- Explain Rust?
Rust is blazingly fast systems programming language that prevents segfaults and guarantees thread safety.
2. What are the advantages of using rust?
It is a choice of developers. To understand advantages, let’s compare Rust with the similar programming language. However, if you want to get into complete details about Rust programming language, we are also mentioning some of the Rust Programming Language Interview Questions and Answers for all. Go through it and feel like you learnt so many things today.
Rust Vs C++
Rust provides safety where C++ is not even capable enough to provide protection to its own abstraction and even the programmers to protect theirs. If any mistake is committed in C++, it shows arbitrary behaviour – technically, it means it has no meaning. Rust isolates you from that part and lets you concentrate you on the problem that you are trying to solve.
Rust Vs Java.
Automatic garbage collection provides rust with an edge over Java. Java is faster but even it can’t match with the speed of C in some domains.
Note it: Rust programming language can do it easily.
Rust Vs Python:
Good Design gives an edge to Rust over Python. Lambda can’t even hold any statement. In Rust, everything is defined in expressions, which means Language part composes in a much better way.
3. How do you get a command line argument in Rust?
The easiest way to use a command line argument in Rust is to put an iterator over the input arguments. Users can access the command line arguments by using functions such as
_______________________________________
std::env::args_os or std::env::args
______________________________
4. Does Rust include move constructors?
No, the values of all types in Rust are moved via memcpy. It moves everything that doesn’t have a copy constructor or doesn’t implement the copy trait.
5. Does Rust guarantee tail-call optimization?
No, Rust doesn’t guarantee TOC (Tail Call Optimization). Not even the standard library is required to compile the rust code. In these cases, the run time is similar to that of C programming language.
6. Could using Rust be a safer option compared to C and C++?
The most vital advantage of using Rust over C languages is its emphasis on producing safe code. As manage memory or pointer arithmetic is necessary in C programs, Rust doesn’t require any of it beginning to end. Rust allows programmers to write unsafe code, but defaulting to its safe code.
7. What is Cargo in Rust?
It’s a build system and package manager built for Rust users to manager projects in it. The Cargo system manages three things for users, building code, downloading the libraries, and rebuilding those libraries.
8. What’s Cargo.lock in Rust?
When a user runs cargo build command it automatically creates a file named as Cargo.lock to keep track of dependencies in the user application.
9. What’s the relation between Rust and its reusable codes?
Rust allows developers to arrange code in a way that fosters its reuse. By easy organization of modules available in Rust, which contain various structures, function and even other modules which users can use privately or make public according to them.
10. What is “cargo new” purposed for?
The cargo new command is used to create a new project in Rust. Rust users can use below syntax create a sample project using Cargo.
$ cargo new project_name –bin
11. Explain the rule of using &self, self and &mut self in the declaration method?
&self : when Read-only reference is required to the function.
self : When a value is to be consumed by the function.
&mut : When a value needs to be mutated by the function with consuming it.
12. Explain the significance of unwrap() everywhere function in Rust?
This function is used to handle errors that extract the volume inside an option. It’s also extremely useful for instant prototypes with any errors.
13. How to debug Rust programs?
We can use gdb or lldb to debug Rust programs as like C and C++ programming.
14. What are the Error Handling procedures in Rust?
Rust Error Handling is categorized into three parts:
Recoverable Error with Results : If an error occurs, the program doesn’t stop completely. Instead, it can easily be interpreted or responded.
Unrecoverable Errors with Panic : If something wrong goes with the code, Rust’s panic macro comes into action, shows the error message, clean the error and then quit.
Panic or Not to Panic : When you are dicey about calling panic or not, write the code that panics and the process will continue as 2nd.
15. Is it possible to write a complete operating system in Rust?
Yes, it’s possible to write a complete operating system in Rust. Even few of latest released operating system in recent days have used Rust as their primary programming language.
16. How to express platform-specific behaviour in Rust?
The following attributes can be used to express platform-specific behaviour in Rust.
- target_os
- target_family
- target_endian
- And so on
17. Is it possible to cross-compile in Rust?
Yes, it is possible to have cross-compilation in Rust but certain coding is required to do the cross compilation.
18. Explain the significance of deref coercion and its functioning?
It is handy coercion that that is used for automatically converting into the reference to the content from the reference to the pointer.
Some examples of deref coercion are:
- ü &Box to &T
- &String to &str
- ü &Vec to &[T]
- ü &Arc to &T
- ü &Rc to &TW
19. When the first version of Rust was released?
The first version of Rust was released in the year 2010.
20. Rust syntax is similar to which programming Language?
Rust is syntactically similar to C++.
21. List some features of Rust?
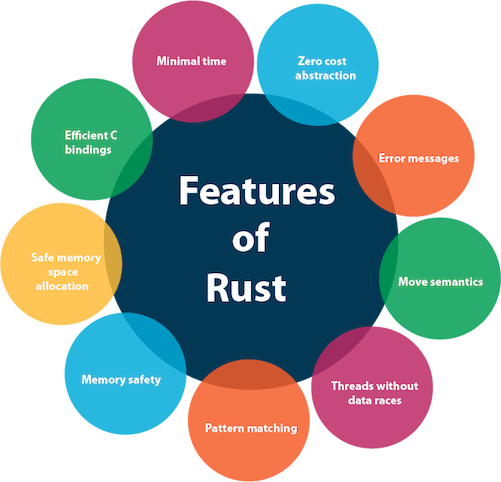
Rust Programming Language comes with following features Sets.
- zero-cost abstractions
- move semantics
- guaranteed memory safety
- threads without data races
- trait-based generics
- pattern matching
- type inference
- minimal runtime
- efficient C bindings
22. Who uses Rust?
Below is list of some reputed companies who use Rust.You can find the complete list from Friends of Rust.
- 360dialog
- OneSignal
- Coursera
- Atlassian
- Braintree
- npm, Inc
- Mozilla
- Academia.edu
- Xero
23. List the Platforms supported by Rust Programming Language?
Linux, Mac, and Windows, on the x86 and x86-64 CPU architecture, are some major platforms supported by Rust Programming Language. For the complete list please visit (https://forge.rust-lang.org/platform-support.html).
24. List steps to install Rust?
On Linux and macOS simply open the terminal and run following commands
$ curl https://sh.rustup.rs -sSf | sh
Above command will download a script, and start the installation process. If everything was good and no error occurred you will see below success message.
Rust is installed now. Great!
If you are on Windows. Installing Rust is very easy just download and run rustup-init.exe File.
25. How to get installed the version of Rust?
rustc –version command is used to get installed version of Rust.
26. How to write and run a Rust Program?
Step to create and run a Rust Program
create a file name main.rs and add following code in it.
_____________________________________________
fn main() {
println!("Hello, Rust!");
}
On Linux or macOS to run open terminal run below command
$ rustc main.rs
$ ./main
__________________________________
27. What Is That Cargo.lock?
When we run cargo build command it creates a new file called Cargo.lock.Cargo uses the Cargo.lock file to keep track of dependencies in your application.
28. What Are The Disadvantages of Rust?
Answer :
- Rust compilation seems slow
- Rust has a moderately-complex type system
- The Rust compiler does not compile with optimizations unless asked to, as optimizations slow down compilation and are usually undesirable during development.
- ü Rust use of LLVM for code generation
29. Does Rust Do Tail-call Optimization?
Answer :
Not, Rust code can be compiled without the standard library; In that case the runtime is roughly equivalent to C programming.
30. What String Type Should You Use?
Answer :
The string types –
- Slice type
- str – UTF-8
- OsStr – OS-compatible
- CStr – C-compatible
- Owned type
- String – UTF-8
- OsString – OS-compatible
- CString – C-compatible
31. What Are The Differences Between The Two Different String Types?
Answer :
- The “String” is an owned buffer of UTF-8 bytes allocated on the heap.
- The “Strings” are Mutable and it can be modified.
- The “&str” is a primitive type and it is implemented by the Rust language while String is implemented in the standard library.
32. How Do I Read A File Into A String?
Answer :
By using the read_to_string() method, which is defined on the Read trait in std::io.
33. What Are The Rules For Using Self, & Self, Or & Mut Self In A Method Declaration?
Answer :
- The “self” is use, when a function needs to consume the value.
- The “& self” is use, when a function only needs a read-only reference to the value.
- The “& mut self” is use, when a function needs to mutate the value without consuming it.
34. How Do I Do Asynchronous Input/output In Rust?
Answer :
There are several libraries providing asynchronous input/output in Rust i.e.
- mio
- tokio
- mioco
- coio-rs
- rotor
- And so on
35. What Is The Deal With Unwrap() Everywhere?
The unwrap() function is use to handle errors that extracts the value inside an Option, if no value is present and It is also useful for quick prototypes where you don’t want to handle an error yet.
36. Does Rust Guarantee A Specific Data Layout?
Not by default, Most of the general case, the enum and struct layouts are undefined.
37. How Do I Write An Opengl App In Rust?
The glium is a library for OpenGL programming in Rust and GLFW is also a solid option.
38. How Can I Write A Gui Application In Rust?
There are different ways to write GUI applications in Rust.
The List of –
- Cocoa
- GTK
- gyscos
- ImGui
- IUP and so on
39. How Do I Cross-compile In Rust?
It is possible but need a bit of work to set up.
40. What Is The Relationship Between A Module And A Crate?
Answer :
Module – A module is a unit of code organization inside a crate.
Crate – A Crate is a compilation unit and it contains an implicit and un-named top-level module.
41. What string type should you use with Rust?
By far, quite a number of string types are available to be used with Rust, choosing one from these, CStr, str, Slice, CString, OsString, OsStr and Owned type, would be more preferable.
42. Could using Rust be a safer option compared to C and C++?
The most vital advantage of using Rust over C languages is its emphasis on producing safe code. As manage memory or pointer arithmetic is necessary in C programs, Rust doesn’t require any of it beginning to end. Rust allows programmers to write unsafe code, but defaulting to its safe code.
43. How does a user asynchronous input/output in Rust?
There are several libraries available providing Rust asynchronous input/output such as tokio, mio, mioco, rotor and coio-rs.
44. How does a user read file input efficiently in Rust?
The following function can be used to read file input efficiency in Rust.
- read()
- read_to_end()
- bytes()
- chars()
- take()
45. How do I get command line arguments in Rust?
The easiest way is to use Args that provides an iterator over the input arguments.
46. Is Rust object oriented?
It is multi paradigm and most of the things can do in Rust but not everything. So, Rust is not object-oriented.
47. Does Rust have a Run-time?
Not! Rust code can be compiled without the standard library; In that case the runtime is roughly equivalent to C programming.
48. How do I do global variables in Rust?
In the Rust, you can globals declarations using const for compile time computed global constants.
Rust currently has limited support for compile time constants and we can define primitives using const declarations.
49. Can I write an operating system in Rust?
Yes! You can do.
50. How do I cross-compile in Rust?
It is possible but need a bit of work to set up.
- Mastering Qualitative Research: The Role of Focus Groups in Data Collection - July 11, 2024
- What is robots ops? - July 10, 2024
- 5 Effective Online Learning Strategies for DevOps Professionals - July 4, 2024