1) What is PHP?
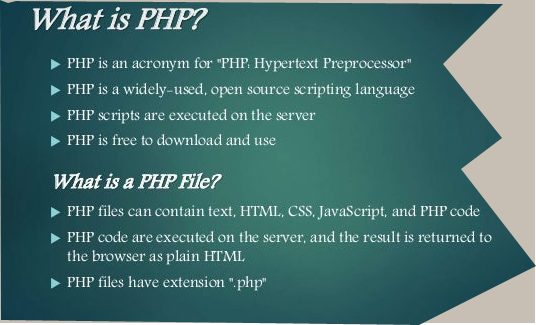
PHP is an open-source, interpreted, and object-oriented scripting language that can be executed at the server-side. PHP is well suited for web development. Therefore, it is used to develop web applications (an application that executes on the server and generates the dynamic page.).
2) What do the initials of PHP stand for?
PHP means PHP: Hypertext Preprocessor.
3) Which programming language does PHP resemble?
PHP syntax resembles Perl and C
4) What does PEAR stand for?
PEAR means “PHP Extension and Application Repository”. It extends PHP and provides a higher level of programming for web developers.
5) What is the actually used PHP version?
Version 7.1 or 7.2 is the recommended version of PHP.
6) How do you execute a PHP script from the command line?
Just use the PHP command line interface (CLI) and specify the file name of the script to be executed as follows:
_______________________________________
php script.php
_____________________________
7) How to run the interactive PHP shell from the command line interface?
Just use the PHP CLI program with the option -a as follows:
____________________________
php -a
_____________________
8) What is the correct and the most two common way to start and finish a PHP block of code?
The two most common ways to start and finish a PHP script are:
__________________________________________________________________________
<?php [ --- PHP code---- ] ?> and <? [--- PHP code ---] ?>
________________________________________________________
9) How can we display the output directly to the browser?
To be able to display the output directly to the browser, we have to use the special tags
10) What is the main difference between PHP 4 and PHP 5?
PHP 5 presents many additional OOP (Object Oriented Programming) features.
11) Is multiple inheritance supported in PHP?
PHP supports only single inheritance; it means that a class can be extended from only one single class using the keyword ‘extended’.
12) How is the comparison of objects done in PHP?
We use the operator ‘==’ to test is two objects are instanced from the same class and have same attributes and equal values. We can test if two objects are referring to the same instance of the same class by the use of the identity operator ‘===’.
13) What is the meaning of a final class and a final method?
‘final’ is introduced in PHP5. Final class means that this class cannot be extended and a final method cannot be overridden.
14) How can PHP and Javascript interact?
PHP and Javascript cannot directly interact since PHP is a server side language and Javascript is a client-side language. However, we can exchange variables since PHP can generate Javascript code to be executed by the browser and it is possible to pass specific variables back to PHP via the URL.
15) What type of operation is needed when passing values through a form or an URL?
If we would like to pass values through a form or an URL, then we need to encode and to decode them using htmlspecialchars() and urlencode().
16) Differentiate between variables and constants in PHP?
Few difference between variables and constants in PHP are given below:
Variables | Constants |
The value of a variable can be changed during the execution. | The constant value can’t be changed during script execution. |
Variables require compulsory usage of the $ sign at the start | No dollar sign ($) is required before using a constant |
It is possible to define a variable by simple assignment. | Constants can’t be defined by simple assignments. They are defined using the define() function. |
The default scope is the current access scope. | Constants can be accessed throughout without any scoping rules. |
17) Explain the difference between $message and $$message?
The main difference between the $message and $$message is given below:
$message | $$message |
$message is a regular variable. | $$message is a reference variable. |
It has a fixed name and stores a fixed value. | It stores data about the variable. |
Data stored in $message is fixed. | The value of the $$message can change dynamically as the value of the variable changes. |
18) Is PHP a case-sensitive language?
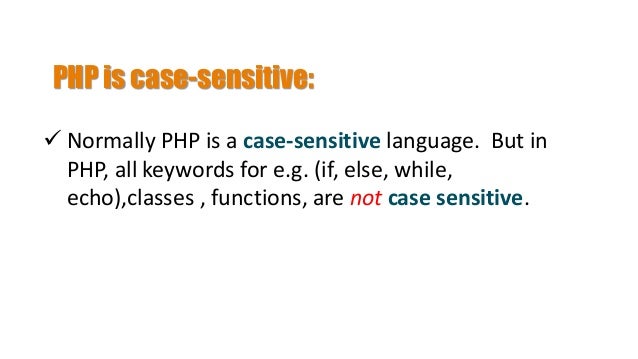
PHP can be considered as a partial case-sensitive language. The variable names are completely case-sensitive but function names are not. Also, user-defined functions are not case-sensitive but the rest of the language is case-sensitive.
For example, user-defined functions in PHP can be defined in lowercase but later referred to in uppercase, and it would still function normally.
19) What are the different types of variables present in PHP?
There are 8 primary data types in PHP which are used to construct the variables. They are:
- Integers: Integers are whole numbers without a floating-point. Ex: 1253.
- Doubles: Doubles are floating-point numbers. Ex: 7.876
- Booleans: It represents two logical states- true or false.
- NULL: NULL is a special type that only has one value, NULL. When no value is assigned to a variable, it can be assigned with NULL.
- Arrays: Array is a named and ordered collection of similar type of data. Ex: $colors = array(“red”, “yellow”, “blue”);
- Strings: Strings are a sequence of characters. Ex: “Hello InterviewBit!”
- Resources: Resources are special variables that consist of references to resources external to PHP(such as database connections).
- Objects: An instance of classes containing data and functions. Ex: $mango = new Fruit();
20) What are the rules for naming a PHP variable?
The following two rules are needed to be followed while naming a PHP variable:
- A variable must start with a dollar symbol, followed by the variable name. For example: $price=100; where price is a variable name.
- Variable names must begin with a letter or underscore.
- A variable name can consist of letters, numbers, or underscores. But you cannot use characters like + , – , % , & etc.
- A PHP variable name cannot contain spaces.
- PHP variables are case-sensitive. So $NAME and $name both are treated as different variables.
21) What is the difference between “echo” and “print” in PHP?
The main difference between echo and print in PHP are given below:
echo | |
echo can output one or more strings. | print can only output one string and it always returns 1. |
echo is faster than print because it does not return any value. | print is slower compared to echo |
If you want to pass more than one parameter to echo, a parenthesis should be used. | Use of parenthesis is not required with the argument list |
22) Tell me some of the disadvantages of PHP?

The cons of PHP are:
- PHP is not suitable for giant content-based web applications.
- Since it is open-source, it is not secure. Because ASCII text files are easily available.
- Change or modification in the core behavior of online applications is not allowed by PHP.
- If we use more features of the PHP framework and tools, it will cause poor performance of online applications.
- PHP features a poor quality of handling errors. PHP lacks debugging tools, which are needed to look for warnings and errors. It has only a few debugging tools in comparison to other programming languages.
23) How can PHP and HTML interact?
PHP scripts have the ability to generate HTML, and it is possible to pass information from HTML to PHP.
PHP is a server-side language whereas HTML is a client-side language. So PHP executes on the server-side and gets its results as strings, objects, arrays, and then we use them to display its values in HTML.
This interaction helps bridge the gaps and use the best of both languages.
24) Explain the importance of Parser in PHP?
A PHP parser is software that converts source code into the code that computer can understand. This means whatever set of instructions we give in the form of PHP code is converted into a machine-readable format by the parser.
You can parse PHP code with PHP using the token get all() function.
25) What are the different types of Array in PHP?
There are 3 main types of arrays that are used in PHP:
Indexed Array
An array with a numeric key is known as the indexed array. Values are stored and accessed in linear order.
Associative Array
An array with strings for indexing elements is known as the associative array. Element values are stored in association with key values rather than in strict linear index order.
Multidimensional Array
An array containing one or more arrays within itself is known as a multidimensional array. The values are accessed using multiple indices.
26) Explain the main types of errors?
The 3 main types of errors in PHP are:
- Notices: Notices are non-critical errors that can occur during the execution of the script. These are not visible to users. Example: Accessing an undefined variable.
- Warnings: These are more critical than notices. Warnings don’t interrupt the script execution. By default, these are visible to the user. Example: include() a file that doesn’t exist.
- Fatal: This is the most critical error type which, when occurs, immediately terminates the execution of the script. Example: Accessing a property of a non-existent object or require() a non-existent file.
27) What are traits?
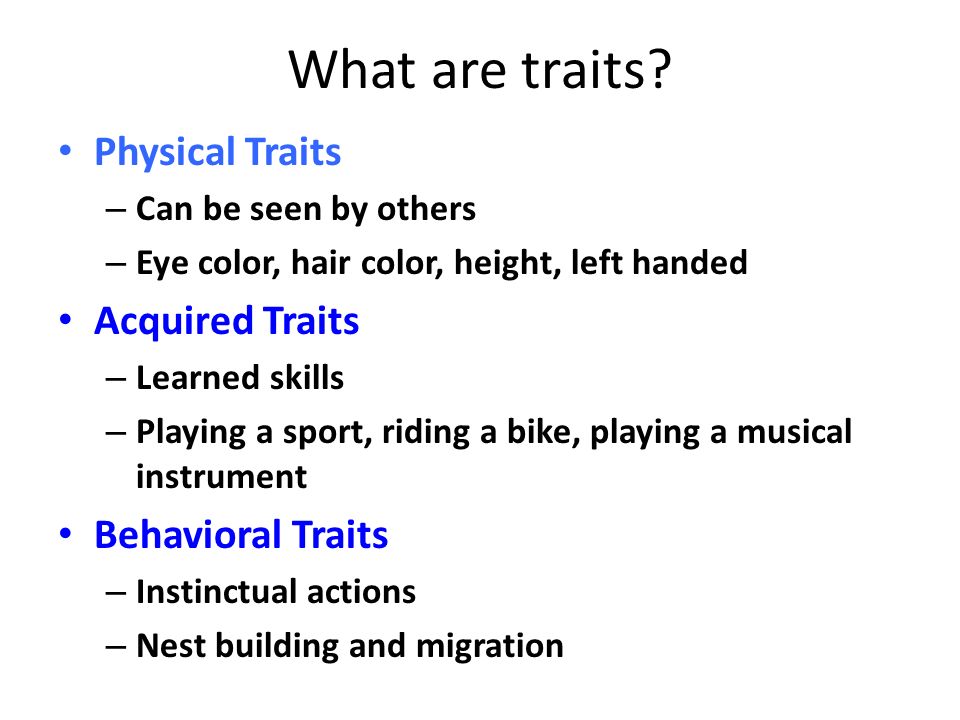
Traits are a mechanism that lets you create reusable code in PHP and similar languages where multiple inheritances are not supported. It’s not possible to instantiate it on its own.
A trait is intended to reduce the limitations of single inheritance by enabling a developer to reuse sets of methods freely in many independent classes living in different hierarchies of class.
28) Does JavaScript interact with PHP?
JavaScript is a client-side programming language, whereas PHP is a server-side scripting language. PHP has the ability to generate JavaScript variables, and this can be executed easily in the browser. Thereby making it possible to pass variables to PHP using a simple URL.
29) How does the ‘foreach’ loop work in PHP?
The foreach statement is a looping construct that is used in PHP to iterate and loop through the array data type.
The working of foreach is simple, with every single pass of the value, elements get assigned a value, and pointers are incremented. This process is repeatedly done until the end of the array has been reached.
The syntax for using the foreach statement in PHP is given below:
_______________________________________________
foreach($array as $value)
{
Code inside the loop;
}
____________________________________
30) What is the most used method for hashing passwords in PHP?
The crypt() function is used for this functionality as it provides a large number of hashing algorithms that can be used. These algorithms include sha1, sha256, or md5 which are designed to be very fast and efficient.
31) What is the difference between the include() and require() functions?
include() function
This function is used to copy all the contents of a file called within the function, text wise into a file from which it is called.
When the file is included cannot be found, it will only produce a warning (E_WARNING) and the script will continue the execution.
require() function:
The require() function performs same as the include() function. It also takes the file that is required and copies the whole code into the file from where the require() function is called.
When the file is included cannot be found, it will produce a fatal error (E_COMPILE_ERROR) and terminates the script.
32) What are cookies? How to create cookies in PHP?
A cookie is a small record that the server installs on the client’s computer. They store data about a user on the browser. It is used to identify a user and is embedded on the user’s computer when they request a particular page. Each time a similar PC asks for a page with a program, it will send the cookie as well.
After verifying the user’s identity in encrypted form, cookies maintain the session id generated at the back end. It must reside in the browser of the machine. You can store only string values not object because you cannot access any object across the website or web apps.
By default, cookies are URL particular. For example, Gmail cookies are not supported by Yahoo and vice versa. Cookies are temporary and transitory by default. Per site 20 cookies can be created in a single website or web app. 50 bytes is the initial size of the cookie and 4096 bytes is the maximum size of the cookie.
In PHP, we can create cookies using the setcookie() function:
setcookie(name, value, expire, path, domain, secure, httponly);
Here name is mandatory and the remaining parameters are optional.
Example:
setcookie(“instrument_selected”, “guitar”)
33) What is the difference between ASP.NET and PHP?
The main difference between ASP.NET and PHP are given below:
ASP.NET | PHP |
A web application framework. | A server-side scripting language. |
It is designed for use on Windows. | It is Platform independent |
Code is compiled and executed. | Interpreted mode of execution |
It has a license cost associated with it | PHP is open-source and freely available. |
34) Why use PHP?
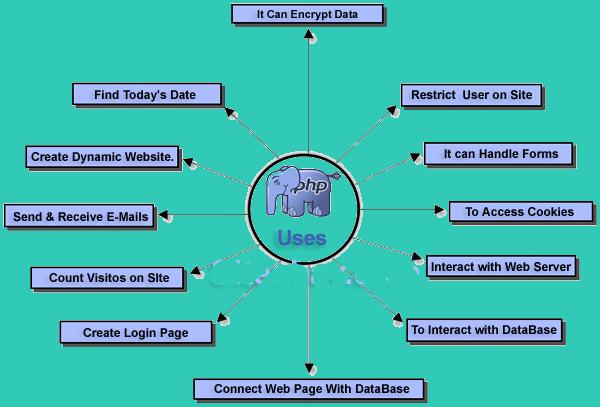
- PHP is a server-side scripting language, which is used to design the dynamic web applications with MySQL database.
- It handles dynamic content, database as well as session tracking for the website.
- You can create sessions in PHP.
- It can access cookies variable and also set cookies.
- It helps to encrypt the data and apply validation.
- PHP supports several protocols such as HTTP, POP3, SNMP, LDAP, IMAP, and many more.
- Using PHP language, you can control the user to access some pages of your website.
- As PHP is easy to install and set up, this is the main reason why PHP is the best language to learn.
- PHP can handle the forms, such as – collect the data from users using forms, save it into the database, and return useful information to the user. For example – Registration form.
35) How can I display text with a PHP script?
Two methods are possible:
_______________________________________________________________
<!--?php echo "Method 1"; print "Method 2"; ?-->
________________________________________________
36) How can we connect to a MySQL database from a PHP script?
To be able to connect to a MySQL database, we must use mysqli_connect() function as follows:
_______________________________________________________________________________________________
<!--?php $database = mysqli_connect("HOST", "USER_NAME", "PASSWORD"); mysqli_select_db($database,"DATABASE_NAME"); ?-->
_________________________________________________________________________
37) How can we access the data sent through the URL with the GET method?
To access the data sent via the GET method, we use $_GET array like this:
________________________________________________________________________
www.url.com?var=value
$variable = $_GET["var"]; this will now contain 'value'
_______________________________________________________
38) How do I escape data before storing it in the database?
The addslashes function enables us to escape data before storage into the database.
39) How is it possible to cast types in PHP?
The name of the output type has to be specified in parentheses before the variable which is to be cast as follows:
- (int), (integer) – cast to integer
- (bool), (boolean) – cast to boolean
- (float), (double), (real) – cast to float
- (string) – cast to string
- (array) – cast to array
- (object) – cast to object
40) Explain whether it is possible to share a single instance of a Memcache between multiple PHP projects?
Yes, it is possible to share a single instance of Memcache between multiple projects. Memcache is a memory store space, and you can run memcache on one or more servers. You can also configure your client to speak to a particular set of instances. So, you can run two different Memcache processes on the same host and yet they are completely independent. Unless, if you have partitioned your data, then it becomes necessary to know from which instance to get the data from or to put into.
41) How to execute a PHP script from the command line?
To execute a PHP script, use the PHP Command Line Interface (CLI) and specify the file name of the script in the following way:
___________________________
php script.php
____________________
42) What is the meaning of ‘escaping to PHP’?
The PHP parsing engine needs a way to differentiate PHP code from other elements in the page. The mechanism for doing so is known as ‘escaping to PHP’. Escaping a string means to reduce ambiguity in quotes used in that string.
43) What are the different types of PHP variables?
There are 8 data types in PHP which are used to construct the variables:
- Integers − are whole numbers, without a decimal point, like 4195.
- Doubles − are floating-point numbers, like 3.14159 or 49.1.
- Booleans − have only two possible values either true or false.
- NULL − is a special type that only has one value: NULL.
- Strings − are sequences of characters, like ‘PHP supports string operations.’
- Arrays − are named and indexed collections of other values.
- Objects − are instances of programmer-defined classes, which can package up both other kinds of values and functions that are specific to the class.
- Resources − are special variables that hold references to resources external to PHP.
44) What is NULL?
NULL is a special data type which can have only one value. A variable of data type NULL is a variable that has no value assigned to it. It can be assigned as follows:
___________________________________________
$var = NULL;
_______________________________
The special constant NULL is capitalized by convention but actually it is case insensitive. So,you can also write it as :
___________________________________
$var = null;
__________________________
A variable that has been assigned the NULL value, consists of the following properties:
- It evaluates to FALSE in a Boolean context.
- It returns FALSE when tested with IsSet() function.
45) How can you compare objects in PHP?
We use the operator ‘==’ to test if two objects are instanced from the same class and have same attributes and equal values. We can also test if two objects are referring to the same instance of the same class by the use of the identity operator ‘===’.
46) Name some of the popular frameworks in PHP?
Some of the popular frameworks in PHP are:
- CakePHP
- CodeIgniter
- Yii 2
- Symfony
- Zend Framework
47) Explain the syntax for ‘foreach’ loop with example?
The foreach statement is used to loop through arrays. For each pass the value of the current array element is assigned to $value and the array pointer is moved by one and in the next pass next element will be processed.
Syntax-
_______________________________________
foreach (array as value)
{
code to be executed;
}
______________________________
Example:
____________________________________________
<?php
$colors = array("blue", "white", "black");
foreach ($colors as $value) {
echo "$value
";
}
?>
___________________________________
48) Name some of the functions in PHP?
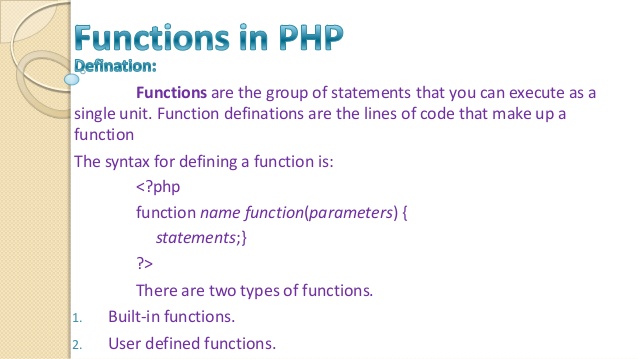
Some of the functions in PHP include:
- ereg() – The ereg() function searches a string specified by string for a string specified by pattern, returning true if the pattern is found, and false otherwise.
- ereg() – The ereg() function searches a string specified by string for a string specified by pattern, returning true if the pattern is found, and false otherwise.
- split() – The split() function will divide a string into various elements, the boundaries of each element based on the occurrence of pattern in string.
- preg_match() – The preg_match() function searches string for pattern, returning true if pattern exists, and false otherwise.
- preg_split() – The preg_split() function operates exactly like split(), except that regular expressions are accepted as input parameters for pattern.
49) What is the use of session and cookies in PHP?
A session is a global variable stored on the server. Each session is assigned a unique id which is used to retrieve stored values. Sessions have the capacity to store relatively large data compared to cookies. The session values are automatically deleted when the browser is closed.
Following example shows how to create a cookie in PHP-
___________________________________________________________________
<?php $cookie_value = "edureka"; setcookie("edureka", $cookie_value, time()+3600, "/your_usename/", "edureka.co", 1, 1); if (isset($_COOKIE['cookie'])) echo $_COOKIE["edureka"]; ?>
_______________________________________________
Following example shows how to start a session in PHP-
_________________________________________________________________
<?php session_start(); if( isset( $_SESSION['counter'] ) ) { $_SESSION['counter'] += 1; }else { $_SESSION['counter'] = 1; } $msg = "You have visited this page". $_SESSION['counter']; $msg .= "in this session."; ?>
__________________________________________________
50) What is GET and POST method in PHP?
The GET method sends the encoded user information appended to the page request. The page and the encoded information are separated by the ? character. For example –
___________________________________________________________
http://www.test.com/index.htm?name1=value1&name2=value2
_____________________________________________
The POST method transfers information via HTTP headers. The information is encoded as described in case of GET method and put into a header called QUERY_STRING.
- Mastering Qualitative Research: The Role of Focus Groups in Data Collection - July 11, 2024
- What is robots ops? - July 10, 2024
- 5 Effective Online Learning Strategies for DevOps Professionals - July 4, 2024