- What is C#?
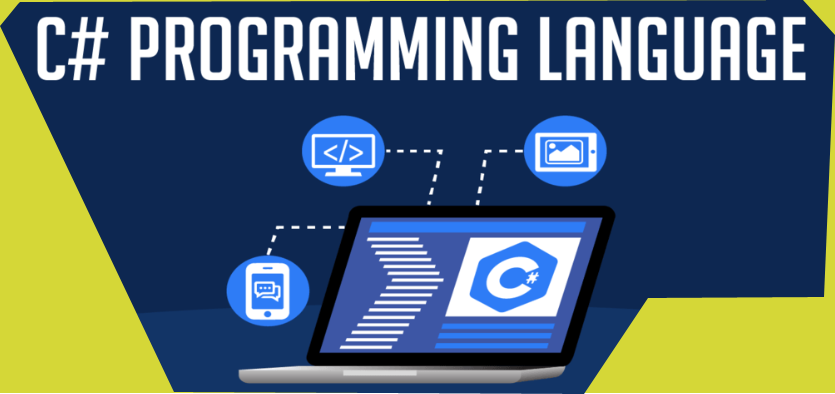
C# is an object-oriented, modern programming language that was created by Microsoft. It runs on the .NET Framework. C# is very close to C/C++ and Java programming languages. The language is proposed to be a simple, modern, general-purpose, object-oriented programming language. The language is used for creating software components.
2. What is Common Language Runtime (CLR)?
CLR is the basic and Virtual Machine component of the .NET Framework. It is the run-time environment in the .NET Framework that runs the codes and helps in making the development process easier by providing various services such as remoting, thread management, type-safety, memory management, robustness, etc. Basically, it is responsible for managing the execution of .NET programs regardless of any .NET programming language. It also helps in the management of code, as code that targets the runtime is known as the Managed Code, and code that doesn’t target to runtime is known as Unmanaged code.
3. What is the JIT compiler process?
Just-In-Time compiler(JIT) is a part of Common Language Runtime (CLR) in .NET which is responsible for managing the execution of .NET programs regardless of any .NET programming language. A language-specific compiler converts the source code to the intermediate language. This intermediate language is then converted into the machine code by the Just-In-Time (JIT) compiler. This machine code is specific to the computer environment that the JIT compiler runs on.
4. What are indexers in C# .NET?

Indexers are known as smart arrays in C#. It allows the instances of a class to be indexed in the same way as an array.
5. What is garbage collection in C#?
Automatic memory management is made possible by Garbage Collection in .NET Framework. When a class object is created at runtime, certain memory space is allocated to it in the heap memory. However, after all the actions related to the object are completed in the program, the memory space allocated to it is a waste as it cannot be used. In this case, garbage collection is very useful as it automatically releases the memory space after it is no longer required.
Garbage collection will always work on Managed Heap, and internally it has an Engine which is known as the Optimization Engine. Garbage Collection occurs if at least one of multiple conditions is satisfied. These conditions are given as follows:
- If the system has low physical memory, then garbage collection is necessary.
- If the system has low physical memory, then garbage collection is necessary.
- If the memory allocated to various objects in the heap memory exceeds a pre-set threshold, then garbage collection occurs.
- If the GC.Collect method is called, then garbage collection occurs. However, this method is only called under unusual situations as normally garbage collector runs automatically.
6. What are the types of classes in C#?

- Abstract class
- Partial class
- Sealed class
- Static class
7. What is the difference between C# abstract class and an interface?
Abstract Class | Interface |
It contains both declaration and definition parts. | It contains only a declaration part. |
Multiple inheritance is not achieved by an abstract class. | Multiple inheritance is achieved by the interface. |
It contains a constructor. | It does not contain a constructor. |
It can contain static members. | It does not contain static members |
It can contain different types of access modifiers like public, private, protected, etc. | It only contains public access modifier because everything in the interface is public. |
The performance of an abstract class is fast. | The performance of the interface is slow because it requires time to search the actual method in the corresponding class. |
It is used to implement the core identity of class. | It is used to implement the peripheral abilities of the class. |
A class can only use one abstract class. | A class can use multiple interfaces. |
If many implementations are of the same kind and use common behavior, then it is superior to use an abstract class. | If many implementations only share methods, then it is superior to use Interface. |
An abstract class can contain methods, fields, constants, etc. | The interface can only contain methods. |
It can be fully, partially, interface or not implemented. | It should be fully implemented. |
8. What is inheritance? Does C# support multiple inheritance?
Inheritance is an important pillar of OOP(Object Oriented Programming). It is the mechanism in C# by which one class is allowed to inherit the features(fields and methods) of another class.
Super Class: The class whose features are inherited is known as superclass(or a base class or a parent class).
Sub Class: The class that inherits the other class is known as a subclass(or a derived class, extended class, or child class). The subclass can add its own fields and methods in addition to the superclass fields and methods.
Reusability: Inheritance supports the concept of “reusability”, i.e. when we want to create a new class and there is already a class that includes some of the code that we want, we can derive our new class from the existing class. By doing this, we are reusing the fields and methods of the existing class.
9. What are extension methods in C#?
In C#, the extension method concept allows you to add new methods in the existing class or in the structure without modifying the source code of the original type, and you do not require any kind of special permission from the original type and there is no need to re-compile the original type. It is introduced in C# 3.0.
10. What is Boxing and Unboxing in C#?
Boxing and unboxing is an important concept in C#. C# Type System contains three data types: Value Types (int, char, etc), Reference Types (object), and Pointer Types. Basically, it converts a Value Type to a Reference Type, and vice versa. Boxing and Unboxing enable a unified view of the type system in which a value of any type can be treated as an object.
Boxing In C#
- The process of Converting a Value Type (char, int, etc.) to a Reference Type(object) is called Boxing.
Boxing is an implicit conversion process in which object type (supertype) is - used.
The Value type is always stored in Stack. The Referenced Type is stored in Heap.
Unboxing In C#
- The process of converting the reference type into the value type is known as Unboxing.
- It is an explicit conversion process.
11. What is the difference between ref and out keywords?
The ref is a keyword in C# which is used for passing the arguments by a reference. Or we can say that if any changes made in this argument in the method will reflect in that variable when the control return to the calling method. The ref parameter does not pass the property.
The out is a keyword in C# which is used for passing the arguments to methods as a reference type. It is generally used when a method returns multiple values. The out parameter does not pass the property.
12. What is the difference between a struct and a class in C#?
A class is a user-defined blueprint or prototype from which objects are created. Basically, a class combines the fields and methods(member function which defines actions) into a single unit.
A structure is a collection of variables of different data types under a single unit. It is almost similar to a class because both are user-defined data types and both hold a bunch of different data types.
13. What is enum in C#?
Enumeration (or enum) is a value data type in C#. It is mainly used to assign the names or string values to integral constants, which make a program easy to read and maintain. For example, the 4 suits in a deck of playing cards may be 4 enumerators named Club, Diamond, Heart, and Spade, belonging to an enumerated type named Suit. Other examples include natural enumerated types (like the planets, days of the week, colors, directions, etc.). The main objective of enum is to define our own data types(Enumerated Data Types). Enumeration is declared using the enum keyword directly inside a namespace, class, or structure.
14. What are partial classes in C#?
A partial class is a special feature of C#. It provides a special ability to implement the functionality of a single class into multiple files and all these files are combined into a single class file when the application is compiled. A partial class is created by using a partial keyword. This keyword is also useful to split the functionality of methods, interfaces, or structure into multiple files.
________________________________________
public partial Class_name
{
// Code
}
________________________________
15. What is Reflection in C#?
Reflection is the process of describing the metadata of types, methods, and fields in a code. The namespace System. Reflection enables you to obtain data about the loaded assemblies, the elements within them like classes, methods, and value types.
16. What is the difference between constant and read-only in C#?
In C#, a const keyword is used to declare constant fields and constant local. The value of the constant field is the same throughout the program or in other words, once the constant field is assigned the value of this field is not be changed. In C#, constant fields and locals are not variables, a constant is a number, string, null reference, boolean values. readonly keyword is used to declare a readonly variable. This readonly keyword shows that you can assign the variable only when you declare a variable or in a constructor of the same class in which it is declared.
17. What is Jagged Arrays?
A jagged array is an array of arrays such that member arrays can be of different sizes. In other words, the length of each array index can differ. The elements of Jagged Array are reference types and initialized to null by default. Jagged Array can also be mixed with multidimensional arrays. Here, the number of rows will be fixed at the declaration time, but you can vary the number of columns.
18. What’s the difference between the System.Array.CopyTo() and System.Array.Clone() ?
The System.Array.CopyTo() technique makes a replica of the components into another existing array. It makes copies the components of one cluster to another existing array. The Clone() technique returns a new array object containing every one of the components in the first array. The Clone() makes a duplicate of an array as an object, consequently should be cast to the real exhibit type before it tends to be utilized to do definitely. The clone is of a similar type as the first Array.
19. What is the difference between to dispose and finalize methods in C#?
The primary difference between dispose() and finalize() is that the dispose() must be explicitly invoked by the user and the finalize() is called by the garbage collector when the object is destroyed.
20. What are delegates in C#?
A delegate is an object which refers to a method, or you can say it is a reference type variable that can hold a reference to the methods. Delegates in C# are similar to the function pointer in C/C++. It provides a way that tells which method is to be called when an event is triggered. For example, if you click a button on a form (Windows Form application), the program would call a specific method. In simple words, it is a type that represents references to methods with a particular parameter list and return type and then calls the method in a program for execution when it is needed.
21. How will you differentiate readonly, const and static variables in c#?
- const keyword allows you to declare a constant field or a constant local which you expect not to modify at any time in the code except at the declaration time you need to assign a value. .
- readonly keyword allows you to declare a field whose values can be assigned or modified at the declaration or in constructor only.
- static keywords allows you to declare the static member which belongs to the type itself instead of object. Fields, Properties, methods constructors, operators, local functions from C# 8 and lambda expressions or anonymous methods from C# 9 onwards can be marked as static inside classes, interfaces or structs.
22. What are the three characteristics of Object Oriented Programming?
There are three main characteristics of Object Oriented Programming.
- Encapsulation is considered as the first principle of OOPs. As per this principle, A Class or Struct can specify the accessibility of its members to code outside of this class. Members not intended to be accessed outside of this class or struct can be hidden.
- Inheritance is one of the three characteristics of OOPs. It allows you to create new classes that can re-use, modify and extend the behavior defined in other classes. In Inheritance, the class which inherits or re-use the other class members is known as derived class and the class whose members are inherited is known as Base class.
- C# does not allow multiple inheritance – means a derived class can have only one direct base class. Inheritance is transitive in nature means If class X inherits from class Y and class Y inherits from class Z then class X inherits from both Y and Z.
- Polymorphism – It is referred to as the third pillar of Object oriented programming. Polymorphism means “Many shapes” – more than one form of an object. It allows you to implement inherited properties and methods in different ways across the abstractions. C# implement polymorphism at compile time and run time.
23. What is the difference between in, ref and out keywords?
- In keyword is like ref or out keywords but ‘in’ arguments can not be modified by the called method. ‘in’ arguments must be initialized before passing to the called method. If you try to modify the value of ‘in’ argument in the calling method it will throw an error. So it’s like a readonly variable.
- ref keyword causes an argument to be passed by reference and may be read or written by the called method. To pass an argument as reference means any change to the parameter in the called method is reflected in the calling method argument. ref argument may be modified by the called method.
- out keyword causes arguments to be passed by reference and is written by a called method. It is similar to ref keyword, except that ref requires that the variable must be initialized before it is passed. To pass variables as out arguments, both the method definition and the calling method must explicitly use the out keyword.
24. What is method shadowing or method hiding in C#?
Shadowing is also known as method Hiding in C#. Shadowing is a VB.Net concept. It hides the base class method implementation. It can be achieved using the ‘new’ keyword. If a method is not overriding the derived method that means it’s hiding it.
Override keyword is used to provide a completely new implementation of a base class method or extend base class method (which is marked ‘Virtual’) in the derived class. While the New keyword just hides the base class method, not extends it.
If you think you need a different implementation of the same function as provided by base class, then you can hide the base class function implementation in child class.
___________________________________________________
class BC
{
public void Display()
{
System.Console.WriteLine("BC::Display");
}
}
class DC : BC
{
public void Display()
{
System.Console.WriteLine("DC::Display");
}
}
class Demo
{
public static void Main()
{
DC obj = new DC();
obj.Display();
}
}
Output : DC:Display
___________________________________
25. Can Virtual or Abstract members be declared private in C#?
No, It will throw a compile time error.
__________________________________________________________
virtual void display() // will throw error here
{
// Implementation
}
_____________________________________________________
26. What is Method Overloading in C#?
Method Overloading is a way to implement polymorphism – you are going to have more than one form of a method. You can implement method overloading by defining two or more methods in a class with the same name but different method signatures. Method Signature includes number, sequence and type of parameters not the return type of method. Method overloading example:
_____________________________________
void check(int x)
{
// Implementation
}
void check(float x)
{
// Implementation
}
void check(int x, int y)
{
// Implementation
}
_________________________________________
In the above example method name is the same ‘check’ in all three cases but method signature is different.
27. How will you differentiate Abstraction and Encapsulation in C#?
- Abstraction is to create the prototype of relevant attributes and interaction of an entity in the form of classes. This Prototype defines an abstraction representation of a concept. For example, A BankAccount base class creates an abstraction or defines the behavior for the Bank Account concept. Now you can create any type of bank account like saving, just by deriving your new class ‘SavingAccount’ from this ‘BankAccount’ base class.
- Encapsulation is the hiding of the functionality and internal state of an object from the code outside of this class and allows access through a public set of properties or functions. For Example, the BankAccount class can contain some private members to hide the data from the code outside of this ‘BankAccount’ class.
- Abstraction can be implemented using Abstract classes and Interface whereas Encapsulation can be implemented using access modifiers such as public, private, protected etc.
- Abstraction helps to solve the problems at design level using Abstract classes or Interfaces while encapsulation helps to solve problems at implementation level using access modifiers.
28. What is Polymorphism? Explain the static and Dynamic Polymorphism in C#?
Object-oriented programming has mainly three pillars, Polymorphism is one of them after encapsulation and inheritance. It has special meaning – “many shapes” and has two aspects:
At run-time, derived class objects may be considered as base class objects in places such as method parameters and collections or arrays. When this polymorphism happens, declared and run-time types of object are different. For example,
___________________________________
public class Shape
{
}
public class Circle : Shape
{
}
public class Rectangle : Shape
{
}
var shapes = new List
{
new Rectangle(), new Triangle(), new Circle()
};
_________________________________
Base classes may have virtual members which can be overridden by the derived class with their own definition and implementation. At the time of execution, When client code calls the method, the override method is executed because CLR looks up the run-time type of object rather than of the compile-time object. In your code you can cause a derived class method to be executed by calling a method of base class as below.
________________________________________________
A o1 = new A();
o1.disp();
B o2 = new B();
o2.disp();
A o3 = new B(); // here
o3.disp(); // derived class method will be executed by calling base class method
____________________________________
MSDN does not provide any information regarding static and dynamic polymorphism, rather than just two distinct aspects which are mentioned in above two points. Some authors consider function and operator overloading as static polymorphism, while some authors describe generic programming by parametric polymorphism So all these are different points of view of authors to explain the polymorphism. But MSDN considers dynamic polymorphism as a more convenient definition of Polymorphism.
29. What is the use of the sealed keyword in C#?
In C#, a sealed modifier is used to prevent your class from being inherited by other classes. When you want that other class should not inherit from your class then you can add a sealed modifier to it. You can create sealed classes in C# as below.
_________________________________________________________________________
class C {}
sealed class D : C {} // other classes will not be able to inherit from class D.
________________________________________________________
You can use a sealed modifier to methods and properties in your class which are overriding methods and properties from base class. Using this way other classes will derive from your class and will not be able to override sealed methods and properties.
30. Explain string interpolation in C#?
In C#, String Interpolation provides the capability to create formatted strings with convenient and readable syntax. The $ special character allows you to create an interpolation string that might contain interpolation expressions. When the interpolation string resolves it replaces the interpolation expression with string representation of the interpolation item. String Interpolation is the feature of C# 6. Example:
___________________________________
var name = "Microsoft";
Console.WriteLine($"Hello {name}"); // O/P: Hello Microsoft
___________________________________
31. What is using statement in C#?
In C#, All unmanaged resources and class libraries must implement the IDisposable or IAsyncDisposable interface.
The using statement ensures the correct use of IDisposable or IAsyncDisposable objects. When the object’s lifetime is limited to a single method then you should use the ‘using’ statement to declare and instantiate disposable objects. An object declared inside a using statement can not be modified or re-assigned meaning it is read-only. It disposes the object in case of any exception or it’s equivalent to try-finally implementation.
using statement example:
_____________________________
string message=@"Hello I am good , first line
How are you, second line
I am fine third line";
using var reader = new StringReader(message);
string? line;
do {
line = reader.ReadLine();
Console.WriteLine(line);
} while(line != null);
_________________________________
32. How to compare two String Objects in C#?
You should use the Compare method to determine the relationship of two strings in sorting order and if you want to test for equality of two strings then you should Instance Equals or static Equals method with a StringComparison type parameter.
33. What is async and await in C#?
Both async & await keywords are used to implement asynchronous operations in C#. These operations could be either I/O bound (such as web service taking time to download some content or file) or CPU bound (any expensive calculation by CPU).
You can mark a method, lambda expression or anonymous method as async. Async method should have at least one await statement to perform operation asynchronously and it returns Task means – the ongoing work. When the control reaches the awaited expression, the method is suspended until the task gets completed because we are waiting for the result of the awaited task.
______________________________
public async Task<int> ExampleMethodAsync()
{
//...
string contents = await httpClient.GetStringAsync(requestUrl);
}
______________________________
34. What is the Constructor Chaining in C#?
We can call an overloaded constructor from another constructor using this keyword but the constructor must belong to the same class because this keyword is pointing to the members of the same class in which this is used. This type of calling the overloaded constructor is also termed as Constructor Chaining.
35. What are Generics in C#?
Generic is a class that allows the user to define classes and methods with the placeholder. Generics were added to version 2.0 of the C# language. The basic idea behind using Generic is to allow type (Integer, String, … etc, and user-defined types) to be a parameter to methods, classes, and interfaces. A primary limitation of collections is the absence of effective type checking. This means that you can put any object in a collection because all classes in the C# programming language extend from the object base class. This compromises type safety and contradicts the basic definition of C# as a type-safe language. In addition, using collections involves a significant performance overhead in the form of implicit and explicit type casting that is required to add or retrieve objects from a collection.
36. Describe Accessibility Modifiers in C#?
Access Modifiers are keywords that define the accessibility of a member, class, or datatype in a program. These are mainly used to restrict unwanted data manipulation by external programs or classes. There are 4 access modifiers (public, protected, internal, private) which defines the 6 accessibility levels as follows:
- public
- private
- private protected
- protected
- internal
- protected internal
37. What is a Virtual Method in C#?
In C# virtual method is a strategy that can be reclassified in derived classes. We can implement the virtual method in the base class and derived class. It is utilized when a method’s fundamental work is similar but in some cases derived class needed additional functionalities. A virtual method is declared in the parent class that can be overriden in the child class. We make a virtual method in the base class by using the virtual keyword and that method is overriden in the derived class using the Override keyword. It is not necessary for every derived class to inherit a virtual method, but a virtual method must be created in the base class. Hence the virtual method is also known as Polymorphism.
38. What is Multithreading with .NET?
Multi-threading is a process that contains multiple threads within a single process. Here each thread performs different activities. For example, we have a class and this call contains two different methods, now using multithreading each method is executed by a separate thread. So the major advantage of multithreading is it works simultaneously, which means multiple tasks execute at the same time. And also maximizing the utilization of the CPU because multithreading works on time-sharing concept mean each thread takes its own time for execution and does not affect the execution of another thread, this time interval is given by the operating system.
39. What is a Hash table class in C#?
The Hashtable class represents a collection of key/value pairs that are organized based on the hash code of the key. This class comes under the System. Collections namespace. The Hashtable class provides various types of methods that are used to perform different types of operations on the hashtables. In Hashtable, keys are used to access the elements present in the collection. For very large Hashtable objects, you can increase the maximum capacity to 2 billion elements on a 64-bit system.
40. Why a private virtual method cannot be overridden in C#?
Because private virtual methods are not accessible outside the class.
41. What is File Handling in C#?
Generally, the file is used to store the data. The term File Handling refers to the various operations like creating the file, reading from the file, writing to the file, appending the file, etc. There are two basic operations that are mostly used in file handling is reading and writing of the file. The file becomes stream when we open the file for writing and reading. A stream is a sequence of bytes that is used for communication. Two streams can be formed from the file one is the input stream which is used to read the file and another is the output stream is used to write in the file. In C#, the System.IO namespace contains classes that handle input and output streams and provide information about file and directory structure.
42. What is LINQ in C#?
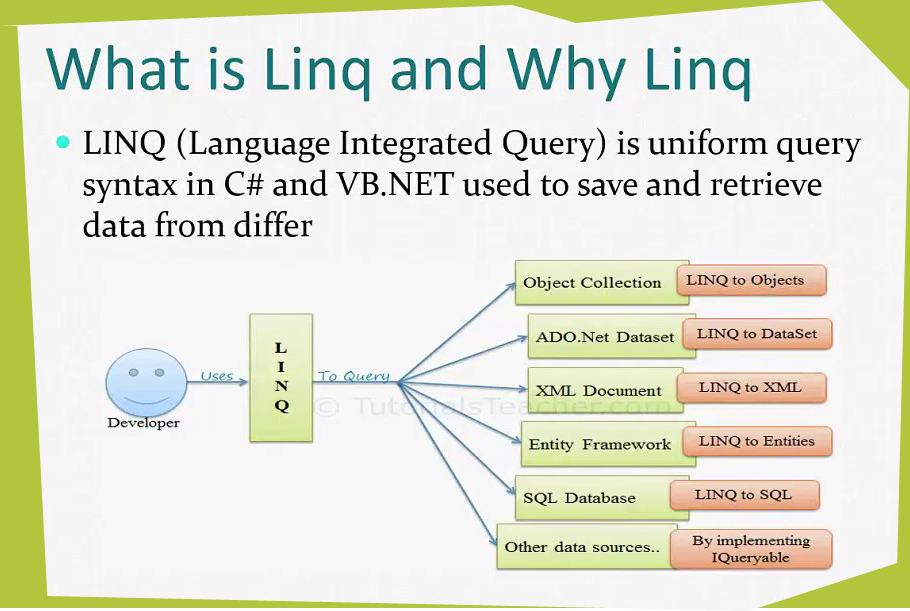
LINQ is known as Language Integrated Query and it is introduced in .NET 3.5 and Visual Studio 2008. The beauty of LINQ is it provides the ability to .NET languages(like C#, VB.NET, etc.) to generate queries to retrieve data from the data source. For example, a program may get information from the student records or accessing employee records, etc. In, past years, such type of data is stored in a separate database from the application, and you need to learn different types of query language to access such type of data like SQL, XML, etc. And also you cannot create a query using C# language or any other .NET language.
To overcome such types of problems Microsoft developed LINQ. It attaches one, more power to the C# or .NET languages to generate a query for any LINQ compatible data source. And the best part is the syntax used to create a query is the same no matter which type of data source is used means the syntax of creating query data in a relational database is the same as that used to create query data stored in an array there is no need to use SQL or any other non-.NET language mechanism. You can also use LINQ with SQL, with XML files, with ADO.NET, with web services, and with any other database.
43. What is File Handling in C#?
Generally, the file is used to store the data. The term File Handling refers to the various operations like creating the file, reading from the file, writing to the file, appending the file, etc. There are two basic operations that are mostly used in file handling is reading and writing of the file. The file becomes stream when we open the file for writing and reading. A stream is a sequence of bytes that is used for communication. Two streams can be formed from the file one is the input stream which is used to read the file and another is the output stream is used to write in the file. In C#, the System.IO namespace contains classes that handle input and output streams and provide information about file and directory structure.
44. Why to use finally block in C#?
Finally block will be executed irrespective of exception. So while executing the code in try block when exception is occurred, control is returned to catch block and at last finally block will be executed. So closing connection to database / releasing the file handlers can be kept in finally block.
45. What is Singleton design pattern in C#?
Singleton design pattern in C# is a common design pattern. In this pattern, a class has just one instance in the program that gives global access to it. Or we can say that a singleton is a class that permits only one instance of itself to be made and usually gives simple access to that instance.
There are different approaches to carry out a singleton design in C#. Coming up next are the regular attributes of a singleton design.
- Private and parameterizes single constructor
- Sealed class.
- Static variable to hold a reference to the single made example
- A public and static method of getting the reference to the made example.
46. How to implement a singleton design pattern in C#?
We can implement a singleton design pattern in C# using:
- No Thread Safe Singleton.
- Thread-Safety Singleton.
- Thread-Safety Singleton using Double-Check Locking.
- Thread-safe without a lock.
- Using .NET 4’s Lazy type.
47. Write a Features of Generics in C#?
Generics is a technique that improves your programs in many ways such as:
- It helps you in code reuse, performance, and type safety.
- You can create your own generic classes, methods, interfaces, and delegates.
- You can create generic collection classes. The .NET Framework class library contains many new generic collection classes in System.Collections.Generic namespace.
- You can get information on the types used in generic data types at run-time.
48. What are namespaces in C#?
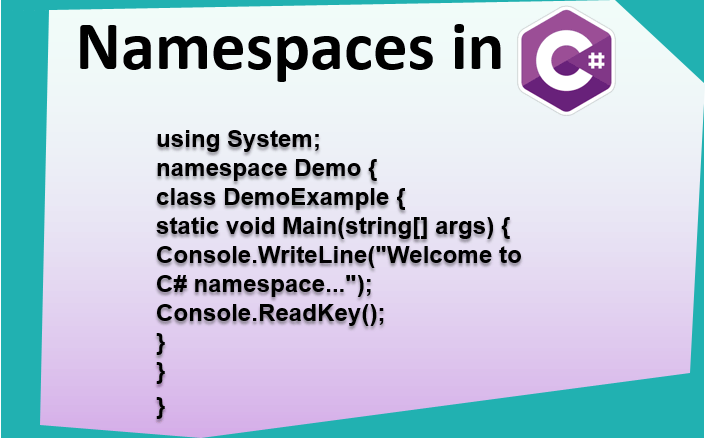
It provides a way to keep one set of names(like class names) different from other sets of names. The biggest advantage of using namespace is that the class names which are declared in one namespace will not clash with the same class names declared in another namespace. It is also referred as named group of classes having common features.
49. Who can be the members of namespaces in C#?
The members of a namespace can be namespaces, interfaces, structures, and delegates.
50. What will be the output of the following code snippet: ?
____________________________________
using System;
public class Program
{
public static void Main(string[] args)
{
int[] i = new int[0];
Console.WriteLine(i[0]);
}
}
______________________________
a) 0
b) IndexOutOfRangeException
c) Nothing is printed as array is empty
d) 1
Answer- Option B
- Mastering Qualitative Research: The Role of Focus Groups in Data Collection - July 11, 2024
- What is robots ops? - July 10, 2024
- 5 Effective Online Learning Strategies for DevOps Professionals - July 4, 2024