Welcome to the Plugin Development Series. I hope this Series Will Help You Write a good plugin.
But First You need to understand Why we make Plugins?
There is a rule in WordPress Development that: DO NOT TOUCH WORDPRESS CORE, This means that you do not edit the core WordPress files or functions that helps WordPress keep running. And also it overwrites each function with every update, Any new function or changes you want to make can be only done through plugins. WordPress plugins can be a function (a single PHP file) or an entire program to add something or modify in main word press functionality. Plugins allow you to greatly extend the functionality of WordPress without touching the WordPress core itself.
What Is A Plugin?
Plugins are packages of code that extend the core functionality of WordPress
WordPress plugins are made up of PHP code and can include other assets such as images, CSS, and JavaScript. By making your own plugin you are extending WordPress, i.e. building additional functionality on top of what WordPress already offers.
Basics Of Plugin.
At its simplest, a WordPress plugin is a PHP file with a WordPress plugin header comment. It’s highly recommended that you create a directory to hold your plugin so that all of your plugin’s files are neatly organized in one place.
To get started creating a new plugin, follow the steps below.
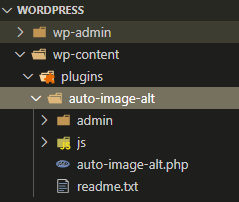
- Navigate to the WordPress installation’s wp-content directory.
- Open the plugins directory.
- Create a new directory and name it after the plugin (e.g.
plugin-name
). - Open the new plugin’s directory.
- Create a new PHP file (it’s also good to name this file after your plugin, e.g.
plugin-name.php
).
Now that you’re editing your new plugin’s PHP file, you’ll need to add a plugin header comment. This is a specially formatted PHP block comment that contains metadata about the plugin, such as its name, author, version, license, etc. The plugin header comment must comply with the header requirements, and at the very least, contain the name of the plugin
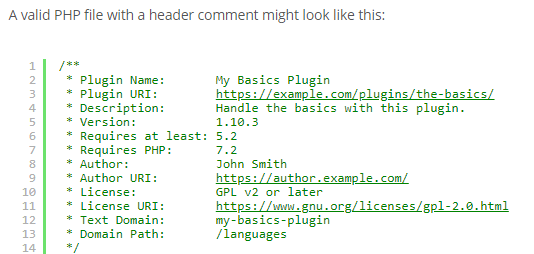
That’s all you need, Your basic plugin setup is ready now go to the plugin option in your WordPress admin panel and activate your plugin. It won’t change anything as you have nothing written on it.
What are Hooks In WordPress Plugin?
WordPress hook is a feature that allows you to manipulate a procedure without modifying the file on WordPress core. A hook can be applied both to action (action hook) and filter (filter hook)
Purpose of Hooks
The primary purpose of hooks is to automatically run a function. In addition, this technique also has the ability to modify, extend, or limit the functionality of a theme or plugin.
Here is an example of a hook in WordPress:
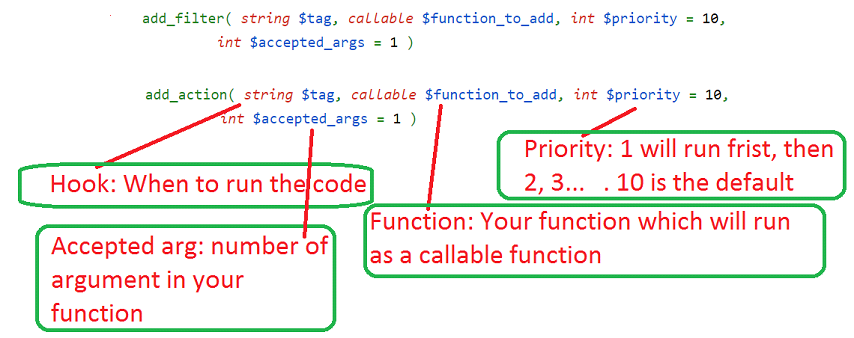
How to Use WordPress Hooks?
Using hooks in WordPress does require a bit of knowledge about HTML and PHP. However, even if you are a complete beginner, creating both action and filter hooks might not be as difficult as you think.
You only need to go to your post page, then switch to the text editor. When you are there, you can paste the hooks that you have copied from other sites or created yourself.
Creating an Action Hook
To add an action hook, you must activate the add_action () function in the WordPress plugin. This function can be activated by writing the patterns below in your functions.php file
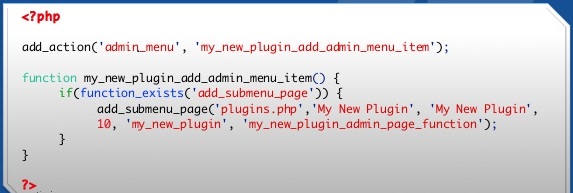
Creating an FilterHook
You can create a filter hook by utilizing apply_filters() function. The hook filter is used to modify, filter, or replace a value with a new one.
Just like using an action hook, it also has a function that filters a value with the associated filter hook functions (apply_filter).
What is more, it has the function to add a hook filter to be associated with another function (add_filter).
Here is an example of a filter hook:
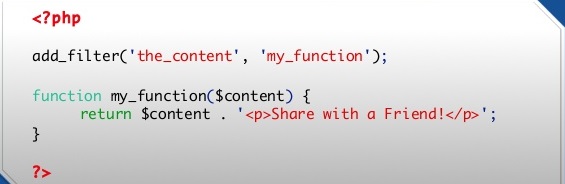
Unhooking Actions and Filters
If you want to disable the command from add_action() or add_filter() in your WordPress code, you can use remove_action() and remove_filter().
These codes are basically a way to exclude certain actions or filter functions. It allows you to modify a plugin that has too many unnecessary hooks which might disrupt your site’s optimization.
Right now, you might ask this; “why not just delete these unnecessary codes?”
Well, it certainly is a viable option if you use your own codes.
However, in WordPress, you often work with someone else’s plugins or themes. It means that you risk making a fatal error if you delete the incorrect lines.
- Laravel – select dropdowns that filter results in a table ‘on change’ - October 30, 2021
- What is Rest and Restful API? Understand the Concept - September 5, 2020
- What is API, clear the Concept. - September 5, 2020