- What is Objective C & why it is used for?
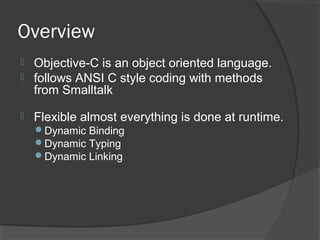
An ANSI-based version of the standard C programming language, Objective-C is the key programming language used for mobile app development by companies that make apps for OS X and iOS. Objective-C is developed on top of C language by adding Small Talk features that make it an object-based language.
2. What Objective-C program consists of?
The objective-c program basically consists of
- Preprocessor commands
- Interface
- Implementation
- Method
- Variables
- Statements & Expressions
- Comments
3. What is the protocol in Objective C?
In Objective-C, a protocol is a language feature, that provides multiple inheritances in a single inheritance language. Objective C supports two types of protocol.
- Ad hoc protocols known as informal protocol
- Compiler protocols are known as formal protocol
4. Explain what is OOP?
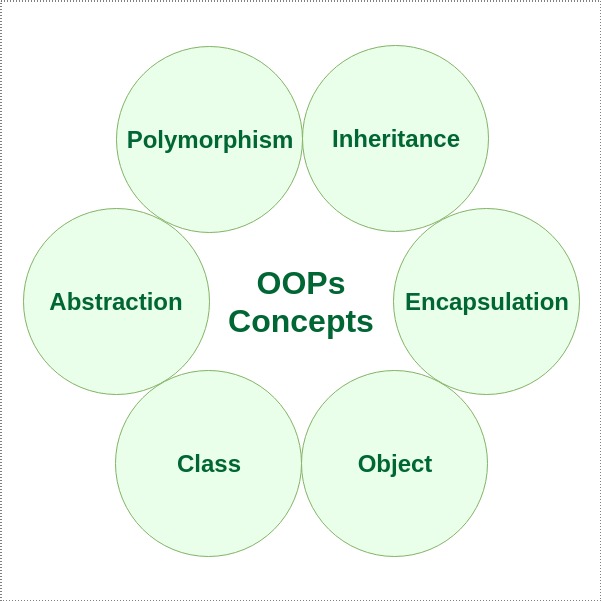
OOP means Object Oriented Programming; it is a type of programming technique that helps to manage a set of objects in a system. With the help of various programming languages, this method helps to develop several computer programs and applications.
5. What is the difference between polymorphism and abstraction?
Abstraction in OOP is the process of reducing the unwanted data and maintaining only the relevant data for the users while polymorphism enables an object to execute their functions in two or more forms.
6. What is parsing? Mention which class can you use for parsing of XML in iPhone?
Parsing is the process to access the data in the XML element. We can use class “NSXML” parser for parsing XML in iPhone.
7. What is an accessor method?
Accessor methods are methods belonging to a class that enables you to get and set the values of instance valuable contained within the class.
8. Which class is used to establish a connection between applications to the web server?
The class used to establish a connection between applications to the web server are
- NSURL
- NSURL REQUEST
- NSURL CONNECTION
9. List out the methods used in NSURL connection?
Methods used in NSURL connection are
- Connection did receive response
- Connection did receive data
- Connection fails with error
- Connection did finish loading
10. Explain class definition in Objective-C?
A class definition begins with the keyword @interface followed by the interface (class) name, and the class body, closed by a pair of curly braces. In Objective-C, all classed are retrieved from the base class called NSObject. It gives basic methods like memory allocation and initialization.
11. What are the characteristics of the category?
Characteristics of category include:
- Even if you don’t have the original source code for implementation, a category can be declared for any class
- Any methods that you define in a category will be available to all instances of the original class as well as any sub-classes for the original class
- At runtime, there is no variation between a method appended by a category and one that is implemented by the original class.
12. What is the use of category in Objective-C?
The use of category in Objective-C is to extend an existing class by appending behavior that is useful only in certain situations. In order to add such extension to existing classes, objective –C provides extensions and categories. The syntax used to define a category is @interface keyword.
13. What is single inheritance in Objective-C?
The objective-c subclass can only be obtained from a single direct parent class this concept is known as “single inheritance.”
14. What is synthesized in Objective-C?
Once you have declared the property in Objective-C, you have to tell the compiler instantly by using synthesize directive. This will tell the compiler to generate a getter&setter message.
15. When would you use NSArray and NSMutableArray?
- NSArray: You will use an NS array when data in the array don’t change. For example, the company name you will put in NS Array so that no one can manipulate it.
- NSMutableArray: This array will be used in an array when data in an array will change. For instance, if you are passing an array to function and that function will append some elements in that array then you will choose NSMutable Array.
16. How is string represented in Objective-C?
In Objective-C, the string is represented by using NSS string and its sub-class NSMutableString provides several ways for creating string objects.
17. Explain what is data encapsulation in Objective-C?
In Objective-C, data encapsulation is referred to as the mechanism of connecting the data and the functions that use them.
18. What are objective- C blocks?
In Objective-C class, there is an object that combines data with related behavior. It enables you to form distinct segments of code that can be passed around to functions or methods as if they were values. Objective-C blocks can be added to collections like NSDictionary or NSArray.
19. Explain how to call a function in Objective-C?
To call the function in Objective-C, you have to do Account -> Object Name -> Display account information -> Method name
20. What is the main difference between function calls and messages?
The main difference between a function call and message is that a function and its arguments are linked together in the compiled code, but a message and a receiving object are not linked until the program is executing and the message is sent.
21. Explain how the class “IMPLEMENTATION” is represented in Objective-C?
In Objective-C the class “ IMPLEMENTATION” is represented with @implementation directive and ends with @end.
22. How messaging works in Objective-C?
Messaging is not bound to method implementation until runtime in Objective-C. The compiler transforms a message expression, into a call on a messaging function, objc_msgSend(). This function connects the receiver and the name of the method mentioned in the message.
23. What is dot notation?
Dot notation involves assessing an instance variable by determining a class “instance” followed by a “dot” followed in turn by the name of the instance variable or property to be accessed.
24. Explain types of protocol in Objective-C?
A formal protocol declares a list of methods that client classes are expected to implement. Formal protocols have their own declaration, adoption, and type-checking syntax. You can designate methods whose implementation is required or optional with the @required and @optional keywords. Subclasses inherit formal protocols adopted by their ancestors. A formal protocol can also adopt other protocols. Formal protocols are an extension to the Objective-C language.
An informal protocol is a category on NSObject, which implicitly makes almost all objects adopters of the protocol. (A category is a language feature that enables you to add methods to a class without subclassing it.) Implementation of the methods in an informal protocol is optional. Before invoking a method, the calling object checks to see whether the target object implements it. Until optional protocol methods were introduced in Objective-C 2.0, informal protocols were essential to the way Foundation and AppKit classes implemented delegation.
25. What is the difference between #import and #include in Objective-C?
import is super set of include, it make sure file is included only once. this save you from recursive inclusion. about “” and <>. “” search in local directory and <> is use for system files.
26. What is fast enumeration?
The object is usually a collection such as an array or set Several Cocoa classes, including the collection classes, adopt the NSFastEnumeration protocol. You use it to retrieve elements held by an instance using a syntax similar to that of a standard C for loop, as illustrated in the following example:
______________________________________
NSArray *anArray = // get an array;
for (id element in anArray)
{
/* code that acts on the element */
}
_____________________________
27. Explain what is @synthesize in Objective-C?
@synthesize creates a getter and a setter for the variable. This lets you specify some attributes for your variables and when you @synthesize that property to the variable you generate the getter and setter for the variable.
28. Explain how to call function in Objective-C?
calling the method is like this
__________________________
[className methodName]
_____________________
however if you want to call the method in the same class you can use self
___________________
[self methodName]
________________
29. Mention whether NSObject is a parent class or derived class.
Objective-C defines a root class from which the vast majority of other classes inherit, called NSObject. When one object encounters another object, it expects to be able to interact using at least the basic behavior defined by the NSObject class description.
30. Explain the difference between atomic and nonatomic synthesized properties.
- Atomic is the default behavior will ensure the present process is completed by the CPU, before another process accesses the variable is not fast, as it ensures the process is completed entirely.
- Non-Atomic is NOT the default behavior faster (for synthesized code, that is, for variables created using @property and @synthesize) not thread-safe may result in unexpected behavior, when two different process access the same variable at the same time.
31. What is GCD? What are advantages over NSThread?
- GrandcentralDispatch: Because your device only has one processor, GCD probably only creates one thread for executing blocks and your blocks execute sequentially. You’ve created 10 different threads, though, and those each get a little piece of the available processing time. Fortunately, sleeping isn’t very processor-intensive, so all your threads run together pretty well. Try a similar test on a machine with 4 or 8 processing cores, and you’ll see GCD run more of your blocks in parallel.
- The nice thing about GCD isn’t that it necessarily offers better performance than threads, it’s that the programmer doesn’t have to think about creating threads or matching the number of threads to the number of available processors. You can create lots of little tasks that will execute as a processor becomes available and let the system schedule those tasks for you.
32. What is responder chain?
The responder chain is a series of linked responder objects. It starts with the first responder and end with the app object. If the first responder cannot handle an event, it forwards the event to the next responder in the responder chain.
33. What is id?
id is a pointer to any type, but unlike void * it always points to an Objective-C object. For example, you can add anything of type id to an NSArray, but those objects must respond to retain and release .
34. Does Objective-C have function overloading?
objective-C does not support method overloading, so you have to use different method names.
35. What is delegate? Can delegates be retained?
A delegate is an object that acts on behalf of, or in coordination with, another object when that object encounters an event in a program.
It’s up to you. If you declare it to be retained (strong in ARC) it’ll be retained.
The rule is to not retain it because it’s already retained elsewhere and more important you’ll avoid retain cycles.
36. What is class extension? Why do we require them?
Objective-C allows you to add your own methods to existing classes through categories and class extensions.
Class Extensions Extend the Internal Implementation.
A class extension bears some similarity to a category, but it can only be added to a class for which you have the source code at compile time (the class is compiled at the same time as the class extension). The methods declared by a class extension are implemented in the @implementation block for the original class so you can’t, for example, declare a class extension on a framework class, such as a Cocoa or Cocoa Touch class like NSString.
The syntax to declare a class extension is similar to the syntax for a category, and looks like this:
____________________________
@interface ClassName ()
@end
______________________
Because no name is given in the parentheses, class extensions are often referred to as anonymous categories. Unlike regular categories, a class extension can add its own properties and instance variables to a class.
37. Can you write setter method for a retain property?
Yes, To provide custom access logic, you will need to write your own getter and setter methods.
38. How does dispatch_once manages to run only once?
dispatch_once() is synchronous process and all GCD methods do things asynchronously (case in point, dispatch_sync() is synchronous)
The entire idea of dispatch_once() is “perform something once and only once”, which is precisely what we’re doing. dispatch_once that’s used to guarantee that something happens exactly once, no matter how violent the program’s threading becomes.
39. Is Objective C, a dynamic language? True/False, explain?
True, Objective-C (don’t forget the hyphen) is a cross between C and Smalltalk. The former is a statically typed language. The latter is dynamically typed. So Objective-C is not only a dynamic language.
40. What are the different types of protocols?

Protocols are of two types – formal and informal.
An extension of the Objective-C language, a formal protocol announces a list of methods that client classes are likely to implement.
An informal protocol is one of the categories on NSObject that makes all objects adopters of the protocol. Implementing methods in an informal protocol is optional.
41. How is #import different from #include?
- import function ensures a file is included only once so that you do not have a problem with recursive include.
- import is a super set of include and it ensures file is included once.
42. What happens when you create a block?
Blocks are also used for callbacks, defining the code to be executed when a task completes. As an example, your app might need to respond to a user action by creating an object that performs a complicated task, such as requesting information from a web service. Because the task might take a long time, you should display some kind of progress indicator while the task is occurring, then hide that indicator once the task is complete.
43. What is the isa member?
Objective-C objects are basically C structs. Each one contains a field called isa, which is a pointer to the class that the object is an instance of (that’s how the object and Objective-C runtime knows what kind of object it is).
44. What happens with the objects if the array is released?
Releasing the array has the same effect as removing all the items from the array. That is, the array no longer claims ownership of them. If anyone else has retained the objects, they’ll continue to exist. If not, they’ll be deallocated.
45. How can we put nil it into dictionary/array?
The nil in initWithObjects: is only there to tell the method where the list ends, because of how C var args work. When you add objects one-by-one with addObject: you don’t need to add a nil.
46. What happen if we send any message to an object which is released?
Calling release on an object does not necessarily mean it’s going to be freed. It just decrements the object’s retain count. It’s not until the retain count reaches 0 the object gets freed (and even then, the object might be in an autorelease pool and still not be freed quite then).
So, you might release your object but you could still be pointing to it. And then it could get autoreleased. And then you send it a message — but maybe the object is garbage now.
47. What is bundle?
A bundle is simply a self contained executable file. When you run it, it executes a main function that is defined inside, and uses only the resources that it contains.
48. What is the difference between a function and a stored procedure in .NET?
Function | Stored Procedure |
Can only return one value | Can return any number of values |
No support for exception handling using try-catch blocks | Supports the usage of try-catch blocks for exception handling |
The argument consists of only one input parameter | Both input and output parameters are present |
A function can be called from a stored procedure | The stored procedure cannot be called from a function |
49. What are the constructor types present in C# .NET?
There are five main types of constructor classes in C# as listed below:
- Copy constructor
- Default constructor
- Parameterized constructor
- Private constructor
- Static constructor
50. What are some of the advantages of using a session?
There are numerous advantages of making use of a session are as mentioned below:
- It is used to store user data across the span of an application.
- It is very easy to implement and store any sort of object in the program.
- Individual entities of user data can be stored separately if required.
- The session is secure, and objects get stored on the runtime server.
- Mastering Qualitative Research: The Role of Focus Groups in Data Collection - July 11, 2024
- What is robots ops? - July 10, 2024
- 5 Effective Online Learning Strategies for DevOps Professionals - July 4, 2024