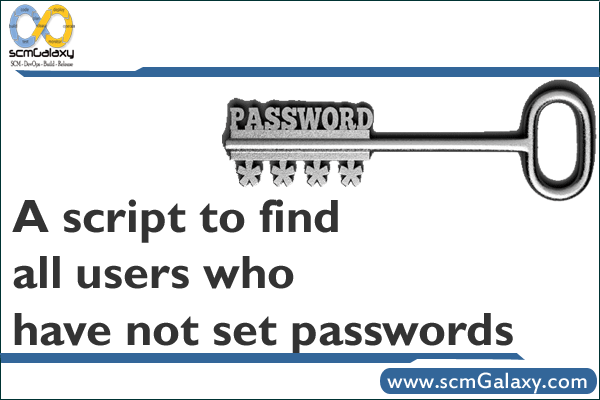
Write a script to find all users who have not set passwords.
#!/usr/bin/ruby
require “P4”
p4 = P4.new
p4.parse_forms
p4.connect
p4.run_users.each do
|u|
user = p4.fetch_user( u[ “User” ] )
puts( user[ “User” ] ) unless user.has_key?( “Password” )
end
#!/
OR
#!/usr/bin/perl
use P4;
my $p4 = new P4;
$p4->ParseForms();
$p4->Init() or die( “Can’t connect to Perforce” );
foreach my $u ( $p4->Users() )
{
my $user = $p4->FetchUser( $u->{ “User” } );
print( $user->{ “User” }, “\n” ) unless defined ( $user->{ “Password” }
);
}
Latest posts by Rajesh Kumar (see all)
- How to remove sensitive warning from ms office powerpoint - July 14, 2024
- AIOps and DevOps: A Powerful Duo for Modern IT Operations - July 14, 2024
- Leveraging DevOps and AI Together: Benefits and Synergies - July 14, 2024