1) What is Ruby programming language?

Ruby is a dynamic, reflective, open source programming language that aims on simplicity and productivity.
Ruby has a blended functions of Perl, small talk, Eiffel, Ada and Lisp. Ruby was designed to developed a new language which makes a stability with the functionality of Imperative languages.
2) Who is the developer of Ruby?
Ruby is designed and developed by Yukihiro “Martz” Matsumoto in mid 1990s in Japan.
The name “Ruby” originated during a chat session between Matsumoto and Keiju Ishitsuka. Two names were selected, “Coral” and “Ruby”. Matsumoto chose the later one as it was the birthstone of one of his colleagues.
3) What are class libraries in Ruby?
Ruby class libraries contain variety of domain such as thread programming, data types, various domains. Following is a list of domains which has relevant class libraries:
- Text processing
- CGI Programming
- Network programming
- GUI programming
- XML programming
4) List some features of Ruby?
Ruby has many features. Some of them are listed below.
- Object-oriented
- Flexible
- Dynamic typing and Duck typing
- Garbage collector
- Keyword arguments
5) Name some operators used in Ruby?
Operators are a symbol which is used to perform different operations.
- Unary operator
- Airthmetic operator
- Bitwise operator
- Logical operator
- Ternary operator
6) What are Ruby variables?
Ruby variables hold data which can be used later in a program. Each variable act as a memory and shas a different name.
There are four types of variables in Ruby:
- Local variable
- Class variable
- Instance variable
- Global variable
7) What is the difference between nil and false in Ruby?
Nil | False |
Nil cannot be a value. | False can be a value. |
Nil is returned where there is no predicate | In case of a predicate, true or false is returned by a method. |
Nil is not a boolean data type. | False is a boolean data type. |
Nil is an object of nilclass. | False is an object of falseclass. |
8) Explain Ruby data types.
Ruby data types represent type of data such as text, string, numbers, etc.
There are different data types in Ruby:
- Numbers
- Strings
- Symbols
- Hashes
- Arrays
- Booleans
9) What is the use of load and require in Ruby?
In Ruby, load and require both are used for loading the available code into the current code. In cases where loading the code required every time when changed or every times someone hits the URL, it is suggested to use ‘load’.
10) Explain while loop in Ruby?
Ruby while loop is used to iterate a program several times. If the number of iterations is not fixed
for a program, while loop is used.
11) Explain break statement in Ruby?
Ruby break statement is used to terminate a loop. It is mostly used in while loop where value is printed till the condition is true.
12) Explain redo statement in Ruby?
Ruby redo statement is used to repeat the current iteration of the loop. The redo statement is executed without evaluating loop’s condition.
13) Explain retry statement in Ruby?

Ruby retry statement is used to repeat the whole loop iteration from the start.
14) How will you comment in Ruby?
Ruby comments are non-executable lines in a program. They do not take part in the execution of a program.
15) Define Ruby methods?
Ruby method prevent us from writing the same code in a program again and again. Ruby methods are similar to functions in other languages.
16) How to create Ruby object?
Objects in Ruby are created by calling new method of the class. It is a unique type of method and predefined in Ruby library.
17) How to use Ruby methods?
To use a Ruby method, we need to first define it. It is defined with def and end keyword.
Method name should always start with a lowercase letter.
18) In how many ways a block is written in Ruby?
A block is written in two ways:
- Multi-line between do and end
- Inline between braces {}
Both are same and have the same functionality.
19) What is yield statement in Ruby?
The yield statement is used to call a block within a method with a value.
20) Explain Ruby module?
Ruby module is a collection of methods and constants. A module method may be instance method or module method. They are similar to classes as they hold a collection of methods, class definitions, constants and other modules. They are defined like classes. Objects or subclasses can not be created using modules. There is no module hierarchy of inheritance.
Modules basically serve two purposes:
- They act as namespace. They prevent the name clashes.
- They allow the mixin facility to share functionality between classes.
21) Explain module mixins in Ruby?
Ruby doesn’t support multiple inheritance. Modules eliminate the need of multiple inheritance using mixin in Ruby.
22) Explain ampersand parameter (&block) in Ruby?
The &block is a way to pass a reference (instead of a local variable) to the block to a method.
Here, block word after the & is just a name for the reference, any other name can be used instead of this.
23) Explain Ruby strings?
Ruby string object holds and manipulates an arbitary sequence of bytes, typically representing characters. They are created using String::new or as literals.
24) How to access Ruby strings elements in an application?
You can access Ruby string elements in different parts with the help of square brackets []. Within square brackets write the index or string.
25) How to write multiline string in Ruby?
Writing multiline string is very simple in Ruby language. We will show three ways to print multiline string.
String can be written within double quotes.
The % character is used and string is enclosed within / character.
In heredoc syntax, we use << and string is enclosed within word STRING.
26) What is concatenating string in Ruby. In how many ways you can create a concatenating string?
Ruby concatenating string implies creating one string from multiple strings. You can join more than one string to form a single string by concatenating them.
There are four ways to concatenate Ruby strings into single string:
- Using plus sign in between strings.
- Using a single space in between strings.
- Using << sign in between strings.
- Using concat method in between strings.
27) What is the use of global variable $ in Ruby?
The global variable is declared in Ruby that you can access it anywhere within the application because it has full scope in the application. The global variables are used in Ruby with $ prepend.
28) In how many ways you can compare Ruby string?
Ruby strings can be compared with three operators:
- With == operator : Returns true or false
- With eql? Operator : Returns true or false
- With casecmp method : Returns 0 if matched or 1 if not matched
29) What are Ruby arrays and how they can be created?
Ruby arrays are ordered collections of objects. They can hold objects like integer, number, hash, string, symbol or any other array.
Its indexing starts with 0. The negative index starts with -1 from the end of the array. For example, -1 indicates last element of the array and 0 indicates first element of the array.
A Ruby array is created in many ways.
- Using literal constructor []
- Using new class method
30) What are class libraries in Ruby?
Ruby class libraries contain variety of domain such as thread programming, data types, various domains. Following is a list of domains which has relevant class libraries:
- Text processing
- CGI Programming
- Network programming
- GUI programming
- XML programming
31) In how many ways items can be added in an array in Ruby?
Ruby array elements can be added in different ways.
- push or <<
- unshift
- insert
32) How to access Ruby array elements? How many methods are used to access Ruby elements.?
Ruby array elements can be accessed using #[] method. You can pass one or more than one arguments or even a range of arguments.
Methods used to access Ruby elements:
- at method
- slice method
- fetch method
- first and last method
- take method
- drop method
33) Explain Ruby hashes?
A Ruby hash is a collection of unique keys and their values. They are similar to arrays but array use integer as an index and hash use any object type. They are also called associative arrays, dictionaries or maps.
If a hash is accessed with a key that does not exist, the method will return nil.
34) How to create a new time instance in Ruby?
A new Time instance can be created with ::new. This will use your current system’s time. Parts of time like year, month, day, hour, minute, etc can also be passed.
While creating a new time instance, you need to pass at least a year. If only year is passed, then time will default to January 1 of that year at 00:00:00 with current system time zone.
35) Explain Ruby ranges. What are the ways to define ranges?
Ruby range represents a set of values with a beginning and an end. They can be constructed using s..e and s…e literals or with ::new.
The ranges which has .. in them, run from beginning to end inclusively. The ranges which has … in them, run exclusively the end value.
Ruby has a variety of ways to define ranges.
- Ranges as sequences
- Ranges as conditions
- Ranges as intervals
36) What are Ruby iterators?
Iterator is a concept used in object-oriented language. Iteration means doing one thing many times like a loop.
The loop method is the simplest iterator. They return all the elements from a collection, one after the other. Arrays and hashes come in the category of collection.
37) How many iterators are there in Ruby?
Following iterators are there in Ruby:
- each iterator
- times iterator
- upto and downto iterator
- step iterator
- each_line iterator
38) How to open a file in Ruby?
A Ruby file can be created using different methods for reading, writing or both.
There are two methods to open a file in Ruby.
- File.new method : Using this method a new file can be created for reading, writing or both.
- File.open method : Using this method a new file object is created. That file object is assigned to a file.
Difference between both the methods is that File.open method can be associated with a block while File.new method can’t.
Syntax:
f = File.new("fileName.rb")
or,
File.open("fileName.rb", "mode") do |f|
39) How to read a file in Ruby?
There are three different methods to read a file.
To return a single line, following syntax is used.
Syntax:
f.gets
code...
To return the whole file after the current position, following syntax is used.
Syntax:
f.rea
code...
To return file as an array of lines, following syntax is used.
Syntax:
f.readlines
[code...]
40) What is sysread method in Ruby?
The sysread method is also used to read the content of a file. With the help of this method you can open a file in any mode
41) How will you rename and delete a file in Ruby?
Ruby files are renamed using rename method and deleted using delete mehtod.
To rename a file, following syntax is used.
Syntax:
File.rename("olderName.txt", "newName.txt")
To delete a file, following syntax is used.
Syntax:
File.delete("filename.txt")
42) How to check whether a directory exist or not in Ruby?
To check whether a directory exists or not exists? Method is used.
Syntax:
puts Dir.exists? "dirName"
43) Explain Ruby exceptions?
Ruby exception is an object, an instance of the class Exception or descendent of that class. When something goes wrong, Ruby program throws an exceptional behavior. By default Ruby program terminates on throwing an exception.
44) What are some built-in Ruby class exceptions?
Built-in subclasses of exception are as follows:
- NoMemoryError
- ScriptError
- SecurityError
- SignalException
45) How an exception is handled in Ruby?
To handle exception, the code that raises exception is enclosed within begin-end block. Using rescue clauses we can state type of exceptions we want to handle.
46) Explain the use of retry statement in Ruby?

Usaually in a rescue clause, the exception is captured and code resumes after begin block. Using retry statement, the rescue block code can be resumed from begin after capturing an exception.
Syntax:
47) Explain the use of ensure statement in Ruby?
There is an ensure clause which guarantees some processing at the end of code. The ensure block always run whether an exception is raised or not. It is placed after last rescue clause and will always executed as the block terminates.
The ensure block will run at any case whether an exception arises, exception is rescued or code is terminated by uncaught exception.
Syntax:
48) What is the difference between calling super and calling super()?
A call to super invokes the parent method with the same arguments that were passed to the child method. An error will therefore occur if the arguments passed to the child method don’t match what the parent is expecting.
A call to super() invokes the parent method without any arguments, as presumably expected. As always, being explicit in your code is a good thing.
49) What will val1 and val2 equal after the code below is executed? Explain your answer.
Answer –
Although these two statements might appear to be equivalent, they are not, due to the order of operations. Specifically, the and and or operators have lower precedence than the = operator, whereas the && and || operators have higher precedence than the = operator, based on order of operations.
To help clarify this, here’s the same code, but employing parentheses to clarify the default order of operations:
50) Which of the expressions listed below will result in “false”?
true | ? “true” : “false” | |
false | ? “true” : “false” | |
nil | ? “true” : “false” | |
1 | ? “true” : “false” | |
0 | ? “true” : “false” | |
“false” | ? “true” : “false” | |
“” | ? “true” : “false” | |
[] | ? “true” : “false” | |
Answer –
In Ruby, the only values that evaluate to false are false and nil. Everything else – even zero (0) and an empty array ([]) – evaluates to true.
This comes as a real surprise to programmers who have previously been working in other languages like JavaScript.
51) What will be the result of each of the following lines of code?
Answer-
The first three lines of code will all print out 10, as expected.
The next two lines of code will both print out 3, as expected.
However, the last line of code (i.e., sum (1,2)) will result in the following:
Syntax:
The problem is the space between the method name and the open parenthesis. Because of the space, the Ruby parser thinks that (1, 2) is an expression that represents a single argument, but (1, 2) is not a valid Ruby expression, hence the error.
Note that the problem does not occur with single argument methods (as shown with our timesTwo method above), since the single value is a valid expression (e.g., (5) is a valid expression which simply evaluates to 5).
52) Is the line of code below valid Ruby code? If so, what does it do? Explain your answer?
Answer-
Yes, it’s valid. Here’s how to understand what it does:
The -> operator creates a new Proc, which is one of Ruby’s function types. (The -> is often called the “stabby proc”. It’s also called the “stabby lambda”, as it creates a new Proc instance that is a lambda. All lambdas are Procs, but not all Procs are lambdas. There are some slight differences between the two.)
This particular Proc takes one parameter (namely, a). When the Proc is called, Ruby executes the block p a, which is the equivalent of puts(a.inspect) (a subtle, but useful, difference which is why p is sometimes better than puts for debugging). So this Proc simply prints out the string that is passed to it.
You can call a Proc by using either the call method on Proc, or by using the square bracket syntax, so this line of code also invokes the Proc and passes it the string “Hello World”.
So putting that all together, this line of code (a) creates a Proc that takes a single parameter a which it prints out and (b) invokes that Proc and passes it the string “Hello world”. So, in short, this line of code prints “Hello World”.
53) Explain each of the following operators and how and when they should be used: ==, ===, eql?, equal?
== – Checks if the value of two operands are equal (often overridden to provide a class-specific definition of equality).
=== – Specifically used to test equality within the when clause of a case statement (also often overridden to provide meaningful class-specific semantics in case statements).
eql? – Checks if the value and type of two operands are the same (as opposed to the == operator which compares values but ignores types). For example, 1 == 1.0 evaluates to true, whereas 1.eql?(1.0) evaluates to false.
equal? – Compares the identity of two objects; i.e., returns true iff both operands have the same object id (i.e., if they both refer to the same object). Note that this will return false when comparing two identical copies of the same object.
54) Explain the difference between:
x += " world"
and
x.concat " world"
Answer-
The += operator re-initializes the variable with a new value, so a += b is equivalent to a = a + b.
Therefore, while it may seem that += is mutating the value, it’s actually creating a new object and pointing the the old variable to that new object.
This is perhaps easier to understand if written as follows:
(Examining the object id of foo and foo2 will also demonstrate that new objects are being created.)
The difference has implications for performance and also has different mutation behavior than one might expect.
55) In Ruby code, you quite often see the trick of using an expression like array.map(&:method_name) as a shorthand form of array.map { |element| element.method_name }. How exactly does it work?
When a parameter is passed with & in front of it (indicating that is it to be used as a block), Ruby will call to_proc on it in an attempt to make it usable as a block. Symbol#to_proc quite handily returns a Proc that will invoke the method of the corresponding name on whatever is passed to it, thus enabling our little shorthand trick to work.
56) Write a single line of Ruby code that prints the Fibonacci sequence of any length as an array?
(Hint: use inject/reduce)
Answer- There are multiple ways to do this, but one possible answer is:
(1..20).inject( [0, 1] ) { | fib | fib << fib.last(2).inject(:+) }
As you go up the sequence fib, you sum, or inject(:+), the last two elements in the array and add the result to the end of fib.
Note: inject is an alias of reduce
57) Why Ruby Is Known As A Language Of Flexibility?

Ruby is known as a language of flexibility because it facilitates its author to alter the programming elements. Some specific parts of the language can be removed or redefined. Ruby does not restrict the user. For example, to add two numbers, Ruby allows to use + sign or the word ‘plus’. This alteration can be done with Ruby’s built-in class Numeric.
58) Mention What Is The Difference Between A Gem And A Plugin In Ruby?
Gem: A gem is a just ruby code. It is installed on a machine, and it’s available for all ruby applications running on that machine.
Plugin: Plugin is also ruby code, but it is installed in the application folder and only available for that specific application.
59) Explain About Garbage Collection Feature Of Ruby?
Garbage collection is a process of reclaiming the memory space. Ruby deletes unallocated and unused objects automatically. This feature can be controlled by applying proper syntax and program through ruby.
Ruby performs garbage collection automatically. Ruby is an object oriented language and every object oriented language tends to allocate many objects during execution of the program.
60) Explain About Float, Dig And Max?
Float class is used whenever the function changes constantly. It acts as a sub class of numeric. They represent real characters by making use of the native architecture of the double precision floating point.
Max is used whenever there is a huge need of Float.
Dig is used whenever you want to represent a float in decimal digits.
61) What Is The Use Of Interpolation In Ruby?
Interpolation is a process of inserting a string into a literal. It is a very important process in Ruby. A string can be interpolated into a literal by placing a hash (#) within {} open and close brackets.
62) What Is The Use Of Load And Require In Ruby?
In Ruby, load and require both are used for loading the available code into the current code. In cases where loading the code required every time when changed or every times someone hits the URL, it is suggested to use ‘load’. It case of autoload, it is suggested to use ‘require’.
63) Explain About Ruby Code Blocks?
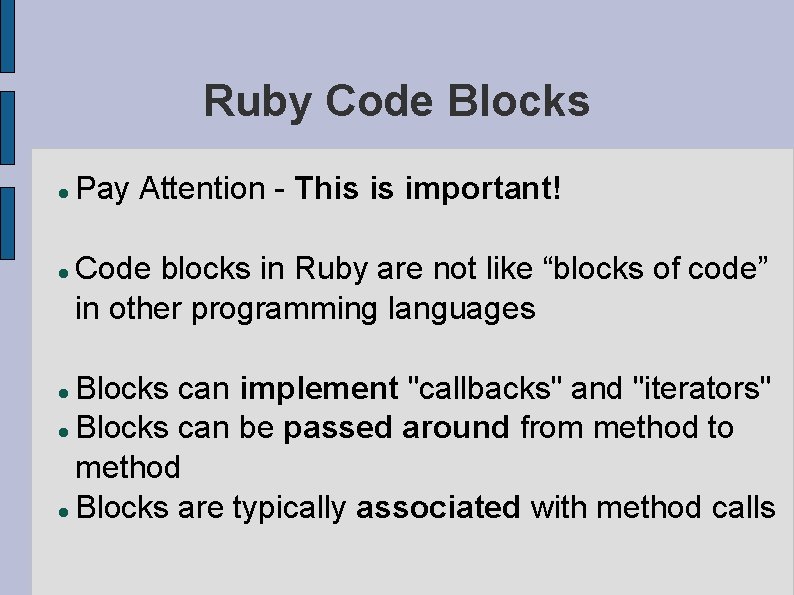
Ruby code blocks form an important part of ruby and are very fun to use. With the help of this feature you can place your code between do-end and you can associate them with method invocations and you can get an impression that they are like parameters. They may appear near to a source of the code and adjacent to a method call. The code is not executed during the program execution but it is executed when the context of its appearance is met or when it enters a method.
64) What Is Rails?
Ruby on Rails is a web application framework. It is written in Ruby. Compare to other frameworks for web application, the big deal is the way Ruby on Rails does. A web application finished in days instead of weeks, it is noticed by the community. Maintenance and/or extension of messy and hard web applications is flexible with ruby rails.
65) How Does Ruby Deal With Extremely Large Numbers?
Unlike other programming languages ruby deals with extremely large numbers it doesn’t have any barriers. There is no limit on the extent of limit of number usage. Ruby performs this function with two different classes they are fixnum and bignum. Fixnum represents easily managed small numbers and bignum represents big numbers. Ruby entirely handles the functioning of these two classes which leaves a programmer to concentrate on his arithmetic operations.
66) Explain About Class Variable And Global Variable?
A class variable starts with an @@ sign which is immediately followed by upper or lower case letter. You can also put some name characters after the letters which stand to be a pure optional. A class variable can be shared among all the objects of a class. A single copy of a class variable exists for each and every given class.
67) Explain About Ruby Names?
Classes, variables, methods, constants and modules can be referred by ruby names. When you want to distinguish between various names you can specify that by the first character of the name. Some of the names are used as reserve words which should not be used for any other purpose. A name can be lowercase letter, upper case letter, number, or an underscore, make sure that you follow the name by name characters.
68) Explain About Normal Method Class?
This function calls a method and it can take any number of arguments and expr. Make sure that you put an asterisk or an ampersand before the expression. Last expr argument can be declared with a hash without any braces. If you want to increase the size of the array value then make sure that you put an asterisk before expression. “::” can be used to separate the class from methods.
69) Explain About The Command Line Options?
Ruby`s language is executed from the command line like most of the scripting languages. Programming and behavior language environment can be controlled from the interpreter itself. Some of the commands which are used are as follows –d, -h, -e prog, -v, -T, -r lib, etc.
70) Mention What Is The Difference Between Procs And Blocks?
The difference between Procs and Blocks,
Block is just the part of the syntax of a method while proc has the characteristics of a block.
Procs are objects, blocks are not.
At most one block can appear in an argument list.
Only block is not able to be stored into a variable while Proc can
71) Explain How Can We Define Ruby Regular Expressions?
Ruby regular expression is a special sequence of characters that helps you match or find other strings. A regular expression literal is a pattern between arbitrary delimiters or slashes followed by %r.
72) Mention What Is The Command To Create A Migration?
To create migration command includes
C:rubyapplication>ruby script/generate migration table_name.
73) How Would You Create Getter And Setter Methods In Ruby?
Setter and getter methods in Ruby are generated with the attr_accessor method. attr_accessor is used to generate instance variables for data that’s not stored in your database column.
You can also take the long route and create them manually.
74) How Does A Symbol Differ From A String?
symbols are immutable and reusable, retaining the same object_id.
Be prepared to discuss the benefits of using symbols vs. strings, the effect on memory usage, and in which situations you would use one over the other.
75) What Are The Three Levels Of Method Access Control For Classes And What Do They Signify? What Do They Imply About The Method?
Public, protected, and private.
Public methods can be called by all objects and subclasses of the class in which they are defined in.
Protected methods are only accessible to objects within the same class.
Private methods are only accessible within the same instance.
76) Explain Some Of The Looping Structures Available In Ruby?
Answer :
For loop, While loop, Until Loop.
77) What Is The Difference Between A Class And A Module?
Answer :
A module cannot be subclassed or instantiated, and modules can implement mixins.
78) Name the three levels of access control for Ruby methods?
Answer- In Ruby, methods may either be public, protected, or private.
Public methods enforce no access control — they can be called in any scope.
Protected methods are only accessible within their defining class and its subclasses.
Private methods can only be accessed and viewed within their defining class . They are only accessible within the context of the current object.
79) What are some advantages of using Ruby?
Ans- You want a programmer who can really play to the strengths of the Ruby programming language. Here are some of the key advantages of this language:
Pure Object-Oriented Language: Everything in Ruby is an object—even methods, classes, and booleans. This greatly simplifies things from the coder’s perspective and opens up a range of possibilities.
Open-Source: Ruby is 100% free and open-source, with a large and enthusiastic community that can be tapped into as a resource.
Metaprogramming: Ruby is widely considered to be one of the best programming languages out there for metaprogramming, or the ability to write code that can act on other code instead of data.
Clean and Simple Syntax: The syntax is simple and concise, which allows developers to solve complex programs with fewer lines of code. It also helps that the code is human readable, and easy to follow.
80) How would you freeze an object in Ruby? Can you provide a simple example?
Ans- Sometimes it can be useful to prevent an object from being changed. This can be accomplished using the freeze method (Object.freeze) as in the sample code below.
water.freeze
if( water.frozen? )
puts “Water object is a frozen object”
else
puts “Water object is a normal object”
end
81) Ruby provides four types of variables. List them and provide a brief explanation for each?
Ans-The four types of variables in Ruby are as follows:
Global variables begin with $ and are accessible from anywhere within the Ruby program regardless of where they are declared—it stands to reason that they must be handled with care.
Local variables begin with a lowercase letter or an underscore. The scope of a local variable is confined to the code construct within which it is declared.
Class variables begin with @@ and are shared by all instances of the class that it is defined in.
Instance variables begin with @ and are similar to class variables except that they are local to a single instance of a class in which they are instantiated.
82) Can you explain the role of thread pooling in relation to the thread lifecycle in Ruby?
Ans- In Ruby, the lifecycle of a single thread starts automatically as soon as CPU resources are available. The thread runs the code in the block where it was instantiated and obtains the value of the last expression in that block and returns it upon completion. Threads use up resources, but running multiple threads at a time can improve an app’s performance. Thread pooling is a technique wherein multiple pre-instantiated reusable threads are left on standby, ready to perform work when needed. Thread pooling is best used when there are a large number of short tasks that must be performed. This avoids the overhead of having to create a new thread every time a small task is about to be performed.
83) Can you explain how Ruby looks up a method to invoke?
Ans- Since Ruby is a pure object-oriented language, it’s important to make sure your developer thoroughly understands how objects work. The first place that Ruby looks for a method is in the object’s metaclass or eigenclass—the class that contains methods directly defined on the object. If the method cannot be found in an object’s metaclass, Ruby will then search for the method in the ancestors of an object’s class. The list of ancestors for any class starts with the class of the object itself, and climbs parent classes until it reaches the Object, Kernel, and BasicObject classes at the top of the Ruby class hierarchy. If Ruby cannot find the method, it will internally send another method aptly called “method_missing?” to the object class. Ruby will repeat another search for this method, and will at least find it in the object class, provided the programmer did not see fit to define the “method_missing?” class earlier in the ancestry of the object.
84) Find and fix the bug within the code below?
Answer- Normally if Ruby encounters an identifier, and the identifier does not reference a defined local variable, Ruby will try to call a method with the given name. However, when Ruby encounters address = a within the initialize method, it treats address = a as a local variable initialization and fails to invoke the setter. This occurs when Ruby encounters an identifier beginning with a lowercase character or underscore on the left-hand side of an assignment operator. The solution is to clarify that we want to call the writer method address = by prepending address with the self keyword. The initialize method has been reproduced below:
def initialize(a)
self.address = a
end
Alternatively, you could also fix the bug by directly assigning the value to the instance variable within the initialize method like so:
def initialize(a)
@address = clean(a)
end
85) What are blocks and procs?
A block is basically Ruby’s version of a closure—a block of code that can be wrapped up into a proc (a type of function) that can then be stored in a variable or passed to a method and run when desired. Blocks can syntactically be written as blocks of code between { } or the do and end keywords. The standard way to create a proc is depicted in the code block below.
> my_proc = Proc.new { |arg1| print "#{arg1}! " }
86) Predict the output of the code below. Explain your answer?
-> (s) {p s} [“I’m a Proc”]
Answer- This question highlights the syntactical elegance of Ruby. A seasoned coder can perform a lot with a single line of Ruby code. The -> operator, or “stabby proc” as it is often called, is a way to create a proc that is also a lambda, or nameless function. This proc takes the parameter s and executes the block {p s}, which is shorthand for puts(s.inspect) before passing the string “I’m a Proc” using the square bracket syntax in place of the typical “call” method. The result is an elegant single line of code that prints the string “I’m a Proc” to the console.
87) The .upcase and .capitalize method are used for capitalizing the whole string.
a) True
b) False
Answer- B
Explanation: The .capitalize method doesn’t change the whole string to uppercase, it just capitalizes the first letter.
88) What is the use of .capitalize method?
a) It capitalizes the entire string
b) It capitalize on the first letter of the string
c) It capitalize the strings which are in small case
d) All of the mentioned
Answer- Answer: b
Explanation: It capitalizes only the first letter.
89) What will be the values of:
var1 = A.a(0)
var2 = A.a(2)
Answer- var1 will be equal to nil
var2 will be equal to 4
Understand: * A conditional statement in Ruby is an expression that returns nil if the conditional is false. * Ruby methods return the last expression in the method body.
In this example:
A.a(0) returns nil for unsuccessful conditional
A.a(2) returns the square of 2, i.e 4
90) What is the difference between Array#map and Array#each?
Note: collect is an alias of map
Answer- Array#each method iterates over the elements of the array and executes the provided block each time. However, it returns the original array unaffected.
Array#map will return a new array of elements containing the values returned by the block it is provided. It also does not affect the original array
Example:
91) How do you remove nil values in array using ruby?
Array#compact removes nil values.
Example:
>> [nil,123,nil,"test"].compact
=> [123, "test"]
92) What is the return value for:
ABC::new::xyz
ABC::new.xyz
ABC.new::xyz
ABC.new.xyz
Answer-ANs- All the statements for the invocation of the xyz method through the object are valid.
When run through IRB:
93) What is the value of the variable upcased in the below piece of code?
upcased = ["one", "two", "three"].map {|n| puts n.upcase }
Answer- upcase = [nil, nil, nil]
Let’s take a look at puts as below:
>> puts "Hi"
Hi
=> nil
Note the nil at the end: that’s the return value from puts. After all, puts is a method, so it has to return something. As it happens, it always returns nil. The printing out of the string is an action the method performs.
Similarly, evaluating the code in question we can understand that while it’s a common learner mistake to expect the result to be ["ONE", "TWO", "THREE"]. In fact, it’s [nil, nil, nil]. Each time through the block, the block evaluates to the return value of puts to be nil.
94) What will be the value of:
defined? foo
defined? bar
Answer-
foo has been at least read by the interpreter, therefore it is defined and assigned a nil value. However, since bar has never been written, it was never defined in the first place.
95) What is the difference between the Object methods clone and dup?
Ans- Object#dup creates a shallow copy of an object. For example, it will not copy any mixed-in module methods, whereas Object#clone will. This can be shown with the following code example:
96) What is the difference between extend and include in ruby?
Ans- include mixes in specified module methods as instance methods in the target class
extend mixes in specified module methods as class methods in the target class
Given the following class definitions:
Here’s how ClassThatIncludes behaves:
Here’s how ClassThatExtends behaves:
We should mention that object.extend ExampleModule makes ExampleModule methods available as singleton methods in the object.
97) How many types of variables are used in Ruby and what are they?
There are 4 types of variables used in Ruby:
class variables start with @@, e.g. @@my_var
instance variables start with @, e.g. @my_var
global variables start with $, e.g. $my_var
local variables are not prefixed, but method arguments and block arguments start with , e.g. _my_arg (in fact, local variables that are prefixed with are considered by linters like RuboCop to be useless variables, where you don’t care what the value is).
98) Describe a closure in Ruby?
Answer-
A closure is an object that is both an invocable function together with a variable binding. The object retains access to the local variables that were in scope at the time of the object definition.
A closure in Ruby retain variables by reference; the closure also extends the lifetimes of its variables. A closure’s reference to its variables is dynamically bound that means the values of the variables are resolved when the Proc object is executed.
It also possible to alter a closure. The binding of a closure can be altered using #binding.
99) How does Ruby on Rails use the Model View Controller (MVC) framework?
Answer–
Web development can often be divided into three separate but closely integrated subsystems:
Model (Active Record): The model handles all the data logic of the application. In Rails, this is handled by the Active Record library, which forms the bridge between the Ruby program code and the relational database.
View (Action View): The view is the part of the application that the end user sees. In Rails, this is implemented by the Action View library, which is based on Embedded Ruby (ERB) and determines how data will be presented.
Controller (Action Controller): The controller is like the data broker of an application, handling the logic that allows the model and view to communicate with one another. This is called the Action Controller in Rails.
100) What is the difference between throw/catch and raise/rescue?
Answer– Throw and catch accept matching symbols as arguments and should be considered a control-flow structure where raise and rescue is used to raise and handle exceptions. throw and catch are not commonly used while exception handling with raise and rescue is used often.
- Mastering Qualitative Research: The Role of Focus Groups in Data Collection - July 11, 2024
- What is robots ops? - July 10, 2024
- 5 Effective Online Learning Strategies for DevOps Professionals - July 4, 2024