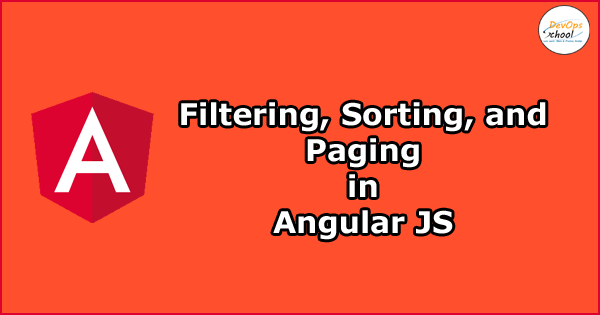
First, we’ll discuss what is AnglularJS and their Benefits.
What is Angular JS
Angular JS is a Javascript Framework that helps build web applications. It is used in Single Page Application (SPA) projects. It extends HTML DOM with additional attributes and makes it more responsive to user actions. AngularJS is open source, completely free, and used by thousands of developers around the world. It is licensed under the Apache license version 2.0.
Benefits of AngularJS
- Dependency Injection
- Two-way Data-Binding
- Testing
- Model View Controller
- Directives, filters etc…
Now, Get started: Step 1
As a first step, we will set up a new Angular JS application called ‘GridApp’ with a basic controller called ‘GridController’ which we will be using throughout this tutorial. I’ve included the following files in the index.html.
- Anglular.min.js
- App.js (Our app initialization file)
- Grid.js (This will be our controller file)
- Bootstrap.min.css
- Main.css (For any custom styles)
Here the folder structure:
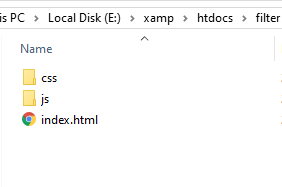
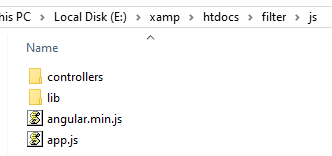
Our index.html should look similar to the code below.
Our app.js file will only contain app initialization code:
Step 2: Displaying data
Now that we have set up our application, let’s move to add and display some data. we will be using random JSON data that I’ll be adding to the ‘grid.js’ file. Alternatively, you can also use an Ajax request (using $http) to fetch the data to display.
Next, we will use the ng-repeat directive to display this data in a table format.
Step 3: Filtering data
Filtering data in Angular JS is also easy. Angular JS comes with a number of amazing filters that you can use to transform data.
Before we add any filtering code though, let’s first add an input search field in our index.html file. Which we’ll be binding to our controller data using the ng-model directive. And add your “grid” div the following code:
We used the ng-repeat directive to iterate through our data and display it. In this case, we will apply our filter to sort data.
ng-repeat=”person in people | filter: queryText”
What we have done this code, we have applied the data filter. Filter in AnglularJS can be applied by using the | (pipe) character followed by the filter name.
The filter used in this case, searches through our data and returns matched items. In AnglularJS data-binding is that whenever you type anything in the search field, the view will automatically be updated with the new results.
Step 4: Sorting data
Now, let’s data sorting in Angular JS. Angular JS also offers a pre-defined filter that can help you order and sort data easily.
These filters take two arguments:
- Order key
- Reverse sort (can be either true or false; true in case of descending order)
We can use this sorting filter in Angular JS. Before we apply this filter though, let’s add some basic sort functionality in our controller ‘grid.js’.
Now we have added default sorting configuration and function that takes a key as an argument. This function also toggles the sorting order. To apply the orderByfilter and update our table heading markup to call this function upon ng-click.
I have also added glyphicon to indicate the current sort order. You can see sorting results, just run the application in a browser.
Step 5: Paging
You need to add the dirPaginate library to our project. You can install it with Bower, or npm, or just download it manually from this link.
Bower: bower install angular-utils-pagination
npm: npm install angular-utils-pagination
Next, include the link to the dirPagination.js file to your index.html file. To add the ‘angularUtils.directives.dirPagination’ module in the controller file.
You can also add a scope variable for the number of items you want to display per page.
scope.itemsPerPage = 5;
To use of dirPaginate, you have to replace your ng-repeat directive with the dir-paginate directive.
To add here is a dirPaginate filter, which will restrict the items shown per page.
The itemsPerPage should be applied after all other filters.
And, then add the following code right after your grid.
This will automatically display/hide the pagination links based on your data.
The final code of our index.html are:
And final code of grid.js
The final results, you can see look like:
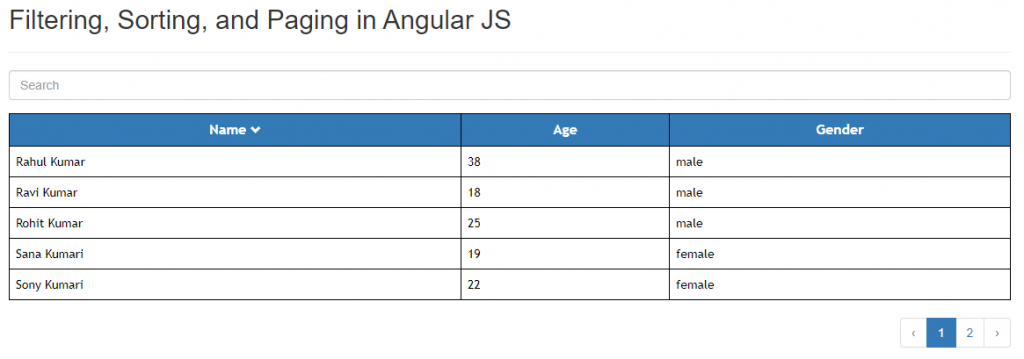
- Top 5 Reliable Phone Number Validation API for Your Next Product - February 10, 2022
- Top 5 IT Management Tools | IT Management Software - July 30, 2021
- Complete guide of PHP certification courses, tutorials & training - July 23, 2021