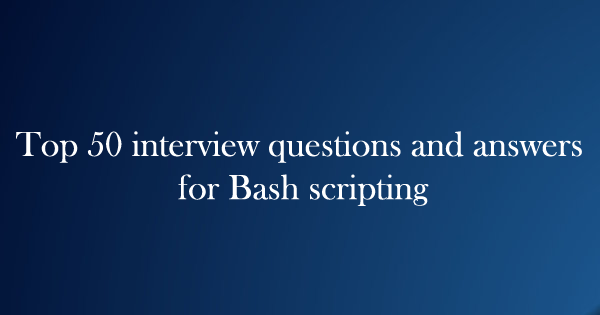
Bash Scripting is a powerful a part of system administration and development used at an extreme level. It’s utilized by the System directors, Network Engineers, Developers, Scientists, and everybody UN agency use Linux/Unix package. They use Bash for system administration, knowledge crunching, net application preparation, automatic backups, making custom scripts for numerous pages, etc.
Script:
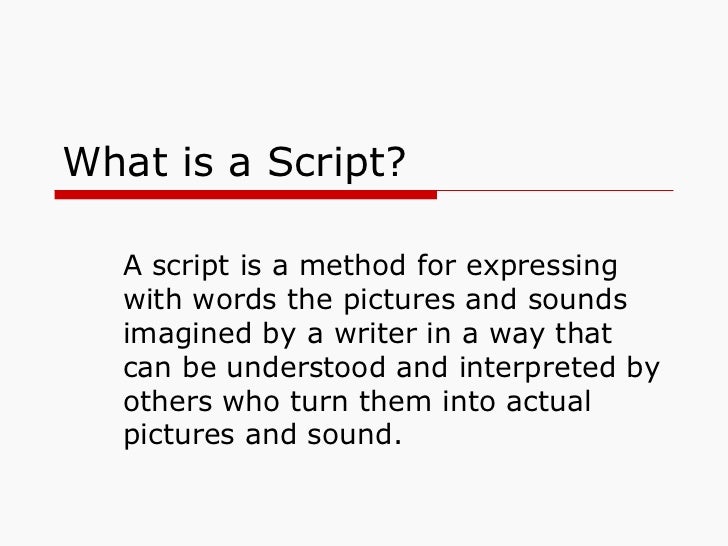
In computer programing, a script could be a set of commands for an applicable run time atmosphere that is employed to alter the execution of tasks.
Bash Script:
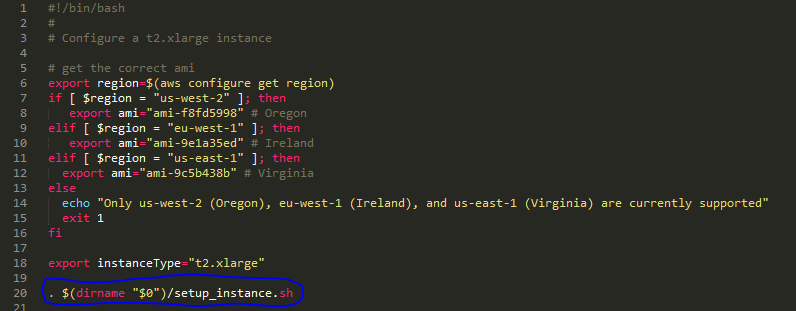
A Bash Shell Script could be a plain document containing a group of assorted commands that we have a tendency to typically sort within the command. Its accustomed alter repetitive tasks on UNIX system filesystem. It would embody a group of commands, or one command, or it would contain the hallmarks of imperative programming like loops, functions, conditional constructs, etc. Effectively, a Bash script could be a bug written within the Bash artificial language.
We had discussed the bash, script and bash scripting. Now, let’s move on to the FAQ’s and interview questions of Bash scripting.
Interview questions and answers for Bash scripting:-
- What is bash script?
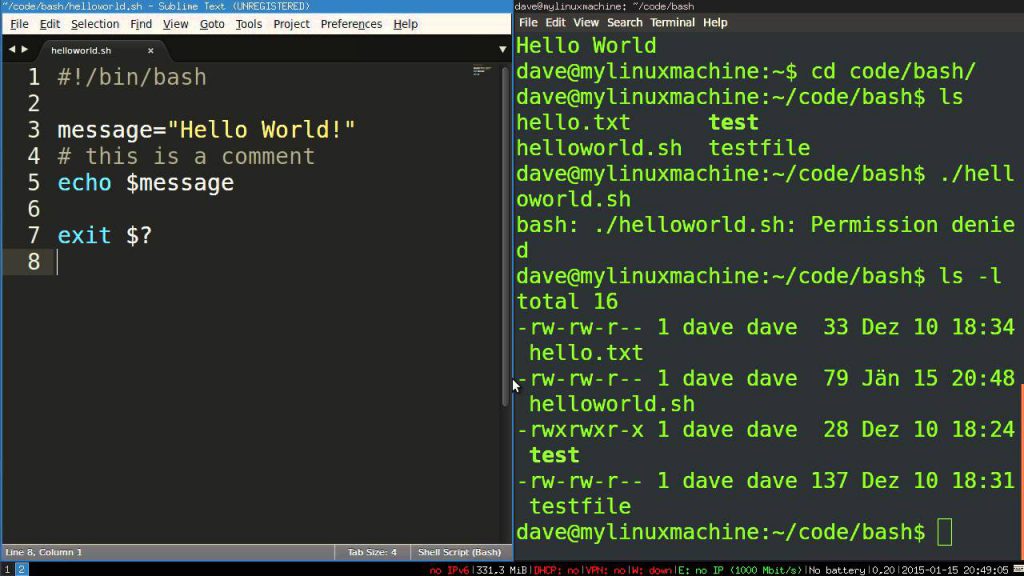
The bash script is a shell programming language. Generally, we run many types of shell commands from the terminal by typing each command separately that require time and efforts. If we need to run the same commands again then we have to execute all the commands from the terminal again. But using a bash script, we can store many shell command statements in a single bash file and execute the file any time by a single command. Many system administration related tasks, program installation, disk backup, evaluating logs, etc. can be done by using proper bash script.
2. What are the advantages of using bash scripts?
Bash script has many advantages which are described below:
- It is easy to use and learn.
- Many manual tasks that need to run frequently can be done automatically by writing a bash script.
- The sequence of multiple shell commands can be executed by a single command.
- Bash script written in one Linux operating system can easily execute in other Linux operating system. So, it is portable.
- Debugging in bash is easier than other programming languages.
- Command-line syntax and commands that are used in the terminal are similar to the commands and syntax used in bash script.
- Bash script can be used to link with other script files.
3. Mention the disadvantages of bash scripts
Some disadvantages of bash script are mentioned below:
- It works slower than other languages.
- The improper script can damage the entire process and generate a complicated error.
- It is not suitable for developing a large and complex application.
- It contains less data structure compare to other standard programming languages.
4. What types of variables are used in bash?
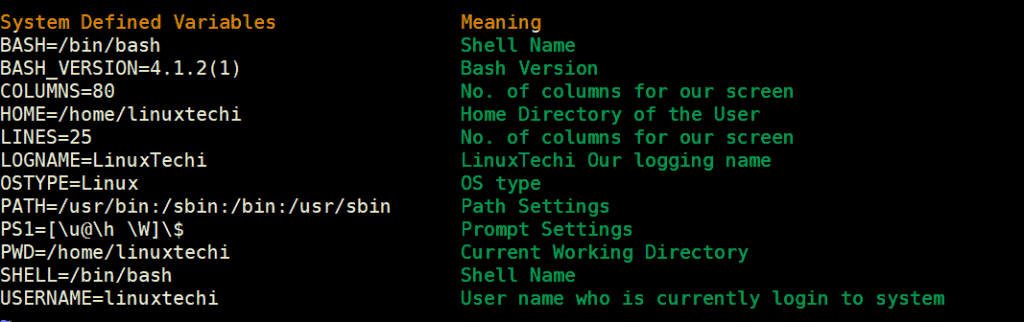
Two types of variables can be used in bash script. These are:
System variables
The variables which are pre-defined and maintained by the Linux operating system are called system variables. These type of variables are always used by an uppercase letter. The default values of these variables can be changed based on requirements.
`set`, `env` and `printenv` commands can be used to print the list of system variables.
#!/bin/bash
# Printing System Variables
#Print Bash shell name
echo $BASH
# Print Bash shell Version
echo $BASH_VERSION
# Print Home directory name
echo $HOME
5. How to declare and delete variables in bash?
The variable can be declared in bash by data type or without data type. If any bash variable is declared without declare command, then the variable will be treated as a string. Bash variable is declared with declare command to define the data type of the variable at the time declaration.
–r, -i, -a, -A, -l, -u, -t and –x options can be used with declare command to declare a variable with different data types.
#!/bin/bash
#Declare variable without any type
num=10
#Values will be combined but not added
result=$num+20
echo $result
#Declare variable with integer type
declare -i num=10
#Values will be added
declare -i result=num+20
echo $result
6. How to add comments in a bash script?
Single line and multi-line comments can be used in bash script. ‘#‘ symbol is used for single-line comment. ‘<<’ symbol with a delimiter and ‘:’ with single (‘) are used for adding multi-line comment.
Example:
#!/bin/bash
#Print the text [Single line comment]
echo "Bash Programming"
<<addcomment
Calculate the sum
Of two numbers [multiline comment]
addcomment
num=25+35
echo $num
: '
Combine two
String data [multiline comment]
'
String="Hello"
echo $string" World"
7. How can you combine strings in a bash script?
String values can be combined in bash in different ways. Normally, the string values are combined by placing together but there are other ways in bash to combine string data.
Example:
#!/bin/bash
#Initialize the variables
str1=”PHP”
str2=”Bash”
str3=”Perl”
# Print string together with space
echo $str1 $str2 $str3
#Combine all variables and store in another variable
str=”$str1, $str2 and $str3″
#Combine other string data with the existing value of the string
str+=” are scripting languages”
#Print the string
echo $str
8. Which commands are used to print output in bash?
`echo` and `printf` commands can be used to print output in bash. `echo` command is used to print the simple output and `printf` command is used to print the formatted output.
Example:
#!/bin/bash
#Print the text
echo "Welcome to LinuxHint"
site="linuxhint.com"
#Print the formatted text
printf "%s is a popular blog site\n" $site
How to take input from the terminal in bash?
`read` command is used in a bash script to take input from the terminal.
#!/bin/bash
#Print message
echo "Enter your name"
#Take input from the user
read name
# Print the value of $name with other string
echo "Your name is $name"
9. How to use command-line arguments in bash?
Command-line arguments are read by $1, $2, $3…$n variables. Command-line argument values are provided in the terminal when executing the bash script. $1 is used to read the first argument, $2 is used to read the second argument and so on.
#!/bin/bash
#Check any argument is provided or not
if [[ $# -eq 0 ]]; then
echo “No argument is given.”
exit 0
fi
#Store the first argument value
color=$1
# Print the argument with other string
printf “You favorite color is %s\n” $color
10. What are the advantages and disadvantages of Bash Scripting?
Advantages
- The syntax and structure of Bash scripts are easy to understand.
- There are various commands which are general and common; hence they are easy to remember.
- As it is an interpreted language, so compilation does not require before running.
- Highly efficient.
Disadvantages
- Input and Output performance is not that good. It is low as compared to other languages.
- The inspection of variables is not strict.
- It usually does not come pre-installed in windows, so there are chances that the code may not be compatible with the operating system.
- Bash does not support many functions, for example- objects, data structures, etc.
- Poor debugging tools.
11. Explain about “s” permission bit in a file?
“S” bit is called “set user id” (SUID) bit.
“S” bit on a file causes the process to have the privileges of the owner of the file during the instance of the program.
For example, executing “passed” command to change current password causes the user to writes its new password to shadow file even though it has “root” as its owner.
12. Create a directory such that anyone in the group can create a file and access any person’s file in it but none should be able to delete a file other than the one created by himself.
We can create the directory giving read and execute access to everyone in the group and setting its sticky bit “t” on as follows:
mkdir direc1
chmod g+wx direc1
chmod +t direc1
13. How can you find out how long the system has been running?
We can find this by using the command “uptime”.
14. How can any user find out all information about a specific user like his default shell, real-life name, default directory, when and how long he has been using the system?
finger “loginName” …where loginName is the login name of the
user whose information is expected.
15. What is the difference between $$ and $!?
$$ gives the process id of the currently executing process whereas $! Shows the process id of the process that recently went into the background.
16. mWhat are zombie processes?
These are the processes which have died but whose exit status is still not picked by the parent process. These processes even if not functional still have its process id entry in the process table.
17. How will you copy a file from one machine to other?
We can use utilities like “ftp,” “scp” or “rsync” to copy a file from one machine to other.
E.g., Using ftp:
FTP hostname
>put file1
>bye
Above copies, file file1 from the local system to destination system whose hostname is specified.
18. I want to monitor a continuously updating log file, what command can be used to most efficiently achieve this?
We can use tail –f filename. This will cause only the default last 10 lines to be displayed on std o/p which continuously shows the updating part of the file.
19. I want to connect to a remote server and execute some commands, how can I achieve this?
We can use ssh to do this:
ssh username@serverIP -p sshport
Example
ssh root@122.52.251.171 -p 22
Once above command is executed, you will be asked to enter the password
20. I have 2 files and I want to print the records which are common to both.
We can use tail –f filename. This will cause only the default last 10 lines to be displayed on std o/p which continuously shows the updating part of the file.
21. I want to connect to a remote server and execute some commands, how can I achieve this?
We can use ssh to do this:
ssh username@serverIP -p sshport Example
ssh root@122.52.251.171 -p 22
Once above command is executed, you will be asked to enter the password
22. I have 2 files and I want to print the records which are common to both.
We can use “comm” command as follows:
comm -12 file1 file2 … 12 will suppress the content which are
unique to 1st and 2nd file respectively.
23. Write a script to print the first 10 elements of Fibonacci series.
#!/bin/sh
a=1
b=1
echo $a
echo $b
for I in 1 2 3 4 5 6 7 8
do
c=a
b=$a
b=$(($a+$c))
echo $b
done
24. How will you connect to a database server from Linux?
We can use isql utility that comes with open client driver as follows:
isql –S serverName –U username –P password
25. What are the 3 standard streams in Linux?
0 – Standard Input1 – Standard Output2 – Standard Error
26. I want to read all input to the command from file1 direct all output to file2 and error to file 3, how can I achieve this?
command <file1 1>file2 2>file3
27. What will happen to my current process when I execute a command using exec?
“exec” overlays the newly forked process on the current process; so when I execute the command using exec, the command gets executed on the current shell without creating any new processes.
E.g., Executing “exec ls” on command prompt will execute ls and once ls exits, the process will shut down
28. How will you emulate wc –l using awk?
awk ‘END {print NR} fileName’
29. Given a file find the count of lines containing the word “ABC”.
grep –c “ABC” file1
30. What is the difference between grep and egrep?
egrep is Extended grep that supports added grep features like “+” (1 or more occurrence of a previous character),”?”(0 or 1 occurrence of a previous character) and “|” (alternate matching)
31. How will you print the login names of all users on a system?
/etc/shadow file has all the users listed.
awk –F ':' '{print $1}' /etc/shadow|uniq -u
32. How will you print the login names of all users on a system?
/etc/shadow file has all the users listed.
awk –F ':' '{print $1}' /etc/shadow|uniq -u
How to set an array in Linux?
Syntax in ksh:
Set –A arrayname= (element1 element2 ….. element)
In bash
A=(element1 element2 element3 …. elementn)
33. Write down the syntax of “for ” loop
Syntax:
for iterator in (elements)
do
execute commands
done
34. How will you find the total disk space used by a specific user?
du -s /home/user1 ….where user1 is the user for whom the total disk space needs to be found.
35. Write the syntax for “if” conditionals in Linux?
Syntax
If condition is successful
then
execute commands
else
execute commands
fi
What is the significance of $?
The command $? gives the exit status of the last command that was executed.
36. How do we delete all blank lines in a file?
sed '^ [(backslash)011(backslash)040]*$/d' file1
where (backslash)011 is an octal equivalent of space and
(backslash)040 is an octal equivalent of the tab
37. How will I insert a line “ABCDEF” at every 100th line of a file?
sed ‘100i\ABCDEF’ file1
38. Write a command sequence to find all the files modified in less than 2 days and print the record count of each.
find . –mtime -2 –exec wc –l {} \;
39. How can subroutines be declared and called in bash?
In bash a function or procedure is called a subroutine. The declaration and calling of a subroutine in bash is different from other languages. No argument can be declared in subroutines unlike other standard programming languages. But local variables can be defined within the subroutine by using the ‘local’ keyword.
Example:
#!/bin/bash
# Initialize the variable $x and $y which are global
x=10
y=35
# Declare the function
myFunc () {
# Declare the local variable $x
local x=15
# Re-assign the global variable $y
y=25
# Calculate the sum of $x and $y
z=$((x+y))
# Print the sum of a local variable, $x, and global variable, $y
echo "The sum of $x and $y equal to $z"
}
# Call the function
myFunc
# Print the sum of global variables, $x, and $y
echo "The sum of $x and $y equal to $z"
40. How to cut and print some part of a string data in bash?
Bash has no built-in function like other languages to cut some portion of the string data. But using parameter expansion any string value can be cut in bash. Three parts can be defined in parameter expansion by separating with a colon to cut any part of the string data. Here, the first two parts are mandatory and the last part is optional. The first part contains the main string variable that will be used to cut, the second part is the starting position from where the string will be cut and the third part is the length of the cutting string. The starting position must be counted from 0 and the length must be counted from 1 of the main string to retrieve the cutting value.
Example:
#!/bin/bash
# Initialize a string value into $string
string="Python Scripting Language"
# Cut the string value from the position 7 to the end of the string
echo ${string:7}
# Cut the string value of 9 characters from the position 7
echo ${string:7:9}
# Cut the string value from 17 to 20
echo ${string:17:-4}
41. Mention some ways to perform arithmetic operations in bash?
Arithmetic operations can be done in multiple ways in bash. ‘let’, ‘expr’, ‘bc’ and double brackets are the most common ways to perform arithmetic operations in bash. The uses of these commands are shown in the following example.
Example:
#!/bin/bash
# Calculating the subtraction by using expr and parameter expansion
var1=$( expr 120 - 100 )
# print the result
echo $var1
# Calculate the addition by using let command
let var2=200+300
# Print the rsult
echo $var2
# Calculate and print the value of division using ‘bc’ to get the result
# with fractional value
echo "scale=2; 44/7" | bc
# Calculate the value of multiplication using double brackets
var3=$(( 5*3 ))
# Print the result
echo $var3
42. How to check a directory exists or not using bash?
Bash has many test commands to check if a file or directory exists or not and the type of the file. ‘-d’ option is used with a directory path as a conditional statement to check if the directory exists or not in bash. If the directory exists, then it will return true otherwise it will return false.
Example:
#!/bin/bash
# Assign the directory with path in the variable, $path
path="/home/ubuntu/temp"
# Check the directory exists or not
if [ -d "$path" ]; then
# Print message if the directory exists
echo "Directory exists"
else
# Print message if the directory doesn’t exist
echo "Directory not exists"
fi
43. How can a bash script be terminated without executing all statements?
Using ‘exit’ command, a bash script can be terminated without executing all statements. The following script will check if a particular file exists or not. If the file exists, then it will print the total characters of the file and if the file does not exist then it will terminate the script by showing a message.
Example:
#!/bin/bash
# Initialize the filename to the variable, $filename
filename="course.txt"
# Check the file exists or not by using -f option
if [ -f "$filename" ]; then
# Print message if the file exists
echo "$filename exists"
else
# Print message if the file doesn't exist
echo "$filename not exists"
# Terminate the script
exit 1
fi
# Count the length of the file if the file exists
length=`wc -c $filename`
# Print the length of the file
echo "Total characters - $length"
44. What are the uses of break and continue statements in bash?
break statement is used to terminate from a loop without completing the full iteration based on a condition and continue statement is used in a loop to omit some statements based on a condition. The uses of break and continue statements are explained in the following example.
Example:
#!/bin/bash
# Initialize the variable $i to 0 to start the loop
i=0
# the loop will iterate fot 10 times
while [ $i -le 10 ]
do
# Increment the value $i by 1
(( i++ ))
# If the value of $i equal to 8 then terminate the loop by using 'break' statement
if [ $i -eq 8 ]; then
break;
fi
# If the value of $i is greater than 6 then omit the last statement of the loop
# by using continue statement
if [ $i -ge 6 ]; then
continue;
fi
echo "the current value of i = $i"
done
# Print the value of $i after terminating from the loop
echo "Now the value of i = $i"
45. How to make a bash file executable?
Executable bash files can be made by using ‘chmod’ command. Executable permission can be set by using ‘+x’ in chmod command with the script filename. Bash files can be executed without the explicit ‘bash’ command after setting the execution bit for that file.
Example:
# Set the execution bit
$ chmod +x filename.sh
# Run the executable file
$ ./filename.sh
46. What is meant by ‘bc’ and how can this command can be used in bash?
The full form of ‘bc’ is Bash Calculator to perform arithmetic operations more accurately in bash. The fractional part is omitted if any arithmetic operation is done in bash by using ‘expr’ command. The fractional part can be rounded also by using scale value with ‘bc’ command.
Example:
#!/bin/bash
# Calculate the division without the fractional value
echo "39/7" | bc
# Calculate the division with the full fractional value
echo "39/7" | bc -l
# Calculate the division with three digits after the decimal point
echo "scale=3; 39/7" | bc
47. How can you print a particular line of a file in bash?
There are many ways to print a particular line in bash. How the ‘awk’, ‘sed’ and ‘tail’ commands can be used to print a particular line of a file in bash is shown in the following example.
Example:
#!/bin/bash
# Read and store the first line from the file by using `awk` command with NR variable
line1=`awk '{if(NR==1) print $0}' course.txt`
# Print the line
echo $line1
# Read the second line from the file by using `sed` command with -n option
line2=`sed -n 2p course.txt`
# Print the line
echo $line2
# Read the last line from the file by using `tail` command with -n option
line3=`tail -n 1 course.txt`
# Print the file
echo $line3
48. What is IFS?
IFS is a special shell variable. The full form of IFS is Internal Field Separator,
it acts as delimiter to separate the word from the line of text. It is mainly used for splitting a string, reading a command, replacing text etc.
Example:
#!/bin/bash
# Declare ':' as delimiter for splitting the text
IFS=":"
# Assign text data with ':' to $text
text="Red:Green:Blue"
# for loop will read each word after splitting the text based on IFS
for val in $text; do
# Print the word
echo $val
done
49. How to find out the length of a string data?
‘expr’, ‘wc’ and ‘awk’ commands can be used to find out the length of a string data in bash. ‘expr’ and ‘awk’ commands use length option, ‘wc’ command uses ‘–c’ option to count the length of the string.
Example:
The uses of the above commands are shown in the following script.
#!/bin/bash
# Count length using `expr` length option
echo `expr length "I like PHP"`
# Count length using `wc` command
echo "I like Bash" | wc -c
# Count length using `awk` command
echo "I like Python" | awk '{print length}'
50. How to run multiple bash script in parallel?
Multiple bash scripts can be executed in parallel by using nohup command. How multiple bash files can be executed in parallel from a folder is shown in the following example.
Example:
# Assign a folder name with the path in the variable $dir that contains
# multiple bash files
dir="home/Ubuntu/temp"
# for loop will read each file from the directory and execute in parallel
for script in dir/*.sh
do
nohup bash "$script" &
done
51. How can conditional statements be used in bash? The most common conditional statement in most programming languages is the if-elseif-else statement. The syntax of if-elseif-else statement in bash is a little bit different from other programming languages. ‘If’ statement can be declared in two ways in a bash script and every type of ‘if’ block must be closed with ‘fi’. ‘if’ statement can be defined by third brackets or first brackets like other programming languages
Syntax:
A.
if [ condition ];
then
statements
fi
B.
if [ condition ]; then
statements 1
else
statement 2
fi
C.
if [ condition ]; then
statement 1
elif [ condition ]; then
statement 2
….
else
statement n
fi
Example:
#!/bin/bash
# Assign a value to $n
n=30
# Check $n is greater than 100 or not
if [ $n -gt 100 ]; then
echo "$n is less than 100"
# Check $n id greater than 50 or not
elif [ $n -gt 50 ]; then
echo "$n is less than 50"
else
echo "$n is less than 50"
fi
This article has all the interview questions and answers of Bash scripting. All the possible FAQ’s are mentioned for the readers. If you are one of them who wants to make their career in bash scripting and want to become a bash script programmer in future, then you can vist devopsschool website for better options.
- Mastering Qualitative Research: The Role of Focus Groups in Data Collection - July 11, 2024
- What is robots ops? - July 10, 2024
- 5 Effective Online Learning Strategies for DevOps Professionals - July 4, 2024