Here we are going to discuss about the PHP Date and times. how can we call current date and time in our code. There are nearly fifty date and time functions, so for this tutorial we will narrow them down to some of them which are very important to us.
Function | Description |
date() | Formats a Local Time/Date |
time() | Returns the Current Time as a Unix Timestamp |
strtotime() | Parses an English Textual Date or Time Into a Unix Timestamp |
localtime() | Get the local time |
datesunrise() | Returns time of sunrise for a given day and location |
date_sunset() | Returns time of sunset for a given day and location |
PHP Date() Function
The date() function formats a timestamp so that it actually makes sense, such as 4:58 PM Thursday, June 18, 2020. The date() function accepts two arguments, according to the following syntax: date(format, timestamp).
Example Code :
Syntax
date(format, timestamp)
PHP provides over thirty-five case-sensitive characters that are used to format the date and time. These characters are:
Character | Description | Example | |
---|---|---|---|
Day | j | Day of the Month, No Leading Zeros | 1 – 31 |
Day | d | Day of the Month, 2 Digits, Leading Zeros | 01 – 31 |
Day | D | Day of the Week, First 3 Letters | Mon – Sun |
Day | l (lowercase ‘L’) | Day of the Week | Sunday – Saturday |
Day | N | Numeric Day of the Week | 1 (Monday) – 7 (Sunday) |
Day | w | Numeric Day of the Week | 0 (Sunday) – 6 (Saturday) |
Day | S | English Suffix For Day of the Month | st, nd, rd or th |
Day | z | Day of the Year | 0 – 365 |
Week | W | Numeric Week of the Year (Weeks Start on Mon.) | 1 – 52 |
Month | M | Textual Representation of a Month, Three Letters | Jan – Dec |
Month | F | Full Textual Representation of a Month | January – December |
Month | m | Numeric Month, With Leading Zeros | 01 – 12 |
Month | n | Numeric Month, Without Leading Zeros | 1 – 12 |
Month | t | Number of Days in the Given Month | 28 – 31 |
Year | L | Whether It’s a Leap Year | Leap Year: 1, Otherwise: 0 |
Year | Y | Numeric Representation of a Year, 4 Digits | 1999, 2003, etc. |
Year | y | 2 Digit Representation of a Year | 99, 03, etc. |
Time | a | Lowercase Ante Meridiem & Post Meridiem | am or pm |
Time | A | Uppercase Ante Meridiem & Post Meridiem | AM or PM |
Time | B | Swatch Internet Time | 000 – 999 |
Time | g | 12-Hour Format Without Leading Zeros | 1 – 12 |
Time | G | 24-Hour Format Without Leading Zeros | 0 – 23 |
Time | h | 12-Hour Format With Leading Zeros | 01 – 12 |
Time | H | 24-Hour Format With Leading Zeros | 00 – 23 |
Time | i | Minutes With Leading Zeros | 00 – 59 |
Time | s | Seconds With Leading Zeros | 00 – 59 |
Timezone | e | Timezone Identifier | Example: UTC, Atlantic |
Timezone | I (capital i) | Whether Date Is In Daylight Saving Time | 1 if DST, otherwise 0 |
Timezone | O | Difference to Greenwich Time In Hours | Example: +0200 |
Timezone | P | Difference to Greenwich Time, With Colon | Example: +02:00 |
Timezone | T | Timezone Abbreviation | Examples: EST, MDT … |
Timezone | Z | Timezone Offset In Seconds | -43200 through 50400 |
Using a combination of these characters and commas, periods, dashes, semicolons and backslashes, you can now format dates and times at which format you want.
PHP Time() Function
The time() function returns the current timestamp. The time() function can also return a modified timestamp. You can add or subtract any number of seconds to the function in order to return the timestamp of a previous or upcoming date and time.
Syntax
time()
For example, to return a timestamp for next week, or to return a timestamp from last week, I can add or subtract 7 days by determining how many seconds are involved (7 days * 24 hours per day * 60 minutes in an hour * 60 seconds in an hour = number of second in 7 days).
The result of above code will look like this:
Last Week: 1591871848
Next Week: 1593081448
Next Month: 1595068648
PHP Strtotime() Function
The strtotime() function accepts an English datetime description and turns it into a timestamp. It is a simple way to determine “next week” or “last monday” without using the time() function and a bunch of math.
Syntax
strtotime(time, now);
Some examples are:
PHP localtime() function
The localtime() function returns an array that contains the time components of a Unix timestamp.
Syntax
localtime(timestamp, is_assoc)
Example Code :
The result will be look like this:
Array ( [0] => 50 [1] => 2 [2] => 12 [3] => 18 [4] => 5 [5] => 120 [6] => 4 [7] => 169 [8] => 0 )
Array ( [tm_sec] => 50 [tm_min] => 2 [tm_hour] => 12 [tm_mday] => 18
[tm_mon] => 5 [tm_year] => 120 [tm_wday] => 4 [tm_yday] => 169 [tm_isdst] => 0 )
PHP datesunrise() Function
The date_sunrise() function returns the time of sunrise for a given day / location.
Note : It returns the time of the sunrise, in the specified format, on success. FALSE on failure.
Syntax
date_sunrise(timestamp, format, latitude, longitude, zenith, gmtoffset)
Example Code :
the result will look like this:
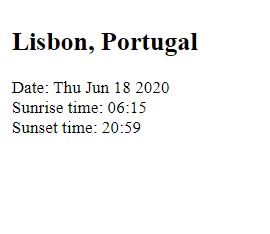
PHP date_sunset() Function
The date_sunset() function returns the time of sunset for a given day / location.
Syntax
date_sunset(timestamp, format, latitude, longitude, zenith, gmtoffset)
Example Code :
The Result will look like this:
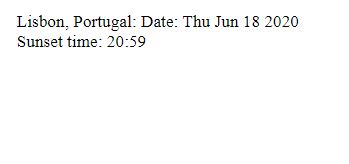

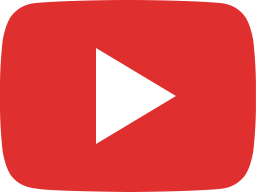
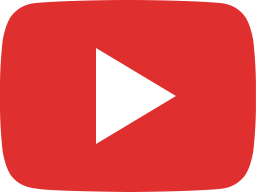

- Top 10 DevOps Blogs and Websites To Follow in 2023 - December 13, 2022
- How To Set Up Apache Virtual Hosts on Ubuntu 20.04.2 LTS - October 28, 2021
- How to Fix ” Vue packages version mismatch:” error in Laravel 5.5 - April 15, 2021