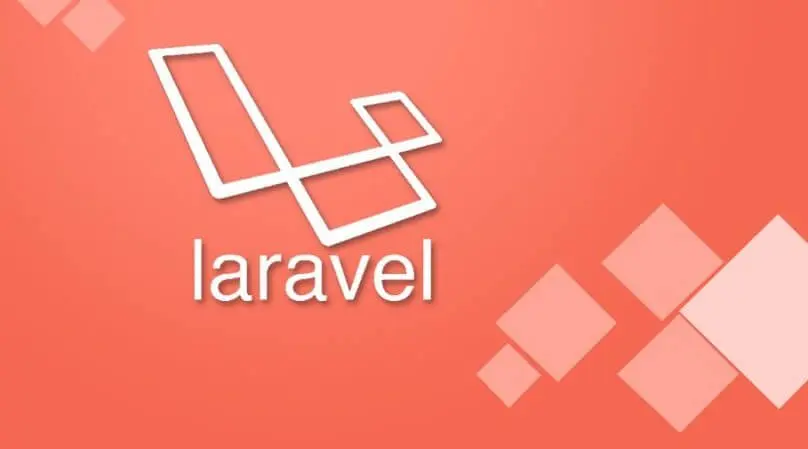
1) What is Laravel?
Laravel is an open-source widely used PHP framework. The platform was intended for the development of web application by using MVC architectural pattern. Laravel is released under the MIT license.
Therefore, its source code is hosted on GitHub. It is a reliable PHP framework as it follows expressive and accurate language rules.
2) What is HTTP middleware?
HTTP middleware is a technique for filtering HTTP requests. Laravel includes a middleware that checks whether application user is authenticated or not.
3) What is a Route?
A route is basically an endpoint specified by a URI (Uniform Resource Identifier). It acts as a pointer in Laravel application.
Most commonly, a route simply points to a method on a controller and also dictates which HTTP methods are able to hit that URI.
4) Why use Route?
Routes are stored inside files under the /routes folder inside the project’s root directory. By default, there are a few different files corresponding to the different “sides” of the application (“sides” comes from the hexagonal architecture methodology).
5) What do you mean by bundles?
In Laravel, bundles are referred to as packages. These packages are used to increase the functionality of Laravel. A package can have views, configuration, migrations, routes, and tasks.
6) Explain reverse routing in Laravel.
Reverse routing is a method of generating URL based on symbol or name. It makes your Laravel application flexible.
7) Explain traits in Laravel.
Laravel traits are a group of functions that you include within another class. A trait is like an abstract class. You cannot instantiate directly, but its methods can be used in concreate class.
8) Explain the concept of contracts in Laravel.
They are set of interfaces of the Laravel framework. These contracts provide core services. Contracts defined in Laravel include corresponding implementation of framework.
10) How will you register service providers?
You can register service providers in the config/app.php configuration file that contains an array where you can mention the service provider class name.
11) What is service container in Laravel?
Service container is a tool used for performing dependency injection in Laravel.
12) Explain the concept of events in Laravel.
An event is an occurrence or action that help you to subscribe and listen for events that occur in Laravel application. Some of the events are fired automatically by Laravel when any activity occurs.
13) Explain dependency injection and their types.
It is a technique in which one object is dependent on another object. There are three types of dependency injection: 1) Constructor injection, 2) setter injection, and 3) interface injection.
14) Explain dependency injection and their types.
It is a technique in which one object is dependent on another object. There are three types of dependency injection: 1) Constructor injection, 2) setter injection, and 3) interface injection.
15) Name databases supported by Laravel.
Laravel supports the following databases:
PostgreSQL
SQL Server
SQLite
MySQL
16) Why are migrations important?
Migrations are important because it allows you to share application by maintaining database consistency. Without migration, it is difficult to share any Laravel application. It also allows you to sync database.
17) How can you generate URLs?
Laravel has helpers to generate URLs. This is helpful when you build links in your templates and API response.
18) Explain fluent query builder in Laravel.
It is a database query builder that provides convenient, faster interface to create and run database queries.
19) What are common HTTP error codes?
The most common HTTP error codes are:
Error 404 – Displays when Page is not found.
Error- 401 – Displays when an error is not authorized
20) What is the use of the dd() function?
This function is used to dump contents of a variable to the browser. The full form of dd is Dump and Die.
21) Does Laravel support caching?
Yes, Laravel supports caching of popular backends such as Memcached and Redis.
22) Why laravel is the best PHP framework in 2021?
Easy Installation
Supports MVC Architecture
Ensures high security
Modular Design
Object-Oriented Libraries
23) How to make a constant and use globally?
You can create a constants.php page in config folder if does not exist. Now you can put constant variable with value here and can use with Config::get(‘constants.VaribleName’);
Example
return [
‘ADMINEMAIL’ => ‘info@bestinterviewquestion.com’,
];
Now we can display with
Config::get(‘constants.ADMINEMAIL’);
24) How to make a helper file in laravel?
We can create a helper file using Composer. Steps are given below:-
Please create a “app/helpers.php” file that is in app folder.
Add
“files”: [
“app/helpers.php”
]
in “autoload” variable.
Now update your composer.json with composer dump-autoload or composer update.
25) What is Service container?
A laravel service container is one of the most powerful tools that have been used to manage dependencies over the class and perform dependency injections.
Advantages of Service Container
Freedom to manage class dependencies on object creation.
Service contain as Registry.
Ability to bind interfaces to concrete classes.
26) How to use cookies in laravel?
- How to set Cookie
To set cookie value, we have to use Cookie::put(‘key’, ‘value’); - How to get Cookie
To get cookie Value we have to use Cookie::get(‘key’); - How to delete or remove Cookie
To remove cookie Value we have to use Cookie::forget(‘key’) - How to check Cookie
To Check cookie is exists or not, we have to use Cache::has(‘key’).
27) What is Auth? How is it used?
Laravel Auth is the process of identifying the user credentials with the database. Laravel managed it’s with the help of sessions which take input parameters like username and password, for user identification. If the settings match then the user is said to be authenticated.
Auth is in-built functionality provided by Laravel; we have to configure.
We can add this functionality with php artisan make: auth
Auth is used to identifying the user credentials with the database.
28) What is with() in Laravel?
with() function is used to eager load in Laravel. Unless of using 2 or more separate queries to fetch data from the database, we can use it with() method after the first command. It provides a better user experience as we do not have to wait for a longer period of time in fetching data from the database.
29) What is composer lock in laravel?
After running the composer install in the project directory, the composer will generate the composer.lock file.It will keep a record of all the dependencies and sub-dependencies which is being installed by the composer.json.
30) Which template engine laravel use?
Laravel uses “Blade Template Engine”. It is a straightforward and powerful templating engine that is provided with Laravel.
31) How to use aggregate functions in Laravel?
Laravel provides a variety of aggregate functions such as max, min, count,avg, and sum. We can call any of these functions after constructing our query.
$users = DB::table(‘admin’)->count();
$maxComment = DB::table(‘blogs’)->max(‘comments’);
32) How to use aggregate functions in Laravel?
Laravel provides a variety of aggregate functions such as max, min, count,avg, and sum. We can call any of these functions after constructing our query.
$users = DB::table(‘admin’)->count();
$maxComment = DB::table(‘blogs’)->max(‘comments’);
33) What is Vapor in Laravel?
Vapor in Laravel is a serverless deployment platform auto-scaling and powered by AWS Lambda. It used to manage Laravel Infrastructure with the scalability and simplicity of serverless.
34) What is Singleton design pattern in laravel?
The Singleton Design Pattern in Laravel is one where a class presents a single instance of itself. It is used to restrict the instantiation of a class to a single object. This is useful when only one instance is required across the system. When used properly, the first call shall instantiate the object and after that, all calls shall be returned to the same instantiated object.
35) What is the use of the cursor() method in Laravel?
The Eloquent cursor () method allows a user to iterate through the database records using a cursor, which will execute only a single query. This method is quite useful when processing large amounts of data to significantly reduce memory usage.
36) What is a REPL?
REPL is a type of interactive shell that takes in single user inputs, process them, and returns the result to the client.
The full form of REPL is Read—Eval—Print—Loop
37) What is eager loading in Laravel?
Eager loading is used when we have to fetch some useful data along with the data which we want from the database. We can eager load in laravel using the load() and with() commands.
18) How to know laravel version?
You can use an artisan command php artisan –version to know the laravel version.
39) What is Blade laravel?
Blade is very simple and powerful templating engine that is provided with Laravel. Laravel uses “Blade Template Engine”.
40) What is Horizon in Laravel 5?
Horizon in Laravel is the queue manager. It provides the user with full control of the queues, it renders the means for configuring how the jobs are processed and generates analytics, plus performs various tasks related to queue from within one nice dashboard.
41) What is the minimum compatible version of PHP for Laravel 7 and 8?
The minimum compatible PHP version for Laravel 7 is PHP 7.2.5 and for Laravel 8 is PHP 7.3.0
42) What is the command you can use to check whether you have installed the composer on your computer?
You can run the following command in the command prompt to check whether you have successfully installed the composer on your computer.
43) What are bundles in Laravel?
Bundles are used to increase the functionality of Laravel. In Laravel, bundles are popularly known as packages. It contains configuration, routes, migrations, views, etc
44) What are the two main routing files found in Laravel?
The two main routing files are:
web.php file in the routes folder.
api.php file in the routes folder.
45) How to identify a blade template file?
Usually, all blade template files reside inside the resources/views folder. Blade files have .blade.php extension.
46) State the location where model files reside in a typical Laravel application?
There is a difference in the location where model files are stored in a Laravel 7 application and a Laravel 8 application.
In a Laravel 7 application, usually, all model files reside inside the app folder.
In a Laravel 8 application usually, all model files reside inside the app/Models folder.
47) What is seeding?
Developers need test data when developing an application. Seeding is the insertion of data to the database for testing purposes.
48) What are the databases supported by the Laravel framework?
The following list below shows the supported databases:
MySQL 5.6+
PostgreSQL (Postgres) 9.4+
SQLite 3.8.8+
SQL Server 2017+
49) What is authorization?
Authorization is the process of verifying whether authenticated users have the required permission to access the requested resources. Laravel uses gates for the authorization process.
50) What is Laravel Forge?
It is a server management tool for PHP applications. It is a great alternative if you are not planning to manage your own servers.
51) What is Laravel Vapor?
It is a completely serverless deployment platform. It is powered by Amazon Web Services (AWS).
52) What are the common tools used to send emails in Laravel?
The following list below shows some common tools that can be used to send emails in Laravel.
Mailtrap
Mailgun
Mailchimp
Mandrill
Amazon Simple Email Service (SES)
Swiftmailer
Postmark
53) Explain validations in laravel?
In Programming validations are a handy way to ensure that your data is always in a clean and expected format before it gets into your database.
Laravel provides several different ways to validate your application incoming data.By default Laravel’s base controller class uses a ValidatesRequests trait which provides a convenient method to validate all incoming HTTP requests coming from client.You can also validate data in laravel by creating Form Request.
Laravel validation Example
$validatedData = $request->validate([
‘name’ => ‘required|max:255’,
‘username’ => ‘required|alpha_num’,
‘age’ => ‘required|numeric’,
]);
54) How to install laravel via composer ?
The installation of Laravel via composer is very easy. You can install Laravel via composer by running the below command in the command prompt.
composer create-project laravel/laravel your-project-name version.
55) What is database migration. How to create migration via artisan ?
Migrations are like version control for your database, that’s allow your team to easily modify and share the application’s database schema. Migrations are typically paired with Laravel’s schema builder to easily build your application’s database schema.
Use below commands to create migration data via artisan.
// creating Migration
php artisan make:migration create_users_table
56) What are service providers in Laravel?
Service Providers are central place where all laravel application is bootstrapped . Your application as well all Laravel core services are also bootstrapped by service providers.
All service providers extend the Illuminate\Support\ServiceProvider class. Most service providers contain a register and a boot method. Within the register method, you should only bind things into the service container. You should never attempt to register any event listeners, routes, or any other piece of functionality within the register method.
57) Explain Laravel’s service container?
One of the most powerful feature of Laravel is its Service Container. It is a powerful tool for resolving class dependencies and performing dependency injection in Laravel.
Dependency injection is a fancy phrase that essentially means class dependencies are “injected” into the class via the constructor or, in some cases, “setter” methods.
58) How to enable query log in Laravel?
Use the enableQueryLog method to enable query log in Laravel
DB::connection()->enableQueryLog();
You can get array of the executed queries by using getQueryLog method:
$queries = DB::getQueryLog();
59) How to use custom table in Laravel Modal?
You can use custom table in Laravel by overriding protected $table property of Eloquent.
Below is sample uses
class User extends Eloquent{
protected $table=”my_user_table”;
}
60) List types of relationships available in Laravel Eloquent?
Below are types of relationships supported by Laravel Eloquent ORM.
One To One
One To Many
One To Many (Inverse)
Many To Many
Has Many Through
Polymorphic Relations
Many To Many Polymorphic Relations
61) How to clear cache in Laravel?
The syntax to clear cache in Laravel is given below:
php artisan cache: clear
php artisan config: clear
php artisan cache: clear
62) What are the validations in Laravel?
Validations are approaches that Laravel use to validate the incoming data within the application.
They are the handy way to ensure that data is in a clean and expected format before it gets entered into the database. Laravel consists of several different ways to validate the incoming data of the application. By default, the base controller class of Laravel uses a ValidatesRequests trait to validate all the incoming HTTP requests with the help of powerful validation rules.
63) What do you understand by Unit testing?
Unit testing is built-in testing provided as an integral part of Laravel. It consists of unit tests which detect and prevent regressions in the framework. Unit tests can be run through the available artisan command-line utility.
64) Explain the Service container and its advantages.
Service container in Laravel is one of the most powerful features. It is an important, powerful tool for resolving class dependencies and performing dependency injection in Laravel. It is also known as IoC container.
Dependency injection is a term which essentially means that class dependencies are “injected” into the class by the constructor or, in some cases,” setter” methods.
Advantages of Service Container
It can handle class dependencies on object creation.
It can combine interfaces to concrete classes.
65) What is the use of PHP compact function?
PHP compact function receives each key and tries to search a variable with that same name. If a variable is found, then it builds an associate array.
66) What do you understand by ORM?
ORM stands for Object-Relational Mapping. It is a programming technique which is used to convert data between incompatible type systems in object-oriented programming languages.
67) How can someone change the default database type in Laravel?
Laravel is configured to use MySQL by default.
To change its default database type, edit the file config/database.php:
Search for ‘default’ =>env(‘DB_CONNECTION’, ‘mysql’)
Change it to whatever required like ‘default’ =>env(‘DB_CONNECTION’, ‘sqlite’)
By using it, MySQL changes to SQLite.
68) In which directory controllers are kept in Laravel?
Controllers are kept in app/http/Controllers directory.
69) How will you explain homestead in Laravel?
Homestead is an official, pre-packaged, vagrant virtual machine which provides Laravel developers all the necessary tools to develop Laravel out of the box. It also includes Ubuntu, Gulp, Bower, and other development tools which are useful in developing full-scale web applications. It provides a development environment which can be used without the additional need to install PHP, a web server, or any other server software on the machine.
70) What do you know about Closures in Laravel?
In Laravel, a Closure is an anonymous method which can be used as a callback function. It can also be used as a parameter in a function. It is possible to pass parameters into a Closure. It can be done by changing the Closure function call in the handle() method to provide parameters to it. A Closure can access the variables outside the scope of the variable.
Example
function handle(Closure $closure) {
$closure();
}
handle(function(){
echo ‘Interview Question’;
});
It is started by adding a Closure parameter to the handle() method. We can call the handle() method and pass a service as a parameter.
71) How can we check the logged-in user info in Laravel?
User() function is used to get the logged-in user
Example
if(Auth::check()){
$loggedIn_user=Auth::User();
dd($loggedIn_user);
}
72) How will you describe Fillable Attribute in a Laravel model?
In eloquent ORM, $fillable attribute is an array containing all those fields of table which can be filled using mass-assignment.
Mass assignment refers to sending an array to the model to directly create a new record in Database.
Code Source
class User extends Model {
protected $fillable = [‘name’, ’email’, ‘mobile’];
// All fields inside $fillable array can be mass-assigned
}
73) How can we create a record in Laravel using eloquent?
We need to create a new model instance if we want to create a new record in the database using Laravel eloquent. Then we are required to set attributes on the model and call the save() method.
Example
public functionsaveProduct(Request $request )
$product = new product;
$product->name = $request->name;
$product->description = $request->name;
$product->save();
74) How can we implement a package in Laravel?
We can implement a package in Laravel by:
Creating a package folder and name it.
Creating Composer.json file for the package.
Loading package through main composer.json and PSR-4.
Creating a Service Provider.
Creating a Controller for the package.
Creating a Routes.php file.
75) What are the requirements for Laravel 5.8?
PHP Version>=7.1.3
OpenSSL PHP Extension
PDO PHP Extension
Mbstring PHP Extension
Tokenizer PHP Extension
XML PHP Extension
Ctype PHP Extension
JSON PHP Extension
76) How will you create a helper file in Laravel?
We can create a helper file using composer as per the given below steps:
Make a file “app/helpers.php” within the app folder.
Add
“files”: [
“app/helpers.php”
]
in the “autoload” variable.
Now update composer.json with composer dump-autoload or composer update.
77) How can we get the user’s IP address in Laravel?
We can get the user’s IP address using:
public function getUserIp(Request $request){
// Gettingip address of remote user
return $user_ip_address=$request->ip();
}
78) How can we check the Laravel current version?
One can easily check the current version of Laravel installation using the -version option of artisan command.
Php artisan -version
79) What do you know about CSRF token in Laravel? How can someone turn off CSRF protection for a specific route?
CSRF protection stands for Cross-Site Request Forgery protection. CSRF detects unauthorized attacks on web applications by the unauthorized users of a system. The built-in CSRF plug-in is used to create CSRF tokens so that it can verify all the operations and requests sent by an active authenticated user.
To turn off CSRF protection for a specific route, we can add that specific URL or Route in $except variable which is present in the app\Http\Middleware\VerifyCsrfToken.phpfile.
Example
classVerifyCsrfToken extends BaseVerifier
{
protected $except = [
‘Pass here your URL’,
];
}
80) How can we get data between two dates using Query in Laravel?
We can use whereBetween() method to retrieve the data between two dates with Query.
Example
Blog::whereBetween(‘created_at’, [$date1, $date2])->get();
81) What do you understand by database migrations in Laravel? How can we use it?
Migrations can be defined as version control for the database, which allows us to modify and share the application’s database schema easily. Migrations are commonly paired with Laravel’s schema builder to build the application’s database schema easily.
A migration file includes two methods, up() and down(). A method up() is used to add new tables, columns or indexes database and the down() method is used to reverse the operations performed by the up() method.
We can generate a migration and its file by using the make:migration.
Syntax
php artisan make:migration blog
By using it, a current date blog.php file will be created in database/migrations.
82) What are some benefits of Laravel over other Php frameworks?
Setup and customisation process is easy and fast as compared to others.
Inbuilt Authentication System
Supports multiple file systems
Pre-loaded packages like Laravel Socialite, Laravel cashier, Laravel elixir, Passport, Laravel Scout
Eloquent ORM (Object Relation Mapping) with PHP active record implementation
Built in command line tool “Artisan” for creating a code skeleton ,database structure and build their migration.
83) Explain Migrations in Laravel.
Laravel Migrations are like version control for the database, allowing a team to easily modify and share the application’s database schema. Migrations are typically paired with Laravel’s schema builder to easily build the application’s database schema.
84)What are Laravel events?
Laravel event provides a simple observer pattern implementation, that allow to subscribe and listen for events in the application. An event is an incident or occurrence detected and handled by the program.
Below are some events examples in Laravel:
A new user has registered
A new comment is posted
User login/logout
New product is added.
85) What is Eloquent Models?
The Eloquent ORM included with Laravel provides a beautiful, simple ActiveRecord implementation for working with your database. Each database table has a corresponding Model which is used to interact with that table. Models allow you to query for data in your tables, as well as insert new records into the table.
86) Does PHP support method overloading?
Method overloading is the phenomenon of using same method name with different signature. You cannot overload PHP functions. Function signatures are based only on their names and do not include argument lists, so you cannot have two functions with the same name.
You can, however, declare a variadic function that takes in a variable number of arguments. You would use func_num_args() and func_get_arg() to get the arguments passed, and use them normally.
function myFunc() {
for ($i = 0; $i < func_num_args(); $i++) {
printf(“Argument %d: %s\n”, $i, func_get_arg($i));
}
}
/*
Argument 0: a
Argument 1: 2
Argument 2: 3.5
*/
myFunc(‘a’, 2, 3.5);
87) How do you do soft deletes?
Scopes allow you to easily re-use query logic in your models. To define a scope, simply prefix a model method with scope:
class User extends Model {
public function scopePopular($query)
{
return $query->where(‘votes’, ‘>’, 100);
}
public function scopeWomen($query)
{
return $query->whereGender('W');
}
}
Usage:
$users = User::popular()->women()->orderBy(‘created_at’)->get();
Sometimes you may wish to define a scope that accepts parameters. Dynamic scopes accept query parameters:
class User extends Model {
public function scopeOfType($query, $type)
{
return $query->whereType($type);
}
}
Usage:
$users = User::ofType(‘member’)->get();
88) How do you generate migrations?
Migrations are like version control for your database, allowing your team to easily modify and share the application’s database schema. Migrations are typically paired with Laravel’s schema builder to easily build your application’s database schema.
To create a migration, use the make:migration Artisan command:
php artisan make:migration create_users_table
The new migration will be placed in your database/migrations directory. Each migration file name contains a timestamp which allows Laravel to determine the order of the migrations.
87) How do you mock a static facade methods?
Facades provide a “static” interface to classes that are available in the application’s service container. Unlike traditional static method calls, facades may be mocked. We can mock the call to the static facade method by using the shouldReceive method, which will return an instance of a Mockery mock.
// actual code
$value = Cache::get(‘key’);
// testing
Cache::shouldReceive(‘get’)
->once()
->with(‘key’)
->andReturn(‘value’);
88) List some Aggregates methods provided by query builder in Laravel?
Aggregate function is a function where the values of multiple rows are grouped together as input on certain criteria to form a single value of more significant meaning or measurements such as a set, a bag or a list.
Below is list of some Aggregates methods provided by Laravel query builder:
count()
$products = DB::table(‘products’)->count();
max()
$price = DB::table(‘orders’)->max(‘price’);
min()
$price = DB::table(‘orders’)->min(‘price’);
avg()
*$price = DB::table(‘orders’)->avg(‘price’);
sum()
$price = DB::table(‘orders’)->sum(‘price’);
89) What are named routes in Laravel?
Named routes allow referring to routes when generating redirects or Url’s more comfortably. You can specify named routes by chaining the name method onto the route definition:
Route::get(‘user/profile’, function () {
//
})->name(‘profile’);
You can specify route names for controller actions:
Route::get(‘user/profile’, ‘UserController@showProfile’)->name(‘profile’);
Once you have assigned a name to your routes, you may use the route’s name when generating URLs or redirects via the global route function:
// Generating URLs…
$url = route(‘profile’);
// Generating Redirects…
return redirect()->route(‘profile’);
90) What is Closure in Laravel?
A Closure is an anonymous function. Closures are often used as callback methods and can be used as a parameter in a function.
function handle(Closure $closure) {
$closure(‘Hello World!’);
}
handle(function($value){
echo $value;
});
91) What is autoloading classes in PHP?
With autoloaders, PHP allows the last chance to load the class or interface before it fails with an error.
The spl_autoload_register() function in PHP can register any number of autoloaders, enable classes and interfaces to autoload even if they are undefined.
spl_autoload_register(function ($classname) {
include $classname . ‘.php’;
});
$object = new Class1();
$object2 = new Class2();
In the above example we do not need to include Class1.php and Class2.php. The spl_autoload_register() function will automatically load Class1.php and Class2.php.
92) What is the benefit of eager loading, when do you use it?
When accessing Eloquent relationships as properties, the relationship data is “lazy loaded”. This means the relationship data is not actually loaded until you first access the property. However, Eloquent can “eager load” relationships at the time you query the parent model.
Eager loading alleviates the N + 1 query problem when we have nested objects (like books -> author). We can use eager loading to reduce this operation to just 2 queries.
.
93) What is CSRF protection and CSRF token?
First let’s understand CSRF – It’s cross-site request forgery attack. Cross-site forgeries are a type of malicious exploit where unauthorized commands can be executed on behalf of the authenticated user. Laravel generates a CSRF “token” automatically for each active user session. This token is used to verify that the authenticated user is the one actually making the requests to the application. @csrf …
If you’re defining an HTML form in your application you should include a hidden CSRF token field in the form so that middleware (CSRF Protection middleware) can validate the request. VerifyCsrfToken middleware is included in web middleware group. @csrf blade directive can be used to generate the token field.
If using JS driven application then you can attach your js HTTP library automatically attach the CSRF token with every HTTP request. resources/js/bootstrap.js is the default file which registers value of csrf-token meta tag with HTTP library.
To remove URIs from CSRF in verifyCsrfToken we can use $except property where can provide URLs which we want to exclude.
94) What are the pros and cons of Laravel?
The following are the pros and cons of the Laravel framework.
Pros of Laravel:
For managing the project dependencies, Laravel uses the Composer allowing developers to mention the package name, version, and the pre-built functionalities that are ready for use in your project, resulting in faster development of the application
It comes with a blade templating engine that is easy to learn and understand. This is useful while working with the PHP/HTML languages. Web development via Laravel allows the composing of the plain PHP codes in a layout shape, thus helping in improving the execution of complex tasks.
You can learn Laravel via Laracast that comes with both free and paid videos for easy learning
Laravel has an object-relational mapper named Eloquent that helps implement the active record pattern and interact with the relational database. It is available as a package for Laravel.
Laravel has a built-in command-line tool called Artisan support, where users can carry out repetitive tasks quickly and effortlessly
With the help of the Laravel schema, developers can create database tables and add desired columns via writing a simple migration script
It comes with a reverse routing feature that makes your application more flexible
Cons of Laravel:
It is challenging to migrate legacy system to Laravel
It comes with heavy documentation that might be difficult to understand for beginners
Upgrades aren’t smooth.
95) How do you install Laravel via composer?
The composer comes as a dependency manager. If it is not installed on your system, you can install it using this link.
After successfully installing the composer on your system, you need to create a project directory for your Laravel project. Later, you need to navigate the path where you have created the Laravel directory and run the following command:
composer create-project laravel/laravel –prefer-dist
This command will help install the Laravel in the current directory. If you want to start Laravel, run the following command.
php artisan serve
Laravel will get started on the development server. Now, run http://localhost:8000/ on your browser. The server requirement is as follows for installing Laravel.
PHP >= 7.1.3.
OpenSSL PHP Extension
PDO PHP Extension
Mbstring PHP Extension
Tokenizer PHP Extension
XML PHP Extension
Ctype PHP Extension
JSON PHP Extension
96) What template is used by the Laravel engine?
Laravel comes with the Blade templating engine, which helps users use the plain PHP code in the view and then compiles and caches that view until the next modification. You can get the files related to the blade views in the resources/views directory with the extension .blade.php.
Below is an example of the Blade file. {{ $slot }}
In the variable $slot, you can assign any desired value to inject into the component.
The Component will look as follows:
@component(‘alert’)
Whoops! Something went wrong!
@endcomponent
@component is the blade directive here.
97) What are the features included in the latest version of Laravel?
The latest stable version of Laravel is Laravel 8. Here are some good features of Laravel 8:
Laravel Jetstream
Models directory
Model factory classes
Migration squashing
Time testing helpers
Dynamic blade components
Rate limiting improvements
98) How can you check the installed version of Laravel?
Firstly, open the command line terminal and navigate to the project directory. Next, execute any of the following commands to check the installed version of Laravel.
php artisan –version
or
php artisan -v
90) How can you create a route in Laravel?
If you want to create a route in Laravel, use controllers or add the code directly to the route. Below is an example, helping you create a route by adding the code directly.
Example: Replace the code in routes/web.php file and add the following code segment.
<?php
use Illuminate\Support\Facades\Route;
Route::get(‘/’, function () {
return “Welcome!”;
});
Run the project in the browser, and you will observe ‘Welcome!’ as the output.
100) What are some tools for sending emails in Laravel?
The following are some commonly used tools for sending emails in Laravel:
- Mailtrap
- Mailgun
- Mailchimp
- Mandrill
- Amazon Simple Email Service (SES)
- Swiftmailer
- Postmark
Related video:
- Mastering Qualitative Research: The Role of Focus Groups in Data Collection - July 11, 2024
- What is robots ops? - July 10, 2024
- 5 Effective Online Learning Strategies for DevOps Professionals - July 4, 2024