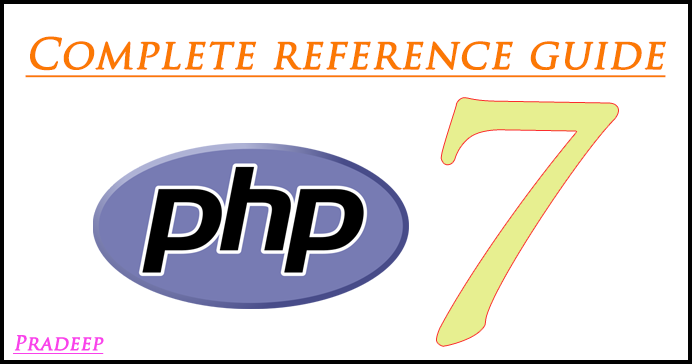
What is Operators in PHP
Operators are symbols that tell the PHP processor to perform certain actions. For example, the addition (+
) symbol is an operator that tells PHP to add two variables or values, while the greater-than (>
) symbol is an operator that tells PHP to compare two values.
The following lists describe the different operators used in PHP.
PHP Arithmetic Operators
The arithmetic operators are used to perform common arithmetical operations, such as addition, subtraction, multiplication etc. Here’s a complete list of PHP’s arithmetic operators:
Operator | Description | Example | Result |
---|---|---|---|
+ | Addition | $x + $y | Sum of $x and $y |
- | Subtraction | $x - $y | Difference of $x and $y. |
* | Multiplication | $x * $y | Product of $x and $y. |
/ | Division | $x / $y | Quotient of $x and $y |
% | Modulus | $x % $y | Remainder of $x divided by $y |
The following example will show you these arithmetic operators in action:
<?php
$x = 10;
$y = 4;
echo($x + $y); // 0utputs: 14
echo($x - $y); // 0utputs: 6
echo($x * $y); // 0utputs: 40
echo($x / $y); // 0utputs: 2.5
echo($x % $y); // 0utputs: 2
?>
PHP Assignment Operators
The assignment operators are used to assign values to variables.
Operator | Description | Example | Is The Same As |
---|---|---|---|
= | Assign | $x = $y | $x = $y |
+= | Add and assign | $x += $y | $x = $x + $y |
-= | Subtract and assign | $x -= $y | $x = $x - $y |
*= | Multiply and assign | $x *= $y | $x = $x * $y |
/= | Divide and assign quotient | $x /= $y | $x = $x / $y |
%= | Divide and assign modulus | $x %= $y | $x = $x % $y |
The following example will show you these assignment operators in action:
<?php
$x = 10;
echo $x; // Outputs: 10
$x = 20;
$x += 30;
echo $x; // Outputs: 50
$x = 50;
$x -= 20;
echo $x; // Outputs: 30
$x = 5;
$x *= 25;
echo $x; // Outputs: 125
$x = 50;
$x /= 10;
echo $x; // Outputs: 5
$x = 100;
$x %= 15;
echo $x; // Outputs: 10
?>
PHP Comparison Operators
The comparison operators are used to compare two values in a Boolean fashion.
Operator | Name | Example | Result |
---|---|---|---|
== | Equal | $x == $y | True if $x is equal to $y |
=== | Identical | $x === $y | True if $x is equal to $y, and they are of the same type |
!= | Not equal | $x != $y | True if $x is not equal to $y |
<> | Not equal | $x <> $y | True if $x is not equal to $y |
!== | Not identical | $x !== $y | True if $x is not equal to $y, or they are not of the same type |
< | Less than | $x < $y | True if $x is less than $y |
> | Greater than | $x > $y | True if $x is greater than $y |
>= | Greater than or equal to | $x >= $y | True if $x is greater than or equal to $y |
<= | Less than or equal to | $x <= $y | True if $x is less than or equal to $y |
The following example will show you these comparison operators in action:
<?php
$x = 25;
$y = 35;
$z = "25";
var_dump($x == $z); // Outputs: boolean true
var_dump($x === $z); // Outputs: boolean false
var_dump($x != $y); // Outputs: boolean true
var_dump($x !== $z); // Outputs: boolean true
var_dump($x < $y); // Outputs: boolean true
var_dump($x > $y); // Outputs: boolean false
var_dump($x <= $y); // Outputs: boolean true
var_dump($x >= $y); // Outputs: boolean false
?>
PHP Incrementing and Decrementing Operators
The increment/decrement operators are used to increment/decrement a variable’s value.
Operator | Name | Effect |
---|---|---|
++$x | Pre-increment | Increments $x by one, then returns $x |
$x++ | Post-increment | Returns $x, then increments $x by one |
--$x | Pre-decrement | Decrements $x by one, then returns $x |
$x-- | Post-decrement | Returns $x, then decrements $x by one |
The following example will show you these increment and decrement operators in action:
<?php
$x = 10;
echo ++$x; // Outputs: 11
echo $x; // Outputs: 11
$x = 10;
echo $x++; // Outputs: 10
echo $x; // Outputs: 11
$x = 10;
echo --$x; // Outputs: 9
echo $x; // Outputs: 9
$x = 10;
echo $x--; // Outputs: 10
echo $x; // Outputs: 9
?>
PHP Logical Operators
The logical operators are typically used to combine conditional statements.
Operator | Name | Example | Result |
---|---|---|---|
and | And | $x and $y | True if both $x and $y are true |
or | Or | $x or $y | True if either $x or $y is true |
xor | Xor | $x xor $y | True if either $x or $y is true, but not both |
&& | And | $x && $y | True if both $x and $y are true |
|| | Or | $x || $y | True if either $$x or $y is true |
! | Not | !$x | True if $x is not true |
The following example will show you these logical operators in action:
<?php
$year = 2014;
// Leap years are divisible by 400 or by 4 but not 100
if(($year % 400 == 0) || (($year % 100 != 0) && ($year % 4 == 0))){
echo "$year is a leap year.";
} else{
echo "$year is not a leap year.";
}
?>
PHP String Operators
There are two operators which are specifically designed for strings.
Operator | Description | Example | Result |
---|---|---|---|
. | Concatenation | $str1 . $str2 | Concatenation of $str1 and $str2 |
.= | Concatenation assignment | $str1 .= $str2 | Appends the $str2 to the $str1 |
The following example will show you these string operators in action:
<?php
$x = "Hello";
$y = " World!";
echo $x . $y; // Outputs: Hello World!
$x .= $y;
echo $x; // Outputs: Hello World!
?>
PHP Array Operators
The array operators are used to compare arrays:
Operator | Name | Example | Result |
---|---|---|---|
+ | Union | $x + $y | Union of $x and $y |
== | Equality | $x == $y | True if $x and $y have the same key/value pairs |
=== | Identity | $x === $y | True if $x and $y have the same key/value pairs in the same order and of the same types |
!= | Inequality | $x != $y | True if $x is not equal to $y |
<> | Inequality | $x <> $y | True if $x is not equal to $y |
!== | Non-identity | $x !== $y | True if $x is not identical to $y |
The following example will show you these array operators in action:
<?php
$x = array("a" => "Red", "b" => "Green", "c" => "Blue");
$y = array("u" => "Yellow", "v" => "Orange", "w" => "Pink");
$z = $x + $y; // Union of $x and $y
var_dump($z);
var_dump($x == $y); // Outputs: boolean false
var_dump($x === $y); // Outputs: boolean false
var_dump($x != $y); // Outputs: boolean true
var_dump($x <> $y); // Outputs: boolean true
var_dump($x !== $y); // Outputs: boolean true
?>
Control Structures .
Control Structures
What is a control structure?
Code execution can be grouped into categories as shown below
- Sequential – this one involves executing all the codes in the order in which they have been written.
- Decision – this one involves making a choice given a number of options. The code executed depends on the value of the condition.
A control structure is a block of code that decides the execution path of a program depending on the value of the set condition.
Let’s now look at some of the control structures that PHP supports.
PHP IF Else
If… then… else is the simplestcontrolstructure. It evaluates the conditions using Boolean logic
When to use if… then… else
- You have a block of code that should be executed only if a certain condition is true
- You have two options, and you have to select one.
- If… then… else if… is used when you have to select more than two options and you have to select one or more
Syntax The syntax for if… then… else is;
<?php
if (condition is true) {
block one
else
block two
}
?>
HERE,
- “if (condition is true)” is the control structure
- “block one” is the code to be executed if the condition is true
- {…else…} is the fallback if the condition is false
- “block two” is the block of code executed if the condition is false
How it works The flow chart shown below illustrates how the if then… else control structure works
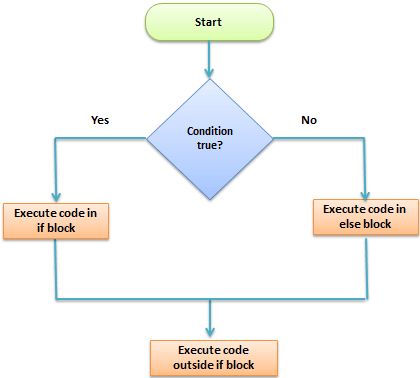
Let’s see this in action The code below uses “if… then… else” to determine the larger value between two numbers.
<?php
$first_number = 7;
$second_number = 21;
if ($first_number > $second_number){
echo "$first_number is greater than $second_number";
}else{
echo "$second_number is greater than $first_number";
}
?>
Output:
21 is greater than 7

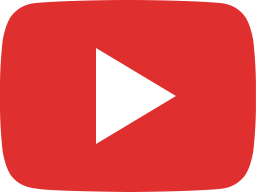
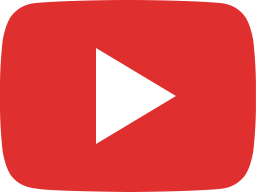

- Laravel – select dropdowns that filter results in a table ‘on change’ - October 30, 2021
- What is Rest and Restful API? Understand the Concept - September 5, 2020
- What is API, clear the Concept. - September 5, 2020