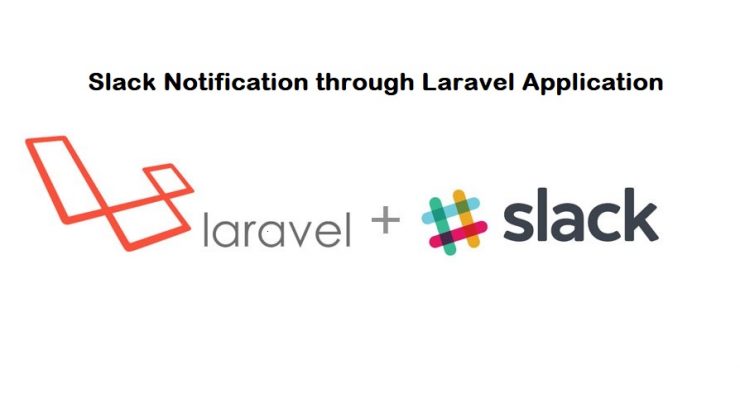
It seems like a topic for basic Laravel developer, but while it’s easy to send emails, but the whole system of various notifications is much deeper, and worth studying. Sub-topics:
In the older version of Laravel, Slack notification was built-in configured with the framework. But on this 5.8.x version, they have created a separate package for Slack Notification. First of all, we have to install that package in our application.
composer require laravel/slack-notification-channel
Configure Incoming Webhooks
First, you need to install the Slack Application, we have to get the Slack webhook URL. For that login, to your Slack application and after login you will land on the main screen now on the left panel
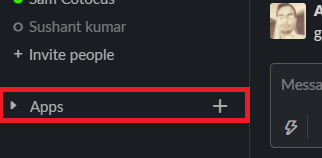
In Your Slack Application, you can see a + sign in the left corner, Add Apps, Click on the icon, you will land on an app search page where you need to search incoming-webhook in the search bar.
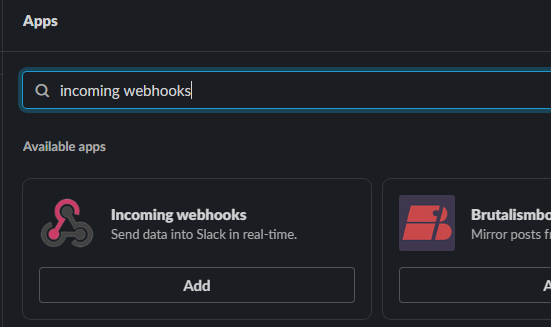
Now install the incoming-webhook application Now go to the setting tab inside the incoming-webhook app which you just install into your slack application. you need to provide the channel name there and you’ll get a Webhook URL.
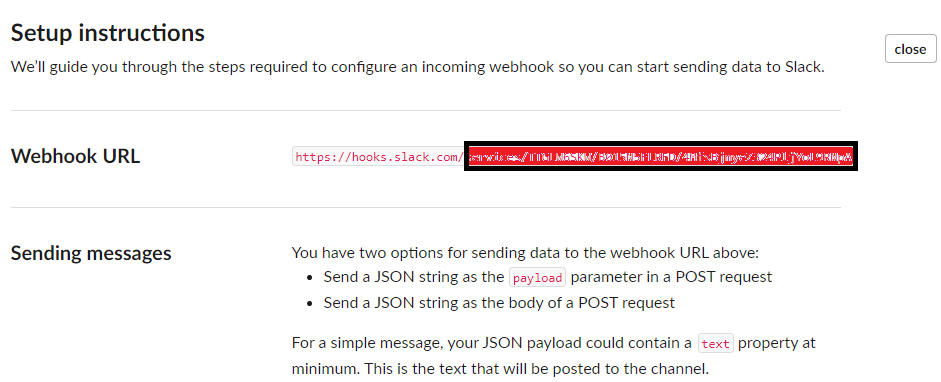
Copy the Webhook URL From the slack website and past it into the very bottom or wherever you like in the .env file which is in your laravel application’s root file
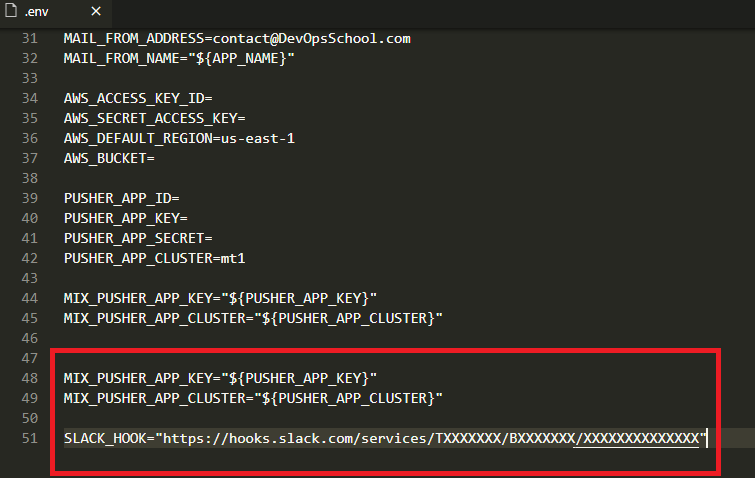
That’s what you need from the Slack app, Now you have to configure your laravel application. For that, you need to create a notification section in laravel. For that, you just need to run this command in your commander.
php artisan make:notification TestNotification
The above command will create a Notifications folder on the app folder from where you can find the TestNotification file. So let’s open and start adding it.
Now add the Slack in a new method’s returned array and create your first slack notification in the new method named toSlack().
public function toSlack($notifiable)
{
$message = "Famous Hello World!";
return (new SlackMessage)
->from('Ghost', ':ghost:')
->to('#channel-name')
->content('Fix service request by '.$message);
}
Now its time to trigger this notification.
Notification::route('slack', env('SLACK_HOOK'))
->notify(new TestNotification());
Complete code for Notification
Paste the above code to send the notification to your slack channel and that’s all.

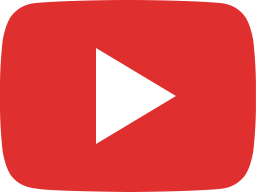

- Laravel – select dropdowns that filter results in a table ‘on change’ - October 30, 2021
- What is Rest and Restful API? Understand the Concept - September 5, 2020
- What is API, clear the Concept. - September 5, 2020