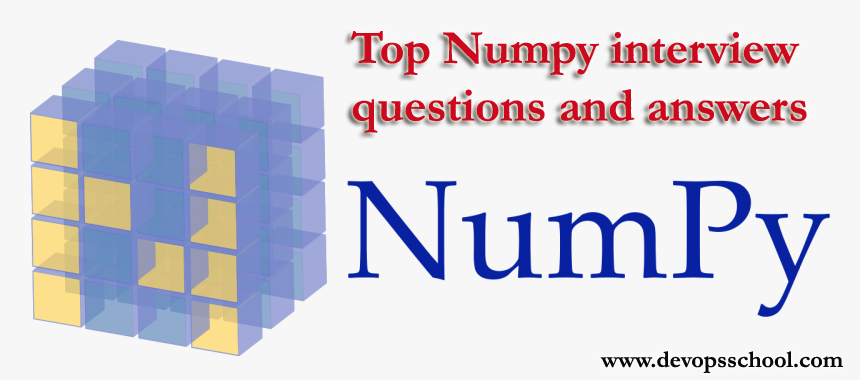
1) What is Numpy?
Ans: NumPy is a general-purpose array-processing package. It provides a high-performance multidimensional array object, and tools for working with these arrays. It is the fundamental package for scientific computing with Python. … A powerful N-dimensional array object. Sophisticated (broadcasting) functions.
2) Why NumPy is used in Python?
Ans: NumPy is a package in Python used for Scientific Computing. NumPy package is used to perform different operations. The ndarray (NumPy Array) is a multidimensional array used to store values of same datatype. These arrays are indexed just like Sequences, starts with zero.
3) What does NumPy mean in Python?
Ans: NumPy (pronounced /ˈnʌmpaɪ/ (NUM-py) or sometimes /ˈnʌmpi/ (NUM-pee)) is a library for the Python programming language, adding support for large, multi-dimensional arrays and matrices, along with a large collection of high-level mathematical functions to operate on these arrays.
4) Where is NumPy used?
Ans: NumPy is an open source numerical Python library. NumPy contains a multi-dimentional array and matrix data structures. It can be utilised to perform a number of mathematical operations on arrays such as trigonometric, statistical and algebraic routines. NumPy is an extension of Numeric and Numarray.
5) How to Install Numpy in Windows?
Ans: Step 1: Download Python for Windows 10/8/7. First, download the Python executable binaries on your Windows system from the official download the page of the Python. …
Step 2: Run the Python executable installer. …
Step 3: Install pip on Windows 10/8/7. …
Step 4: Install Numpy in Python using pip on Windows 10/8/7.
Installation Process of Numpy..
step1: Open the terminal
step2: type pip install numpy
6) How to import numpy in python?
Ans: import numpy as np
7) how to create 1D Array ?
Ans: num=[1,2,3]
num = np.array(num)
print(“1d array : “,num)
8) How to create 2D Array ?
Ans: num2=[[1,2,3],[4,5,6]]
num2 = np.array(num2)
print(“\n2d array : “,num2)
9) How to create 3D Array or ND Array ?
Ans: num3=[[[1,2,3],[4,5,6],[7,8,9]]]
num3 = np.array(num3)
print(“\n3d array : “,num3)
10) How to use shape for 1D Array ?
Ans: num=[1,2,3] if not defined
print(‘\nshpae of 1d ‘,num.shape)
11) How to use shape for 2D Array ?
Ans: num2=[[1,2,3],[4,5,6]] if not added
print(‘\nshpae of 2d ‘,num2.shape)
12) How to use shape for 3d or Nd Array ?
Ans: num3=[[[1,2,3],[4,5,6],[7,8,9]]] if not added
print(‘\nshpae of 3d ‘,num3.shape)
13) How to identified datatyp for numpy array?
Ans: print(‘\n data type num 1 ‘,num.dtype)
print(‘\n data type num 2 ‘,num2.dtype)
print(‘\n data type num 3 ‘,num3.dtype)
14) Print 5 zeros ?
Ans: arr = np.zeros(5)
print(‘single arrya’,arr)
15) print zeros with 2 rows and 3 columns ?
Ans: arr2 = np.zeros((2,3))
print(‘\nprint 2 rows and 3 cols : ‘,arr2)
16) use of eye() diagonal values ?
Ans: arr3 = np.eye(4)
print(‘\ndiaglonal values : ‘,arr3)
17) use of diag() square matrix ?
Ans: arr3 = np.diag([1,2,3,4])
print(‘\n square matrix’,arr3)
18) Print Range Between 1 To 15 and show 4 integers random numbers
Ans: rand_arr = np.random.randint(1,15,4)
print(‘\n random number from 1 to 15 ‘,rand_arr)
19) Print Range Between 1 To 100 and show 4 integers random numbers
Ans: rand_arr3 = np.random.randint(1,100,20)
print(‘\n random number from 1 to 100 ‘,rand_arr3)
20) Print Range Between random number 2 row and 3 cols integers random numbers
Ans: rand_arr2 = np.random.randint([2,3])
print(‘\n random number 2 row and 3 cols ‘,rand_arr2)
21) I’ve Found A Bug. What Do I Do?
The SciPy development team works hard to make SciPy as reliable as possible, but, as in any software product, bugs do occur. If you find bugs that affect your software, please tell us by entering a ticket in the SciPy bug tracker, or NumPy bug tracker, as appropriate.
22) How Can I Get Involved In Scipy?
Drop us a mail on the mailing lists. We are keen for more people to help out writing code, unit tests, documentation (including translations into other languages), and helping out with the website.
23) Is There Commercial Support Available?
Yes, commercial support is offered for SciPy by Enthought.
24) What Is The Difference Between Numpy And Scipy?
In an ideal world, NumPy would contain nothing but the array data type and the most basic operations: indexing, sorting, reshaping, basic elementwise functions, et cetera. All numerical code would reside in SciPy. However, one of NumPy’s important goals is compatibility, so NumPy tries to retain all features supported by either of its predecessors. Thus NumPy contains some linear algebra functions, even though these more properly belong in SciPy. In any case, SciPy contains more fully-featured versions of the linear algebra modules, as well as many other numerical algorithms. If you are doing scientific computing with python, you should probably install both NumPy and SciPy. Most new features belong in SciPy rather than NumPy.
25) How Do I Make Plots Using Numpy/scipy?
Plotting functionality is beyond the scope of NumPy and SciPy, which focus on numerical objects and algorithms. Several packages exist that integrate closely with NumPy to produce high quality plots, such as the immensely popular Matplotlib and the extensible, modular toolkit Chaco.
26) How Do I Make 3d Plots/visualizations Using Numpy/scipy?
Like 2D plotting, 3D graphics is beyond the scope of NumPy and SciPy, but just as in the 2D case, packages exist that integrate with NumPy. Matplotlib provides basic 3D plotting in the mplot3d subpackage, whereas Mayavi provides a wide range of high-quality 3D visualization features, utilizing the powerful VTK engine.
27) Why Both Numpy.linalg And Scipy.linalg? What’s The Difference?
One of the design goals of NumPy was to make it buildable without a Fortran compiler, and if you don’t have LAPACK available NumPy will use its own implementation. SciPy requires a Fortran compiler to be built, and heavily depends on wrapped Fortran code.
The linalg modules in NumPy and SciPy have some common functions but with different docstrings, and scipy.linalgcontains functions not found in numpy.linalg, such as functions related to LU decomposition and the Schur decomposition, multiple ways of calculating the pseudoinverse, and matrix transcendentals like the matrix logarithm. Some functions that exist in both have augmented functionality in scipy.linalg; for example scipy.linalg.eig() can take a second matrix argument for solving generalized eigenvalue problems.
28) Do Numpy And Scipy Support Python 3.x?
NumPy and SciPy support the Python 2.x series, (versions 2.6 and 2.7), as well as Python 3.2 and newer. The first release of NumPy to support Python 3 was NumPy 1.5.0. Python 3 support in SciPy starts with version 0.9.0.
29) Does Numpy/scipy Work With Jython?
No. Simply put, Jython runs on top of the Java Virtual Machine and has no way to interface with extensions written in C for the standard Python (CPython) interpreter.
30) Does Numpy/scipy Work With Ironpython (.net)?
Some users have reported success in using NumPy with Ironclad on 32-bit Windows. The current status of Ironclad support for SciPy is unknown, but there are several complicating factors (namely the Fortran compiler situation on Windows) that make it less feasible than NumPy.
31) What Is The Preferred Way To Check For An Empty (zero Element) Array?
If you are certain a variable is an array, then use the size attribute. If the variable may be a list or other sequence type, use len().
The size attribute is preferable to len because:
a = numpy.zeros((1,0))
a.size
0
whereas
len(a)
1
32) I Want To Load Data From A Text File. How Do I Make This Code More Efficient?
Use numpy.loadtxt(). Even if your text file has header and footer lines or comments, loadtxt can almost certainly read it; it is convenient and efficient.
If you find this still too slow, you should consider changing to a more efficient file format than plain text. There are a large number of alternatives, depending on your needs (and on which version of NumPy/SciPy you are using):
Text files: slow, huge, portable, human-readable; built into
NumPy Raw binary: no metadata, totally unportable, fast; built into
NumPy pickle: somewhat slow, somewhat portable (may be incompatible with different NumPy versions); built into NumPy
MATLAB format: portable; built into SciPy (scipy.io.loadmat())
HDF5: high-powered kitchen-sink format; both PyTables and h5py provide a NumPy friendly interface on top of the core HDF5 library written in C.
FITS: standard kitchen-sink format in astronomy; the astropy library provides a convenient Python interface through its io.fits package. .npy: NumPy native binary data format, simple, efficient, portable; built into NumPy as of 1.0.5.
33) Why Not Just Have A Separate Operator For Matrix Multiplication?
From Python 3.5, the @ symbol will be defined as a matrix multiplication operator, and Numpy and Scipy will make use of this. This addition was the subject of PEP 465. The separate matrix and array types exist to work around the lack of this operator in earlier versions of Python.
34) How Do I Find The Indices Of An Array Where Some Condition Is True?
Answer :The prefered idiom for doing this is to use the function numpy.nonzero() , or the nonzero() method of an array. Given an array a, the condition a > 3 returns a boolean array and since False is interpreted as 0 in Python and NumPy, np.nonzero(a > 3)yields the indices of a where the condition is true.
import numpy as np
a = np.array([[1,2,3],[4,5,6],[7,8,9]])
a > 3
array([[False, False, False],
[ True, True, True],
[ True, True, True]], dtype=bool)
np.nonzero(a > 3)
(array([1, 1, 1, 2, 2, 2]), array([0, 1, 2, 0, 1, 2]))
The nonzero() method of the boolean array can also be called.
(a > 3).nonzero()
(array([1, 1, 1, 2, 2, 2]), array([0, 1, 2, 0, 1, 2]))
35) How Do I Count The Number Of Times Each Value Appears In An Array Of Integers?
Use numpy.bincount(). The resulting array is
arr = numpy.array([0, 5, 4, 0, 4, 4, 3, 0, 0, 5, 2, 1, 1, 9])
numpy.bincount(arr)
The argument to bincount() must consist of positive integers or booleans. Negative integers are not supported.
36) List the advantages NumPy Arrays have over (nested) Python lists?
Python’s lists, even though hugely efficient containers capable of a number of functions, have several limitations when compared to NumPy arrays. It is not possible to perform vectorised operations which includes element-wise addition and multiplication.
They also require that Python store the type information of every element since they support objects of different types. This means a type dispatching code must be executed each time an operation on an element is done. Also, each iteration would have to undergo type checks and require Python API bookkeeping resulting in very few operations being carried by C loops.
37) List the steps to create a 1D array and 2D array
A one-dimensional array is created as follows:
num=[1,2,3]
num = np.array(num)
print(“1d array : “,num)
A two-dimensional array is created as follows:
num2=[[1,2,3],[4,5,6]]
num2 = np.array(num2)
print(“\n2d array : “,num2)
38) How do you create a 3D array?
A three-dimensional array is created as follows:
num3=[[[1,2,3],[4,5,6],[7,8,9]]]
num3 = np.array(num3)
print(“\n3d array : “,num3)
39) What are the steps to use shape for a 1D array, 2D array and 3D/ND array respectively?
1D Array:
num=[1,2,3] if not added
print(‘\nshpae of 1d ‘,num.shape)
2D Array:
num2=[[1,2,3],[4,5,6]] if not added
print(‘\nshpae of 2d ‘,num2.shape)
3D or ND Array:
num3=[[[1,2,3],[4,5,6],[7,8,9]]] if not added
print(‘\nshpae of 3d ‘,num3.shape)
40) How can you identify the datatype of a given NumPy array?
Use the following sequence of codes to identify the datatype of a NumPy array.
print(‘\n data type num 1 ‘,num.dtype)
print(‘\n data type num 2 ‘,num2.dtype)
print(‘\n data type num 3 ‘,num3.dtype)
41) What is the procedure to count the number of times a given value appears in an array of integers?
You can count the number of the times a given value appears using the bincount() function. It should be noted that the bincount() function accepts positive integers or boolean expressions as its argument. Negative integers cannot be used.
Use NumPy.bincount(). The resulting array is
arr = NumPy.array([0, 5, 4, 0, 4, 4, 3, 0, 0, 5, 2, 1, 1, 9])
NumPy.bincount(arr)
42) What is the procedure to find the indices of an array on NumPy where some condition is true?
You may use the function numpy.nonzero() to find the indices or an array. You can also use the nonzero() method to do so.
In the following program, we will take an array a, where the condition is a > 3. It returns a boolean array. We know False on Python and NumPy is denoted as 0. Therefore, np.nonzero(a > 3) will return the indices of the array a where the condition is True.
import numpy as np
a = np.array([[1,2,3],[4,5,6],[7,8,9]])
a > 3
array([[False, False, False],
[ True, True, True],
[ True, True, True]], dtype=bool)
np.nonzero(a > 3)
(array([1, 1, 1, 2, 2, 2]), array([0, 1, 2, 0, 1, 2]))
You can also call the nonzero() method of the boolean array.
(a > 3).nonzero()
(array([1, 1, 1, 2, 2, 2]), array([0, 1, 2, 0, 1, 2]))
43) Shown below is the input NumPy array. Delete column two and replace it with the new column given below.
import NumPy
sampleArray = NumPy.array([[34,43,73],[82,22,12],[53,94,66]])
newColumn = NumPy.array([[10,10,10]])
Expected Output:
Printing Original array
[[34 43 73]
[82 22 12]
[53 94 66]]
Array after deleting column 2 on axis 1
[[34 73]
[82 12]
[53 66]]
Array after inserting column 2 on axis 1
[[34 10 73]
[82 10 12]
[53 10 66]]
Solution:
import NumPy
print(“Printing Original array”)
sampleArray = NumPy.array([[34,43,73],[82,22,12],[53,94,66]])
print (sampleArray)
print(“Array after deleting column 2 on axis 1”)
sampleArray = NumPy.delete(sampleArray , 1, axis = 1)
print (sampleArray)
arr = NumPy.array([[10,10,10]])
print(“Array after inserting column 2 on axis 1”)
sampleArray = NumPy.insert(sampleArray , 1, arr, axis = 1)
print (sampleArray)
44) Create a two 2-D array. Plot it using matplotlib
Solution:
import NumPy
print(“Printing Original array”)
sampleArray = NumPy.array([[34,43,73],[82,22,12],[53,94,66]])
print (sampleArray)
print(“Array after deleting column 2 on axis 1”)
sampleArray = NumPy.delete(sampleArray , 1, axis = 1)
print (sampleArray)
arr = NumPy.array([[10,10,10]])
print(“Array after inserting column 2 on axis 1”)
sampleArray = NumPy.insert(sampleArray , 1, arr, axis = 1)
print (sampleArray)
45) Why NumPy is faster than list?
NumPy Arrays are faster than Python Lists because of the following reasons: An array is a collection of homogeneous data-types that are stored in contiguous memory locations. On the other hand, a list in Python is a collection of heterogeneous data types stored in non-contiguous memory locations
46) What is the main use of NumPy?
NumPy can be used to perform a wide variety of mathematical operations on arrays. It adds powerful data structures to Python that guarantee efficient calculations with arrays and matrices and it supplies an enormous library of high-level mathematical functions that operate on these arrays and matrices.
47) Does NumPy use multiple cores?
I know that numpy is configured for multiple cores, since I can see tests using numpy. dot use all my cores, so I just reimplemented mean as a dot product, and it runs way faster.
48) What is difference between NumPy and Pandas?
Difference between Pandas and NumPy: … NumPy library provides objects for multi-dimensional arrays, whereas Pandas is capable of offering an in-memory 2d table object called DataFrame. NumPy consumes less memory as compared to Pandas. Indexing of the Series objects is quite slow as compared to NumPy arrays.
49) Can you import libraries in coding interviews?
During a coding interview, you can always ask if you can use such-and-such a function from the library, but generally you can as long as that doesn’t save you from actually having to solve the problem. If you’re asked to implement a sort, for example, you shouldn’t do it by calling the library sort.
50) Does NumPy use SIMD?
NumPy comes with a flexible working mechanism that allows it to harness the SIMD features that CPUs own, in order to provide faster and more stable performance on all popular platforms.
Related video:
- Mastering Qualitative Research: The Role of Focus Groups in Data Collection - July 11, 2024
- What is robots ops? - July 10, 2024
- 5 Effective Online Learning Strategies for DevOps Professionals - July 4, 2024