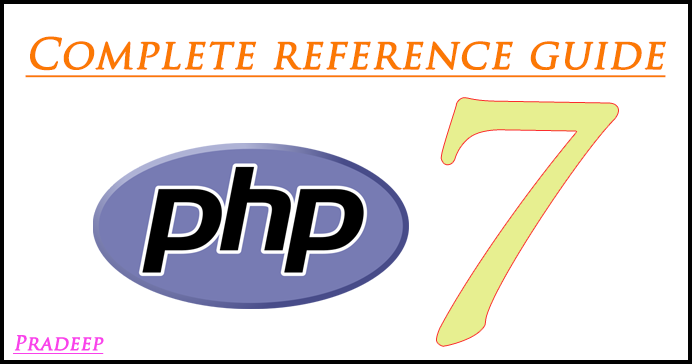
About Web Programming With PHP
This website is designed to serve as a first course in undergraduate web application programming in computer science curriculum. Besides the basic concepts of web applications, the PHP language is also introduced.
In no way this website must be considered as a complete self-learning course. The aim of this website is to help to teach a classroom course. However, it is possible to use it as a guide for self-learning, but with a great effort.
Topics covered include: web application design, web forms, web page templates, PHP basic syntax, dealing with web forms, and access to a database from PHP.
You should expect to spend approximately 30 hours on this course.
What are the Requirements
In order to succeed in this course, you must have taken a course in programming, preferably programming with C, C++ or Java. You must know basic HTML and CSS. Moreover, you must have some background in databases and SQL.
How to Mastering web applications
If you successfully complete this course, this is only the begining. If you really desire to become a web application geek, you should also know:
- HTML5.
- CSS3 and a CSS framework.
- JavaScript and a JavaScript framework, such as JQuery.
- PHP object oriented programming and a PHP framework, such as CodeIgniter.
Server-Side web programming
- Server-side pages are programs written using one of many web programming languages/frameworks examples: PHP, Java/JSP, Ruby on Rails, ASP.NET, Python, Perl
- The web server contains software that allows it to run those programs and send back their output as responses to web requests
- Each language/framework has its pros and cons we use PHP for server-side programming in this textbook .
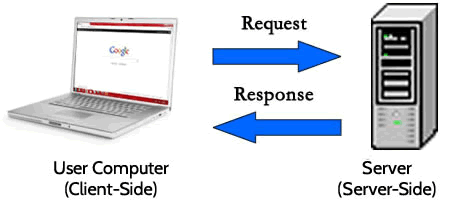
A single PHP file is like using magic on a webpage. In HTML, you have a standard page that is sent to anyone that visits your website. With PHP, you can dynamically change the page based on each individual user. Honestly, learn PHP and put it to the test. You will be extremely impressed with what an open source programming language can accomplish. I am not going to tell you that PHP is the best server side language that is out there, but it is the best for a tight budget or a beginner
What exactly does PHP do?
- It can create custom content based on different variables
- It is excellent at tracking user information
- It can write or read information to databases, if partnered with a database language
- It can run on any type of platform and servers
- It can do anything a standard HTML file can… and much more
How to add Comments
We arrive at the cornerstone of all languages, including PHP, comments. I cannot stress the importance of commenting your code. As a webmaster, I create all kinds of web applications, but more importantly, I maintain the code previously crafted before I arrived. So many times, I have wondered “what the heck were they doing here?”…And after about 10-20 minutes of digesting their code, do I truly understand what they were trying to accomplish. Please comment. Please. With that being said, try to not over comment either. It is fine to do it on every few lines starting out as a beginner. However, when you get more advanced, try to comment large sections (each function and at least every 30 lines) and explain what you are doing in the following code.
Enough of the lecturing, how do I actually comment? Commenting is super easy just like in other languages. In PHP, you can make comments in two ways.
What is Variables
This is PHP, and we want to be dynamic. What’s more dynamic than a variable? Nothing. I know we are all still a little scared of variables from our crazy high school math teachers, but in reality, they are possibly your greatest asset in PHP. However, PHP variables have a few strict rules, which in my opinion is the best way to have them.
What is String Variable
Onto the most flexible variable in PHP, the string variable. You might notice how I keep referring to strings as variables. However, strings are much more like objects with methods and properties. Only the string object’s properties is what you might think of as the string. However, I won’t discuss objects much in the early tutorials because they are a bit more complicated. You can manipulate a string in almost every way imaginable in PHP. Previously you already learned how to create a string variable by putting the actual string in quotes following the variable name.
What are Operators
Somebody bring in the math squad. Relax, how complicated can standard arithmetic operators be? In PHP there are 6 groups of operators: arithmetic, assignment, increment/decrement, comparison, logical, and array operators. We will just deal with the first 3 for now. Quick note: We are going to assume from now on that the code is inside the <?php ?> tags.
Difference Between Include and Require
Have you ever written some HTML code that was exactly the same across multiple pages, like a header or a footer? Your life is about to get so much easier. These awesome creatures known as PHP Server Side Includes will save you so many headaches and keystrokes later.
It is generally good practice to use the include function when writing code, and then later as you review it to replace it with the require function. Based on their overall purpose, they do exactly the same thing. They both “include” a file such as another PHP file that includes a standard header for your website. This is entirely like writing it out manually on each page. However, the difference between the two functions is that the require function stops executing your code as soon as an error occurs. The include function would still continue executing. Running the rest of the code after an error is usually not a good idea, so use require.
What is If Statements
In PHP, if statements are the foundation of programming logic. If statements contain statements that are only executed when the condition is satisfied.
What is Switch Statement
The PHP switch statement is pretty much a simplified way to write multiple if statements for one variable. As you use them, you will begin to realize why they are much more convenient that writing a whole lot of if statements or elseif statements. If statements check one conditional, but switch statements can check for many different cases. The simple syntax of switch statements provide more readable code as opposed to using a lot of else if statements.
What is Functions
PHP Functions are extremely useful, and they can save you from writing bunch of redundant code. These are arguably the most power component to any language. It can separate your code to create a sense of logical flow. You can also add parameters, or arguments to functions, where you can see that power at the bottom of this page. Functions have a limited scope, which I believe is an asset. This means that if you define a variable inside the function, you cannot reference that variable outside of the function.
What is For Loop
PHP for loops are a complicated concept. It is a combination of a statement, a condition, and another statement. Basically, a loop is a repeating if statement.
What is While Loop
PHP while loops are similar to for loops, but with a different structure. Personally, I use for loops much more often than I use while loops. However, while loops have their own powerful uses that you will discover later in your programming life.
What is Break and Continue
While not used very often in PHP, continue and break deserve much more credit. Continue and break provide extra control over your loops by manipulating the flow of the iterations. Continue is the nicer one of the two as it prevents the current iteration, or flow through one pass of the loop, and just tells PHP to go to the next round of the loop. Break provides more aggressive control by completing skipping out of the loop and onto the code following the loop. If you have ever played the card game UNO, continue is basically the skip card, and break is someone slamming their cards down screaming I am the winner and leaves the room. Let’s go through some examples to see how to use them.
What are Arrays
PHP Arrays are like the ultimate variable of PHP. It is also acts as a storage for a collection of elements. Arrays can contain other variables like strings, integers, or even other arrays in a list type format. Arrays are often used whenever you have an unknown amount of variables that you need to store for retrieval later. PHP array values can be outputted by iterating though them using a loop, or you can simply call a specific element by its index or key value. The key value of an array can be an implicit indexing system if you don’t provide the key. You can see more about the keys or indexes later in this tutorial. Arrays an asset to any programmer wanting more dynamic variables. Let’s look on how to construct an array in PHP.
When to use POST and GET
Now, let’s get to the main reason you want to learn a server scripting language. You want to learn how to take user input and do something with it right? PHP POST, GET, and REQUEST are the primary ways to take input from the user. You can use these variables to correlate with form element data or strings in the url. Once PHP receives that input, you can call these variables to get their values to process the input. But first, we should start with how forms work. Submitting a form is really easy,

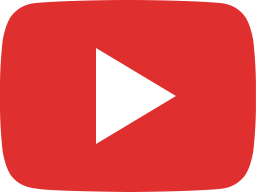
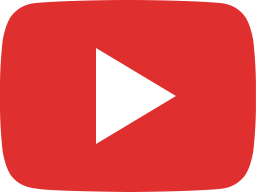

- Laravel – select dropdowns that filter results in a table ‘on change’ - October 30, 2021
- What is Rest and Restful API? Understand the Concept - September 5, 2020
- What is API, clear the Concept. - September 5, 2020