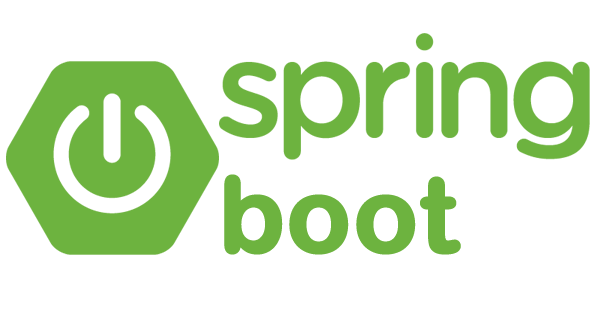
1) What is Spring boot?
Sprint boot is a Java-based spring framework used for Rapid Application Development (to build stand-alone microservices). It has extra support of auto-configuration and embedded application server like tomcat, jetty, etc.
2) What are the advantages of using Spring Boot?
The advantages of Spring Boot are listed below:
Easy to understand and develop spring applications.
Spring Boot is nothing but an existing framework with the addition of an embedded HTTP server and annotation configuration which makes it easier to understand and faster the process of development.
Increases productivity and reduces development time.
Minimum configuration.
We don’t need to write any XML configuration, only a few annotations are required to do the configuration.
3) What are the Spring Boot key components?
Below are the four key components of spring-boot:
Spring Boot auto-configuration.
Spring Boot CLI.
Spring Boot starter POMs.
Spring Boot Actuators.
4) What is the starter dependency of the Spring boot module?
Spring boot provides numbers of starter dependency, here are the most commonly used –
Data JPA starter.
Test Starter.
Security starter.
Web starter.
Mail starter.
Thymeleaf starter.
5) How does Spring Boot works?
Spring Boot automatically configures your application based on the dependencies you have added to the project by using annotation. The entry point of the spring boot application is the class that contains @SpringBootApplication annotation and the main method.
Spring Boot automatically scans all the components included in the project by using @ComponentScan annotation.
6) What does the @SpringBootApplication annotation do internally?
The @SpringBootApplication annotation is equivalent to using @Configuration, @EnableAutoConfiguration, and @ComponentScan with their default attributes. Spring Boot enables the developer to use a single annotation instead of using multiple. But, as we know, Spring provided loosely coupled features that we can use for each annotation as per our project needs.
7) What is the purpose of using @ComponentScan in the class files?
Spring Boot application scans all the beans and package declarations when the application initializes. You need to add the @ComponentScan annotation for your class file to scan your components added to your project.
8) How does a spring boot application get started?
Just like any other Java program, a Spring Boot application must have a main method. This method serves as an entry point, which invokes the SpringApplication#run method to bootstrap the application.
@SpringBootApplication
public class MyApplication {
public static void main(String[] args) { SpringApplication.run(MyApplication.class); // other statements }
}
9) What are starter dependencies?
Spring boot starter is a maven template that contains a collection of all the relevant transitive dependencies that are needed to start a particular functionality.
Like we need to import spring-boot-starter-web dependency for creating a web application.
org.springframework.boot spring-boot-starter-web
10) What is Spring Initializer?
Spring Initializer is a web application that helps you to create an initial spring boot project structure and provides a maven or gradle file to build your code. It solves the problem of setting up a framework when you are starting a project from scratch.
11) What is Spring Boot CLI and what are its benefits?
Spring Boot CLI is a command-line interface that allows you to create a spring-based java application using Groovy.
Example: You don’t need to create getter and setter method or access modifier, return statement. If you use the JDBC template, it automatically loads for you.
12) What Are the Basic Annotations that Spring Boot Offers?
The primary annotations that Spring Boot offers reside in its org.springframework.boot.autoconfigure and its sub-packages. Here are a couple of basic ones:
@EnableAutoConfiguration – to make Spring Boot look for auto-configuration beans on its classpath and automatically apply them.
@SpringBootApplication – used to denote the main class of a Boot Application. This annotation combines @Configuration, @EnableAutoConfiguration, and @ComponentScan annotations with their default attributes.
13) What is Spring Boot dependency management?
Spring Boot dependency management is used to manage dependencies and configuration automatically without you specifying the version for any of that dependencies.
14) Can we create a non-web application in Spring Boot?
Yes, we can create a non-web application by removing the web dependencies from the classpath along with changing the way Spring Boot creates the application context.
15) Is it possible to change the port of the embedded Tomcat server in Spring Boot?
Yes, it is possible. By using the server.port in the application.properties.
16) What is the default port of tomcat in spring boot?
The default port of the tomcat server-id 8080. It can be changed by adding sever.port properties in the application.property file.
17) Can we override or replace the Embedded tomcat server in Spring Boot?
Yes, we can replace the Embedded Tomcat server with any server by using the Starter dependency in the pom.xml file. Like you can use spring-boot-starter-jetty as a dependency for using a jetty server in your project.
18) Can we disable the default web server in the Spring boot application?
Yes, we can use application.properties to configure the web application type i.e spring.main.web-application-type=none.
19) Explain @RestController annotation in Sprint boot?
It is a combination of @Controller and @ResponseBody, used for creating a restful controller. It converts the response to JSON or XML. It ensures that data returned by each method will be written straight into the response body instead of returning a template.
20) What is the difference between @RestController and @Controller in Spring Boot?
@Controller Map of the model object to view or template and make it human readable but @RestController simply returns the object and object data is directly written in HTTP response as JSON or XML.
21) What are the Spring Boot Annotations?
The @RestController is a stereotype annotation. It adds @Controller and @ResponseBody annotations to the class. We need to import org.springframework.web.bind.annotation package in our file, in order to implement it.
22) What is Spring Boot dependency management?
Spring Boot manages dependencies and configuration automatically. You don’t need to specify version for any of that dependencies.
Spring Boot upgrades all dependencies automatically when you upgrade Spring Boot.
23) What are the Spring Boot properties?
Spring Boot provides various properties which can be specified inside our project’s application.properties file. These properties have default values and you can set that inside the properties file. Properties are used to set values like: server-port number, database connection configuration etc.
24) What are the Spring Boot Starters?
Starters are a set of convenient dependency descriptors which we can include in our application.
Spring Boot provides built-in starters which makes development easier and rapid. For example, if we want to get started using Spring and JPA for database access, just include the spring-boot-starter-data-jpa dependency in your project.
25) What is Spring Boot Actuator?
Spring Boot provides actuator to monitor and manage our application. Actuator is a tool which has HTTP endpoints. when application is pushed to production, you can choose to manage and monitor your application using HTTP endpoints.
26) What is thymeleaf?
It is a server side Java template engine for web application. It’s main goal is to bring elegant natural templates to your web application.
It can be integrate with Spring Framework and ideal for HTML5 Java web applications.
27) How to connect Spring Boot to the database using JPA?
Spring Boot provides spring-boot-starter-data-jpa starter to connect Spring application with relational database efficiently. You can use it into project POM (Project Object Model) file.
28) How to connect Spring Boot application to database using JDBC?
Spring Boot provides starter and libraries for connecting to our application with JDBC. Here, we are creating an application which connects with Mysql database. It includes the following steps to create and setup JDBC with Spring Boot.
29) What is @RestController annotation in Spring Boot?
The @RestController is a stereotype annotation. It adds @Controller and @ResponseBody annotations to the class. We need to import org.springframework.web.bind.annotation package in our file, in order to implement it.
30) What is @RequestMapping annotation in Spring Boot?
The @RequestMapping annotation is used to provide routing information. It tells to the Spring that any HTTP request should map to the corresponding method. We need to import org.springframework.web.annotation package in our file.
31) How to create Spring Boot application using Spring Starter Project Wizard?
There is one more way to create Spring Boot project in STS (Spring Tool Suite). Creating project by using IDE is always a convenient way. Follow the following steps in order to create a Spring Boot Application by using this wizard.
32) Spring Vs Spring Boot?
Spring is a web application framework based on Java. It provides tools and libraries to create a complete cutomized web application.
Wheras Spring Boot is a spring module which is used to create spring application project that can just run.
33) Can We Override Or Replace The Embedded Tomcat Server In Spring Boot?
Yes, we can replace the embedded tomcat server by using the starter dependencies. Like spring-boot-starter-jetty as a dependency for each project as you need.
34) Can We Disable The Default Web Server In The Spring Boot Application?
The major strong point in Spring is to provide flexibility to build your application loosely coupled. Spring provides features to disable the webserver in a quick configuration. Yes we can use the application properties to configure the web application type i.e, spring.main.web-application-type=none.
35) How To Disable A Specific Auto-Configuration Class?
You can use the exclude attribute of @EnableAutoConfiguration, if you find any specific auto-configuration classes that you do not want are being applied.
//By using “exclude”
@EnableAutoConfiguration(exclude={DataSourceAutoConfiguration.class})
36) What Does The @SpringBootApplication Annotation Do Internally?
As per the Spring Boot doc, the @SpringBootApplication annotation is equivalent to using @Configuration, @EnableAutoConfiguration, and @ComponentScan with their default attributes. Spring Boot enables the developer to use a single annotation instead of using multiple. But as we know, Spring provides loosely coupled features that we can use for each individual annotation as per our project needs.
37) How To Use A Property Defined In Application.Properties File Into Your Java Class?
Use the @Value annotation to access the properties which are defined in the application – properties file.
@Value(“${custom.value}”)
Private String custom Val;
38) Explain @RestController Annotation In Spring Boot?
@RestController is a convenience annotation for creating Restful controllers. It is a specialization of @Component and is autodetected through classpath scanning. It adds the @Controller and @ResponseBody annotations. It converts the response to JSON or XML.
Which eliminates the need to annotate every request handling method of the controller class with the @ResponseBody annotation. It is typically used in combination with annotated handler methods based on the @RequestMapping annotation.
Indicates that the data returned by each method will be written straight into the response body instead of rendering template.
39) What Is The Difference Between RequestMapping And GetMapping?
RequestMapping can be used with GET, POST,PUT, and many other requests methods using the method attribute on annotation. Whereas GetMapping is only an extension of RequestMapping, which helps you to improve clarity on requests.
40) Mention The Possible Sources Of External Configuration.
The most possible source of external configuration:
Application properties
Command line properties
Profile specific properties
41) Why we should avoid the Spring boot framework?
Besides advantages, there are few issues, where we should think about to adopt the spring boot framework to develop the microservice based architecture.
Spring boot unnecessary increase the size of the build with unused dependencies.
Not able to create the war file manually and difficult to configure externally.
Doesn’t provide much control and tuning the running build.
It’s only suitable for micro-services which eventually need to deploy in docker, but not large or mono lithics web services.
Spring boot doesn’t provide good compatibility if we are integrating third party framework.
42) Why do we encourage to use the spring boot over the other framework?
Spring boot provides good compatibility with other spring frameworks which is used to provide the security, persistency features. Spring boot provides good support with docker containerization, which makes it a good choice to deploy the microservice based application and easy to maintain.
Provide the easiest way to configure the java beans.
Provide a powerful batch processing and manage rest endpoints.
Provide auto-configuration mechanism, that means no manual configuration needed.
Provide annotation-based configuration, so no need to configure the xml file manually.
Ease the dependency management.
It includes the Embedded servlet container.
Spring boot comes with spring cloud framework, which has many libraries which are used to handle all types of nonfunctional requirement, which is usually not available in other frameworks.
43) How spring boot work internally?
Spring boot provides many abstraction layers to ease the development, underneath there are vital libraries which work for us.
Below is the key function performing internally.
Using @EnableAutoConfigure annotation the spring boot application configures the spring boot application automatically.
E.g. If you need MySQL DB in your project, but you haven’t configured any database connection, in that case, Spring boot auto configures as in memory database.
The entry point of spring boot application is a class which contains @SpringBootApplication annotation and has the main method.
Spring boot scan all the components included in the project by using @ComponentScan annotation.
Let’s say we need the Spring and JPA for database connection, then we no need to add the individual dependency we can simply add the spring-boot-starter-data-jpa in the project.
Spring boot follows the naming convention for dependency like spring-boot-starter.
Considering above there are other internal functions which play a significant role in spring boot.
44) What is the important dependency of spring boot application?
Below are the key dependencies which you need to add in maven based or Gradle based applications, to make the application compatible to use spring boot functionality.
spring-boot-starter-parent
spring-boot-starter-web
spring-boot-starter-actuator
spring-boot-starter-security
spring-boot-starter-test
spring-boot-maven-plugin
These dependencies come with associated child dependencies, which are also downloaded as a part of parent dependencies.
45) What are the basic components of spring boot?
Below is the basic component, which plays a vital role in spring boot framework for configuration, development, deployment, and execution of microservices based application.
Spring boot starter.
Spring boot auto configurator.
Spring boot actuator.
Spring boot CLI.
Spring boot Initilizr.
46) What are the important annotation for Spring rest? What is the use case of this annotation?
@RestController: Define at class level, so that spring container will consider as RestENd point
@RequestMapping(value = “/products”): Define the REST URL for method level.
@PathVariable: Define as a method argument
@RequestBody: Define as a method argument
@ResponseEntity: To convert the domain object into the response format
@hasAuthority: To grant the access of corresponding endpoints
@GetMapping: To make endpoint compatible for get request.
@PostMapping: To make endpoint compatible for post request.
@PutMapping: To make endpoint compatible for put request.
@DeleteMapping: To make endpoint compatible for delete request.
@ResponseStatus: To generate the HTTP status.
@ResponseBody: To Generate the response message.
47) What is Spring Application Properties and how it is used?
Spring Boot lets us define Application Properties to support us for work in different environments. These properties include parameters like application name, server port number, data source details, profiles, and many other useful properties. Spring Boot supports YAML based properties configurations to run the application.
We can simply put an “application.yml” file in “src/main/resources” directory, and it will be auto-detected. So, by using this default file, we don’t need to explicitly register a PropertySource, or provide a path to a property file, unlike native Spring where we have explicitly define them.
A sample application.yml file looks like below:
spring:
application:
name: SampleRestService
profiles:
active: prod
server.port = 9090
48) Name & explain Common Boot Starters.
spring-boot-starter-actuator: This starter is very useful and will be used most especially when you are developing microservices etc. This provides production-ready features to help you monitor and manage your application. It provides a lot of inbuilt endpoints, for example, health endpoint, /env endpoint.
spring-boot-starter-web: Starter for building web, including RESTful, applications using Spring MVC. Uses Tomcat as the default embedded container
spring-boot-starter-data-jpa: This is for using Spring Data JPA, default vendor is hibernated however you can override it to some other vendor, for example, ibatis.
spring-boot-starter-data-MongoDB: Starter for using MongoDB document-oriented database and Spring Data MongoDB.
Spring-boot-starter-thymeleaf: Starter for building MVC web applications using Thymeleaf views
spring-boot-starter-security: Starter for using Spring Security.
spring-boot-starter-test: Starter for testing Spring Boot applications with libraries including JUnit, Hamcrest and Mockito
49) What is Spring Boot DevTools?
Spring Boot provides ‘spring-boot-devtools’ module with features that help the programmers while developing the application. It basically improves the experience of developing a Spring Boot application. One of the key features is automatic reload of application as soon as there is a change; hence developer does not need to stop and start the application each time. The is an intelligent feature as it only reloads the actively developed classes but not the third-party JARs.
Another key feature is that by def,ault it disables the caching of view templates and static files. This enables a developer to see the changes as soon as they make them.
In case, we want to disable any of these features, then we need to set them in an application.yml file. For example -Dspring.devtools.restart.enabled=false will avoid automatic application restart.
Below are a few of the features provided:
Property defaults
Automatic Restart
Live Reload
Global settings
Remote applications
50) What is Spring Cloud Contract?
Spring Cloud Contract implements the Consumer Driven Contracts (CDC) approach via the ‘Spring Cloud Contract Verifier’ project.
Consumer Driven Contracts is a software development and evolution approach where each consumer of service develops a contract that contains the consumer’s expectations about the APIs provided by Service. The full collection of all of the consumers’ contracts constitutes the requirement set for the service.
Once the service owners have all of the contracts for their consumers, the service owners can develop a test suite that verifies the service APIs. This test suite provides rapid feedback about failures when the service changes.
In Spring Cloud Contract, a “producer” is the owner of an API and a “consumer” is the user of an API.
Service A (as a consumer) creates a contract that service B (as a producer) will have to abide by. This contract acts as the invisible glue between services – even though they live in separate code bases and run on different JVMs. Breaking changes can be detected immediately during build time.
Related video:
- Mastering Qualitative Research: The Role of Focus Groups in Data Collection - July 11, 2024
- What is robots ops? - July 10, 2024
- 5 Effective Online Learning Strategies for DevOps Professionals - July 4, 2024