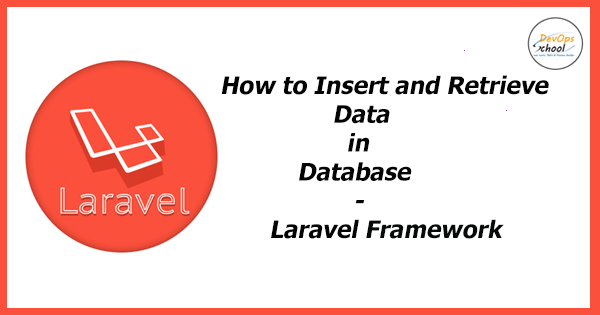
In this example, I am going to show you how to insert data in the database using laravel framework PHP.
First, creating table the SQL query:
CREATE TABLE student_details
(
id int NOT NULL AUTO_INCREMENT,
first_name varchar(50),
last_name varchar(50),
city_name varchar(50),
email varchar(50),
PRIMARY KEY (id)
);
Now, create three file for insert data in Laravel.
Step 1. Create a controller name as StudInsertController.php.
The file location is : (app/Http/Controllers/StudInsertController.php)
Step 2. Create view page name as stud_create.php
The file location is: (resources/views/stud_create.php)
Then put this code in your StudInsertController.php.
Then put this code in your stud_create.blade.php.
Step 3. Then go to routes as web.php and put this code.
The file location is : (routes/web.php)
Route::get('insert','StudInsertController@insertform');
Route::post('create','StudInsertController@insert');
Then, you can see the data is inserted.
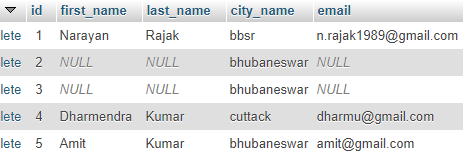
So, the data is inserted in the database, then we need to retrieve a record or data from the MySQL database.
Step 4. Create a controller for view name as StudViewController.php.
The file location is : (app/Http/Controllers/StudViewController.php)
Step 5. Create a view page name as stud_view.blade.php.
The file location is : (resources/views/stud_view.blade.php).
Then put this code StudViewController.php.
Then, retrieve the students data then put this code stud_view.blade.php.
Step 6. Then go to routes as web.php and put this code.
The file location is (routes/web.php)
Route::get('view-records','StudViewController@index');
Then, you can see the view page.
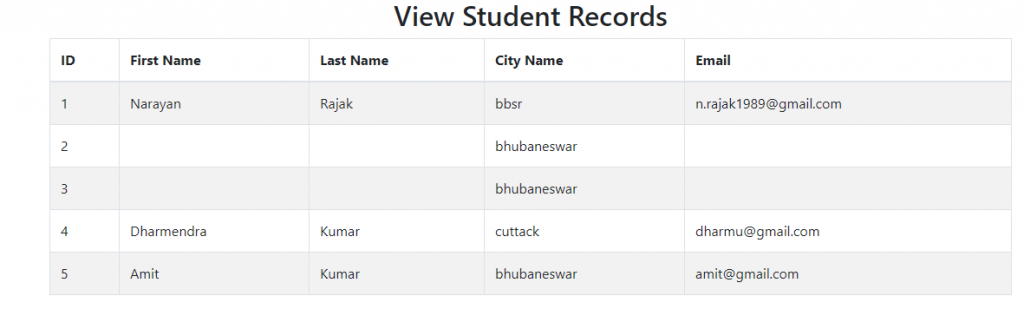

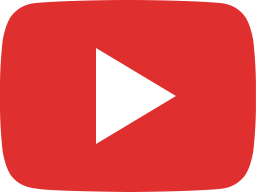

- Top 5 Reliable Phone Number Validation API for Your Next Product - February 10, 2022
- Top 5 IT Management Tools | IT Management Software - July 30, 2021
- Complete guide of PHP certification courses, tutorials & training - July 23, 2021