JWT (JSON WEB TOKEN)
JSON Web Token (JWT) is an open standard that allows two parties to securely send data and information as JSON objects. This information can be verified and trusted because it is digitally signed. It is a Token format standardized by the IETF organization.
JWT authentication has added the wider adoption of stateless API services. It makes it convenient to authorise and verify clients accessing API resources. It is a critical part of the authentication system in javascript powered applications.
To Install JWT in your Laravel Project, Just Follow the Below Steps :-
Step 1. Install via composer
Run the following command to pull in the latest version:
composer require tymon/jwt-auth
Step 2. Add service provider ( Laravel 5.4 or below )
Add the service provider to the providers
array in the config/app.php
config file as follows:
Step 3. Publish the config
Run the following command to publish the package config file:
php artisan vendor:publish --provider="Tymon\JWTAuth\Providers\LaravelServiceProvider"
You should now have a config/jwt.php
file that allows you to configure the basics of this package.
Step 4. Generate secret key
I have included a helper command to generate a key for you:
php artisan jwt:secret
This will update your .env
file with something like JWT_SECRET=foobar
It is the key that will be used to sign your tokens. How that happens exactly will depend on the algorithm that you choose to use.
Step 5. Bootstrap file changes.
Add the following snippet to the bootstrap/app.php
file under the providers section as follows:
// Add this line
$app->register(App\Providers\AuthServiceProvider::class);
Step 6. Re-Generate secret key
I have included a helper command to generate a key for you:
php artisan jwt:secret
This will update your .env
file with something like JWT_SECRET=foobar
It is the key that will be used to sign your tokens. How that happens exactly will depend on the algorithm that you choose to use.
Step 7. Update your User model
Firstly you need to implement the Tymon\JWTAuth\Contracts\JWTSubject
contract on your User model, which requires that you implement the 2 methods getJWTIdentifier()
and getJWTCustomClaims()
.
The example below should give you an idea of how this could look. Obviously you should make any changes, as necessary, to suit your own needs.
Step 8. Configure Auth guard
Note: This will only work if you are using Laravel 5.2 and above.
Inside the config/auth.php
file you will need to make a few changes to configure Laravel to use the jwt
guard to power your application authentication.
Make the following changes to the file:
Here we are telling the api
guard to use the jwt
driver, and we are setting the api
guard as the default.
We can now use Laravel’s built in Auth system, with jwt-auth doing the work behind the scenes!
Step 9. Add some basic authentication routes
First let’s add some routes in routes/api.php
as follows:
Step 10. Create the AuthController
Then create the AuthController
, either manually or by running the artisan command:
php artisan make:controller AuthController
Then add the following:
All Done.
Now you can test API Login/Register with Postman.
Keep Coding 😀😀😀

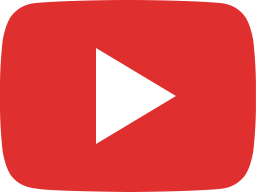

- What is On-Page Optimization and Off-page Optimization - March 14, 2024
- [SOLVED] Flutter : PlatformException(sign_in_failed, com.google.android.gms.common.api.ApiException: 10: , null, null) - December 7, 2021
- [Solved] Flutter : Error: The getter ‘subhead’ isn’t defined for the class ‘TextTheme’ from package:flutter/src/material/text_theme.dart’ – searchable_dropdown - December 6, 2021