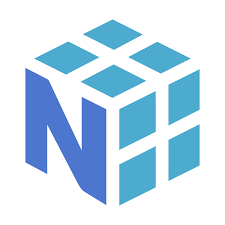
Introduction
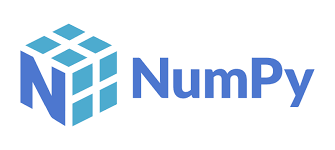
- NumPy is the fundamental package for scientific computing in Python.NumPy arrays facilitate advanced mathematical and other types of operations on large numbers of data. Typically, such operations are executed more efficiently and with less code than is possible using Python’s built-in sequences.
- It is a Python library that provides a multidimensional array object, various derived objects (such as masked arrays and matrices), and an assortment of routines for fast operations on arrays, including mathematical, logical, shape manipulation, sorting, selecting, I/O, discrete Fourier transforms, basic linear algebra, basic statistical operations, random simulation and much more.
let’s start with questions and answers
1. What is NumPy?
Answer: NumPy(Numerical Python) is an open-source library of Python. It is designed to perform complex mathematical, image processing, quantum computing, statistical operations, etc., on matrices and multidimensional arrays. The NumPy N-dimensional arrays are used to perform indexing, slicing, splitting, joining, reshaping, and other complex manipulations.
2. NumPY stands for?
Answer: Numerical Python
3. NumPy is often used along with packages like?
Answer:
- Matplotlib
- SciPy
4. The most important object defined in NumPy is an N-dimensional array type called?
Answer: ndarray
5. Name a few use cases where NumPy is useful.
Answer:
- To perform complex mathematical computations on arrays
- To use multi-dimensional arrays and matrices in operations
- To execute trigonometric, statistical, and algebraic functions
- To execute transforms and methods for shape manipulation
- To generate random numbers
- To add/ delete/ sort/ split arrays
6. How is the seed function used in NumPy?
Answer:
- The seed function sets the seed(provides input) of a pseudo-random number generator in NumPy.
- By pseudo-random we mean that the numbers appear as randomly generated but actually are predetermined through algorithms.
- The numpy.random.seed or np.random.seed function can not be used alone and is used together with other functions.
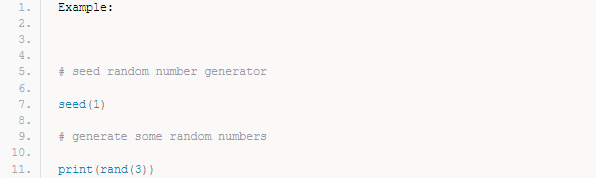
7. Why do we use NumPy?
Answer: NumPy is the fundamental package for scientific computing in Python. It is a Python library that provides:
- a multidimensional array object,
- various derived objects (such as masked arrays and matrices), and
- an assortment of routines for fast operations on arrays, including
*mathematical,
*logical,
*shape manipulation,
*sorting,
*selecting,
*I/O,
*discrete Fourier transforms,
*basic linear algebra,
*basic statistical operations,
*random simulation and much more.
8. What is the difference between ndarray and array in NumPy?
Answer:

9. What are the differences between np.mean() vs np.average() in Python NumPy?
Answer:
- np.mean always computes arithmetic mean, and has some additional options for input and output (e.g. what datatypes to use, where to place the result).
- np.average can compute a weighted average if the weights parameter is supplied.
10. What does NumPy mean in Python?
Answer: NumPy (pronounced /ˈnʌmpaɪ/ (NUM-py) or sometimes /ˈnʌmpi/ (NUM-pee)) is a library for the Python programming language, adding support for large, multi-dimensional arrays and matrices, along with a large collection of high-level mathematical functions to operate on these arrays.
11. Where is NumPy used?
Answer: NumPy is an open-source numerical Python library. NumPy contains a multi-dimensional array and matrix data structures. It can be utilized to perform a number of mathematical operations on arrays such as trigonometric, statistical, and algebraic routines. NumPy is an extension of Numeric and Numarray.
12. How to import numpy in python?
Answer: Import numpy as np
13. How to create 1D Array?
Answer:
num=[1,2,3]
num = np.array(num)
print(“1d array : “,num)
14. How to create a 2D Array?
Answer:
num2=[[1,2,3],[4,5,6]]
num2 = np.array(num2)
print(“\n2d array : “,num2)
15. How to create 3D Array or ND Array?
Answer:
num3=[[[1,2,3],[4,5,6],[7,8,9]]]
num3 = np.array(num3)
print(“\n3d array : “,num3)
16. How to use shape for 1D Array?
Answer:
num=[1,2,3] if not defined
print(‘\nshpae of 1d ‘,num.shape)
17. How to use shape for 2D Array?
Answer:
num2=[[1,2,3],[4,5,6]] if not added
print(‘\nshpae of 2d ‘,num2.shape)
18. How to use shape for 3d or Nd Array?
Answer:
num3=[[[1,2,3],[4,5,6],[7,8,9]]] if not added
print(‘\nshpae of 3d ‘,num3.shape)
19. How to identify datatype for a numpy Array?
Answer:
print(‘\n data type num 1 ‘,num.dtype)
print(‘\n data type num 2 ‘,num2.dtype)
print(‘\n data type num 3 ‘,num3.dtype)
20. Print size of numarray
Answer: print(‘\n size \n’,num.size) #100
21.Use of shape()
Answer: print(‘\n shape \n’,num.shape) # 10,10
22. Why was numpy created?
Answer: NumPy was created to do numerical and scientific computing in the most natural way with Python.
23. How to find the percentile scores of a numpy array?
Answer:
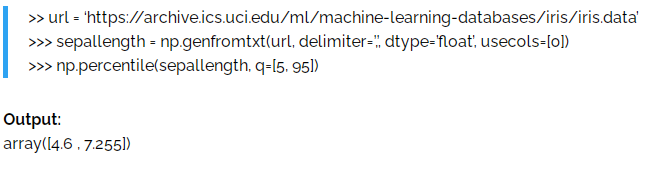
24. What is the use of concatenate()
Answer:
x=np.array([[1,2],[3,4]])
y=np.array([[5,6]])
z=np.concatenate((x,y))
print(“array of z value is : \n”,z)
25. What is the meaning of axis=0 and axis=1?
Answer: axis=0 is used for reading Rows and axix=1 is used for reading Columns.
26. How to reshape an array?
Answer:
arr = np.arange(10)
arr.reshape(2, -1) # Setting to -1 automatically decides the number of cols
Output:
array([[0, 1, 2, 3, 4],
[5, 6, 7, 8, 9]]
27. How to get the common items between two python numpy arrays?
Answer:
a = np.array([1,2,3,2,3,4,3,4,5,6])
b = np.array([7,2,10,2,7,4,9,4,9,8])
np.intersect1d(a,b)
Output:
> array([2, 4])
28. Does Numpy/scipy Work With IronPython (.net)?
Answer: Some users have reported success in using NumPy with Ironclad on 32-bit Windows. The current status of Ironclad support for SciPy is unknown, but there are several complicating factors (namely the Fortran compiler situation on Windows) that make it less feasible than NumPy.
29. Why Not Just Have A Separate Operator For Matrix Multiplication?
Answer: From Python 3.5, the @ symbol will be defined as a matrix multiplication operator, and Numpy and Scipy will make use of this. This addition was the subject of PEP 465. The separate matrix and array types exist to work around the lack of this operator in earlier versions of Python.
30. How Do I Count The Number Of Times Each Value Appears In An Array Of Integers?
Answer:
Use numpy.bincount(). The resulting array is
arr = numpy.array([0, 5, 4, 0, 4, 4, 3, 0, 0, 5, 2, 1, 1, 9])
numpy.bincount(arr)
The argument to bincount() must consist of positive integers or booleans. Negative integers are not supported.
31. What Is The Preferred Way To Check For An Empty (zero Element) Array?
Answer:
If you are certain a variable is an array, then use the size attribute. If the variable may be a list or other sequence type, use len().
The size attribute is preferable to len because:
a = numpy.zeros((1,0))
a.size
0
whereas
len(a)
1
32. Do Numpy And Scipy Support Python 3.x?
Answer: NumPy and SciPy support the Python 2.x series, (versions 2.6 and 2.7), as well as Python 3.2 and newer. The first release of NumPy to support Python 3 was NumPy 1.5.0. Python 3 support in SciPy starts with version 0.9.0.
33. What is the difference between matrix and array?
Answer: Array:-
It is a collection of elements.
Matrix:-
Is the simple row and column and both are different things in different spaces.
Here a collection of single dimension arrays is called a matrix.
34. How to Install Numpy library?
Answer:
Following are the steps to Install the NumPy library on a Windows PC.
- Download & Install the Python executable binaries on your Windows system from Python.org.
- Run the Python executable installer to complete the installation.
- Install pip on your machine.
- Then use the below pip commands to install NumPy Library on your PC.
- Open the command prompt and use the command “pip install numpy”
- This will install the Numpy library on your machine.
35. How to import numpy library in your code?
Answer: When writing your code you will need to import the NumPy library in your program as the first line of code. The syntax to import any library is very simple and it can be done by using the import keyword as given below.
36. What are the pros of Numpy Arrays over Python arrays and lists?
Answer:
- NumPy arrays are an alternative for lists and arrays in Python
Arrays in Numpy are equivalent to lists in python.NumPy arrays can perform some functions that could not be performed when
applied to python arrays due to their heterogeneous nature. - NumPy arrays maintain minimal memory usage as they are treated as objects. Python deletes and creates these objects continually, as per the requirements. Hence, the memory allocation is less as compared to Python lists. NumPy has features to avoid memory wastage in the data buffer.
- NumPy array has support for multi-dimensional arrays. We can also create multi-dimensional arrays in NumPy.These arrays have multiple rows and columns.
- NumPy includes easy-to-use functions for mathematical computations on the array data set. Numpy has many functions and features that make it ideal for Maths operations. There are functions for Linear Algebra, bitwise operations, Fourier transform, arithmetic operations, string operations, etc.
37. Does Numpy Support Nan?
Answer:
nan, short for “not a number”, is a special floating point value defined by the IEEE-754 specification along with inf (infinity) and other values and behaviours. In theory, IEEE nan was specifically designed to address the problem of missing values, but the reality is that different platforms behave differently, making life more difficult. On some platforms, the presence of nanslows calculations 10-100 times. For integer data, no nan value exists. Some platforms, notably older Crays and VAX machines, don’t support nan whatsoever.
Despite all these issues NumPy (and SciPy) endeavor to support IEEE-754 behaviour (based on NumPy’s predecessor numarray). The most significant challenge is a lack of cross-platform support within Python itself. Because NumPy is written to take advantage of C99, which supports IEEE-754, it can side-step such issues internally, but users may still face problems when, for example, comparing values within Python interpreter. In fact, NumPy currently assumes IEEE-754 behavior of the underlying floats, a decision that may have to be revisited when the VAX community rises up in rebellion.
Those wishing to avoid potential headaches will be interested in an alternative solution which has a long history in NumPy’s predecessors – masked arrays. Masked arrays are standard arrays with a second “mask” array of the same shape to indicate whether the value is present or missing. Masked arrays are the domain of the numpy.ma module, and continue the cross-platform Numeric/numarray tradition. See “Cookbook/Matplotlib/Plotting values with masked arrays” (TODO) for example, to avoid plotting missing data in Matplotlib. Despite their additional memory requirement, masked arrays are faster than nans on many floating point units.
38. Print the probabilty arr = [0.23, 0.09, 1.2, 1.24, 9.99] using fix() this example of fuzzy logic or probability
Answer:
arr_num = [0.23, 0.09, 1.2, 1.24, 9.99]
print(“Input probability : “,arr)
out_arr = np.fix(arr_num)
print(“Output probability : “,out_arr)
39.Linear algebra
Answer:
Dot product: product of two arrays
f = np.array([1,2])
g = np.array([4,5])
14+25
np.dot(f, g)
40. What is the use of == operator ?
Answer:
print(‘\n print 2 is equal to num’,num[num==2]) #print [2]
print(‘\n print 0 is equal to num’,num[num==0]) #print [] because empty 0 is not available
41.Mask convert boolean values to number #num = np.arange(1,11)
Answer:
mask = num<4
print(‘print lower than 4 numbers’,num[mask]) #1,2,3
42.Mask convert boolean values to number #num = np.arange(1,11)
Answer:
mask = num>5
print(‘print bigger than 5 numbers’,num[mask]) #5,7,8,9,10
43. Print shape
Answer:
num = np.random.randint(1,50,10)
print(‘\nprint shape befor reshape : ‘,num.shape)
44.Create matrix 2 * 2 with value ranging from 1 to 4
Answer:
arr = np.arange(1,5).reshape(2,2)
print(arr)
45. Print row 1 column 1 output will 5
Answer:
num = np.array([[1,2,3],[4,5,6],[7,8,9]])
print(‘\nprint 5 :’,num[1][1])
46. 1D Slicing with above numpy arra..print[5,15,25,35]
Answer: print(‘\n second upto last : ‘,num[1:])
47. Print first position, last position and 2nd and 3rd position
Answer:
num = np.array([5,15,25,35]) if not added
print(‘\n first position : ‘,num[0]) #5
print(‘\n third position : ‘,num[2]) #25
48.Print Range Between 1 To 100 and show 4 integers random numbers
Answer:
rand_arr3 = np.random.randint(1,100,20)
print(‘\n random number from 1 to 100 ‘,rand_arr3)
49.Use of diag() square matrix ?
Answer:
arr3 = np.diag([1,2,3,4])
print(‘\n square matrix’,arr3)
50.Print 5 zeros?
Answer:
arr = np.zeros(5)
print(‘single arrya’,arr)
- What is Mobile Virtual Network Operator? - April 18, 2024
- What is Solr? - April 17, 2024
- Difference between UBUNTU and UBUNTU PRO - April 17, 2024